In C++, you can use the `std::cout` object along with the newline character `\n` or the `std::endl` manipulator to output text followed by a newline in a simple and effective manner.
Here’s a code snippet demonstrating both methods:
#include <iostream>
int main() {
std::cout << "Hello, World!\n"; // Using newline character
std::cout << "Hello, Universe!" << std::endl; // Using std::endl
return 0;
}
Understanding Output in C++ with cout
What is cout?
The `cout` object, part of the C++ Standard Library, is used to output data to the console. It forms a critical component of the C++ input/output (I/O) system. Unlike some other programming languages that require specific functions for printing output, C++ uses `cout` in combination with the stream insertion operator (`<<`) to produce console output. This concise syntax makes it easy to display multiple items in a single statement, enhancing the programming experience.
The Need for New Lines
Using new lines in console output is essential for improving readability. When outputting multiple pieces of information, especially in complex applications or reports, a new line provides clear separation between different outputs. This organization allows users to easily read and comprehend displayed information, which is particularly important in user interfaces and debugging processes.
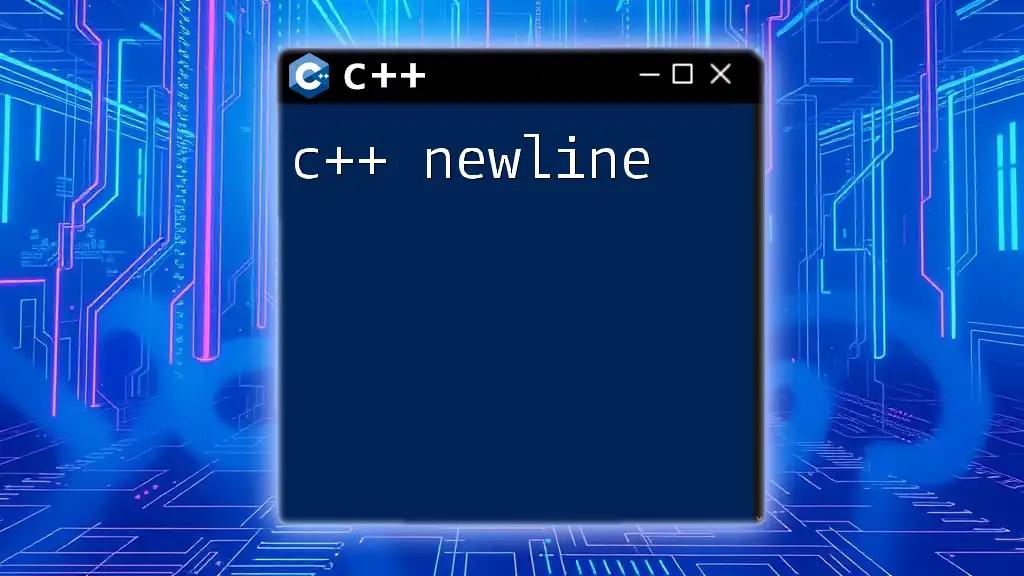
Creating New Lines with cout
Using the newline escape sequence (`\n`)
The simplest way to introduce a new line in your output is by using the newline escape sequence, denoted as `\n`. This character instructs the output stream to move to the next line.
Code Example:
#include <iostream>
int main() {
std::cout << "Hello, World!\n";
std::cout << "Welcome to C++ Programming!\n";
return 0;
}
This example shows how two separate output statements are separated by `\n`. When executed, the console will display each string on its own line, enhancing readability. The command functions by inserting a line break right where it’s included.
Using std::endl
Another way to create new lines in C++ is through the use of `std::endl`, which not only inserts a new line but also flushes the output buffer. This flushing ensures that the output is immediately displayed on the console, which can be crucial when your program involves user interaction.
Code Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
std::cout << "Welcome to C++ Programming!" << std::endl;
return 0;
}
In this example, each call to `std::cout` is followed by `std::endl`. While this method is often more visually appealing, keep in mind that flushing the output buffer can impact performance in a program with heavy output. Thus, it is generally advisable to use `\n` when immediate output is not required.
