In C++, the "not equal" operator is represented by `!=`, which is used to compare two values for inequality.
if (a != b) {
// Code to execute if a is not equal to b
}
Understanding the Not Equal Operator in C++
What is the Not Equal Operator?
In C++, the not equal operator is represented by the symbol `!=`. This operator is used to compare two values and returns `true` if they are not equal and `false` if they are equal. Understanding how to use this operator is vital for executing conditional logic in your programs.
Syntax of the Not Equal Operator
The syntax for using the not equal operator is straightforward. For example:
if (a != b) {
// code to execute if a is not equal to b
}
You can compare various data types using this operator, including integers, characters, and strings, making it versatile and widely used in C++ programming.
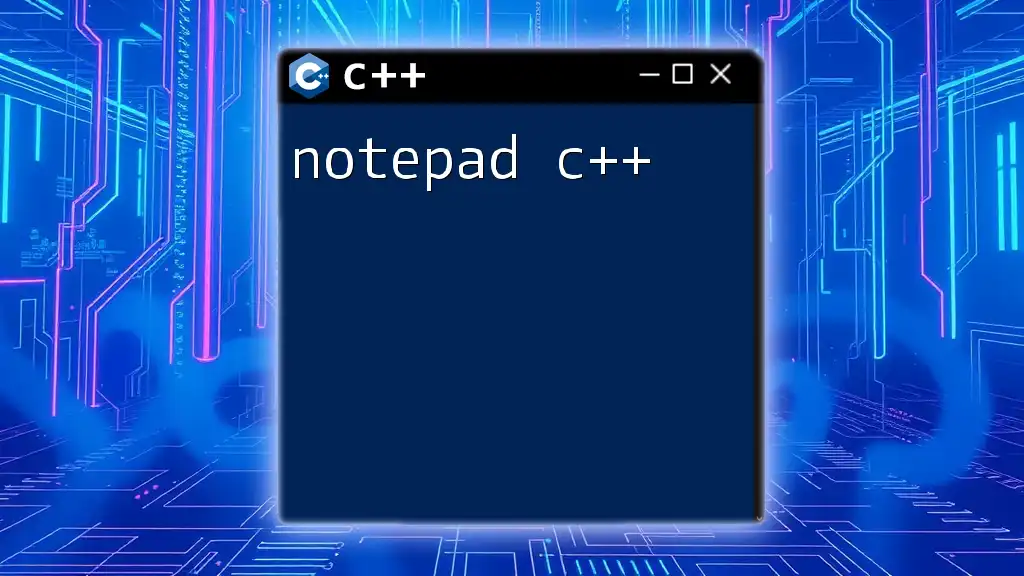
Practical Usage of Not Equal in C++
Basic Example: Integer Comparison
To illustrate how the not equal operator works, consider the following example comparing two integers.
int a = 5;
int b = 10;
if (a != b) {
std::cout << "a is not equal to b" << std::endl;
}
In this code, since `a` and `b` hold different values, the message "a is not equal to b" will be printed to the console. This demonstrates how simple it is to utilize the `!=` operator to perform comparisons.
Comparing Other Data Types
Character Comparison
The not equal operator can also be used to compare character variables. Here's an example:
char c1 = 'A';
char c2 = 'B';
if (c1 != c2) {
std::cout << "c1 is not equal to c2" << std::endl;
}
In this scenario, since characters `c1` and `c2` are different, it outputs "c1 is not equal to c2". This reinforces the idea that `!=` can be applied to various data types within C++.
String Comparison
One of the more complex types to compare is strings. Here's how you can use the not equal operator with string objects:
#include <string>
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 != str2) {
std::cout << "str1 is not equal to str2" << std::endl;
}
In the code above, the output will read as "str1 is not equal to str2." Do note that you must include the `<string>` library to work with C++ string objects effectively.
