The Visual C++ Build Toolset is a collection of tools and libraries within the Visual Studio environment that enable developers to compile and build C++ applications efficiently.
// Example of a simple C++ program using Visual C++ build toolset
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Visual C++ Build Toolset
What is a Build Toolset?
A build toolset serves as a collection of tools that automate the process of converting source code into executable programs. This includes various functions such as compiling, linking, and managing dependencies. In the context of C++, a build toolset simplifies the workflow of developers, allowing them to focus on writing code rather than managing the build process.
Key Components of Visual C++ Build Toolset
Compiler
The Microsoft C++ Compiler is the heart of the Visual C++ build toolset. It translates your C++ code into machine code, which the computer can execute. Compiling a simple C++ program can be done via command line with the following command:
cl /EHsc hello.cpp
In this command, `cl` invokes the compiler, and `/EHsc` specifies exception handling model settings. The result is an object file that forms the building block for executable code.
Linker
Once your C++ code is compiled, the next step is linking. The linker consolidates multiple object files into a single executable. This process is essential when your program consists of several modules, or when you're using external libraries. For instance, to link against the standard C++ library, the following command can be issued:
link hello.obj
This command combines all necessary object files into an executable named `hello.exe`.
Build Configuration Tools
Build configuration tools allow developers to set specific settings for various build types, such as Debug or Release. These configurations control optimizations, logging, and error messages, impacting runtime behavior and performance. Understanding how to configure these options is vital for maximizing the efficiency and effectiveness of your builds.
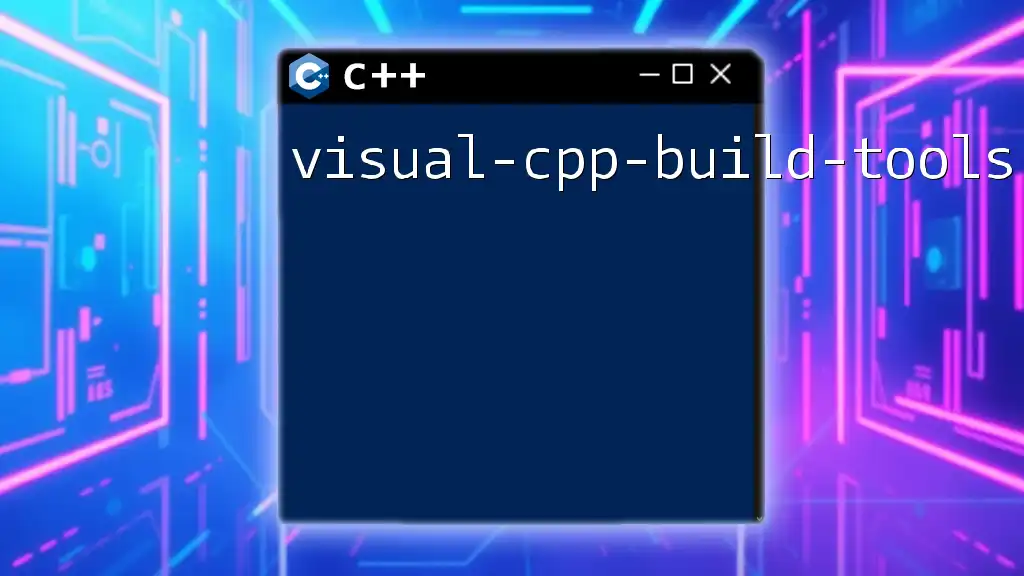
Setting Up Visual C++ Build Tools
Installation of Visual C++ Build Tools
To start using the Visual C++ build toolset, installation is the first step. The Visual Studio installer offers a user-friendly interface. During installation, you can choose from various workloads, with the Desktop development with C++ workload being the most relevant for building C++ applications.
Simply start the installer, select the C++ workload, and proceed with the installation. By choosing the right components, you can optimize your setup for better performance and usability.
Environment Setup
Once installed, setting up your environment is crucial. You need to ensure that the necessary tools are accessible from the command line. Setting the PATH environment variable allows you to use commands such as `cl` and `link` globally.
To check if your setup is correct, navigate to the command prompt and execute:
cl
If properly set up, this command should return version information about the Microsoft C++ Compiler.
