In C++, files and streams provide a way to read from and write to files using stream classes like `ifstream` and `ofstream`, facilitating data input and output operations.
Here's a simple code snippet to demonstrate reading from a file and writing to another file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("input.txt");
std::ofstream outputFile("output.txt");
std::string line;
while (std::getline(inputFile, line)) {
outputFile << line << std::endl;
}
inputFile.close();
outputFile.close();
return 0;
}
Understanding C++ Streams
What are C++ Streams?
In C++, streams are abstractions used for reading and writing data. They represent a sequence of characters flowing into or out of your program. Streams can be classified into two main categories: input streams, which are used for reading data, and output streams, which are used for writing data.
C++ provides several built-in stream classes, including:
- `iostream` for standard input and output.
- `ifstream` for input file stream operations.
- `ofstream` for output file stream operations.
Understanding how these streams work is essential for effectively handling files and streams in C++.
Stream Buffers
At the core of stream handling is the buffer mechanism, which temporarily holds data before it's read from or written to an external source (such as a file). Buffers help in improving I/O performance by minimizing the number of system calls.
For instance, when writing to a file, rather than writing each character immediately, the data is collected in a buffer and written out in one go. This reduces the overhead associated with frequent writes to the filesystem.
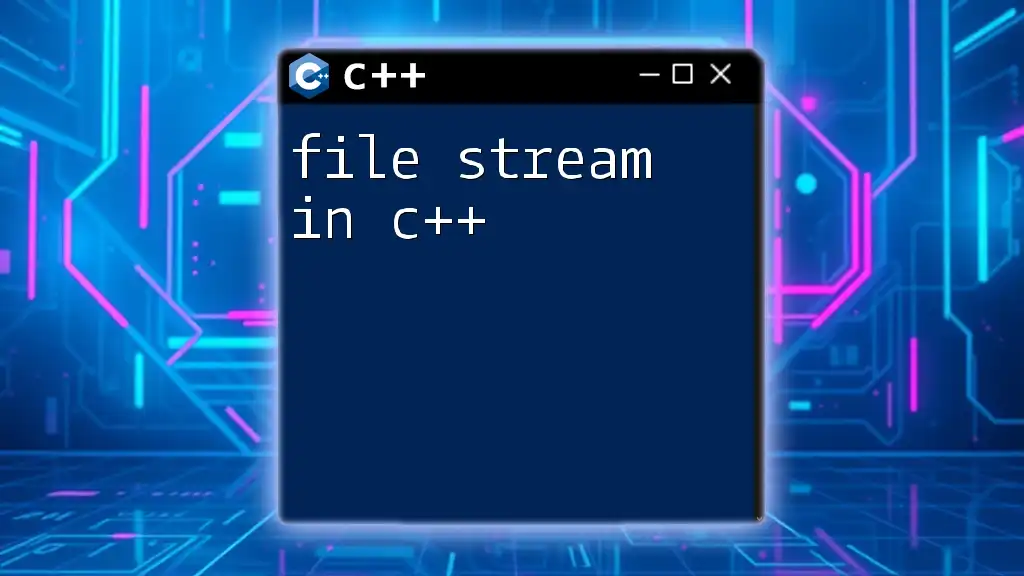
Working with Files in C++
Opening and Closing Files
To interact with files, you first need to open them. In C++, you can open a file using the `open()` method of the `ifstream` and `ofstream` classes. For a safe exit from file operations, it's also crucial to close any opened files using the `close()` method.
Here’s a simple example of opening and closing a file:
#include <fstream>
using namespace std;
int main() {
ofstream outputFile;
outputFile.open("example.txt");
if (outputFile.is_open()) {
outputFile << "Hello, World!";
outputFile.close();
}
return 0;
}
Modes of File Operations
When opening a file, you can specify the mode you want to work in. This controls whether you’re reading from, writing to, or appending to the file. The common modes include:
- `ios::in`: Opens a file for reading.
- `ios::out`: Opens a file for writing.
- `ios::app`: Appends data to the end of the file.
- `ios::binary`: Opens a file in binary mode, which is essential for non-text files (like images or executables).
