In C++, you can use `cout` to print Unicode characters by including the appropriate headers and using wide character strings. Here's a code snippet demonstrating this:
#include <iostream>
#include <locale>
#include <codecvt>
int main() {
// Set the locale to support Unicode
std::locale::global(std::locale("en_US.UTF-8"));
std::wcout << L"Hello, Unicode: 😊 こんにちは" << std::endl;
return 0;
}
Understanding Unicode
What is Unicode?
Unicode is a comprehensive character encoding standard that allows the representation of text in computers and other devices. It extends beyond the limitations of ASCII (American Standard Code for Information Interchange) by supporting an extensive range of characters from various languages and scripts. Unicode enables the accurate representation of text, ensuring that users can communicate in their native languages without compromising integrity.
Unicode Character Sets
Unicode encompasses different encoding systems, primarily UTF-8, UTF-16, and UTF-32. UTF-8 is the most commonly used encoding format on the web because it is both compact and backward compatible with ASCII. UTF-16 and UTF-32 represent characters in a larger number of bytes but can be advantageous for certain internal processing tasks. Understanding these encoding strategies is crucial for any modern application that intends to handle international text, making it a fundamental aspect when working with `c++ cout unicode`.
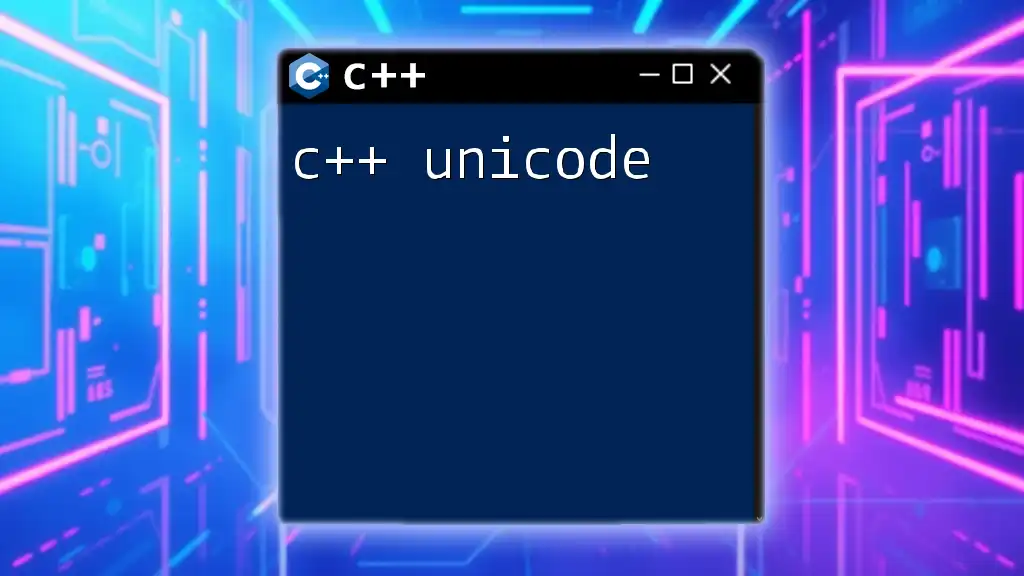
Setting Up Your Environment for Unicode Support
Requirements for C++ Unicode Support
Make sure you are using a modern C++ compiler that supports Unicode. Compilers such as GCC, Clang, and MSVC (Microsoft Visual C++) fully support Unicode starting from C++11.
Configuring Your IDE for Unicode Output
Proper configuration of your Text Editor or Integrated Development Environment (IDE) is crucial for handling Unicode output. Here are some insights into configuring popular IDEs:
- Visual Studio: Ensure that your project properties are set to "Unicode" under Character Set options.
- Code::Blocks: Set the encoding of your source files to UTF-8 to handle Unicode characters effectively.
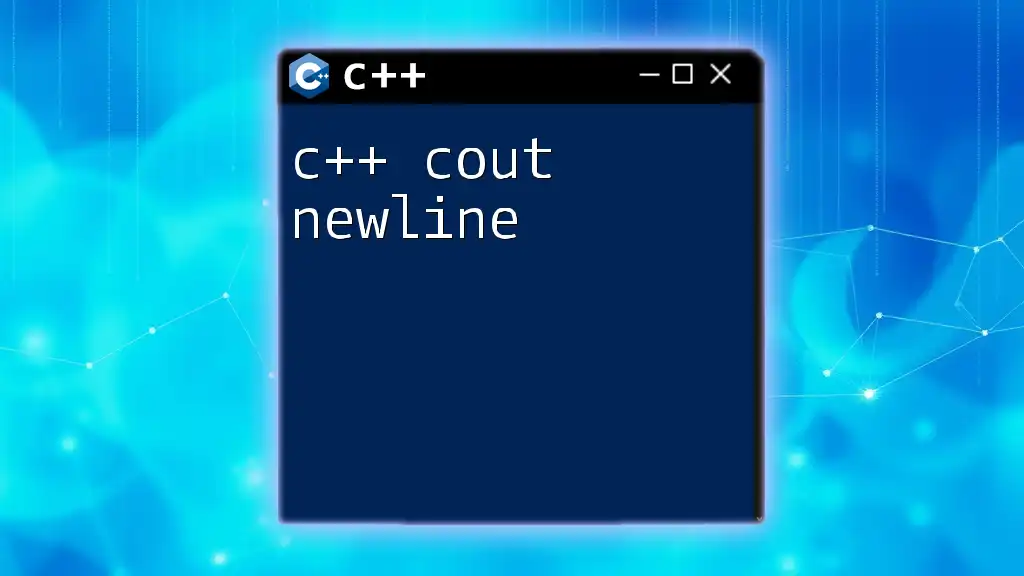
Using Unicode with C++ cout
Basic Output with cout
To get started with `cout`, simply use the standard output stream found in the `iostream` library. Here is a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code outputs a simple greeting to the console. However, to incorporate Unicode characters, adjustments will be required.
