C++ sockets provide a means for network communication between devices, enabling the sending and receiving of data across networks using TCP/IP protocols.
Here's a simple example of creating a TCP socket server in C++:
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
int server_fd, new_socket;
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
// Creating socket file descriptor
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
std::cerr << "Socket failed" << std::endl;
return -1;
}
// Attaching socket to the port 8080
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
// Binding the socket
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
// Listening for incoming connections
listen(server_fd, 3);
std::cout << "Listening on port 8080..." << std::endl;
// Accepting a connection
new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen);
std::cout << "Connection accepted" << std::endl;
// Close the socket
close(new_socket);
close(server_fd);
return 0;
}
Understanding Sockets
A socket is an endpoint for sending or receiving data across a computer network. It serves as a communication channel between two applications, whether they reside on the same machine or on different machines connected over a network. The essence of socket programming lies in enabling this interaction.
Socket programming is crucial in modern software development because most applications require some form of network communication—whether it be a web server, a chat application, or a file transfer program. Understanding how to properly use sockets is foundational for anyone looking to develop networked applications using C++.
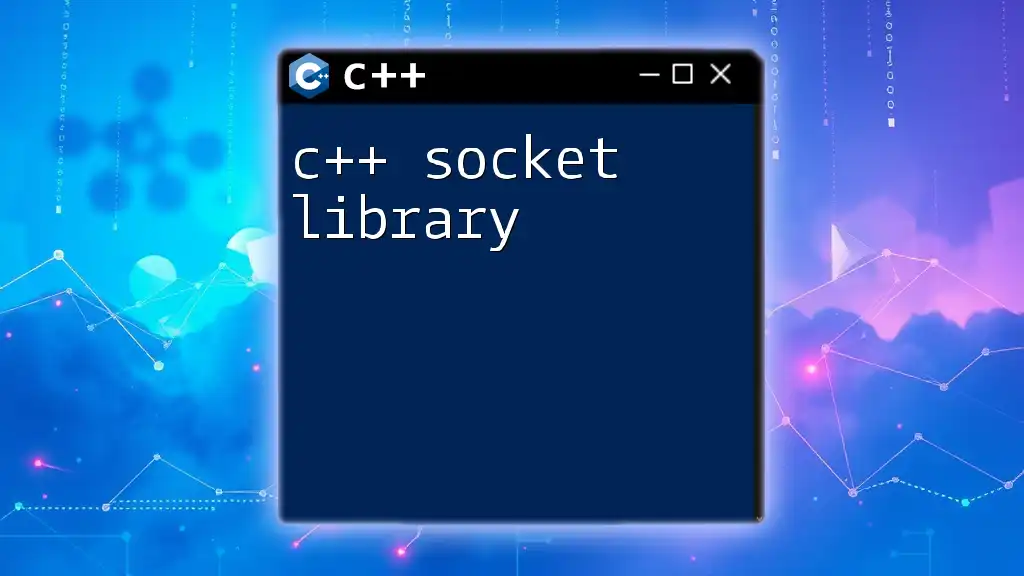
What is C++ Socket Programming?
C++ socket programming refers to the practice of using C++ to interact with sockets. Unlike some other languages that provide built-in networking libraries, C++ requires the use of system-level socket APIs. These tools allow developers to create, configure, and manage sockets effectively.
There are two primary types of sockets to be aware of:
- TCP Sockets: Used for reliable, connection-oriented communication. TCP (Transmission Control Protocol) ensures that data packets are delivered in order and error-free.
- UDP Sockets: Used for connectionless communication. UDP (User Datagram Protocol) is faster but does not guarantee packet delivery, making it suitable for applications where speed is more critical than reliability, such as video streaming or online gaming.