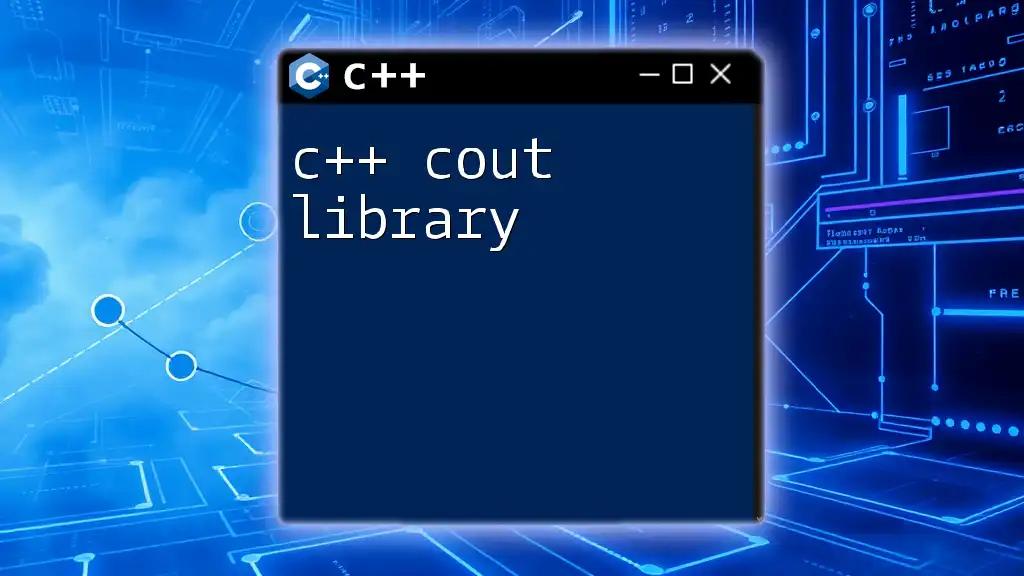
Practical Applications of New Lines in C++
Formatting Output for Better User Experience
New lines help improve user experience by organizing output in a clear and structured manner. For instance, when presenting a report, using new lines can delineate sections, aiding comprehension.
Code Example:
#include <iostream>
int main() {
std::cout << "Report:\n";
std::cout << "-----------------\n";
std::cout << "Total Sales: $5000\n";
std::cout << "Total Expenses: $3000\n";
std::cout << "Net Profit: $2000\n";
return 0;
}
In this snippet, the `\n` character separates each line while also enhancing the overall format of the report. This structured output allows readers to quickly grasp the essential information.
Combining New Lines and Other Manipulators
C++ allows for combining new lines with various output manipulators to further enhance presentation. Manipulators like `std::setw()` control the width of the output fields, providing a tidy and professional appearance.
Code Example:
#include <iostream>
#include <iomanip> // for std::setw
int main() {
std::cout << std::setw(10) << "Item" << std::setw(10) << "Price" << std::endl;
std::cout << std::setw(10) << "Apple" << std::setw(10) << "$1" << std::endl;
std::cout << std::setw(10) << "Banana" << std::setw(10) << "$0.50" << std::endl;
return 0;
}
Here, the `std::setw()` manipulator controls the width of the output for 'Item' and 'Price', resulting in a neatly aligned table. Each output line is separated by `std::endl`, ensuring that the output is clear and organized.
