In C++, the `cout` object can display integer values in hexadecimal format using the `std::hex` manipulator. Here's a code snippet demonstrating this:
#include <iostream>
int main() {
int num = 255;
std::cout << std::hex << num << std::endl; // Outputs: ff
return 0;
}
What is Hexadecimal Representation?
Hexadecimal is a base-16 number system that uses sixteen distinct symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, A, B, C, D, E, and F. This representation contrasts with the decimal system (base-10) and binary system (base-2), making hexadecimal particularly useful in computing. Hexadecimal can represent larger values more concisely, which is especially important in contexts like memory addresses, color codes in web design, and low-level programming tasks.
One of the compelling reasons for using hexadecimal in programming is its straightforward conversion to and from binary, simplest for the human eye to interpret. For example, the binary number `11111111` converts neatly into the hexadecimal representation `FF`.
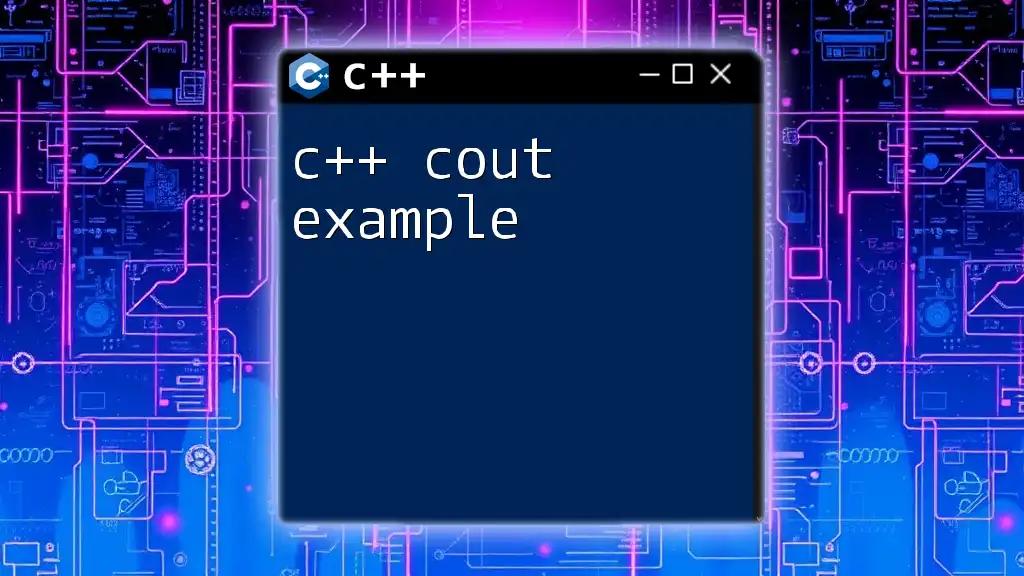
Using cout with Hexadecimal
In C++, the `std::cout` output stream allows you to display data in various formats. Using the `std::hex` manipulator is a powerful way to present integer values as hexadecimal strings. When you apply `std::hex`, all subsequent integer outputs will be displayed in hexadecimal until the manipulator is reset.
Basic Syntax of Using std::hex
To start using `std::cout` with hexadecimal representation, incorporate the following syntax. Below is a simple code snippet that converts a decimal number to its hexadecimal representation:
#include <iostream>
using namespace std;
int main() {
int number = 255;
cout << "Decimal: " << number << " in Hexadecimal: " << hex << number << endl;
return 0;
}
In this example, the program displays `255` in both decimal and hexadecimal format.
Formatting Output with cout
When working with `std::hex`, you can enhance your output using various formatting options. For example, the `std::showbase` manipulator will prepend `0x` to hexadecimal outputs, while `std::uppercase` will display letters in uppercase. These combined features can significantly enhance the clarity of your outputs.
Example of Multiple Formatters
Here’s how you can format outputs in different ways:
#include <iostream>
using namespace std;
int main() {
int number = 255;
cout << "Hex without prefix: " << hex << number << endl;
cout << "Hex with prefix: " << showbase << hex << number << endl;
cout << "Uppercase Hex: " << uppercase << hex << number << endl;
return 0;
}
In this code, you see how using `std::showbase` adds clarity, making it easy for users to identify that the number is in hexadecimal format.