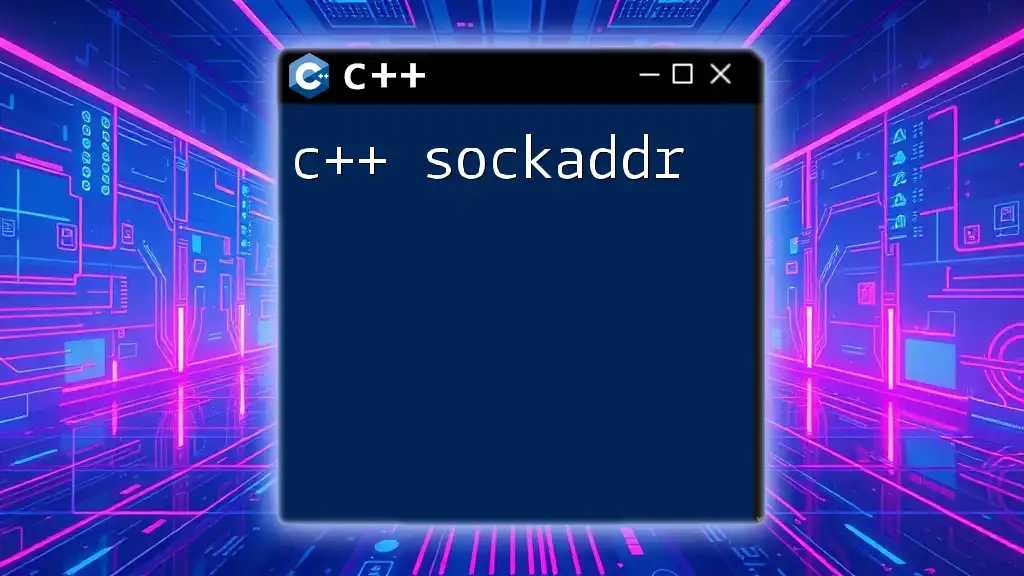
Setting Up Your C++ Development Environment
Required Libraries
To start programming with C++ sockets, you typically need to include certain header files and link against specific libraries. For Linux systems, the necessary headers include:
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <cstring>
On Windows, the setup is slightly different due to the different socket APIs provided by Winsock. You will use:
#include <winsock2.h>
#pragma comment(lib, "ws2_32.lib")
Make sure to initialize Winsock with `WSAStartup()` before using sockets.
Sample Project Structure
A basic C++ socket application structure might look like this:
my_socket_app/
├── src/
│ ├── server.cpp
│ └── client.cpp
├── include/
│ └── socket_utils.h
├── lib/
├── Makefile
This structure keeps your code organized, separating source files, header files, and libraries.
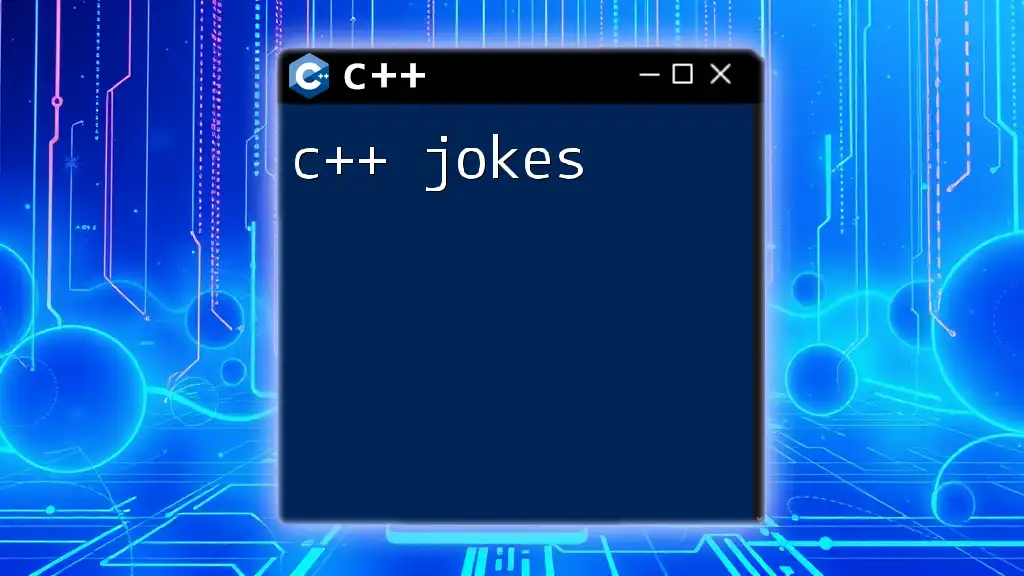
C++ Socket Basics
Creating a Socket
The first step in socket programming is creating a socket. This is achieved through the `socket` function, which returns a socket descriptor.
