In C++, the "does not equal" comparison is represented by the `!=` operator, which checks if two values are not the same.
Here’s an example:
if (a != b) {
// This block executes if a is not equal to b
std::cout << "a is not equal to b" << std::endl;
}
Understanding the Not Equal Sign in C++
What Does "Does Not Equal" Mean in Programming?
In programming, the term "does not equal" refers to a comparison between two values or expressions, indicating whether they are unequal. This concept is essential for decision-making processes in code; without it, distinguishing between different states, values, or outputs would be extremely challenging.
Introduction to C++
C++ is a powerful general-purpose programming language known for its performance and efficiency. A fundamental aspect of developing in C++ is the ability to compare data. Comparing values helps in controlling the flow of the program, enabling you to execute certain segments of code based on specific conditions.
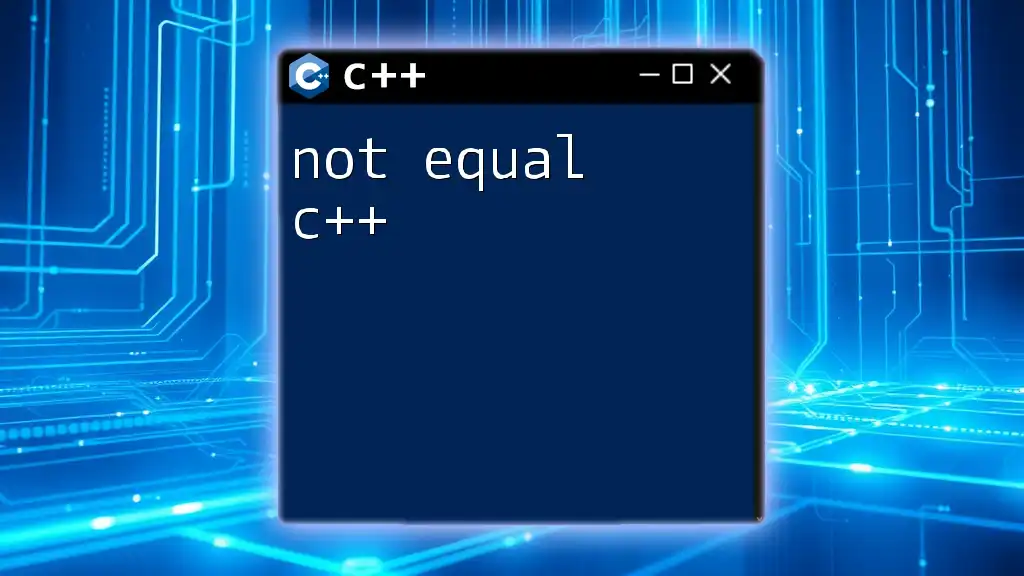
The Not Equal Operator in C++
Explanation of the Not Equal Operator
The not equal operator, denoted as `!=`, is used to compare two values to determine if they are unequal. It returns a boolean value: true if the values are not equal and false if they are. This operator is vital for performing comparisons and implementing logical conditions throughout your code.
How to Use the Not Equal Operator
Single Data Types
Using the not equal operator in basic data types, such as integers, is straightforward:
int a = 5;
int b = 10;
if (a != b) {
// code to execute if a is not equal to b
}
In this example, since `a` is not equal to `b`, the condition evaluates to true, and the code block inside the `if` statement executes. This kind of comparison is crucial in scenarios where you need to validate data or check for specific conditions during program execution.
Complex Data Types
The not equal operator can also be used with more complex data types, such as strings. Here’s an example:
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 != str2) {
// code to execute if str1 is not equal to str2
}
In this snippet, `str1` is compared to `str2`. Since they are not equal, the condition evaluates to true, executing the code inside the `if` block. Understanding this application is crucial for handling user inputs or comparing text, especially in scenarios involving conditions for executing specific functionalities.
