File streams in C++ allow you to read from and write to files using the `fstream` library, enabling easy manipulation of file data.
#include <fstream>
#include <iostream>
int main() {
std::ofstream outFile("example.txt"); // create and open a text file
outFile << "Hello, file!"; // write to the file
outFile.close(); // close the file
std::ifstream inFile("example.txt"); // open the file for reading
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl; // read the file line by line
}
inFile.close(); // close the file
return 0;
}
Understanding Streams in C++
In C++, a stream represents an abstraction that is used for input and output operations. By using streams, you can read data from input devices (like keyboards) and write data to output devices (such as displays or files). The use of streams simplifies the process of data handling by providing a consistent and unified interface.
C++ supports several types of streams:
- Input streams (`istream`) for reading data.
- Output streams (`ostream`) for writing data.
- Bidirectional streams (`iostream`) that allow both reading and writing.
With the concept of streams, C++ makes file handling operations intuitive and manageable, enabling you to focus more on your application's functionality rather than the complexities of data manipulation.
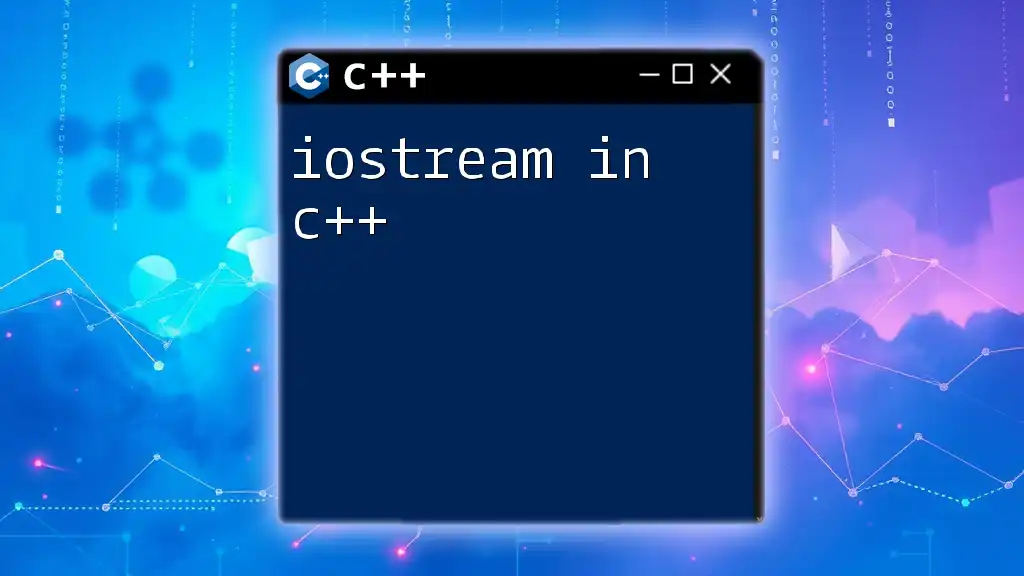
The C++ Standard Library and File Streams
To work with file streams in C++, you primarily rely on the `<fstream>` library. This library provides the essential classes for file operations, such as `ifstream` for input file streams, `ofstream` for output file streams, and `fstream` for bidirectional streams.
To utilize the functionalities of file streams, always include the `<fstream>` header at the beginning of your program:
#include <fstream>
The availability of these classes allows you to easily open, read, write, and close files, streamlining the file handling process in your applications.