Here’s a simple example of how to create a socket in C++:
#include <sys/socket.h>
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("Socket creation failed");
}
Types of Sockets
Stream Sockets
Stream sockets (TCP) provide a reliable connection, ensuring that data packets are delivered in sequence. This type of socket is indispensable for applications that require guaranteed delivery, such as HTTP servers or FTP.
Datagram Sockets
On the other hand, datagram sockets (UDP) are faster than stream sockets but lack the reliability of TCP. They can be used in applications where speed is critical, and the occasional loss of data packets is acceptable, such as in real-time applications like VoIP.
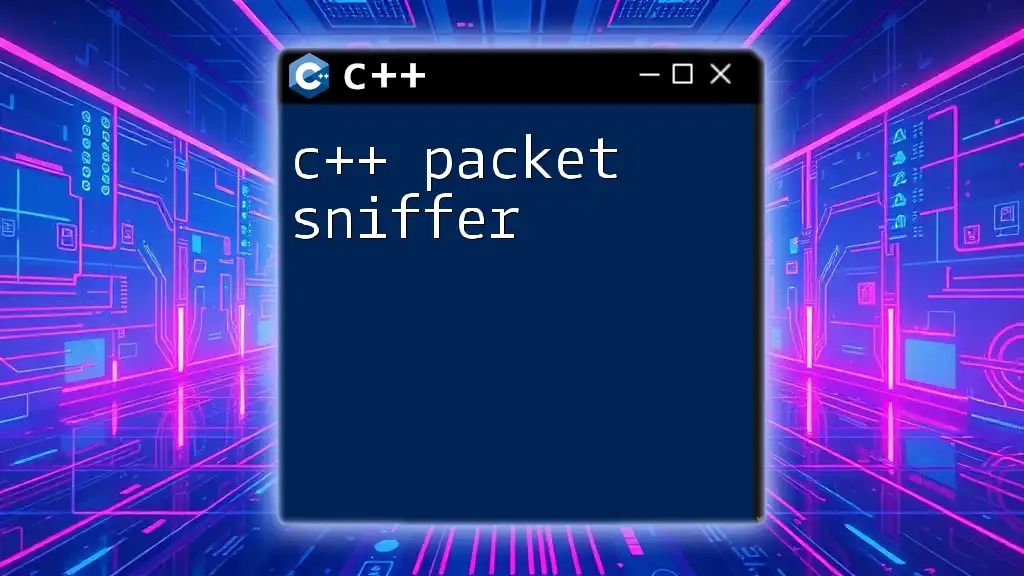
Socket Programming in C++
Server-Side Socket Programming
Creating a Server Socket
For a server to accept client connections, it must first create a server socket. This involves setting up the socket and binding it to an address.
Here’s how you might do this:
#include <netinet/in.h>
// Create socket
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in address;
// Fill address with port number and IP
Binding and Listening
After creating the socket, you need to bind it to a specific IP address and port using the `bind` function, and then you can listen for incoming connections.
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
This sets up the server to listen for connections on the specified port.
Accepting Connections
Once the server is listening, it can accept incoming client connections using the `accept` function.
Here’s an example of how to use it:
int new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen);
This function blocks until a client connects, at which point it returns a new socket descriptor for the accepted connection.