Indeed, understanding how modes work is crucial when managing files and streams in C++ effectively. Here is an example of how to specify modes when opening a file:
ifstream inputFile("example.txt", ios::in); // Reading mode
ofstream outputFile("example.txt", ios::app); // Append mode
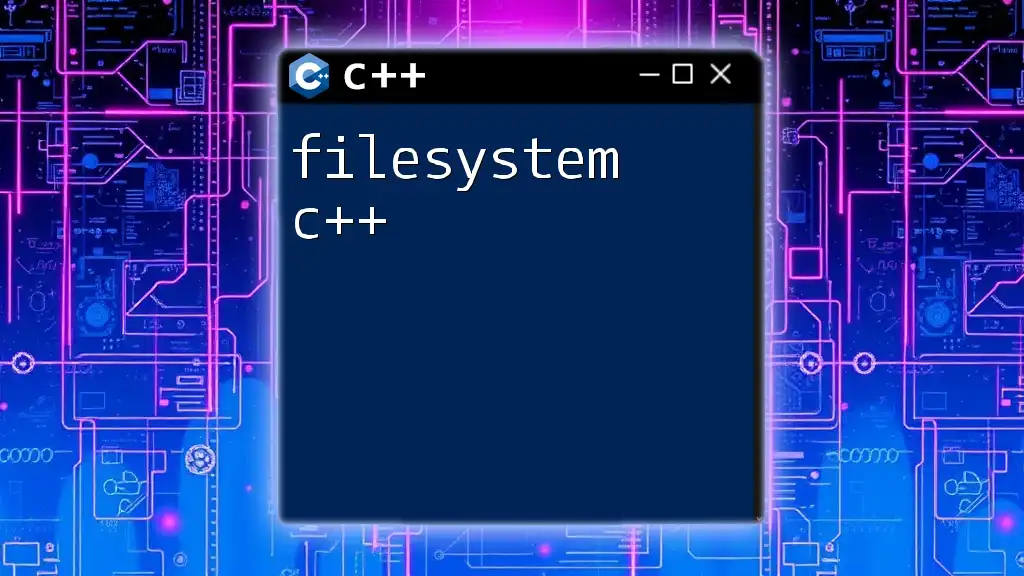
Reading from Files
Input File Streams
To read data from files, you utilize the `ifstream` class. This class provides several methods for retrieving data, including the extraction operator `>>` and `getline()` for reading lines.
Consider the code below, which demonstrates reading strings from a text file:
#include <fstream>
#include <string>
#include <iostream>
using namespace std;
int main() {
ifstream inputFile("example.txt");
string line;
while (getline(inputFile, line)) {
cout << line << endl;
}
inputFile.close();
return 0;
}
This code reads through each line of "example.txt" and outputs it to the console. It’s important to close the file afterward to avoid file corruption or leaks.
Error Handling in File Operations
When dealing with file operations, robust error handling is vital. You should always check if the file opened successfully before attempting to read or write to it. The `fail()` function can be used to verify that the stream is in a valid state.
For example, you might handle errors like this:
if (!inputFile) {
cerr << "Error opening file!" << endl;
}
This simple check can save you from problems later in your program.
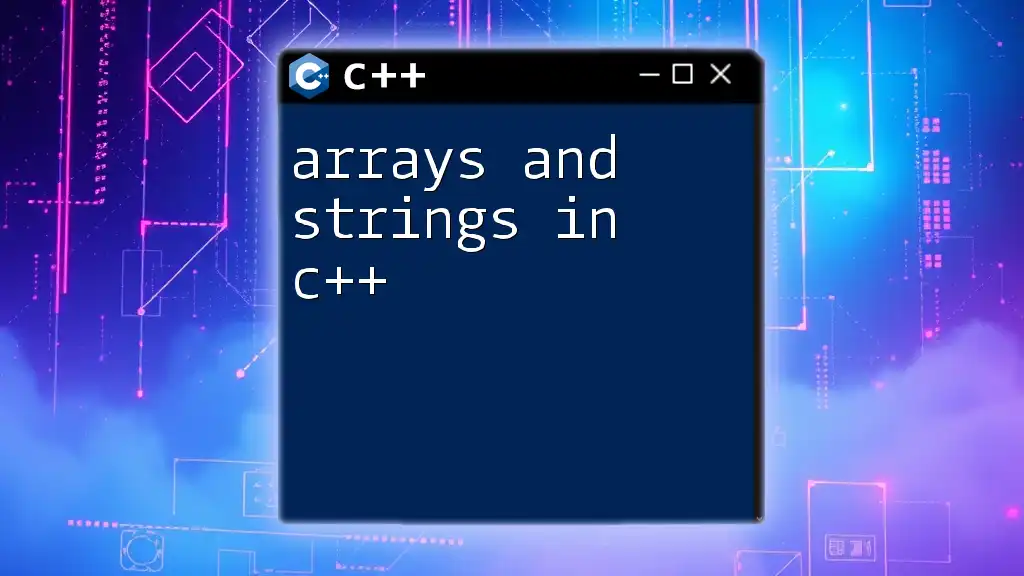
Writing to Files
Output File Streams
For writing data, the `ofstream` class comes into play. With `ofstream`, you can easily output data to a file using the insertion operator `<<`.