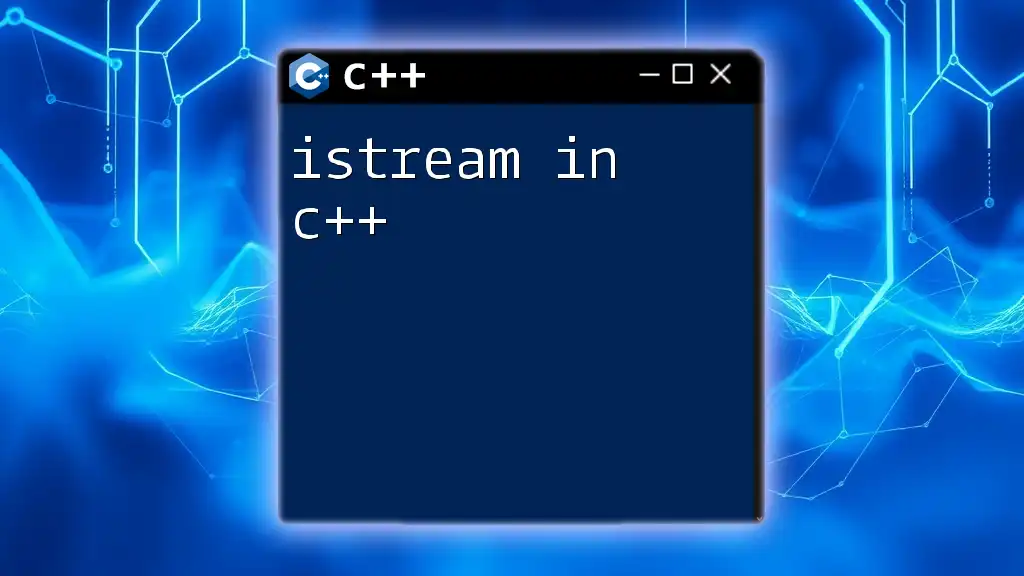
Creating File Streams
Opening a File
To work with a file, the first step is to open it. C++ provides a straightforward syntax through the `ifstream` (input file stream) and `ofstream` (output file stream) classes.
Here’s a simple example demonstrating how to open a file:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inputFile("example.txt");
std::ofstream outputFile("output.txt");
if (!inputFile) {
std::cerr << "Error opening input file" << std::endl;
}
if (!outputFile) {
std::cerr << "Error opening output file" << std::endl;
}
return 0;
}
It's crucial to always check whether the file has been successfully opened, as this allows you to handle errors appropriately.
Modes of File Operations
When opening a file, you can specify different modes that determine how you will interact with the file. Common modes include:
- `ios::in`: Opens a file for reading.
- `ios::out`: Opens a file for writing. If the file already exists, its content will be erased.
- `ios::app`: Opens a file for writing, appending new data at the end without deleting existing data.
Choosing the correct mode is critical to your file operations’ success and ensures that you don't unintentionally lose data. Here's how you can specify the mode while opening a file:
std::ofstream outputFile("example.txt", std::ios::app); // Opens in append mode
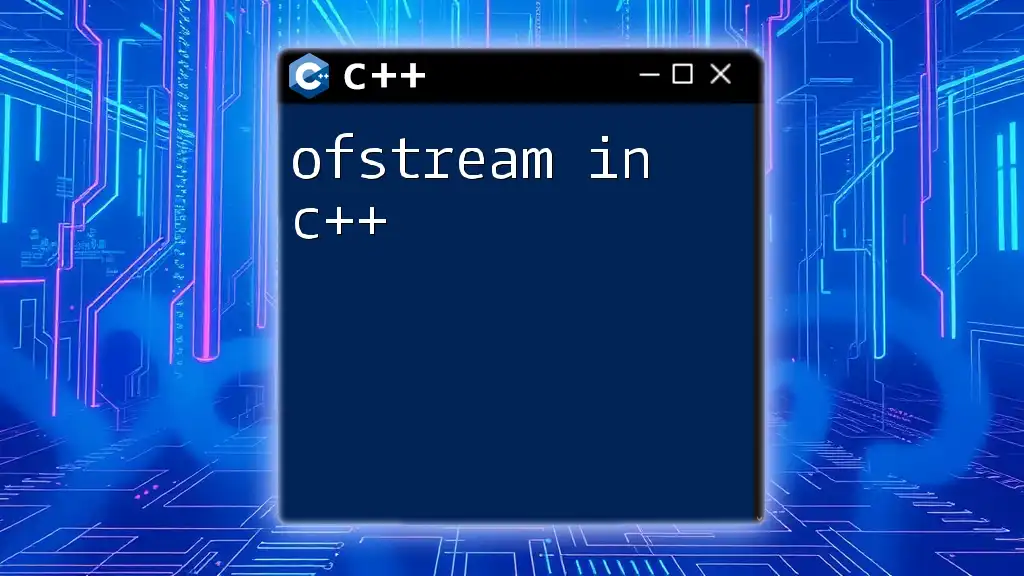
Reading from Files
Basic File Reading Techniques
To read data from files in C++, you have two primary approaches: using `getline()` for lines or the extraction operator (`>>`) for formatted input.