Client-Side Socket Programming
Creating a Client Socket
Similar to the server, the client must create a socket for connecting to the server:
int sock = 0;
sock = socket(AF_INET, SOCK_STREAM, 0);
Connecting to the Server
To establish a connection, the client uses the `connect` function, specifying the server's address and port number.
connect(sock, (struct sockaddr *)&server_address, sizeof(server_address));
This call attempts to connect the client socket to the server. If successful, the client can now send and receive data.
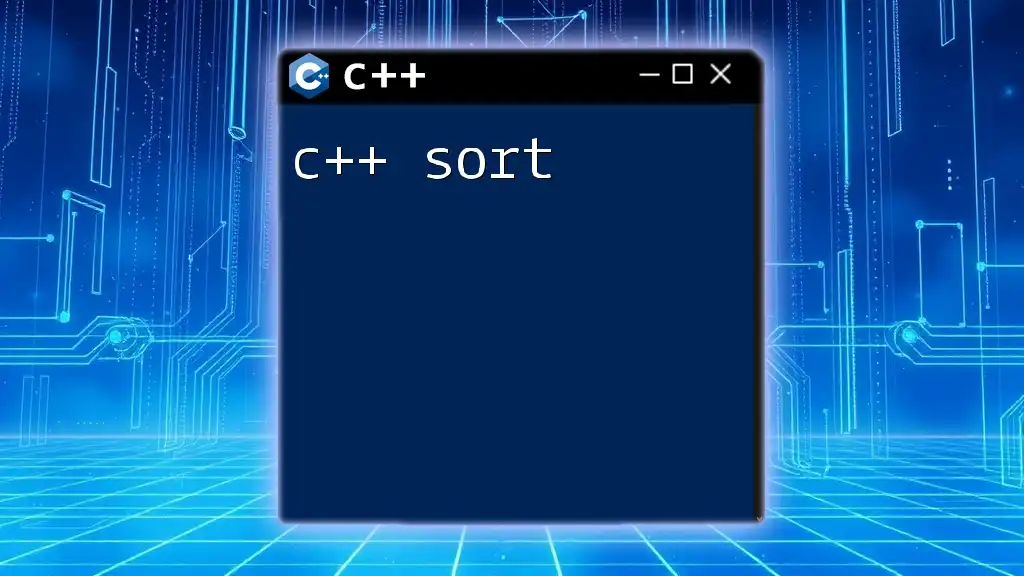
Data Transmission in Socket Programming
Sending and Receiving Data
Once the connection is established, both the client and server can communicate by sending and receiving data. C++ provides the `send` and `recv` functions for this purpose.
A simple sending and receiving example might look like this:
send(sock, "Hello from Client", strlen("Hello from Client"), 0);
recv(new_socket, buffer, sizeof(buffer), 0);
This code snippet demonstrates how a client sends a message to the server, which can then receive it.
Handling Multiple Connections
Introduction to Multithreading
Handling multiple connections is an essential aspect of socket programming, especially for server applications. One effective way to manage multiple clients is through multithreading.
Using C++ Standard Library or a threading library, you can create a new thread for each client connection.
Example of a Multi-Client Server
Here’s a simple structure for a multithreaded server:
std::thread t(handleClient, new_socket);
In this case, `handleClient` is a function that manages communication with the connected client.