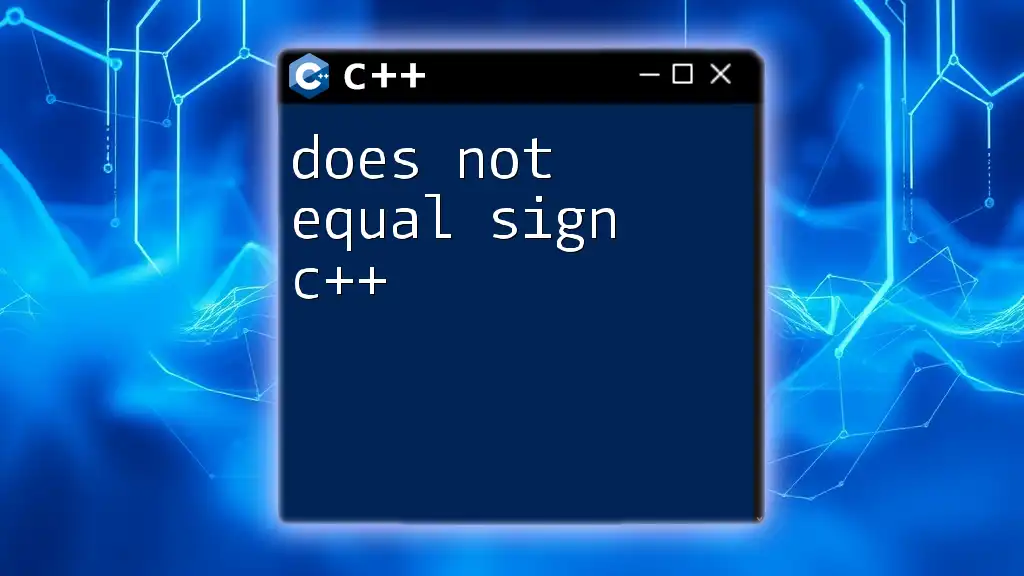
Common Use Cases for Not Equal in C++
Conditional Statements
The not equal operator is often used in conditional statements, particularly `if` statements, to dictate program flow. For example, consider a simple user input check:
std::string input;
std::cout << "Enter a name: ";
std::cin >> input;
if (input != "Admin") {
std::cout << "Welcome, " << input << std::endl;
}
In this case, the program greets users only if their name isn't "Admin". This showcases how `!=` allows you to control logic flow based on user input.
Loops and Other Constructs
The not equal operator is also useful in loops, like `while` loops, where you want to iterate until a certain condition is met:
int count = 0;
while (count != 5) {
std::cout << count << std::endl;
count++;
}
This loop prints the value of `count` each time, terminating only when `count` reaches 5. The clarity that the not equal operator provides in the loop condition is essential for effective iteration control.
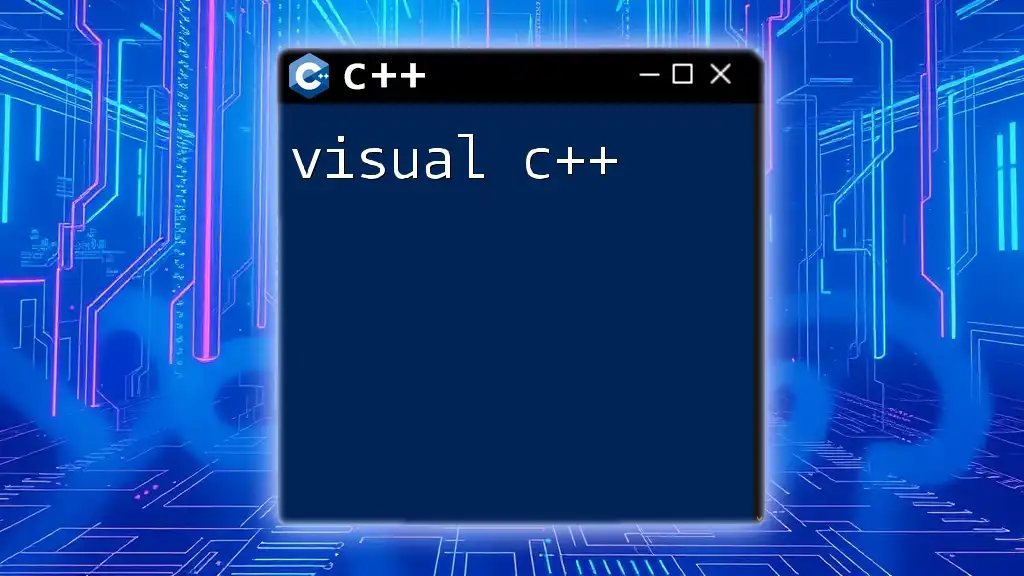
Handling Special Cases
Not Equal with Pointers
The not equal operator can be applied to pointers as well, offering a way to validate pointer states. Here's an example:
int x = 10;
int* ptr1 = &x;
int* ptr2 = nullptr;
if (ptr1 != ptr2) {
std::cout << "ptr1 is not equal to ptr2 (ptr is not null)" << std::endl;
}
This code checks if `ptr1` is not null and thus holds the address of `x` while `ptr2` is initialized to `nullptr`. This example illustrates a practical application of the not equal operator when dealing with pointers in C++.
Comparing Floating Point Numbers
When working with floating-point numbers, caution is necessary due to precision issues in arithmetic. Here's a demonstration:
double a = 0.1;
double b = 0.2;
if (a + a != b) {
std::cout << "a + a is not equal to b" << std::endl;
}
While it seems logical at first, floating-point arithmetic may not yield expected results due to inherent precision problems. It's essential to consider such factors when using the not equal operator for floating-point comparisons.