The `getline()` function reads an entire line from a file, which is particularly useful for processing text files. Here’s an example demonstrating how to read lines from a file:
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
Alternatively, the extraction operator can be used for more structured data input. Here’s a quick example:
int number;
while (inputFile >> number) {
std::cout << "Read number: " << number << std::endl;
}
In both cases, ensure proper error handling to catch issues during the reading process.
Reading Binary Files
When it comes to binary files, the reading process differs from text files. Binary files store data in a format that is not human-readable, making it essential to open the file in binary mode. Here’s how to read a binary file:
std::ifstream binaryFile("example.bin", std::ios::binary);
char buffer[128];
binaryFile.read(buffer, sizeof(buffer));
Reading binary files is useful when dealing with non-textual data, such as images or custom data structures.
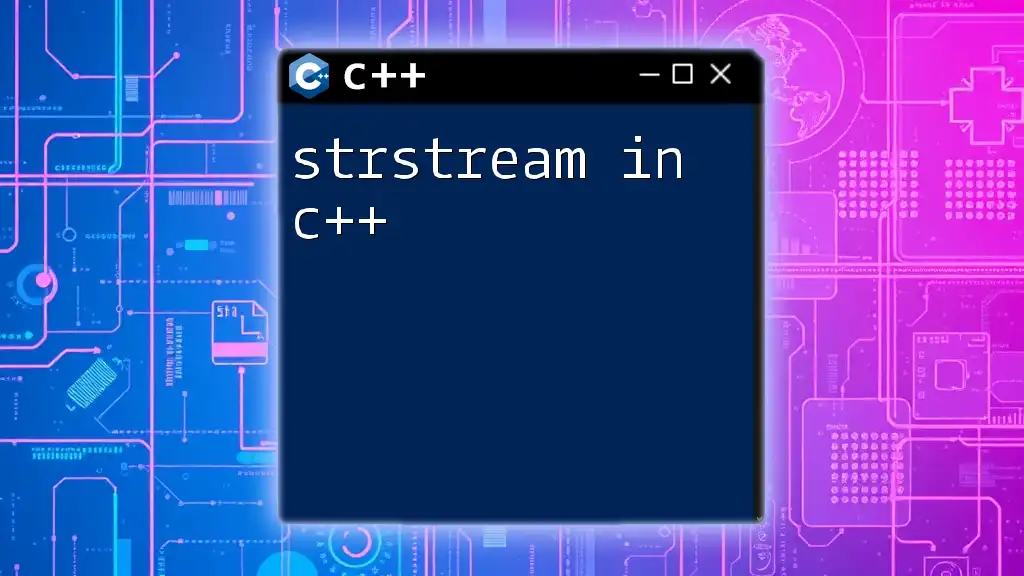
Writing to Files
Basic File Writing Techniques
To write data to files, you typically use the insertion operator (`<<`). Here’s an example of writing text to a file:
outputFile << "Hello, C++ File Streams!" << std::endl;
It's a common practice to flush the stream to ensure that all buffered output is written to the file promptly, particularly in performance-sensitive applications.
Writing Binary Data
When working with binary data, you can write directly to files using the `write()` function. Here's an example code snippet for writing an integer to a binary file:
std::ofstream binaryOutput("example.bin", std::ios::binary);
int number = 42;
binaryOutput.write(reinterpret_cast<char*>(&number), sizeof(number));
This method enables you to store data types in their raw binary form, which can be crucial for applications needing efficient data storage.