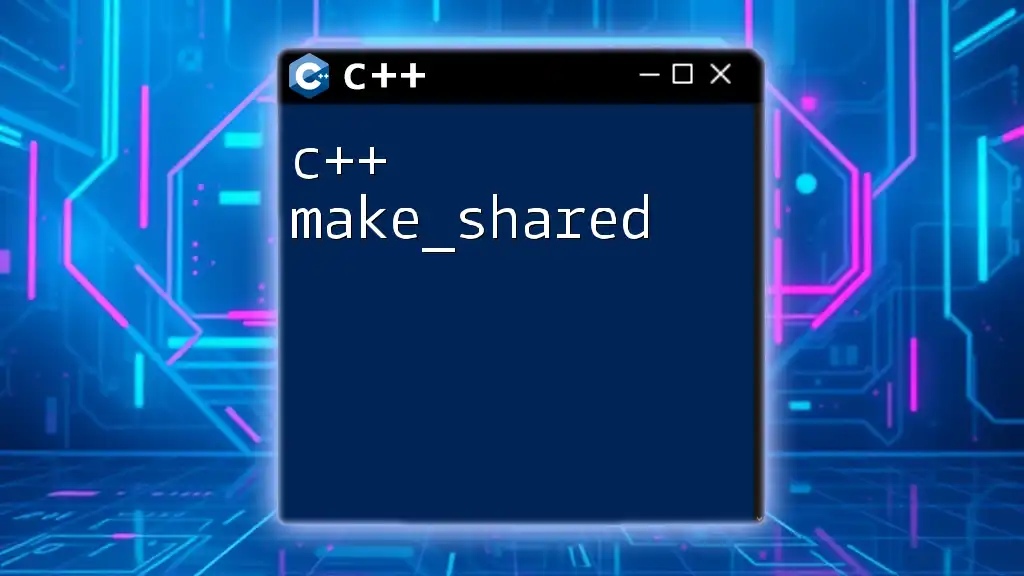
Error Handling in Socket Programming
Common Socket Errors
Error handling is critical in socket programming. Common errors include failed socket creation, binding issues, or connection problems. You can use the `errno` variable to understand the nature of the error.
Best Practices for Error Handling
Implementing robust error handling adds reliability to your application. For example, check the return values of socket functions and print meaningful error messages when something goes wrong:
if (sock < 0) {
perror("Socket creation error");
exit(EXIT_FAILURE);
}
This practice helps in debugging and ensuring that your application can gracefully handle unexpected conditions.
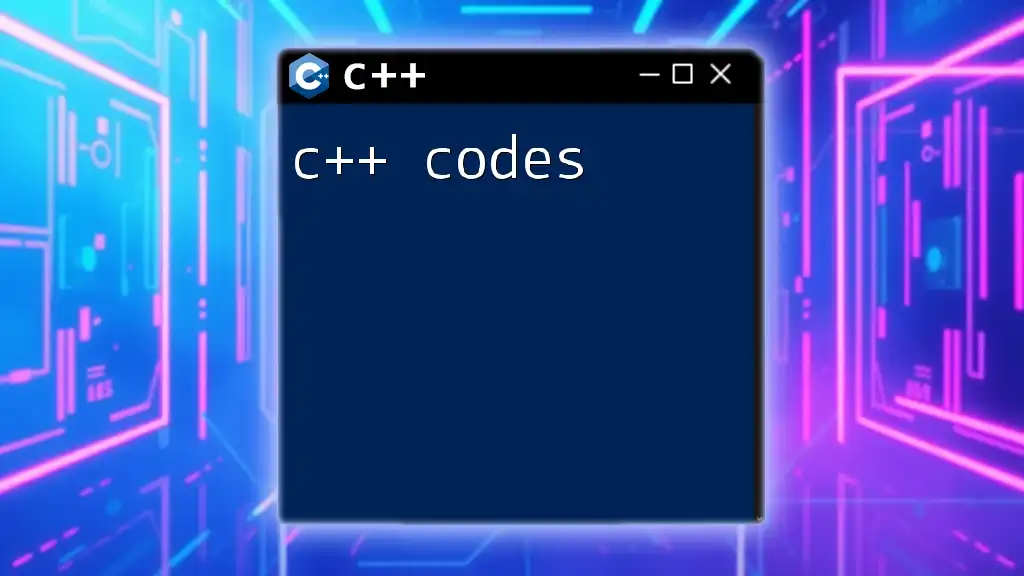
Conclusion
C++ socket programming is a powerful skill that opens doors to building a variety of networked applications. From creating simple TCP/IP servers to developing complex real-time systems, understanding how to work with C++ sockets is essential for every developer interested in network programming.
By mastering the concepts and techniques outlined in this guide, you can create robust, efficient, and reliable applications that communicate over networks. Practice by implementing your own socket applications, and don’t hesitate to refer to available resources for further learning and support.