Below is a straightforward example showing how to write data into a file:
ofstream outputFile("output.txt");
outputFile << "Writing to a file\n";
outputFile << "Number: " << 42 << endl;
outputFile.close();
In this snippet, two pieces of data are written to `output.txt`, showcasing how C++ handles text output seamlessly.
Flushing and Clearing Buffers
Sometimes, you might want to flush the output buffer to ensure all data is written out immediately. Flushing a buffer can be necessary to confirm that all output is committed before carrying out further operations. This can be achieved using the `flush` manipulator.
Here’s an example:
outputFile << "This will be flushed immediately" << flush;
This line ensures that all preceding data is flushed to the file instantly, rather than waiting for the buffer to fill.
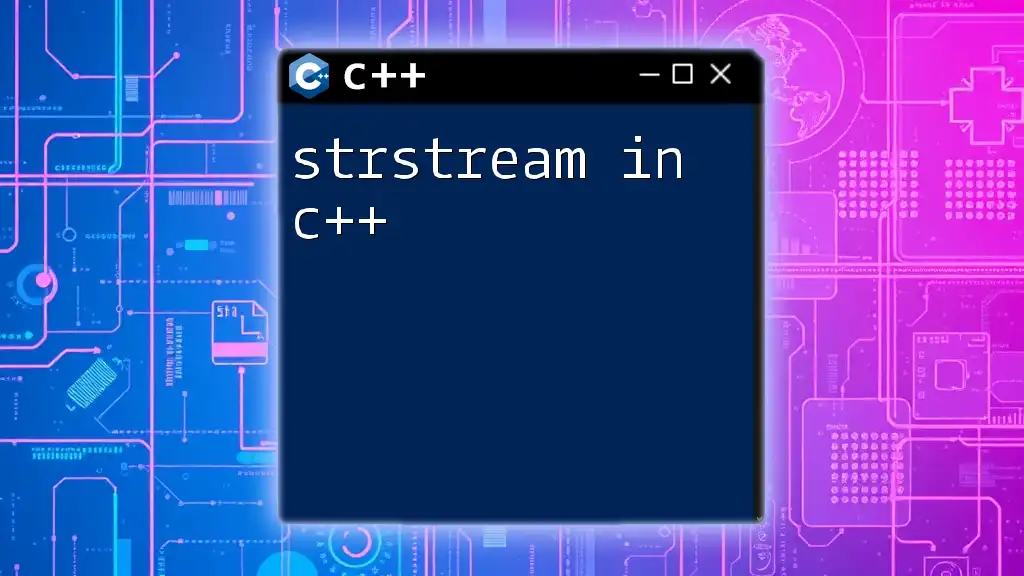
File Manipulation Functions
Seeking and Tellg Methods
Navigating through a file is made easy with the `seekg()` and `tellg()` functions. The `seekg()` function sets the position of the next character to be read, while `tellg()` returns the current position in the stream.
Consider the following example demonstrating file positioning:
inputFile.seekg(0, ios::beg); // Moves to the beginning of the file
This functionality is particularly useful when you need to read specific sections of a file without starting over from the beginning.
Deleting and Renaming Files
C++ also allows for file management operations such as deleting and renaming files. The `remove()` and `rename()` functions can be leveraged to manipulate files easily.
Here is a code example demonstrating how to delete a file:
#include <cstdio>
if (remove("example.txt") != 0) {
perror("Error deleting the file");
}
This code will attempt to remove "example.txt" and display an error if it cannot be found or deleted.
