In this Unreal C++ tutorial, you'll learn how to create a simple actor that rotates continuously in the game world.
Here's a basic example of implementing continuous rotation in an Unreal Engine C++ actor:
#include "YourActor.h"
#include "GameFramework/Actor.h"
// Sets default values
AYourActor::AYourActor()
{
PrimaryActorTick.bCanEverTick = true; // Enable ticking
}
// Called every frame
void AYourActor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// Rotate the actor continuously
FRotator NewRotation = GetActorRotation() + FRotator(0, 1, 0); // Rotate around Y-axis
SetActorRotation(NewRotation);
}
Setting Up Your Environment
Before diving into game development with Unreal Engine, it is crucial to set up your environment correctly.
Installing Unreal Engine
To get started, download the Epic Games Launcher. After installation, navigate to the Unreal Engine tab, where you can easily download the latest version of Unreal Engine. Ensure your system meets the installation requirements, which typically include:
- A compatible Windows or Mac operating system
- At least 8 GB of RAM
- An up-to-date graphics card
Setting Up Visual Studio
Unreal Engine integrates deeply with Visual Studio, making it a vital component for C++ development.
To set up Visual Studio, ensure you install a compatible version, preferably Visual Studio 2019 or later. During installation, select the Game Development with C++ workload, which includes necessary components such as the Unreal Engine Extension.
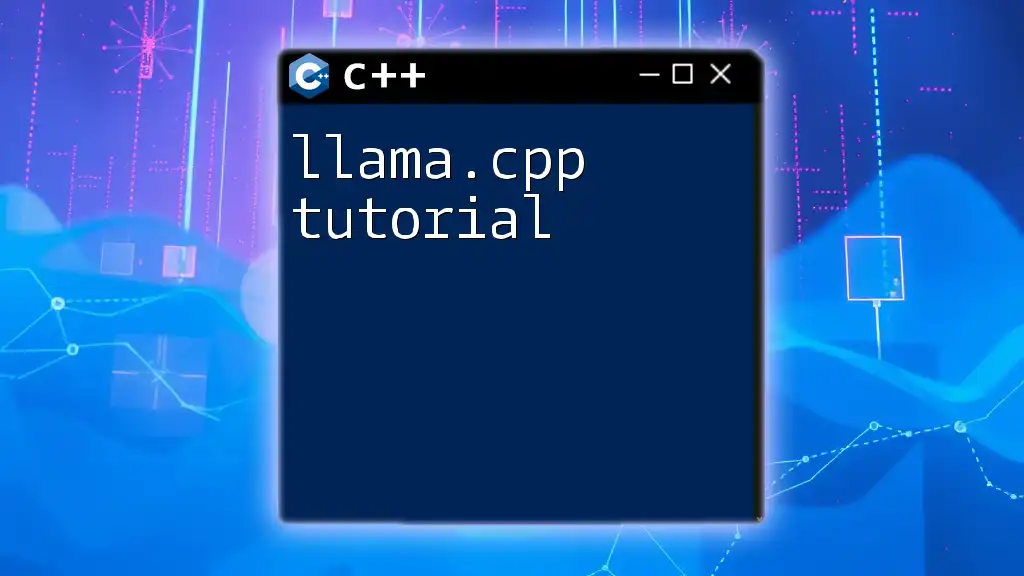
Getting Started with Your First Project
Creating a New Unreal C++ Project
Once your environment is set up, create a new C++ project from the Unreal Engine Launcher.
Choose a project template based on what you intend to build, such as First Person, Third Person, or Top Down. This will provide you with some default functionality and assets, significantly easing your learning curve.
Exploring the Unreal Editor
After your project loads, familiarize yourself with the Unreal Editor, consisting of various panels such as the Viewport, World Outliner, and Content Browser. Understanding these components will accelerate your C++ development process within Unreal Engine.