Practical Use Cases
Using the `c++ cout hex` command is not just a syntactical choice; it plays an essential role in various applications. For instance, hexadecimal formats are widely used in representing memory addresses and color values in graphics programming.
Example with Memory Addresses
Consider the following code snippet that displays the memory address of a variable in hexadecimal:
#include <iostream>
using namespace std;
int main() {
int a = 42;
cout << "Address of a in Hexadecimal: " << hex << &a << endl;
return 0;
}
This program converts the address of variable `a` into hexadecimal format. Such representations can be crucial for understanding memory management and debugging.
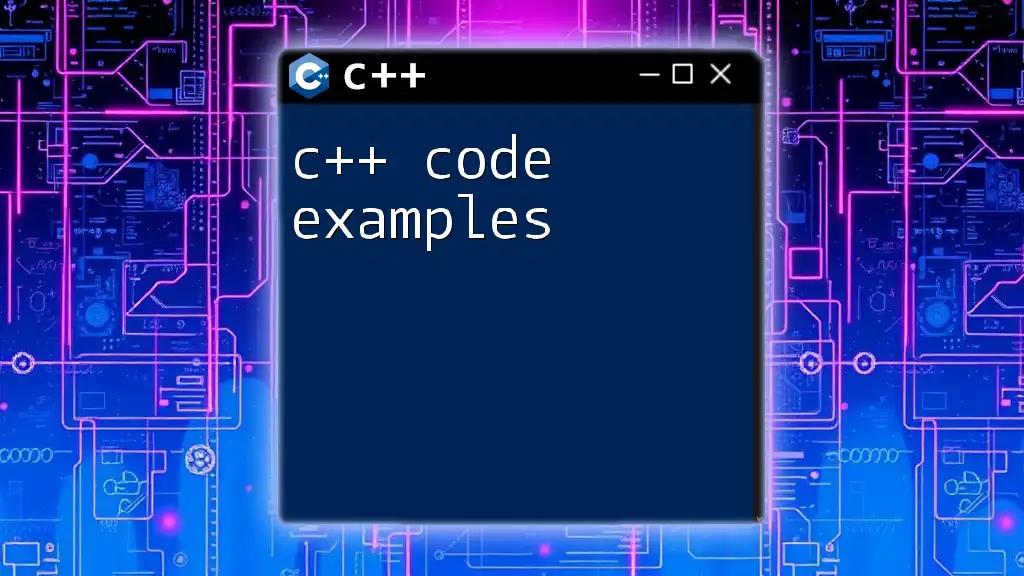
Advanced Output Formatting
C++ allows for advanced output formatting through syntax that combines multiple manipulators. This can be particularly useful in more extensive applications where clarity is essential.
Combining with Other Manipulators
To create well-formatted output, you can combine `std::hex` with manipulators like `std::setw` and `std::setfill`. This allows you to create a more structured output that can benefit user experience.
Code Example
Here's how you can implement advanced formatting:
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
int number = 4500;
cout << "Formatted Hex: " << setfill('0') << setw(4) << hex << number << endl;
return 0;
}
By using `setfill('0')` and `setw(4)`, output values are padded with zeros, ensuring a consistent width in the output, which can be vital for alignment in tabular data.
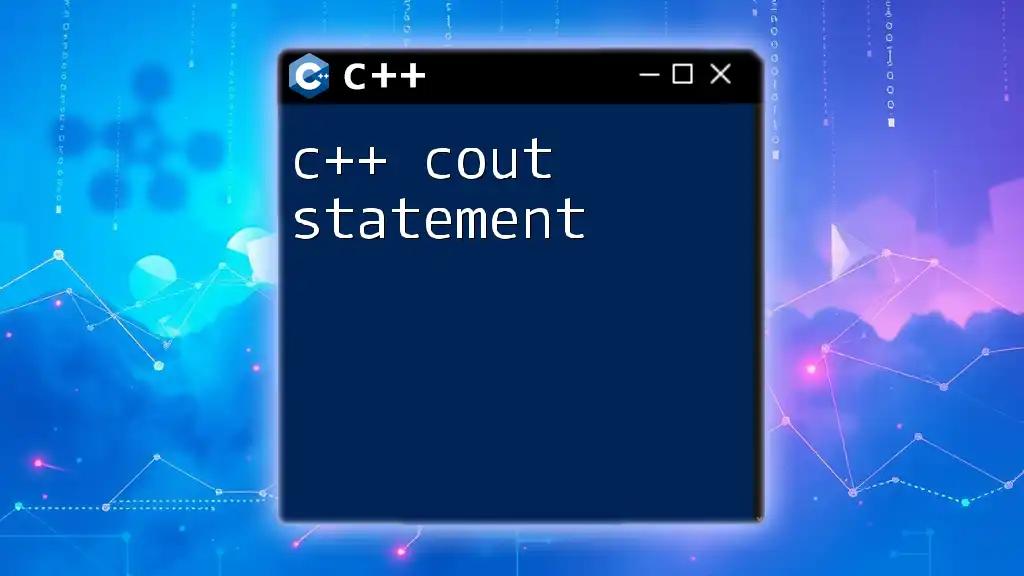
Common Pitfalls
When using `std::cout` with hexadecimal output, there are common issues that beginners may encounter.
