In this C++ tutorial for Unreal Engine, you'll learn how to create a simple Actor that spins continuously in the game world using the `Tick` function.
#include "YourActor.h"
void AYourActor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
FRotator NewRotation = GetActorRotation();
NewRotation.Yaw += 1.0f; // Adjust rotation speed here
SetActorRotation(NewRotation);
}
Setting Up Your Development Environment
Installing Unreal Engine
To get started with your C++ journey in Unreal Engine, the first step is to download and install Unreal Engine. You can do this by visiting the [official Unreal Engine website](https://www.unrealengine.com/) and navigating to the "Get Started" section. Here, you will find options for downloading the Epic Games Launcher, which is required for installing Unreal Engine.
Once you have the launcher installed, follow these simple steps:
- Launch the Epic Games Launcher and log in or create an account.
- Navigate to the Library tab where you can find the "Install Engine" section.
- Choose the latest stable version of Unreal Engine and click Install.
Selecting the right version is important; make sure you choose one that supports C++ development, typically the latest version unless specified otherwise.
Installing Visual Studio
Unreal Engine relies on Visual Studio for C++ development, so you will need to install it. It's recommended to use the Community Edition of Visual Studio as it is free and fully equipped for development.
To install Visual Studio, follow these steps:
- Download the [Visual Studio Community Edition](https://visualstudio.microsoft.com/).
- During installation, ensure that you select the "Desktop development with C++" workload. This option installs all necessary components for Unreal Engine development, including C++ compilers and libraries.
- After installation, verify that the Unreal Engine integration is set up correctly within Visual Studio, enabling seamless project building.
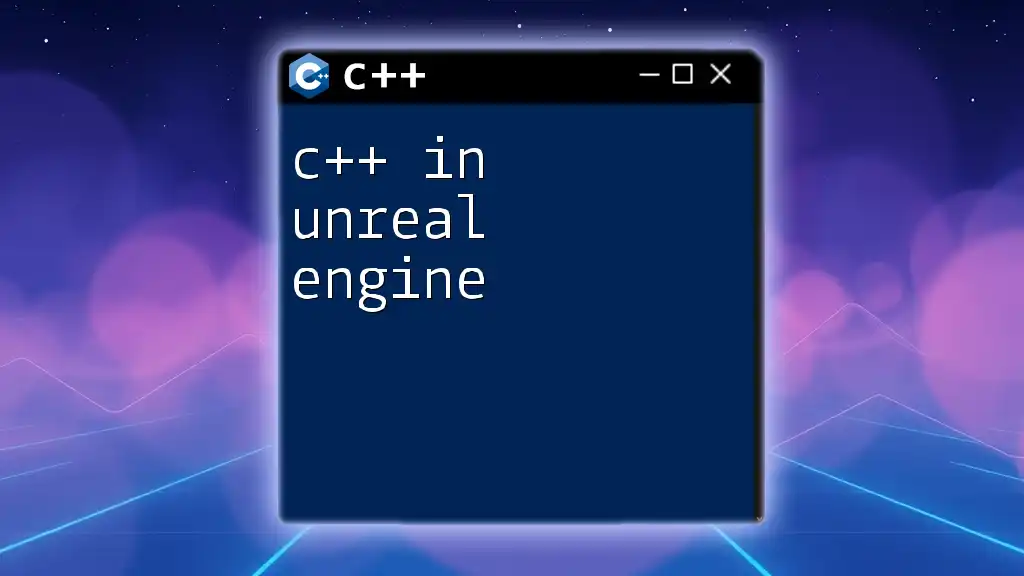
Creating Your First Unreal Engine Project
Starting a New Project
Now that your development environment is set up, it's time to create a new C++ project in Unreal Engine. When you launch Unreal Engine, you will be presented with several project templates.
- Choose a template that aligns with your goals. For beginners, the "First Person" template may offer a straightforward introduction.
- When prompted, select C++ as the project type and configure project settings such as project name and location.
- Click Create Project to initiate construction.
Understanding the Project Structure
As your project loads, familiarizing yourself with its structure is crucial.