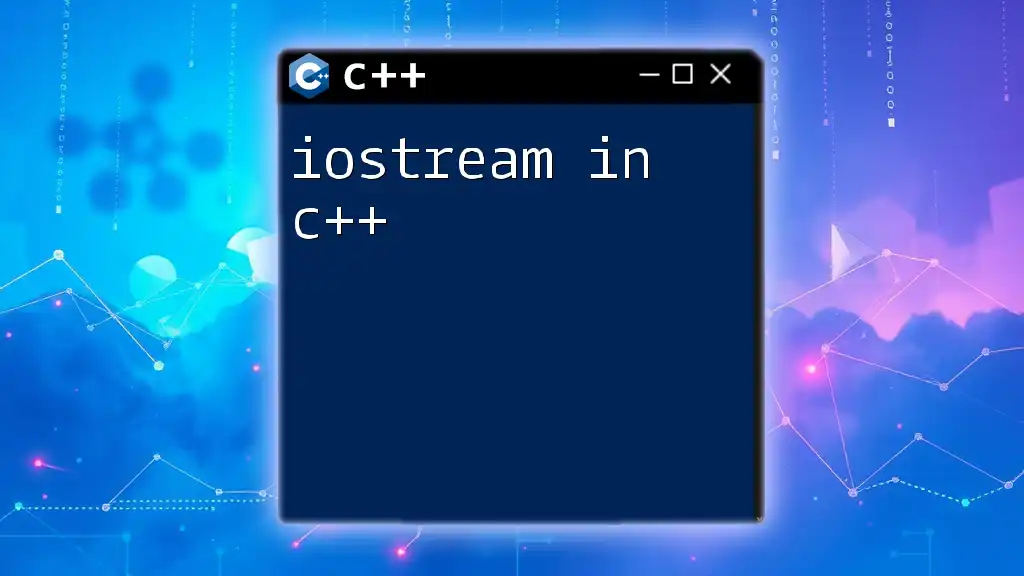
Practical Use Cases of the Not Equal Operator
Conditional Statements
The `!=` operator is often used within conditional statements to dictate program flow based on specific comparisons. For instance, validating user input or checking for specific conditions can greatly benefit from this operator. It's prevalent in error checking routines, where you may need to confirm that a user’s input does not match predefined invalid values.
Looping Constructs
Using Not Equal in Loops
Loops are another area where the not equal operator shines. Here’s an example using a `while` loop:
int i = 0;
while (i != 5) {
// code to execute until i equals 5
i++;
}
In this loop, the condition checks if `i` does not equal `5`. The loop will continue executing while this condition holds true. When `i` finally equals `5`, the loop terminates. This demonstrates how the not equal operator can assist in controlled repetition and help establish boundaries in execution.
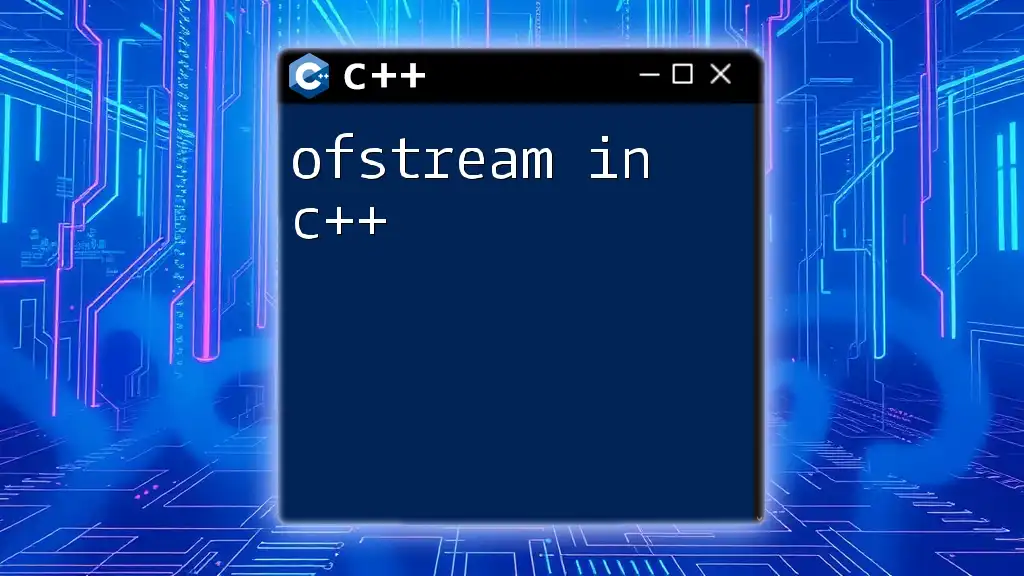
Best Practices for Using the Not Equal Operator in C++
Clarity in Code
When using the `!=` operator, the clarity of your code is paramount. Use descriptive variable names and provide context for comparisons. This practice not only enhances readability but also helps others (and your future self) understand the intent behind the comparisons at a glance. If readers can easily grasp the purpose of an inequality check, the code becomes more maintainable and less prone to errors.
Common Mistakes to Avoid
While using the not equal operator, developers may encounter several common pitfalls:
-
Accidental Assignment: One frequent mistake is confusing the not equal operator with the assignment operator. For example, using a single equals sign `=` instead of `!=` can lead to logical errors.
-
Floating Point Comparisons: Comparing floating-point numbers for inequality can result in unexpected behavior due to precision limitations. It is often advisable to implement a tolerance range when checking for equality with floats.
By being aware of these common mistakes and understanding best practices, you'll be better equipped to implement the not equal operator effectively.
