In C++, `clock_t` is a data type defined in the `<ctime>` header that is used to measure processor time consumed by a program, allowing you to track performance by recording the clock ticks at the start and end of a process.
Here's a code snippet to demonstrate its usage:
#include <iostream>
#include <ctime> // Include for clock_t and clock()
int main() {
clock_t start = clock(); // Record the starting clock tick
// Code block whose execution time is measured
for (long i = 0; i < 1e6; ++i); // Dummy workload
clock_t end = clock(); // Record the ending clock tick
double duration = static_cast<double>(end - start) / CLOCKS_PER_SEC; // Calculate duration in seconds
std::cout << "Execution time: " << duration << " seconds." << std::endl;
return 0;
}
The clock_t Type
Definition of clock_t
In C++, `clock_t` is a data type that is used to represent processor time. It allows developers to measure the amount of CPU time consumed by a program's execution. This information is crucial for performance analysis, as it helps developers identify bottlenecks and optimize resource usage. The `clock_t` type is defined in the `<ctime>` header, which includes the functions and types necessary for handling time measurements.
Why Use clock_t?
Using `clock_t` to measure performance provides a level of accuracy that is beneficial for most applications. Compared to other timing mechanisms, such as `time(NULL)` which measures real-world time, `clock_t` focuses solely on CPU processing time. This distinction is particularly useful for benchmarking algorithms and understanding their efficiency without being influenced by external factors, such as system load or user interactions.
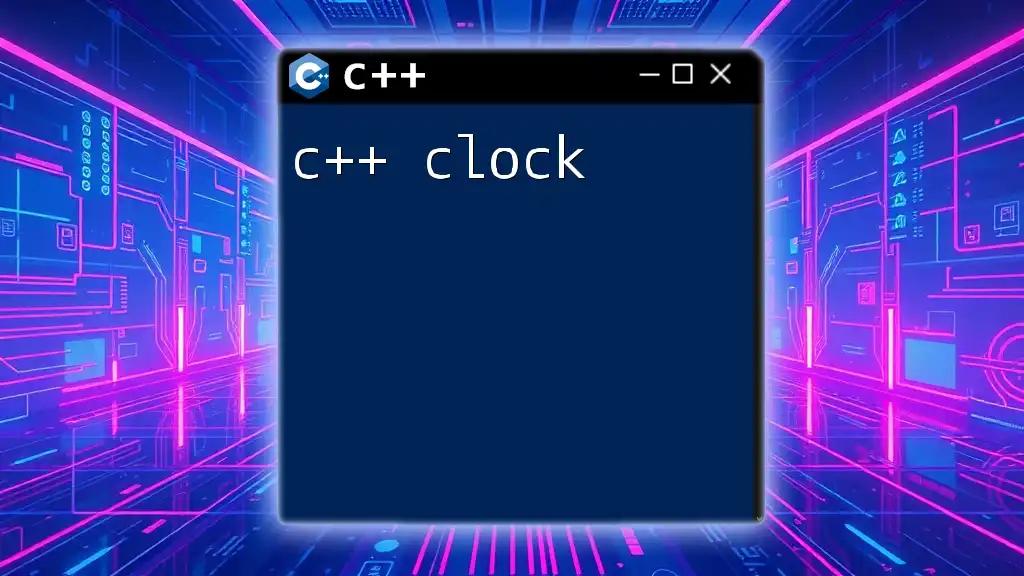
Including the Necessary Headers
Required Headers for Using clock_t
To use `clock_t`, you need to include the `<ctime>` header in your program. This header provides all the necessary declarations for time-related functions and types.
Here’s how to set it up:
#include <iostream>
#include <ctime>

Working with clock_t
Capturing the Current Time
To start measuring time, you can capture the current CPU time using the `clock()` function. This function returns the processor time consumed by the program since its start in `clock_t` ticks.
clock_t start = clock();
Calculating Execution Time
Once you have captured the start time, you can execute the code segment you want to measure. After the execution, you can capture the end time and calculate the difference to determine the execution duration.
Here’s a code snippet demonstrating how to measure the performance of a simple function:
void exampleFunction() {
// Simulate a task
for (int i = 0; i < 1000000; ++i);
}
int main() {
clock_t start = clock();
exampleFunction();
clock_t end = clock();
double duration = double(end - start) / CLOCKS_PER_SEC;
std::cout << "Execution Time: " << duration << " seconds" << std::endl;
return 0;
}
In this example, `exampleFunction` simulates a workload by running a loop. The time taken for this function to complete is measured in seconds, allowing you to understand the performance impact of that loop.
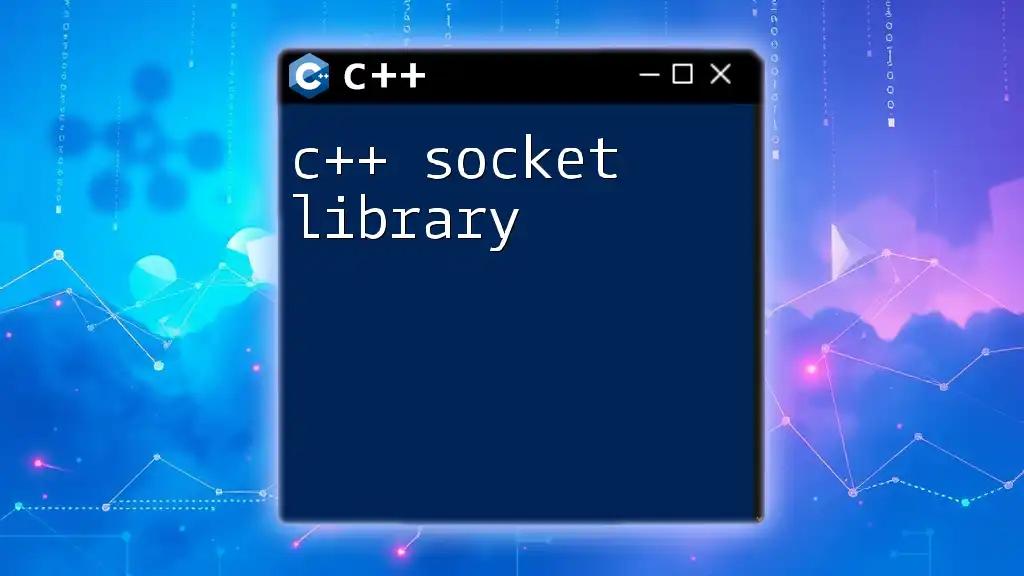
Understanding the CLOCKS_PER_SEC Macro
What is CLOCKS_PER_SEC?
The `CLOCKS_PER_SEC` macro provides the number of clock ticks per second. It is essential for converting the raw ticks returned by `clock()` into seconds, giving you a clear understanding of how long your code took to execute.
To convert the difference in clock ticks to seconds, you divide the difference by `CLOCKS_PER_SEC`:
double duration = double(end - start) / CLOCKS_PER_SEC;