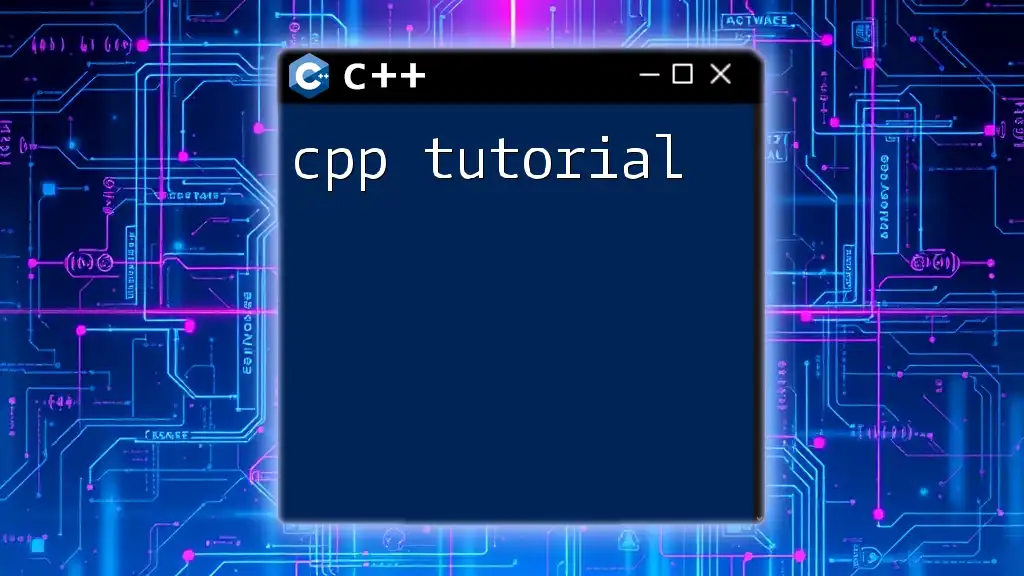
Understanding the Basics of C++ in Unreal Engine
Key Programming Concepts
While Unreal Engine abstracts many programming details through Blueprints, understanding C++ fundamentals is essential.
-
Classes, Objects, and Inheritance: C++ is object-oriented, meaning you define classes and create objects based on these classes. Understanding inheritance allows you to build on existing functionality.
-
C++ Syntax Basics: Familiarize yourself with C++ syntax, including variables, data types, and control structures. For example:
int Score = 0; // Variable declaration
if (Score > 10) {
// some code
}
C++ in Unreal: A Unique Paradigm
Unreal Engine introduces unique programming paradigms that differ from standard C++ development.
The Actor class is a foundation for C++ development in Unreal. Creating game objects typically involves subclassing AActor, allowing you to manipulate their properties and behaviors within the game world.
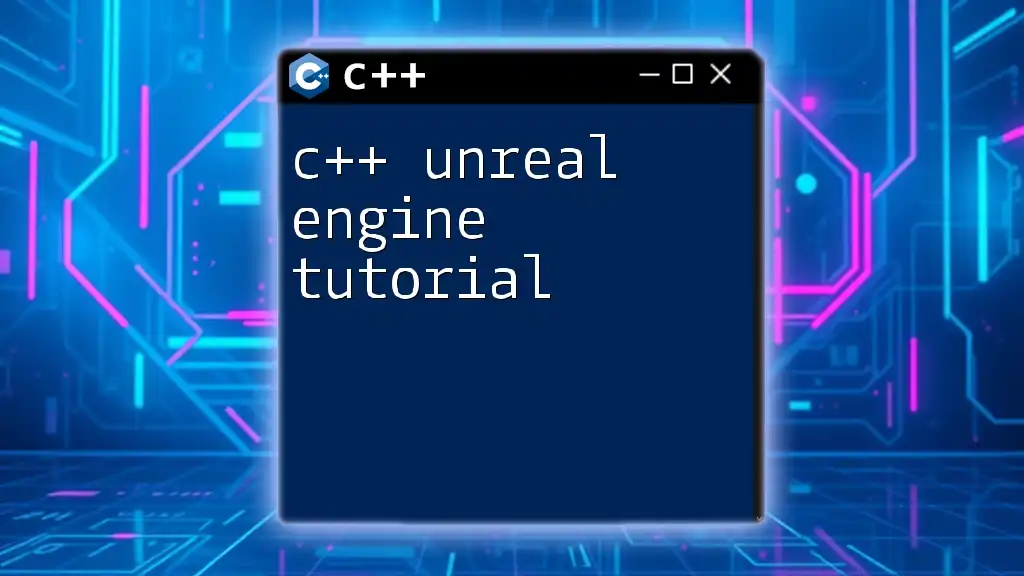
Creating Your First Game Actor
Defining a New C++ Class
Start creating a new C++ class by navigating to the File menu and selecting New C++ Class. Choose Actor as the parent class to create a simple game object.
As a practical demonstration, here’s a basic Actor class definition:
#include "GameFramework/Actor.h"
class AMyActor : public AActor {
public:
AMyActor();
protected:
virtual void BeginPlay() override;
virtual void Tick(float DeltaTime) override;
};
This code snippet defines a new Actor called AMyActor, setting up essential lifecycle methods.