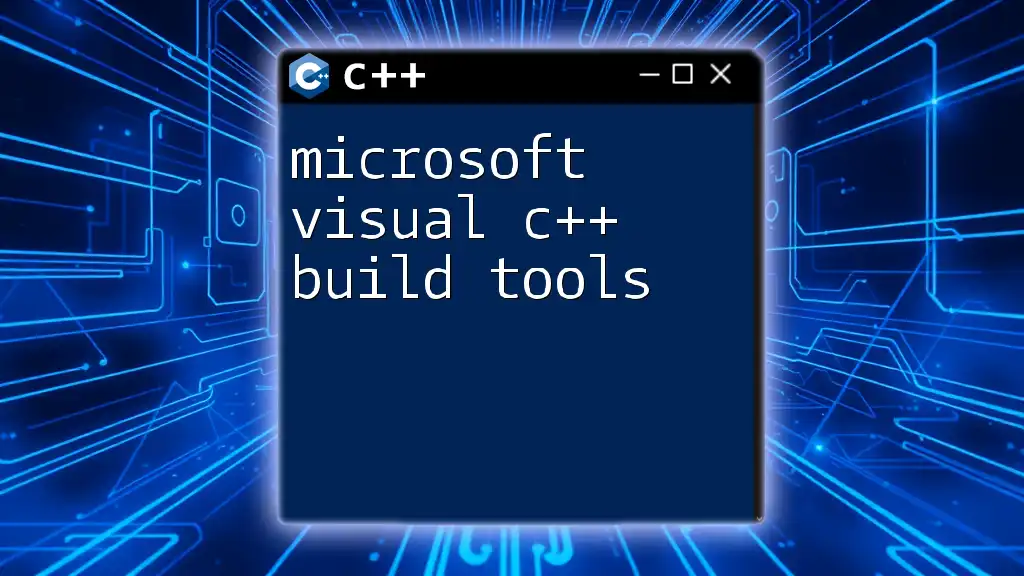
Using Visual C++ Build Tools
Building Projects with Visual Studio
The Visual Studio IDE is a comprehensive environment that facilitates C++ development and the build process. After opening Visual Studio, you can create a new project by selecting File > New > Project and choosing the appropriate C++ template.
Once the project is set up, simply click on the Build menu and select Build Solution. This command compiles your code and links the necessary files, producing an executable.
Command Line Build with MSBuild
For those who prefer the command line, MSBuild is a powerful tool that enables building C++ projects directly from the terminal. MSBuild offers numerous advantages, including automated builds in scripts and better integration with CI/CD pipelines.
To build a C++ project, navigate to the project directory and run:
msbuild MyProject.vcxproj /p:Configuration=Release
This command instructs MSBuild to compile the configuration specified (in this case, Release).
Integration with CMake
CMake has become a standard tool for managing the build process in C++ projects, facilitating cross-platform builds. It generates native build scripts based on a configuration file named `CMakeLists.txt`.
To configure a project with Visual C++ build tools using CMake, create a `CMakeLists.txt` file. Here’s a simple example:
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(HelloWorld main.cpp)
To generate the necessary files using Visual C++, navigate to the root of your CMake project in the command prompt and run:
cmake -G "Visual Studio 16 2019" .
This command signifies the use of Visual Studio 2019 as the generator, preparing the project for build.