- Source Folder: Contains all of your C++ code. It's where you will write the classes that make up your game.
- Content Folder: This directory holds assets like textures, sounds, and models essential for your project.
- Config Folder: Files here configure various settings important for game functionality, performance, and gameplay mechanics.
Additionally, understanding the build system is important. In Unreal Engine, the `.uproject` file defines your project settings, while `.uplugin` files are used for plugins if you decide to expand your project’s capabilities.
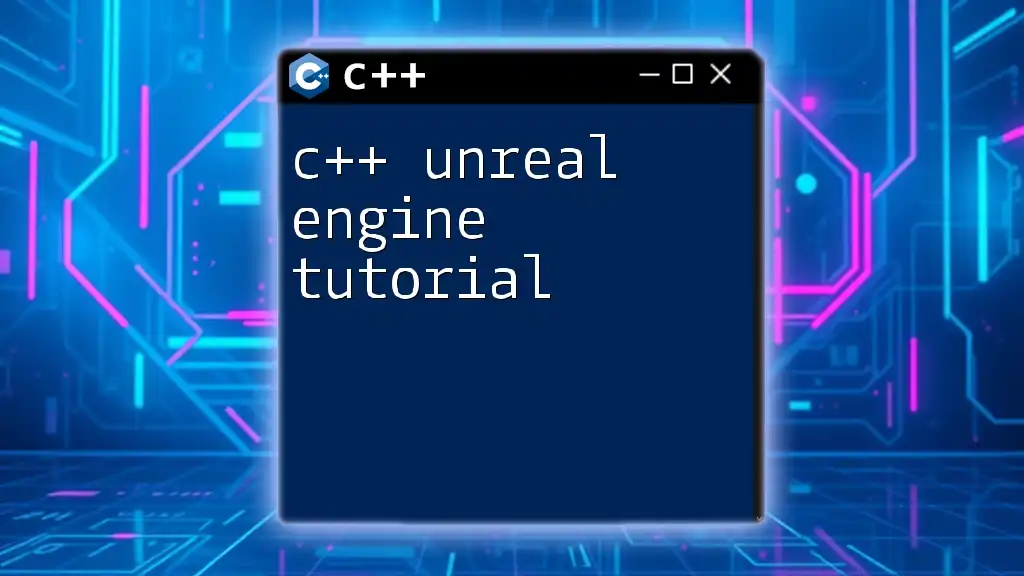
The Basics of C++ in Unreal Engine
Introduction to C++ Syntax
Before diving into Unreal Engine’s C++ functionalities, a quick recap of basic C++ syntax is beneficial. Familiarizing yourself with variables, data types, and functions is key to effective programming.
For instance, creating a simple function could look like this:
void PrintHello() {
std::cout << "Hello, Unreal Engine World!" << std::endl;
}
This example demonstrates a basic function that outputs a phrase to the console.
Creating Your First C++ Class
Creating a C++ class in Unreal Engine is straightforward. Here are the steps:
- In the Source folder, right-click and select New C++ Class.
- Choose the Actor class since it serves as a base class for most objects in Unreal.
- Name your class, e.g., `MyFirstActor`, and create it.
Your `MyFirstActor.h` header file might look like this:
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "MyFirstActor.generated.h"
UCLASS()
class MYPROJECT_API AMyFirstActor : public AActor {
GENERATED_BODY()
public:
AMyFirstActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Within this framework, the constructor, `BeginPlay`, and `Tick` functions are defined, providing a skeletal structure for your game logic.