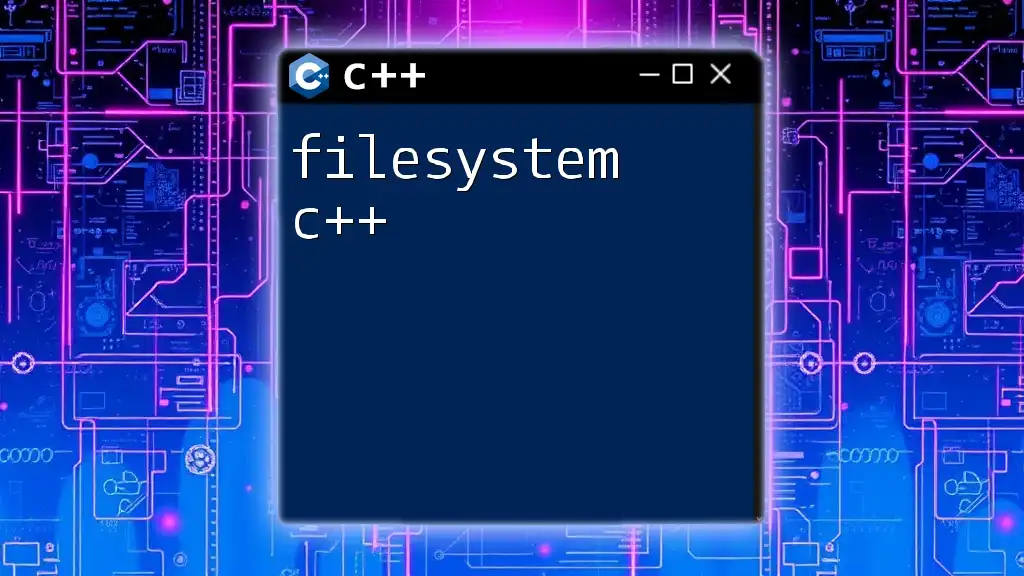
Closing File Streams
After you finish working with files, it’s essential to close them. Closing file streams releases the resources tied to the file and ensures that all data is properly saved. The syntax for closing file streams is straightforward:
inputFile.close();
outputFile.close();
Neglecting to close files can lead to memory leaks and data corruption, so make it a habit to close files once you're done.
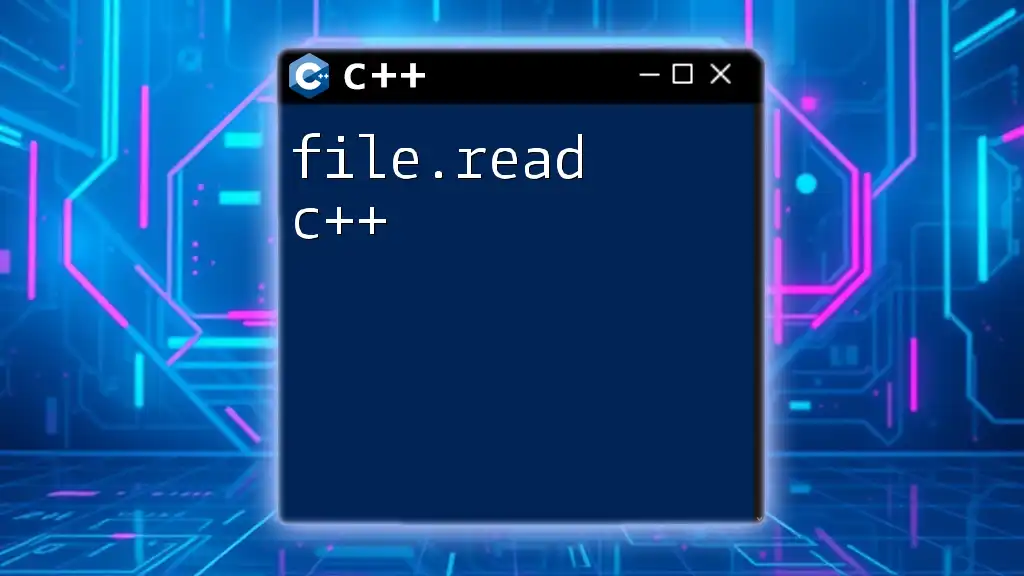
Error Handling in File Streams
Error handling in file streaming is critical for reliable applications. Common types of errors can occur during file opening, reading, or writing operations. C++ provides methods like `fail()`, `eof()`, and `bad()` to check the status of streams.
For example, checking whether a read operation was successful can be done as follows:
if (inputFile.fail()) {
std::cerr << "Error reading from the file." << std::endl;
}
Implementing robust error handling can help identify issues early and improve user experience.
