C++ pseudocode is a high-level representation of a program's logic that uses simplified syntax to outline algorithms without getting bogged down in the complexities of actual C++ syntax.
// Example C++ pseudocode for a simple program that adds two numbers
START
DECLARE num1, num2, sum AS INTEGER
PRINT "Enter first number:"
INPUT num1
PRINT "Enter second number:"
INPUT num2
sum = num1 + num2
PRINT "The sum is:", sum
END
Understanding the Basics of C++ Pseudocode
What is Pseudocode? Pseudocode is an informal way of describing a program's logic or algorithm in a human-readable format. Unlike actual programming languages, pseudocode does not conform to strict syntax rules, allowing you to focus on the concept instead of the details. This makes it an essential tool for both novice and seasoned programmers who want to map out their thoughts before diving into coding.
Why Use Pseudocode in C++? Utilizing pseudocode in the C++ programming process has several benefits. It simplifies problem-solving by breaking complex problems into understandable steps. Additionally, it enhances comprehension, enabling developers to visualize the structure of their code before implementation. Ultimately, it serves as a bridge between human language and programming languages, fostering clearer communication of ideas.
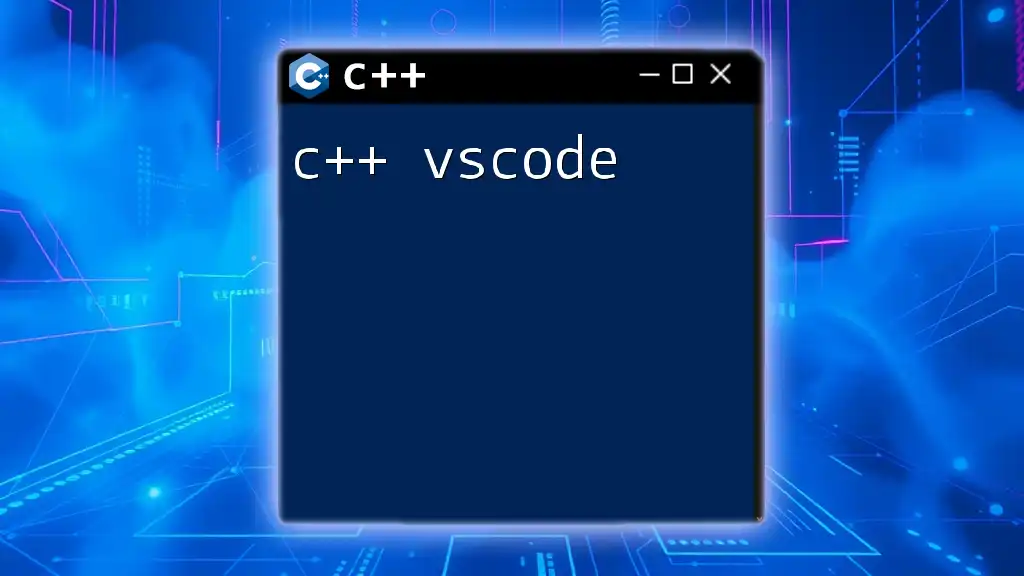
Writing Pseudocode for C++
To craft effective pseudocode, it’s crucial to follow a structured approach. The following steps provide guidance in writing coherent and purposeful pseudocode.
Steps to Write Effective Pseudocode:
-
Identify the Problem Requirements: Begin by clearly defining what the program is supposed to accomplish. Understanding the goals will shape your pseudocode and ensure that it remains focused.
-
Break Down the Problem: Divide the problem into smaller, manageable parts. This segmentation makes it easier to construct logical steps.
-
Use Simple Language: Your pseudocode should use straightforward language that conveys ideas clearly and succinctly. Avoid complex jargon, making your pseudocode accessible to those with varied programming backgrounds.
Example of Pseudocode Structure
In C++ pseudocode, clear input and output representations are vital. Here are some key components to include:
- Input/Output Representation: Use keywords like `INPUT`, `OUTPUT`, or `PROMPT` to denote data exchange.
- Control Structures: Ensure you correctly implement decision-making processes through structures such as if-else statements or loops.
Pseudocode Example C++
Here is an example of simple pseudocode that outlines a program to add two numbers:
BEGIN
DECLARE NUMBER1, NUMBER2, SUM AS INTEGER
PROMPT "Enter first number" INTO NUMBER1
PROMPT "Enter second number" INTO NUMBER2
SUM = NUMBER1 + NUMBER2
OUTPUT "The sum is" + SUM
END
In this example, we clearly identify the beginning and ending of the program, declare variables, take user input, perform calculations, and output results.