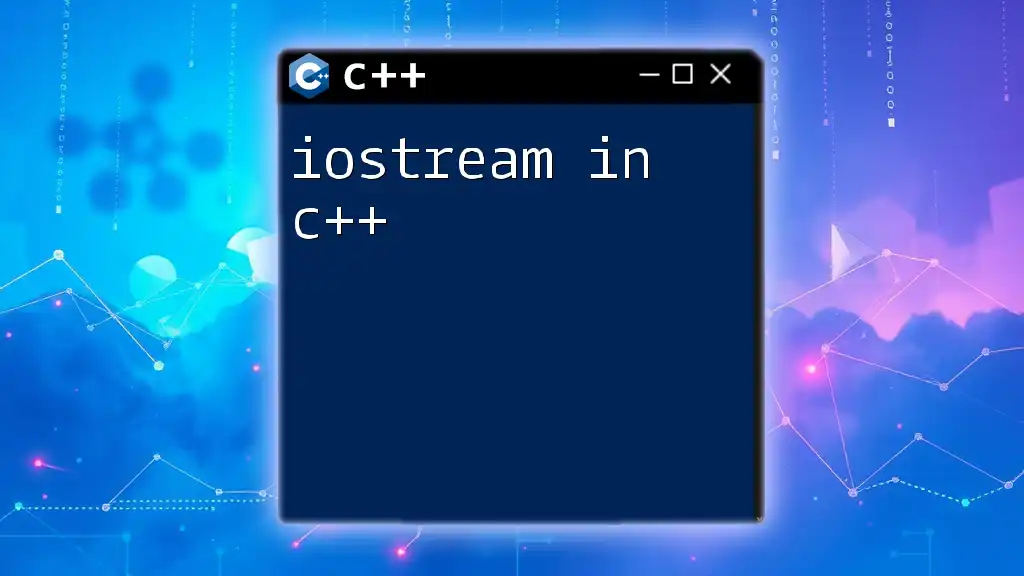
Working with Binary Files
Why Use Binary Files?
Binary files offer several advantages compared to text files; primarily, they can represent data in its native format without the need for conversion to strings. They are particularly useful for storing complex data structures, images, or any data that isn’t easily represented in text.
Writing and Reading Binary Data
With binary file handling, you can read and write data as it is stored in memory, which is particularly efficient. Below is a basic example of writing and reading a structure in binary format:
struct Data { int id; double value; };
ofstream binaryFile("data.bin", ios::binary);
Data d = {1, 99.99};
binaryFile.write(reinterpret_cast<char*>(&d), sizeof(d));
binaryFile.close();
Reading from a Binary File
You can subsequently read this binary data back into a structure:
ifstream binaryFile("data.bin", ios::binary);
Data d;
binaryFile.read(reinterpret_cast<char*>(&d), sizeof(d));
cout << "ID: " << d.id << ", Value: " << d.value << endl;
binaryFile.close();
This method confirms the efficiency and directness of handling binary data in files and streams in C++.
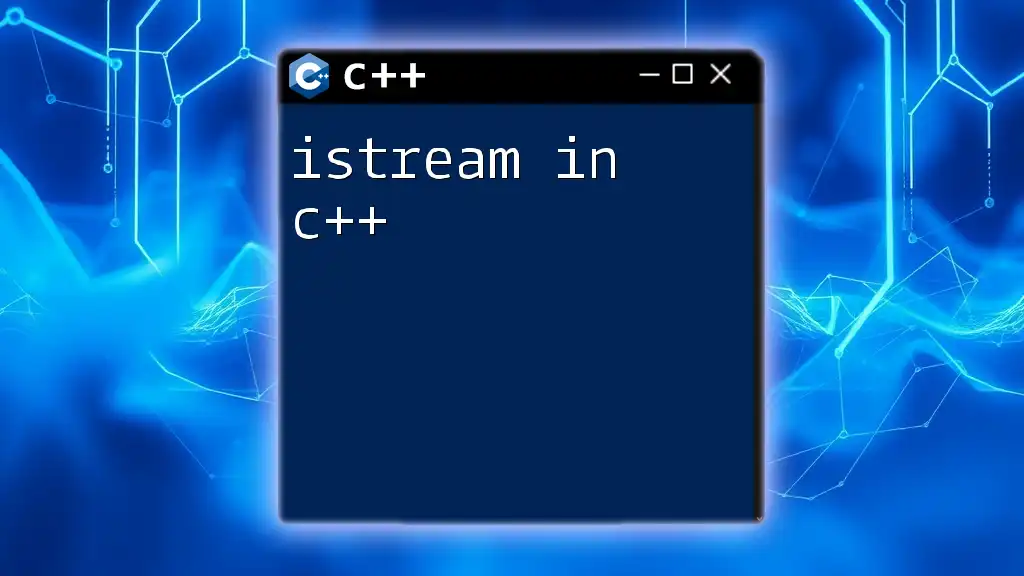
Conclusion
In conclusion, understanding files and streams in C++ is critical for effective programming. Mastery of file handling—opening, reading from, writing to, and manipulating files—opens up various possibilities for data processing. Embrace the concepts discussed here and practice them for more profound insights and capabilities in C++. Whether it’s managing text or binary files, becoming adept at these operations adds significant value to your C++ programming toolkit.