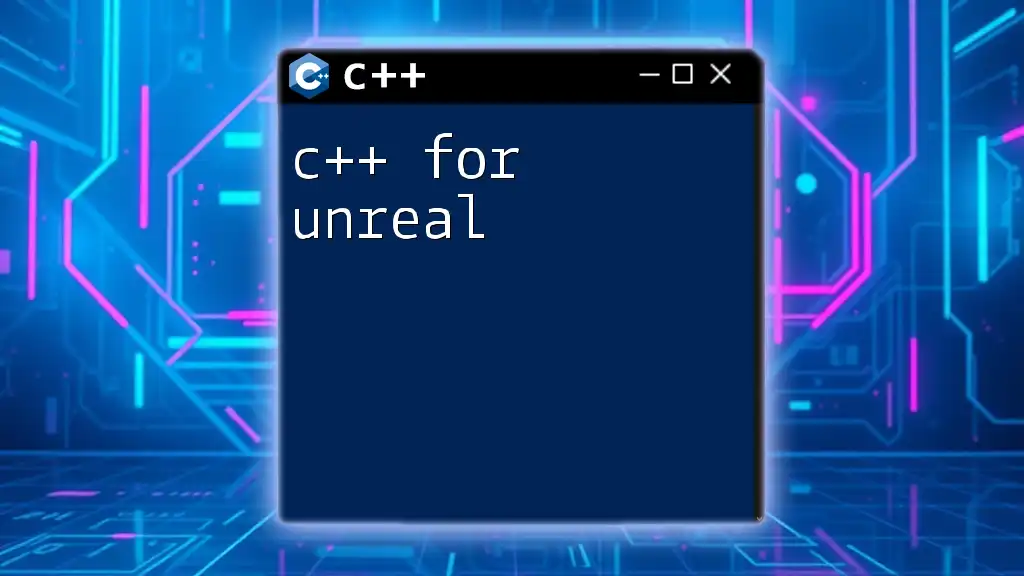
Unreal Engine C++ Gameplay Framework
Understanding Actors and Components
In Unreal Engine, Actors are the building blocks of your game world; everything you see in the game is an Actor or a component of an Actor. Components allow you to add functionality and behaviors to Actors without needing a whole new class for each variation.
When creating a custom Actor, like a `LightSourceActor`, you can utilize components such as `UStaticMeshComponent` to visually represent the Actor:
#include "LightSourceActor.h"
#include "Components/StaticMeshComponent.h"
ALightSourceActor::ALightSourceActor() {
PrimaryActorTick.bCanEverTick = true;
UStaticMeshComponent* MeshComponent = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("BaseMesh"));
RootComponent = MeshComponent;
}
Introduction to Blueprints and C++
One of the most potent features of Unreal Engine is how it integrates Blueprints, a visual scripting system, with C++. It enables designers to make rapid iterations without needing to write code.
You can expose C++ functions to Blueprints, allowing designers to interact with your C++ logic. For instance, suppose you have a function that increases the player’s score; by marking it with the `UFUNCTION` macro, it becomes usable in Blueprints:
UFUNCTION(BlueprintCallable, Category="Score")
void IncreaseScore(int Amount);
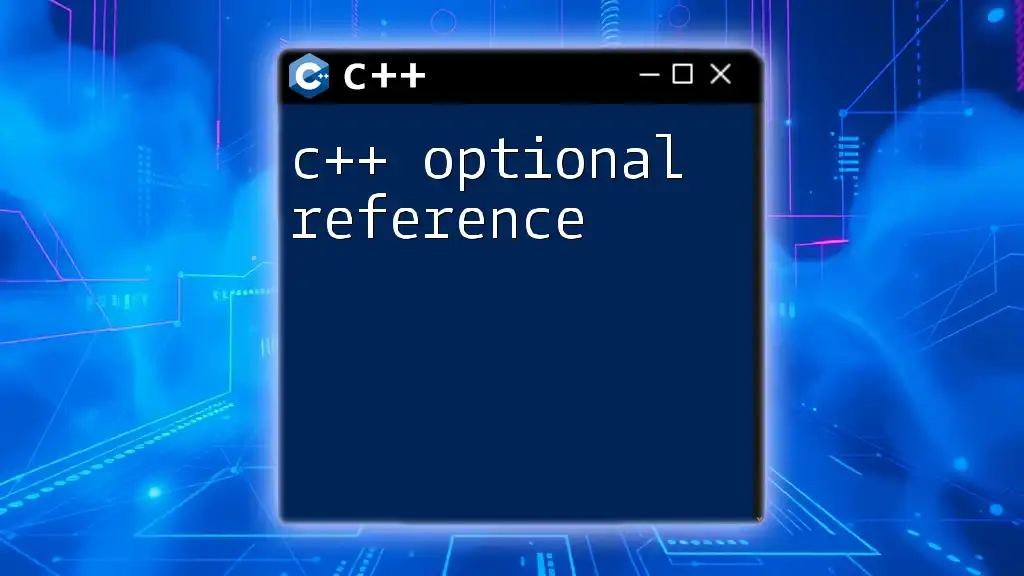
Game Mechanics with C++
Implementing Player Input
Unreal Engine has a sophisticated Input System that allows developers to handle player actions seamlessly. To get started, you will first define input actions and axis mappings in the project settings.