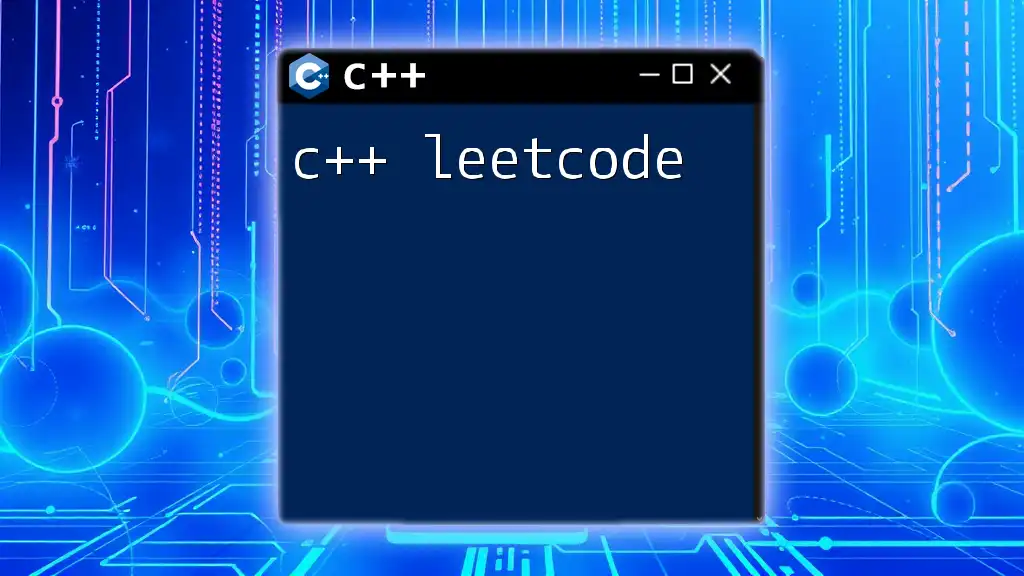
Pseudocode for Common C++ Algorithms
When working with C++, you may often need to write pseudocode for common algorithms. Two basic types of algorithms you would benefit from practicing include sorting and searching algorithms.
Sorting Algorithms
Understanding sorting is fundamental in programming. For example, let’s consider a simple Bubble Sort algorithm:
FUNCTION BUBBLE_SORT(ARRAY)
FOR i FROM 0 TO LENGTH(ARRAY) - 1
FOR j FROM 0 TO LENGTH(ARRAY) - i - 1
IF ARRAY[j] > ARRAY[j + 1] THEN
SWAP ARRAY[j] WITH ARRAY[j + 1]
END IF
END FOR
END FOR
END FUNCTION
This pseudocode illustrates the mechanics of Bubble Sort, emphasizing clarity in its structure and flow without diving into C++ syntax.
Searching Algorithms
Similarly, when it comes to searching for an element in an array, you can implement a Linear Search. Below is the pseudocode for that:
FUNCTION LINEAR_SEARCH(ARRAY, TARGET)
FOR i FROM 0 TO LENGTH(ARRAY) - 1
IF ARRAY[i] = TARGET THEN
RETURN i
END IF
END FOR
RETURN -1
END FUNCTION
This example concisely outlines how to search for an element, making the procedure of checking each array item explicit.