Implementing Basic Functionality
Within the constructor of your class, you can add components. For instance, to add a static mesh component, include this line in your constructor:
MyStaticMeshComponent = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("MyStaticMesh"));
RootComponent = MyStaticMeshComponent;
This line sets up a static mesh component for your Actor, anchoring it to the root component.
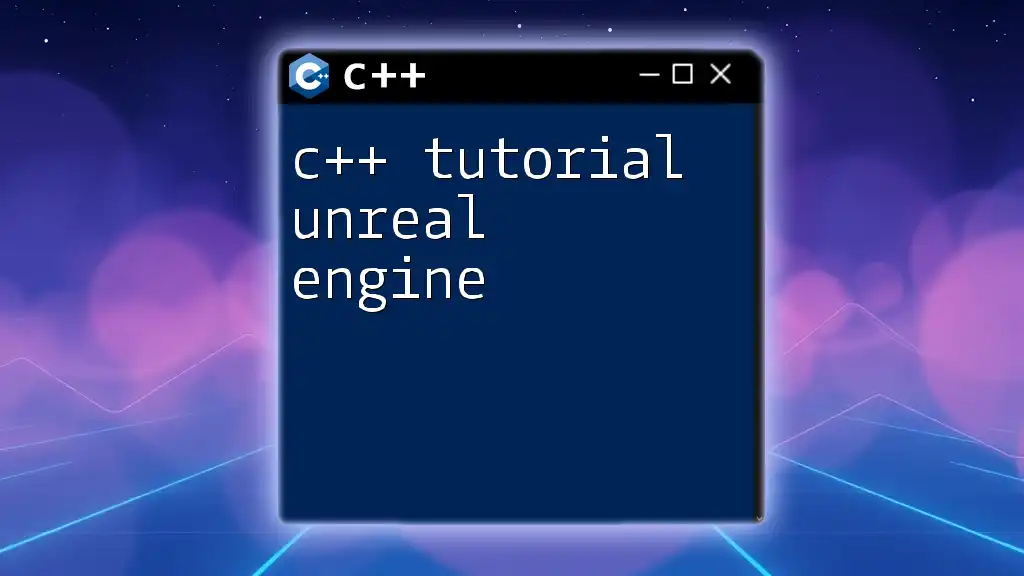
Interacting with C++ and Blueprints
Integrating C++ with Blueprints
One of Unreal Engine’s strengths is its ability to combine C++ with Blueprints. Exposing your C++ classes and variables to Blueprints enhances flexibility and usability.
To achieve this, use the UPROPERTY and UFUNCTION macros. For example, the following exposes a variable and a function to Blueprints:
UPROPERTY(BlueprintReadWrite, Category="Properties")
float MyValue;
UFUNCTION(BlueprintCallable, Category="Actions")
void MyFunction();
Blueprints users can now modify MyValue directly from the editor and call MyFunction when needed.
Creating Blueprintable Classes
To make your C++ class blueprintable, simply ensure you use the UCLASS macro above your class definition. This allows designers to create instances of your C++ classes within the Blueprint Editor, combining the strengths of both programming worlds.
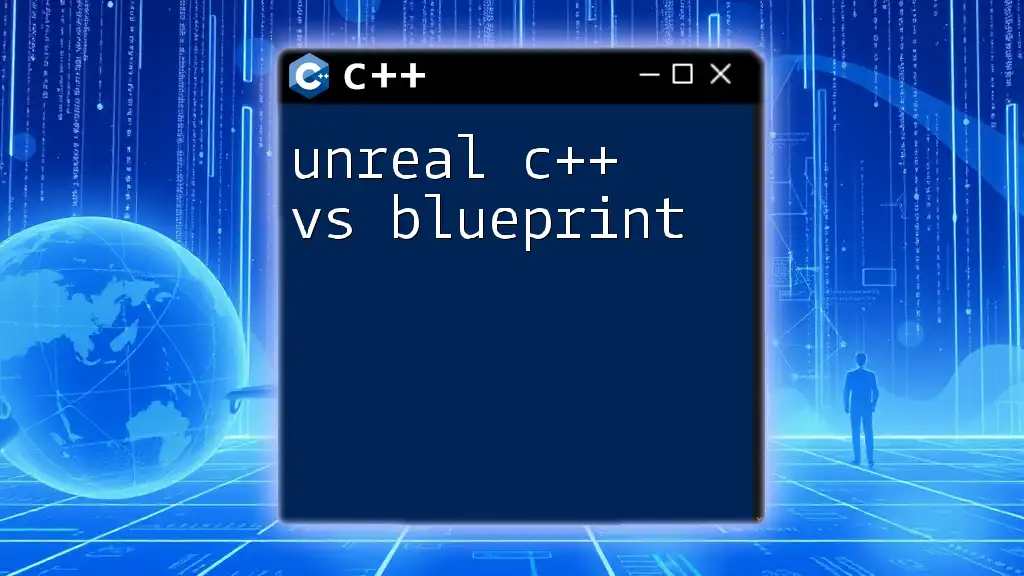
Building Game Logic
Handling Input in C++
In your game, you'll likely want to respond to player input. To do this, first set up input mapping in the Project Settings under Input. This will help you define key bindings to actions or events.