Accidentally Mixing Decimal and Hexadecimal Outputs
One prevalent mistake is mixing decimal and hexadecimal outputs without considering their formats. This can lead to confusing outputs that may mislead users.
Not Resetting Formatters
Another common pitfall is forgetting to reset manipulators. If you print several values after setting `std::hex`, all of them will default to hexadecimal, which may not be your intent.
Example of a Pitfall
Consider the following program:
#include <iostream>
using namespace std;
int main() {
cout << "Decimal: " << 15 << endl;
cout << "Hex: " << hex << 15 << " Decimal: " << 15 << endl; // Confusing output
return 0;
}
In this snippet, although the decimal value `15` is processed correctly the first time, the second line’s `Decimal: 15` output is also affected by the previously set hex format, resulting in unintentional confusion.
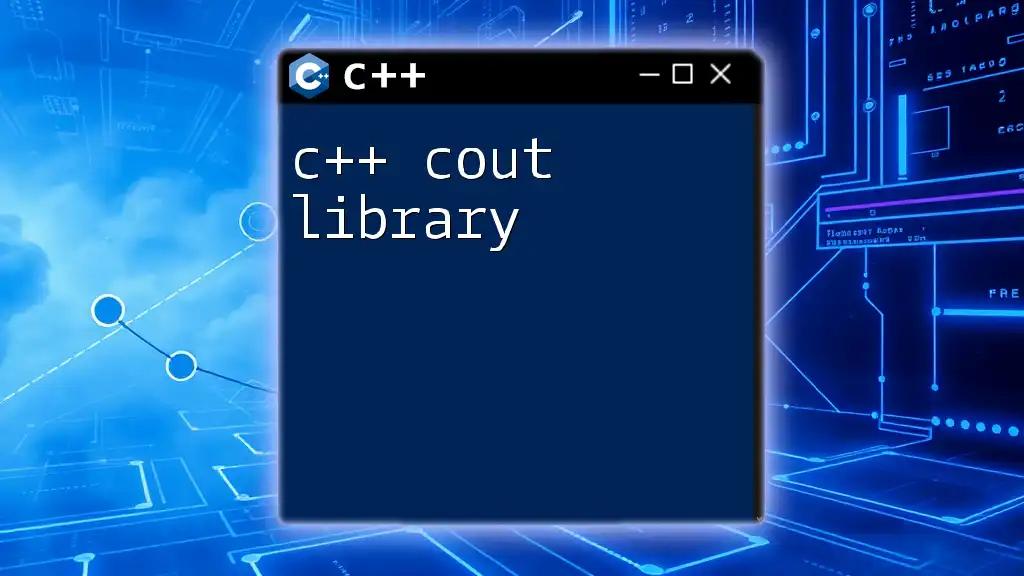
Best Practices for Using cout with Hexadecimal
To make effective use of `c++ cout hex`, consider implementing best practices.
Tips for Clear Output
- Keep hex outputs consistent: Decide when to use hexadecimal representations to prevent ambiguity in your outputs.
- Commenting your code: Use comments to document the intent behind hexadecimal outputs so future developers can follow your reasoning.
README for Developers
Documentation and clear coding styles aid in collaboration. Encourage good practices by creating README files that outline expected output formats and coding styles. This promotes consistency across your codebase.