Outputting Unicode Characters
Directly in Code
You can include Unicode characters directly in your C++ code. Make sure that your source file is saved in UTF-8 encoding. Below is a simple example:
#include <iostream>
using namespace std;
int main() {
cout << "Here is a heart: ❤️" << endl;
return 0;
}
This code effectively demonstrates how to output a Unicode character, such as a heart symbol, directly within your program.
Using Unicode Escape Sequences
An alternative approach is to utilize escape sequences to represent Unicode characters. Each character has a unique escape sequence that you can use. Here's how to output a black star:
#include <iostream>
using namespace std;
int main() {
cout << "Here is a star: \u2605" << endl; // Black Star
return 0;
}
The `\u` prefix tells the compiler to treat the following 4 hexadecimal digits as a Unicode character, resulting in the star being printed to the console.
Outputting Unicode from External Files
Sometimes, reading Unicode text from external files is necessary. This requires the use of wide-character streams (wifstream) for Unicode support. Below is an example of how to read and output Unicode strings:
#include <iostream>
#include <fstream>
#include <string>
#include <locale>
#include <codecvt>
using namespace std;
int main() {
wifstream file("unicode_text.txt");
file.imbue(locale(file.getloc(), new codecvt_utf8<wchar_t>));
wstring line;
while (getline(file, line)) {
wcout << line << endl;
}
file.close();
return 0;
}
In this example, we set up a wide input stream with the appropriate locale for UTF-8 encoding, enabling us to read Unicode text stored in a file.
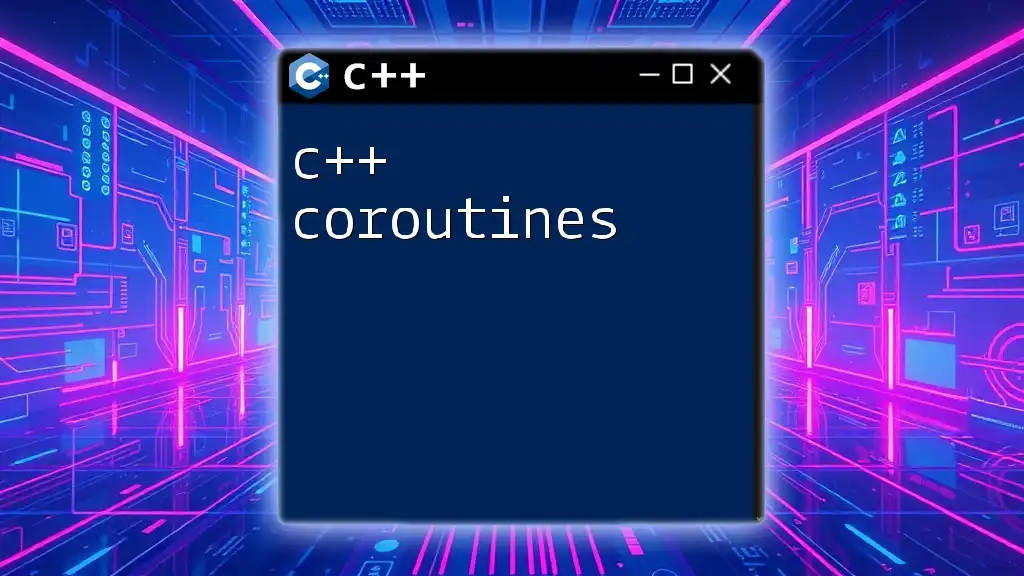
Best Practices for Unicode Output
Ensuring Proper Encoding
To ensure that your output streams are set up to handle Unicode correctly, it's essential to check your settings and use the proper encodings across all components of your application. Inconsistent encoding between source files, libraries, and output can lead to display issues or loss of character representation. Consistency is key!
Handling Locale
Locale plays a significant role in determining how text is formatted and displayed. You can set and switch locales in C++ to accommodate different languages and regions. Here’s how to set the locale for your output:
#include <iostream>
#include <locale>
using namespace std;
int main() {
locale loc("en_US.UTF-8");
cout.imbue(loc);
cout << "English: Hello" << endl;
locale loc_fr("fr_FR.UTF-8");
cout.imbue(loc_fr);
cout << "French: Bonjour" << endl;
return 0;
}
Setting the locale helps ensure that your text representation matches user expectations based on their language or region.