Then, in your character class, implement the following to manage key presses:
void SetupPlayerInputComponent(UInputComponent* PlayerInputComponent) override {
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAction("Jump", IE_Pressed, this, &AMyCharacter::Jump);
}
This snippet binds the jump action to the jump method in your character class.
Event Handling and Delegates
Understanding Unreal Engine's delegates is crucial for making your game interactive. Delegates are a way to define callback methods that can be called in response to events.
Here's how you can implement and bind events:
MyButton->OnClicked.AddDynamic(this, &MyClass::MyFunction);
This code binds an event triggered by a UI button click to a method in your class, enabling dynamic interaction between your game’s components.
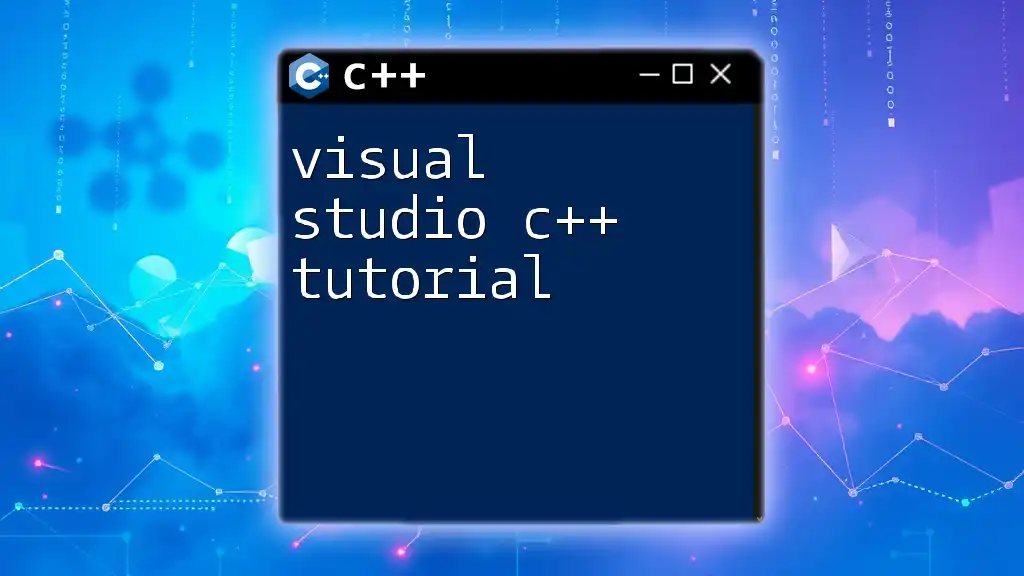
Debugging and Testing Your Game
Using Unreal Engine Debugging Tools
Effective debugging is a vital skill in game development. Unreal Engine offers robust debugging tools, allowing you to set breakpoints, inspect variables, and trace execution flow.
Use logging through the UE_LOG macro to output messages during gameplay. For example:
UE_LOG(LogTemp, Warning, TEXT("MyActor has been spawned!"));
Testing Your Game in the Editor
Running your game within the editor is straightforward. Click the Play button to start testing various features and interactions within your game world. This immediate feedback loop enables rapid iteration and refinement of gameplay mechanics.