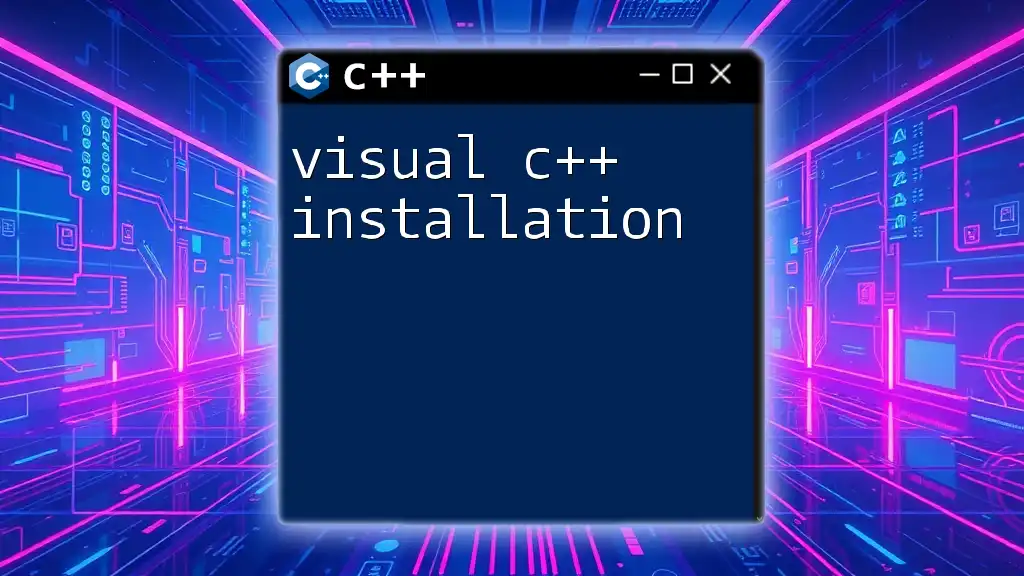
Best Practices for C++ Build Systems
Efficient Build Management
Managing builds efficiently can significantly improve development speed. Organizing your codebase logically and modularly ensures that changes in one part of the project require less recompilation. Using precompiled headers can further speed up the build process. By including commonly used headers in a precompiled header file, you save time during subsequent compilations.
Debugging Build Issues
Build issues are common, especially as projects grow in size. Familiarize yourself with common compiler and linker error messages. Effective troubleshooting often involves examining the output logs. Utilize the /showIncludes compiler flag to understand which header files are included during compilation, aiding in tracking down problems.
Continuous Integration (CI)
Integrating Visual C++ builds into Continuous Integration systems like Jenkins or Azure DevOps enhances collaboration and ensures code quality. You can automate the build process and run tests every time changes are made to the code, significantly reducing the chances of bugs slipping through.
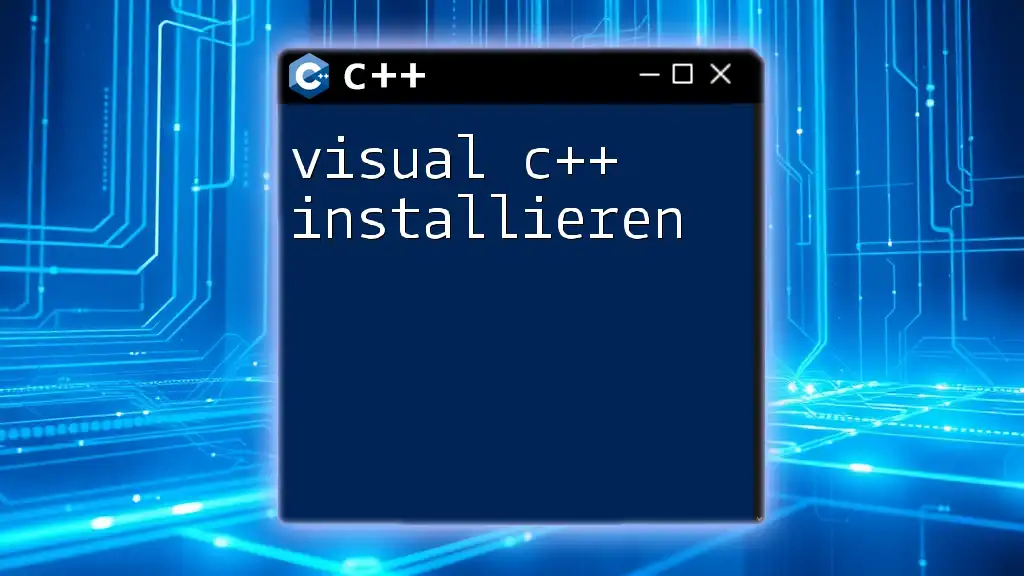
Conclusion
Understanding the Visual C++ build toolset is essential for any C++ developer seeking to improve their workflow and efficiency. Mastering the components, setup, and best practices unlocks a more productive development process. As you explore Visual C++ further, remember that consistent experimentation and learning can significantly heighten your proficiency. The C++ community offers rich resources, so take advantage of them to grow your skills.