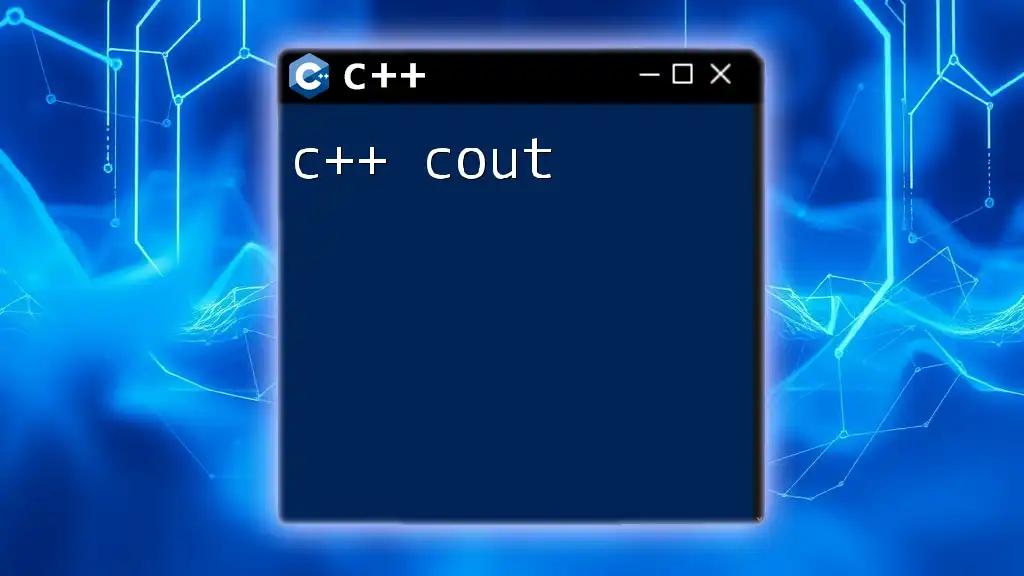
Conclusion
Using the `c++ cout hex` feature allows for clear representation of numeric data and enhances the readability of your output. Experimenting with various formatting options enriches your programming experience. Don't hesitate to dive deeper into hexadecimal usage to unlock its full potential in your coding arsenal!
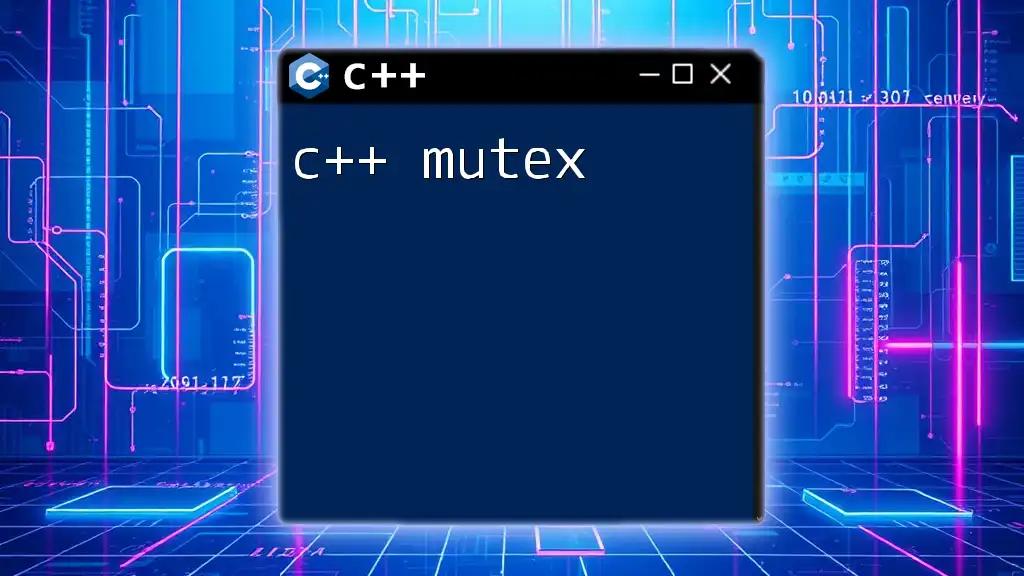
Call to Action
We want to hear from you! If you found this guide helpful or have further questions about using hexadecimal in C++, please leave a comment below. Also, subscribe to our blog for more insights and tips about C++ programming. Happy coding!