Limitations of clock_t
Precision and Accuracy Limitations
While `clock_t` can be useful, it does come with limitations. The resolution of `clock()` can vary across platforms, potentially affecting your timing results. On some systems, it may only provide millisecond precision, which might not be sufficient for finer analysis.
Potential Solutions
For more accurate and detailed performance measurements, consider using the modern `std::chrono` library, which provides a high-resolution clock. Here’s a comparison that emphasizes the benefits of using `std::chrono`:
#include <chrono>
auto start = std::chrono::high_resolution_clock::now();
// Code to measure
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution Time: " << duration.count() << " seconds" << std::endl;
The `std::chrono` library offers consistent and high-resolution timing suitable for thorough performance evaluations.

Practical Applications of clock_t
Real-World Scenarios
The applications of `clock_t` extend beyond simple examples:
-
Performance Benchmarking in Algorithms: You can use `clock_t` to compare different sorting algorithms, searching algorithms, or any computational task where efficiency is critical.
-
Comparing Different Algorithms' Efficiencies: By measuring the execution time of various implementations, you can determine which algorithm performs better under specified conditions.
-
Analyzing Time Complexity: By using `clock_t` to evaluate how execution time grows with input size, you can draw conclusions about the complexity of your algorithms.

Common Mistakes to Avoid
Incorrect Time Calculation
One common mistake is miscalculating the time due to placing the clock capture statements incorrectly. Ensure that you only measure the execution time of the code you intend to measure.
Neglecting to Reset Start Time
If you intend to measure multiple segments of your code, always reset your start time before each measurement to ensure you capture the right duration. This prevents accumulation of time from previous measurements.
Assuming Consistency Across Platforms
Timing results can vary across different systems depending on hardware, OS scheduling, and other factors. Always test performance on the target environment to gather accurate data.
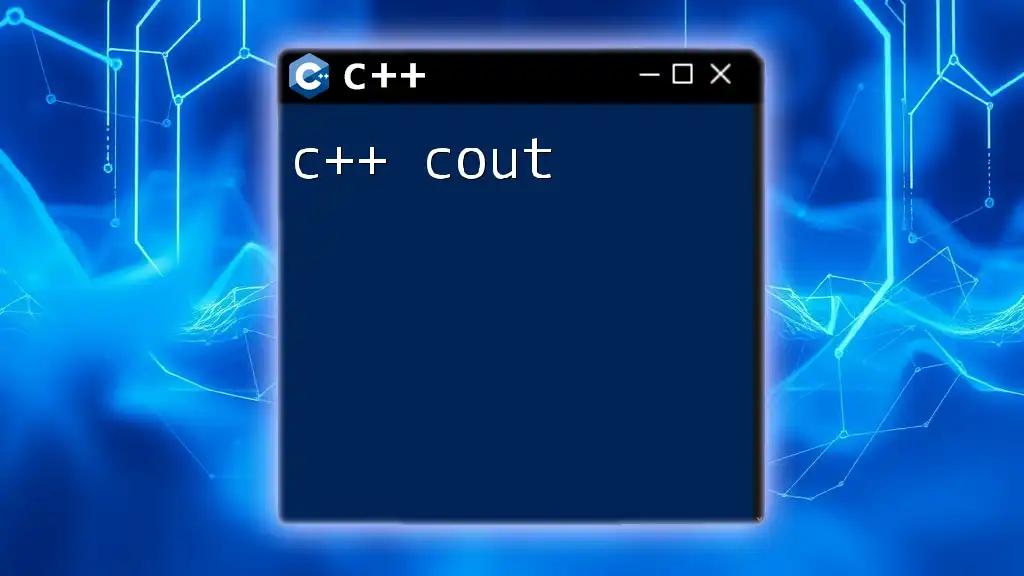
Conclusion
In summary, `c++ clock_t` is an invaluable tool for measuring CPU processing time, allowing developers to gauge code performance efficiently. Understanding how to utilize this feature effectively can lead to more refined and optimized applications. As coding practices evolve, exploring more advanced timing techniques, such as `std::chrono`, will enhance your skills further. As you dive into performance measurements, remember to practice and test your approaches to gain practical experience and insight into your coding optimizations.