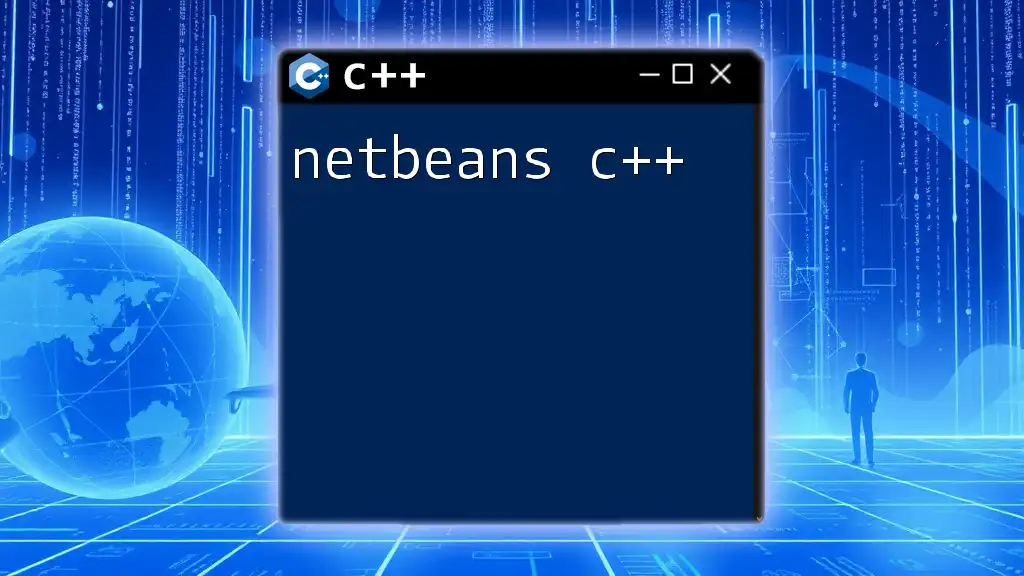
Common Errors and Debugging Tips
Errors When Using Not Equal
A common mistake programmers make is comparing incompatible types or failing to recognize scope and initialization issues. Always ensure that variables are initialized properly and of compatible types before using the not equal operator to avoid unexpected behavior.
Debugging Not Equal Comparisons
When a comparison fails to yield the expected results, you can adopt certain strategies to debug your code:
- Print out the values being compared just before the condition is executed to verify their state.
- Check variable initialization carefully; uninitialized variables can lead to erroneous outcomes.
- Consider edge cases, such as the impact of precision on floating-point comparisons.
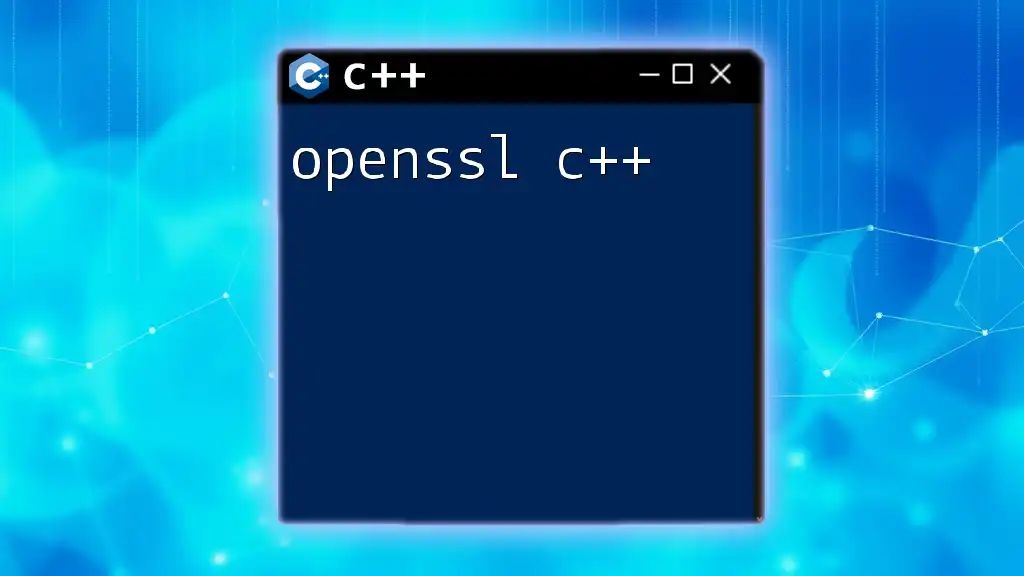
Conclusion
In summary, the not equal operator in C++ is a powerful tool for comparison that is essential for controlling program flow. By mastering the usage of `!=`, you can enhance your ability to write clear, logical, and effective C++ code. Practice using the not equal operator in various scenarios to build your proficiency and confidence in C++.