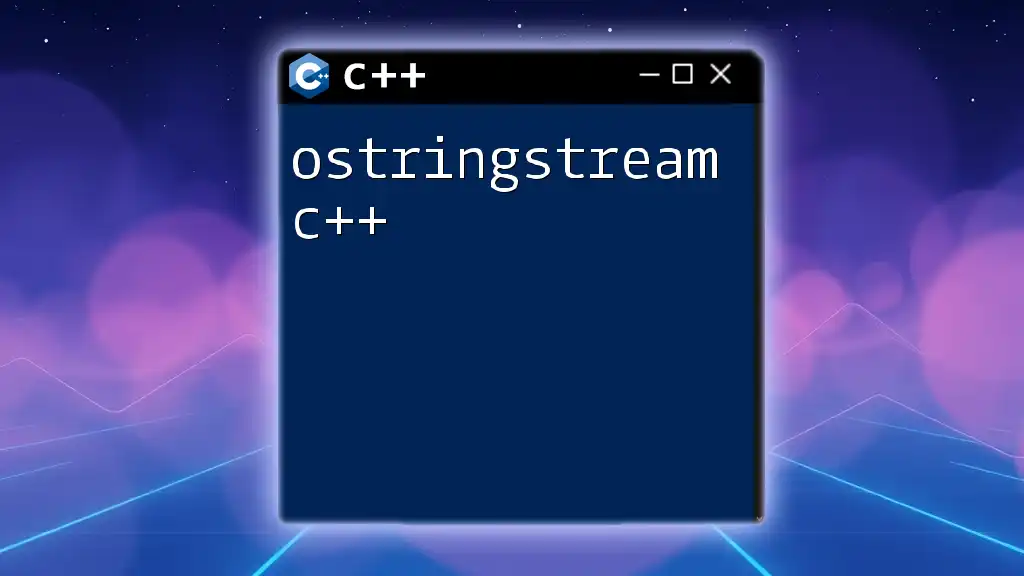
Best Practices for File Stream Management
When dealing with file streams in C++, consider the following best practices for optimal management:
- Always check if files open successfully.
- Close file streams after operations are complete to free up resources.
- Use RAII (Resource Acquisition Is Initialization) principles by managing file streams with automatic storage duration.
- When working with large files, optimize by reading and writing in chunks instead of line by line.
These practices not only enhance code quality but also ensure better performance and maintainability of your applications.
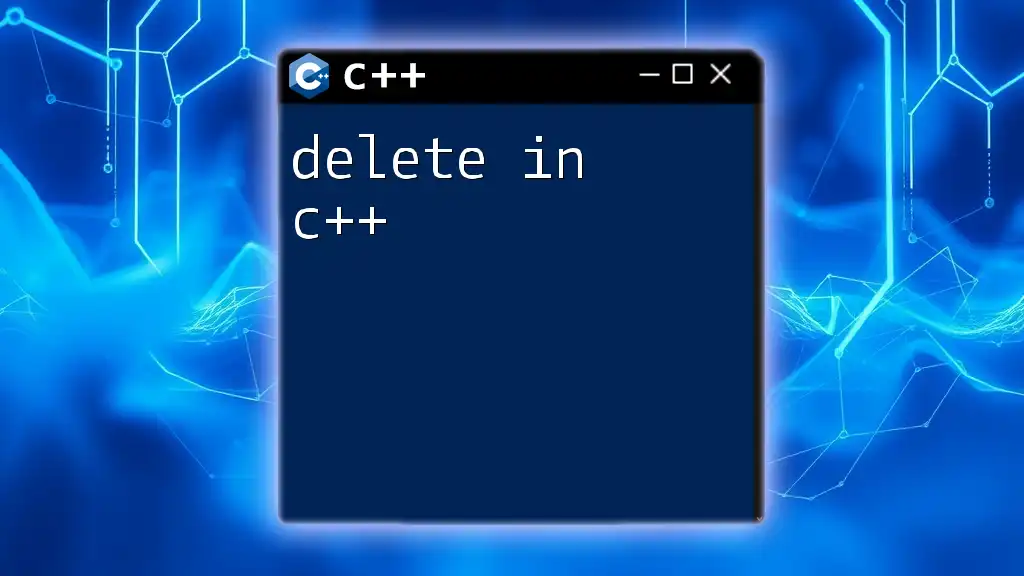
Conclusion
In conclusion, understanding and utilizing file streams in C++ can greatly enhance your programming capabilities. With the `<fstream>` library, you have powerful tools at your disposal to manage file operations. Remember to always check for errors, choose the correct file modes, and properly manage stream resources to make your applications robust and efficient.