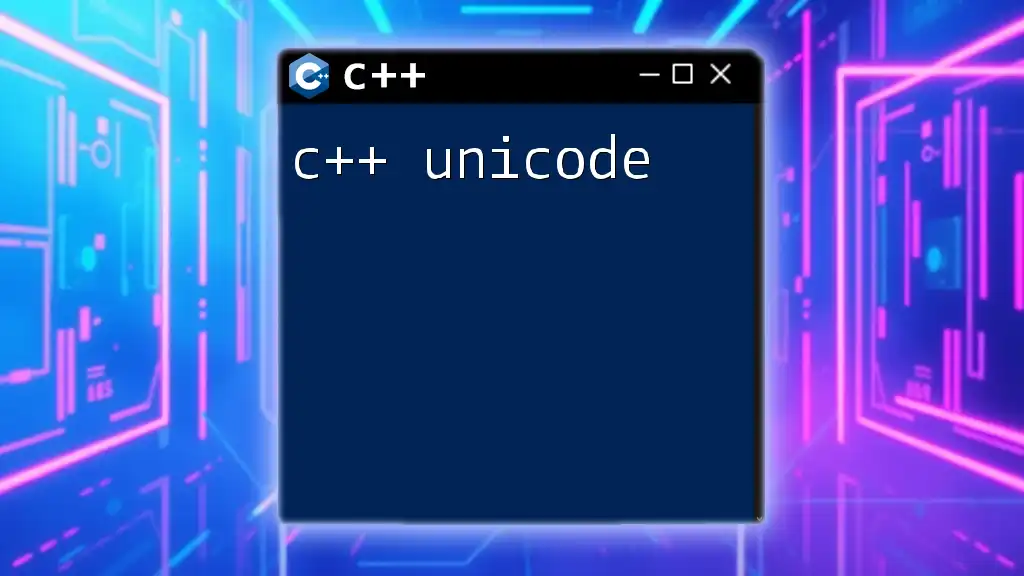
Translating Pseudocode into C++
Once you have written the pseudocode, the next logical step is translating it into actual C++ code. This process involves understanding the basic syntax and structures of C++, allowing you to turn your ideas into functional applications.
From Pseudocode to C++ Code: Here’s how the earlier pseudocode example is transformed into C++:
#include <iostream>
int main() {
int number1, number2, sum;
std::cout << "Enter first number: ";
std::cin >> number1;
std::cout << "Enter second number: ";
std::cin >> number2;
sum = number1 + number2;
std::cout << "The sum is " << sum << std::endl;
return 0;
}
This example reflects the logical steps from the pseudocode by using proper C++ syntax while maintaining clarity.
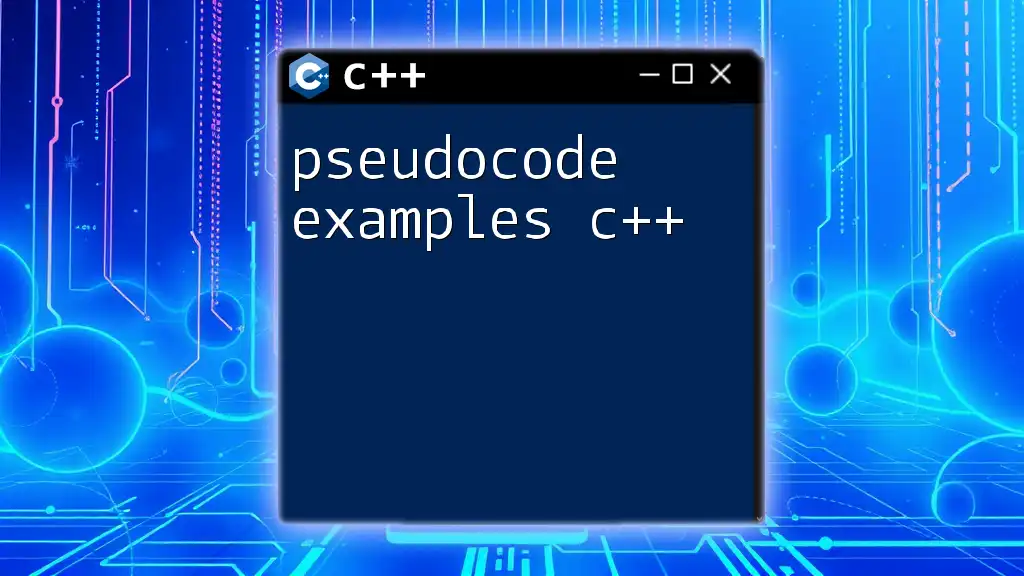
Practical Tips for Writing Pseudocode
To improve the effectiveness of your pseudocode, consider the following best practices:
-
Keep it Simple and Concise: Striving for brevity enhances readability. Unnecessary details can clutter your pseudocode.
-
Use Self-Explanatory Names: Meaningful variable names will make your pseudocode clearer. Avoid vague identifiers that may confuse readers.
-
Review and Revise: Once drafted, take the time to revise your pseudocode for clarity and accuracy. A second read can help you spot ambiguous segments that may confuse readers.
Common Mistakes to Avoid:
Avoid the temptation to overcomplicate your logic. Pseudocode is meant to be a simplified representation of your code. Stick to standard conventions and structure to ensure clarity.