For example, after setting up a binding for "Jump" using the Editor, you can capture the input within your C++ class:
void AMyCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent) {
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAction("Jump", IE_Pressed, this, &AMyCharacter::StartJump);
}
void AMyCharacter::StartJump() {
Jump();
}
Creating Game Objects
Creating interactive game objects is pivotal in gameplay design. To create an object like a collectible item, you would define a new Actor class, adding necessary functionality for when a player interacts with it.
For instance:
void AMyCollectibleItem::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent,
AActor* OtherActor,
UPrimitiveComponent* OtherComp,
FVector NormalImpulse,
const FHitResult& Hit) {
if (OtherActor) {
// Logic for when the player collects the item
Destroy();
}
}
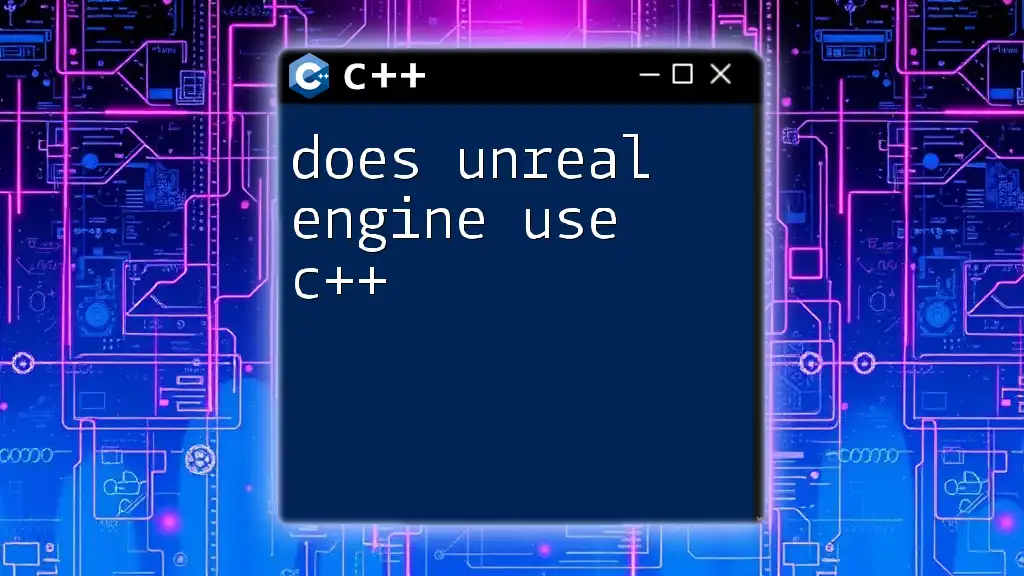
Advanced C++ Concepts in Unreal Engine
Inheritance in Unreal Engine
Understanding inheritance in C++ is crucial for scalable game development. By extending existing classes, you can create a hierarchy of behavior without rewriting code. For example, if you have a `Character` class, you can create a `PlayerCharacter` class that inherits from it:
class APlayerCharacter : public ACharacter {
// Additional functionalities for player character
};
Using Unreal Engine Macros
Unreal Engine utilizes a unique system of macros that simplifies integrating your C++ code with Unreal's property system. Important macros include:
- UCLASS: Marks a class as a UObject class.
- UPROPERTY: Exposes class variables to Unreal's editor and garbage collector, enabling easy access and editing.
- UFUNCTION: Used to expose functions to Blueprints.