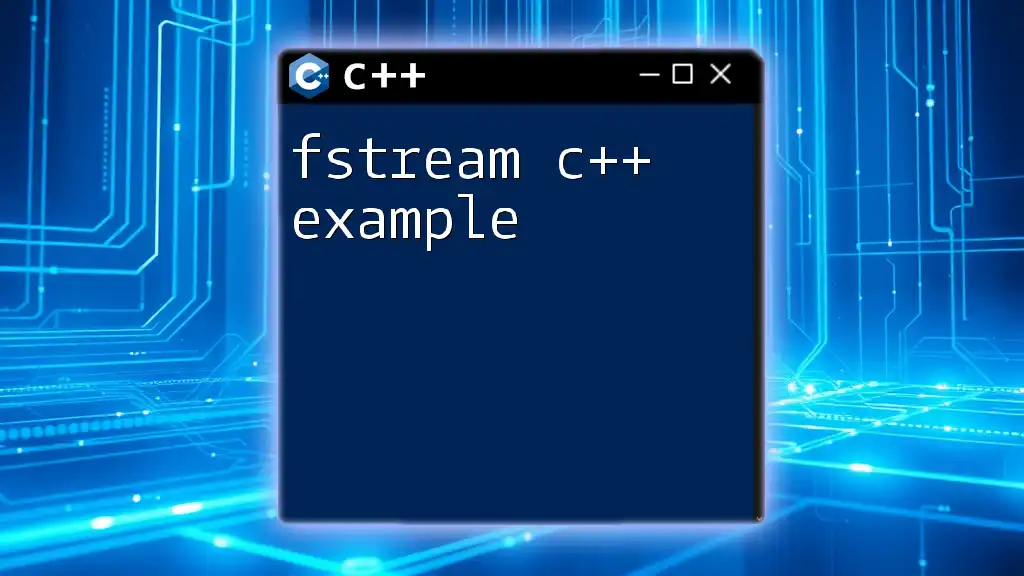
Additional Resources
For further learning, explore literature such as "C++ Primer" or online platforms like cppreference.com, which can provide deeper insights into file stream handling in C++.
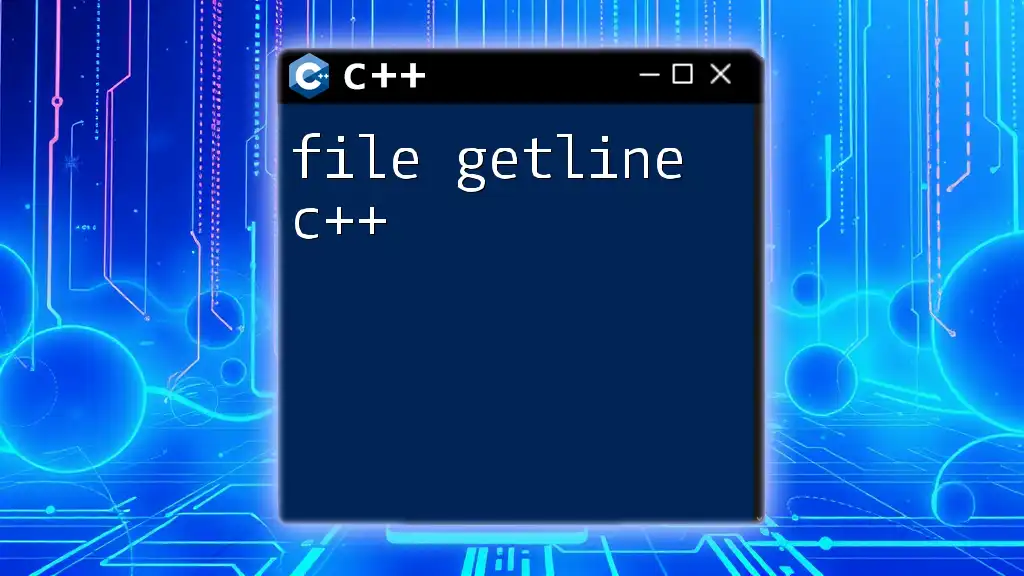
Call to Action
If you found this guide helpful, subscribe to our newsletter for more in-depth tutorials and courses that will help solidify your understanding of C++ and its myriad functionalities.