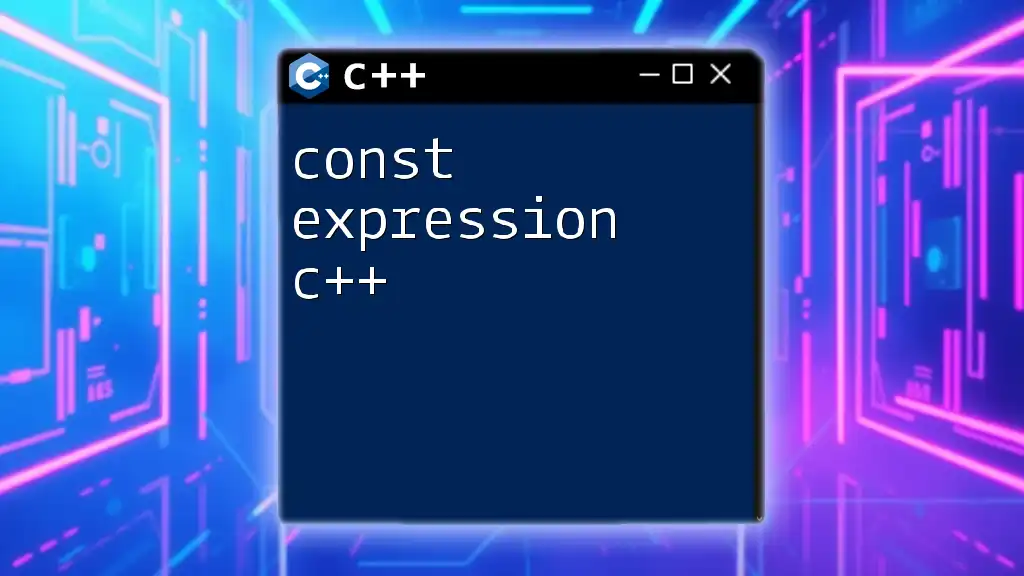
Conclusion
Recap of Key Points
In summary, the does not equal sign in C++ is a fundamental operator that plays a crucial role in comparison and decision-making within your code. Mastering its usage will undoubtedly enhance your programming skills and enable you to write more effective C++ applications.
Encouragement to Further Explore C++
I encourage you to delve deeper into C++ programming and explore other operators and concepts introduced in the language. The more you practice and familiarize yourself with different expressions, the more proficient you will become.
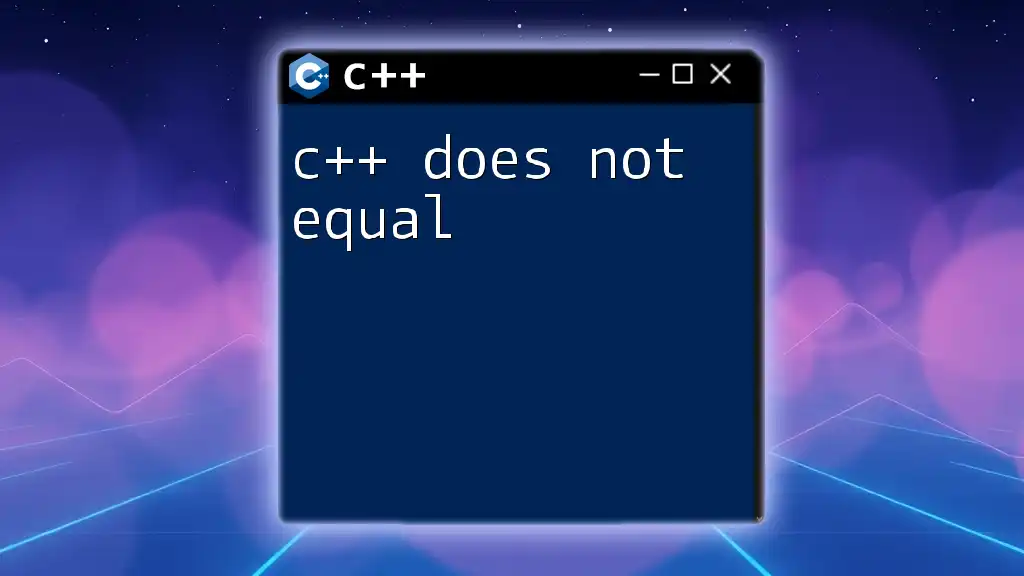
Additional Resources
Recommended Readings and Tools
To further expand your knowledge, I recommend exploring books on C++ programming, leveraging online courses, and utilizing coding platforms like repl.it or Codecademy for hands-on practice. These resources will complement your understanding and help solidify your grasp on how to effectively use the not equal operator and other C++ capabilities.