Example of using macros effectively:
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category="Gameplay")
int Health;
UFUNCTION(BlueprintCallable, Category="Gameplay")
void TakeDamage(int DamageAmount);
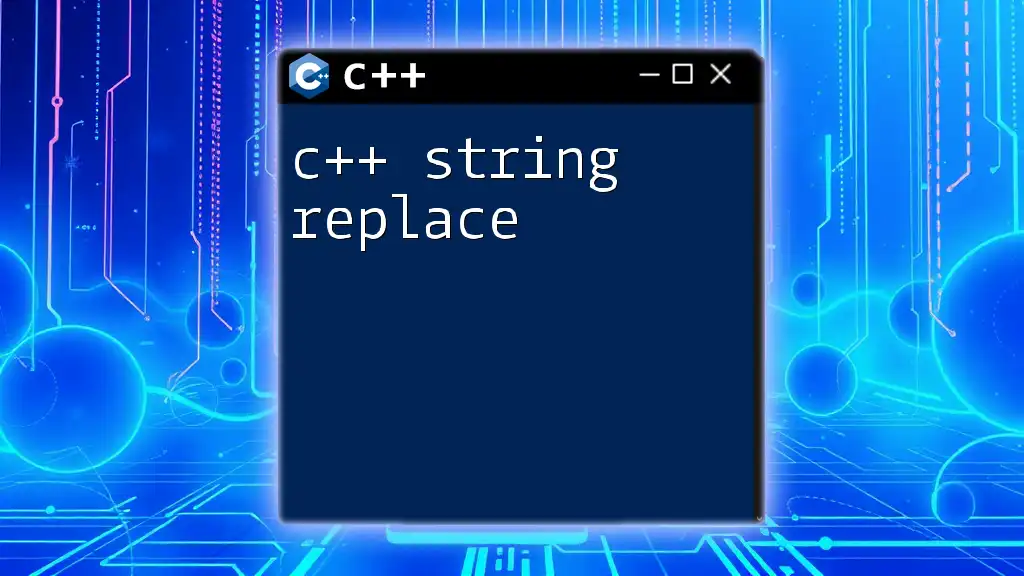
Debugging and Testing Your C++ Code
Setting Breakpoints in Visual Studio
Debugging is an integral aspect of developing games. By setting breakpoints in Visual Studio, you can pause your game's execution and inspect variables, allowing you to analyze states and behaviors dynamically. This technique is invaluable for identifying errors and improving game logic.
Writing Unit Tests for C++
Testing your code through unit tests is an excellent practice in game development. Unreal Engine supports unit tests, enabling you to automate checks on critical code functionalities. By implementing simple tests, you can confirm that changes do not introduce new errors.
Example of a simple unit test:
// TestExample.h
#include "Misc/AutomationTest.h"
IMPLEMENT_SIMPLE_AUTOMATION_TEST(FMyTest, "GameTests.MyTest", EAutomationTestFlags::ApplicationContextMask | EAutomationTestFlags::SmokeFilter)
bool FMyTest::RunTest(const FString& Parameters) {
// Set up test conditions
// Perform assertions
TestEqual(TEXT("Expected value to be true"), true, true);
return true;
}
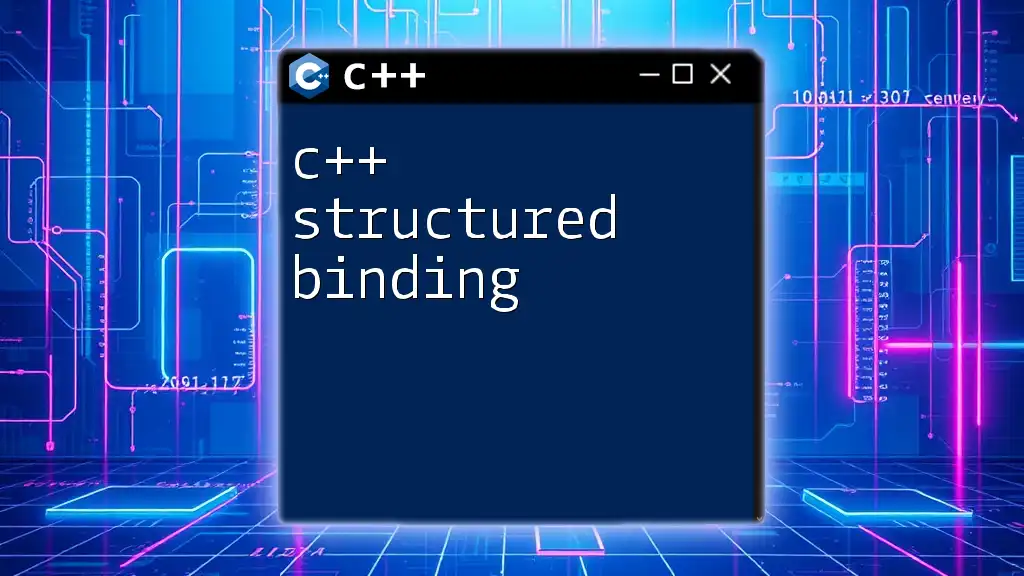
Building and Packaging Your Game
Compiling Your Code