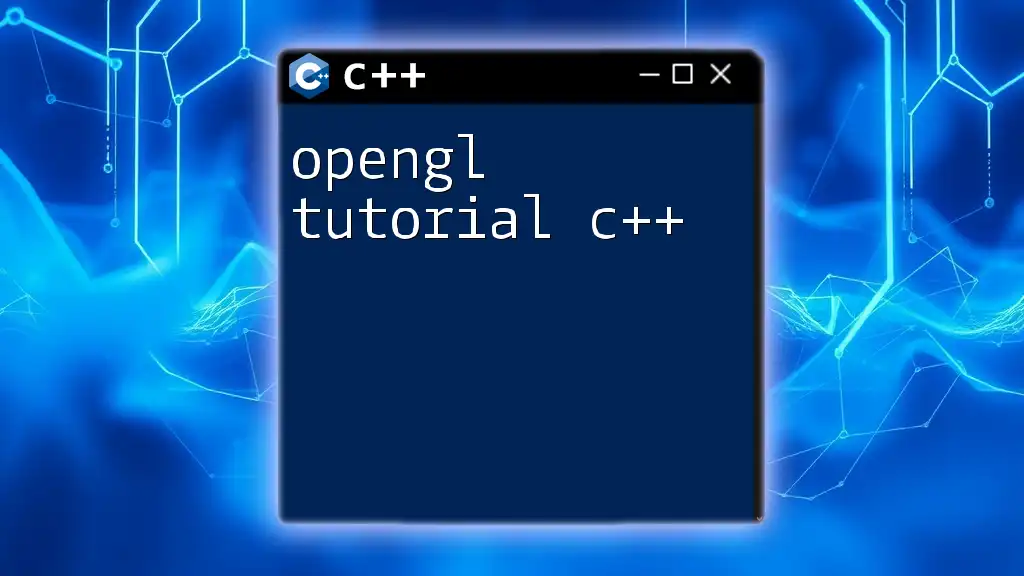
Extending Your Knowledge
Learning Resources
Expanding your knowledge of Unreal Engine C++ development is essential for long-term success. Consider the following resources:
- Official Unreal Engine Documentation: Comprehensive and continually updated.
- Online Courses: Websites like Udemy and Coursera offer structured learning paths.
- Community Forums: Engage with the community on platforms like the Unreal Engine forums or Stack Overflow.
Continuing Your Development Journey
Keep yourself updated with the latest Unreal Engine news, updates, and innovations. Engaging in community projects, participating in game jams, or contributing to open-source projects can dramatically improve your skills and broaden your understanding of the game development landscape.
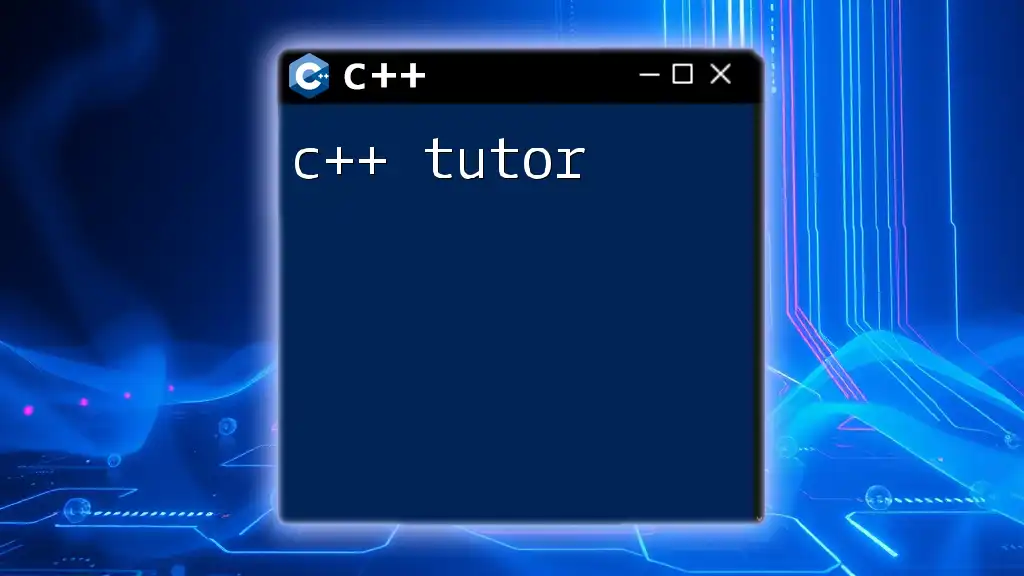
Conclusion
In wrapping up this Unreal C++ tutorial, remember that practice and active engagement with the community play critical roles in your learning journey. Don’t hesitate to experiment, make mistakes, and ask questions. The world of game development is an exciting and continually evolving field, and your journey is just beginning!