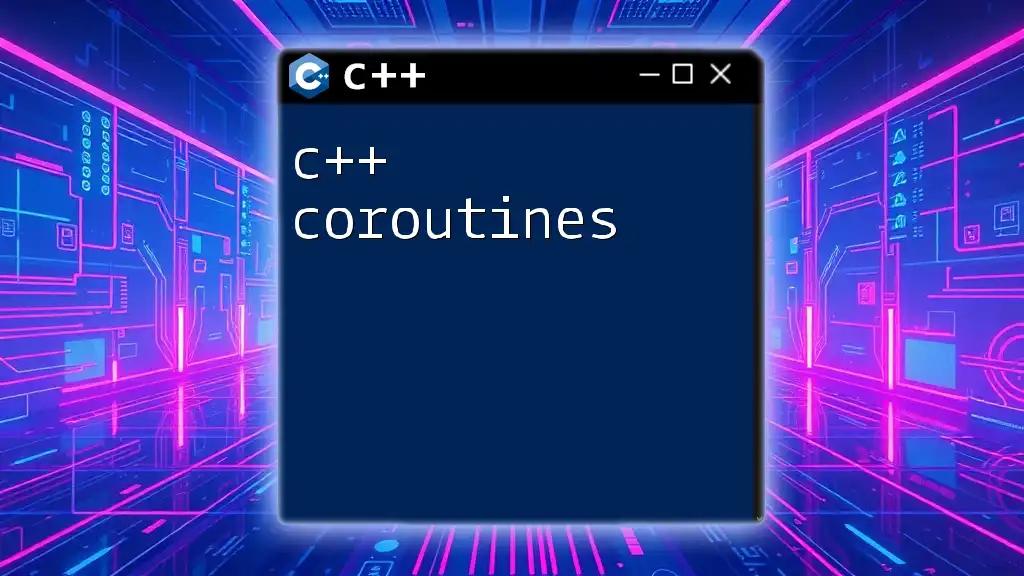
Common Mistakes and Troubleshooting
Forgetting to Include the Correct Header
When using `cout`, it's vital to remember to include the correct header file, which is `<iostream>`. Neglecting this can lead to compilation errors, as the compiler won't recognize the `cout` object. Always start your C++ files with the necessary includes to avoid unnecessary confusion.
Misunderstanding Buffer Flushing
Another common source of error is misunderstanding how output buffering works. Using `std::endl` causes the output buffer to flush, ensuring immediate display of output content. However, in programs where performance is crucial and real-time updates aren't required, excessive flushing can slow down execution. If performance is a priority, consider using `\n` for new lines while controlling the flushing manually when necessary.
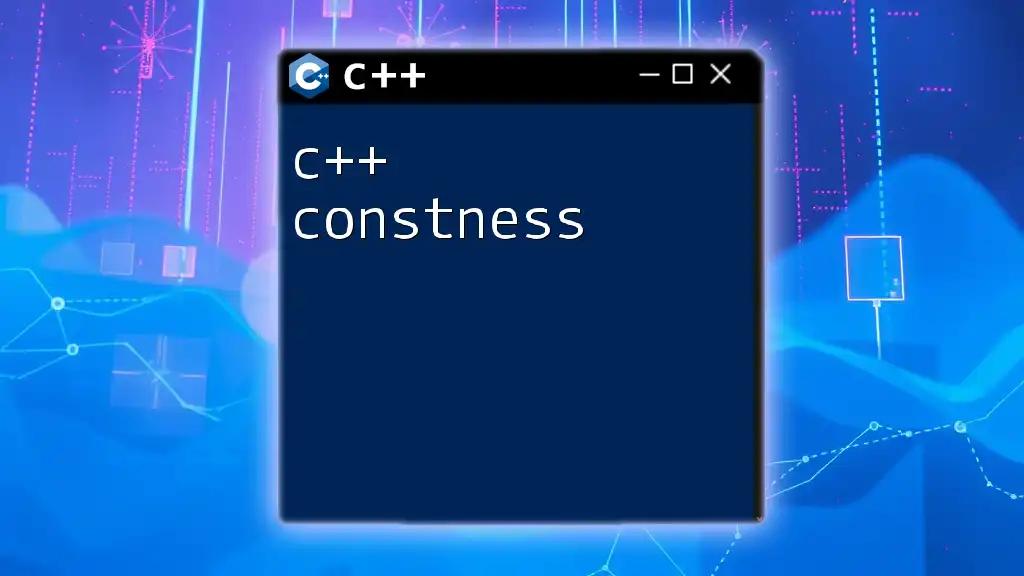
Conclusion
Understanding how to effectively use new lines with `cout` is a fundamental aspect of producing readable and organized console output in C++. By mastering both the newline escape sequence and `std::endl`, you can enhance user experience and communication through your applications. Practice formatting your outputs and explore various combinations to see the best results for your specific needs. Happy coding!