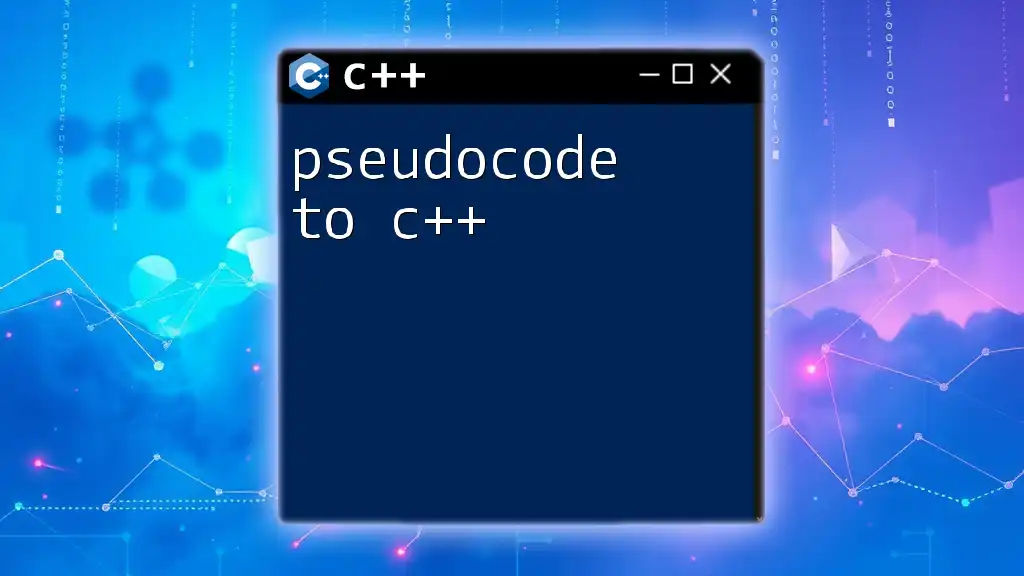
Tools and Resources for Pseudocode
There are several tools available that facilitate the writing of pseudocode. Online pseudocode editors can assist in formatting and structuring your notes. Various platforms provide templates and gathering places where you can share and collaborate on your pseudocode projects.
For further reading, consider exploring books that focus on programming logic and algorithm development. Online forums and programming communities can also offer insights and support as you refine your skills.
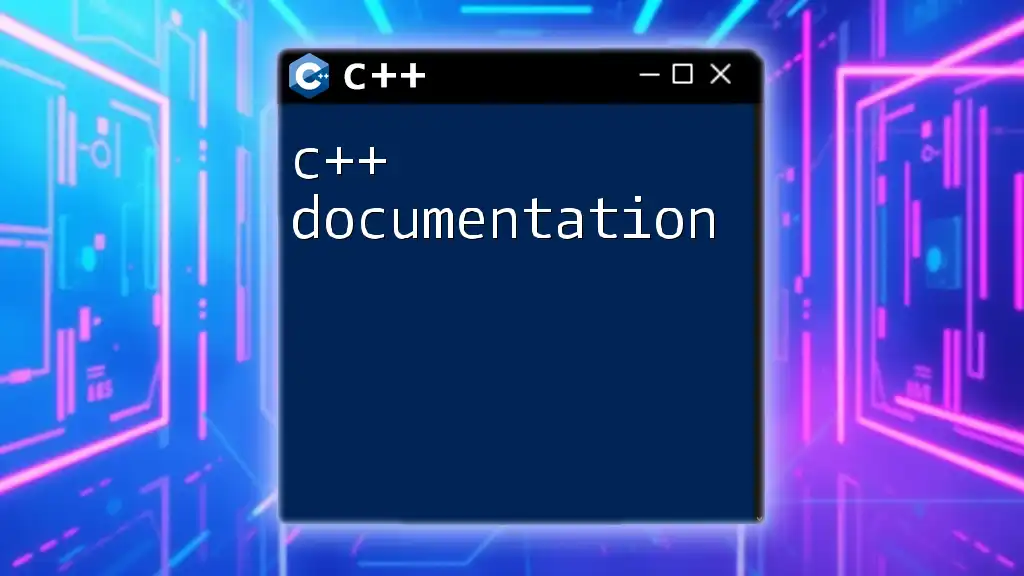
Conclusion
By incorporating C++ pseudocode into your programming practices, you can significantly improve your problem-solving abilities and code quality. It encourages a structured approach to programming that clarifies your thoughts. Start today by practicing writing pseudocode for different programming tasks, and you will see how much more effectively you can write your C++ code.