Once you've written your C++ code, it must be compiled to integrate it with Unreal Engine. Within the Unreal Engine Editor, you can simply click on the Compile button at the top to build your project. Pay attention to the Output Log for any error messages that may arise, as they will guide you in troubleshooting issues.
Packaging Your Game
After completing your game, you may want to share it with others. Packaging your game prepares it for distribution.
To package your project, follow these steps:
- Navigate to `File > Package Project`.
- Choose your desired platform, such as Windows, iOS, or Android.
- Follow the prompts to configure settings like package location and build configurations.
Always optimize your game before packaging to ensure high performance and minimize file sizes, enhancing the user experience.
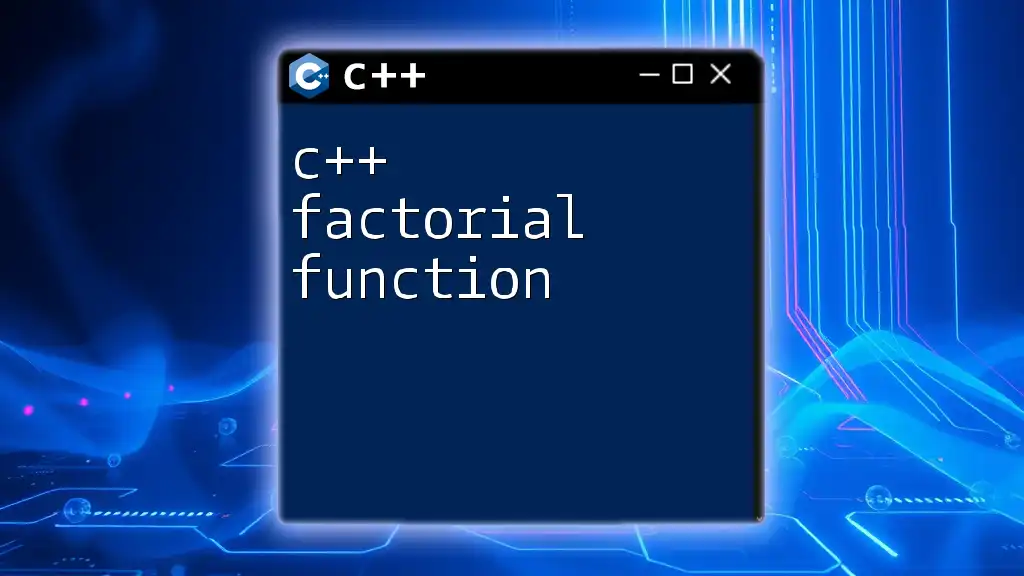
Conclusion
As you've learned throughout this tutorial, C++ in Unreal Engine opens a world of possibilities for game development. From setting up your environment to diving into advanced concepts like inheritance and macros, you now have a solid foundation to expand your skills further. Remember that practice is key in mastering C++, so take the knowledge you've gained and experiment with your own projects.
Feel free to join our community for additional resources, support, and networking with fellow developers as you pursue your journey in game development with C++.