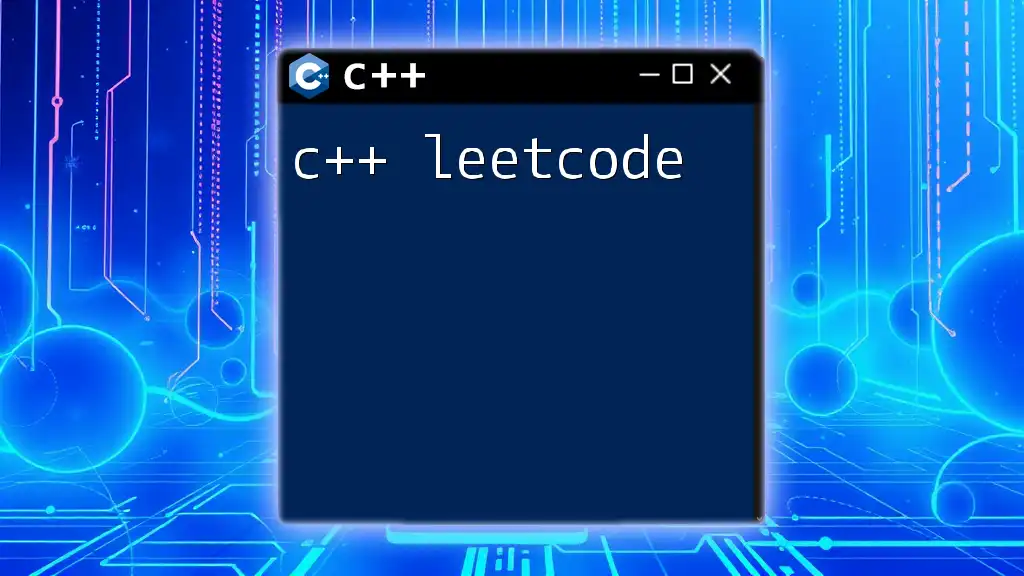
Troubleshooting Unicode Output Issues
Common Problems
When working with Unicode output in C++, you might encounter several common issues. These include:
- Characters not displayed correctly: This often occurs when the output does not support the character encoding used.
- Byte order mark (BOM) issues: If your text files include a BOM, some programs might misinterpret the encoding.
Solutions and Workarounds
If you're facing these issues, check the following:
- Compiler Settings: Confirm that your compiler is set to handle Unicode.
- Encoding Consistency: Ensure that all parts of your program, including files and libraries, use UTF-8 or the same consistent encoding.
- Debugging Display Issues: Using a hexadecimal display might help identify underlying character encoding issues when output appears incorrect.
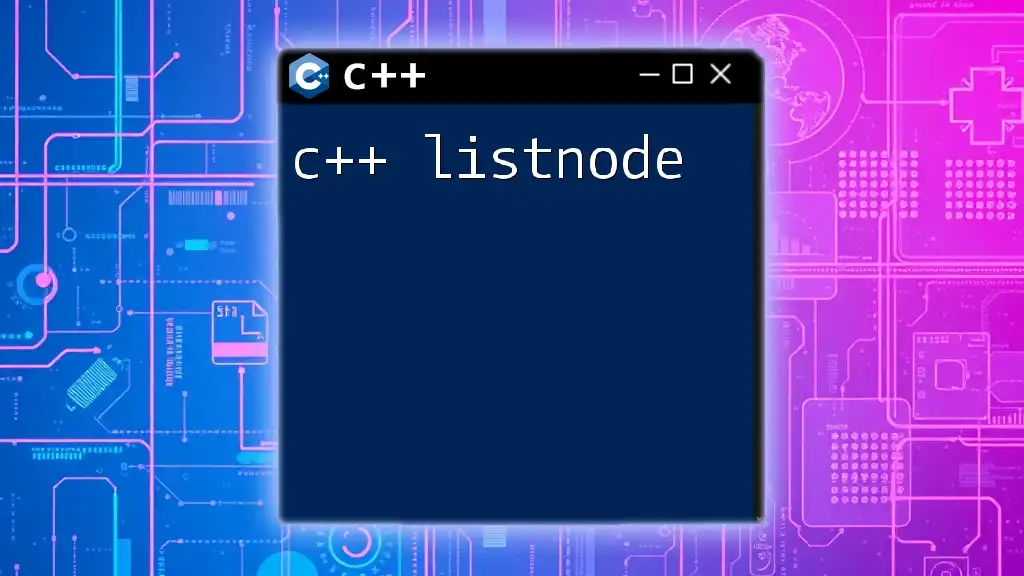
Conclusion
In this comprehensive guide, we've explored the various aspects of working with `c++ cout unicode`. By understanding Unicode, setting up your environment properly, using `cout` to output Unicode characters, adhering to best practices, and resolving common issues, you can successfully incorporate Unicode in your C++ applications.
As you continue to explore programming nuances, consider engaging with resources that delve deeper into languages and their representations. Our company is here to assist you with more programming tips and tutorials to enhance your skills further!