The `cpp check` command is used to process C preprocessor directives and can be utilized to verify the correctness of C/C++ code by expanding macros and including header files.
Here's a simple example of how to use it in a terminal:
cpp -E example.cpp
What is `cppcheck`?
`cppcheck` is a static analysis tool specifically designed for C and C++ code. Its main purpose is to identify bugs, undefined behavior, and code style issues before the code is compiled and executed. By integrating `cppcheck` into the development process, programmers can catch potential errors early, leading to higher-quality, more maintainable code.
Advantages of using `cppcheck`
-
Detecting Bugs and Undefined Behavior: One of the primary functions of `cppcheck` is to reveal problematic code patterns that may lead to runtime errors. This includes issues like dereferencing null pointers, memory leaks, and data race conditions.
-
Code Style Analysis: Aside from identifying functional problems, `cppcheck` also evaluates the coding style. This can help maintain consistency across a codebase, making it easier for teams to collaborate and understand each other's code.
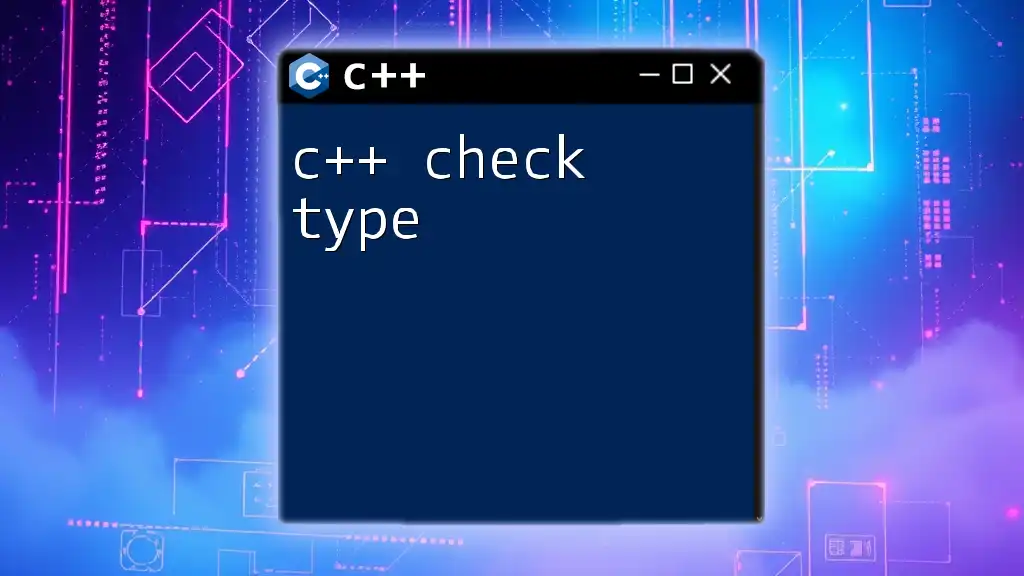
Installing `cppcheck`
Before diving into the usage of `cppcheck`, it’s essential to get it set up on your machine. Below is a guide for installation on various operating systems.
Prerequisites
Ensure you have a C/C++ development environment set up, including a C/C++ compiler. This is necessary for effective analysis of your code.
Installation on Different Operating Systems
-
Windows:
To install `cppcheck` on Windows, download the installer from GitHub or the official website. Run the installer and follow the instructions to get it up and running.
-
Linux:
Most Linux distributions include `cppcheck` in their package managers. You can simply use the following commands:
- For Debian-based systems (like Ubuntu):
sudo apt-get install cppcheck
- For Red Hat-based systems:
sudo yum install cppcheck
-
macOS:
If you're using macOS, you can install `cppcheck` via Homebrew. If you don’t have Homebrew installed, you can find it at https://brew.sh. Once Homebrew is ready, execute:
brew install cppcheck
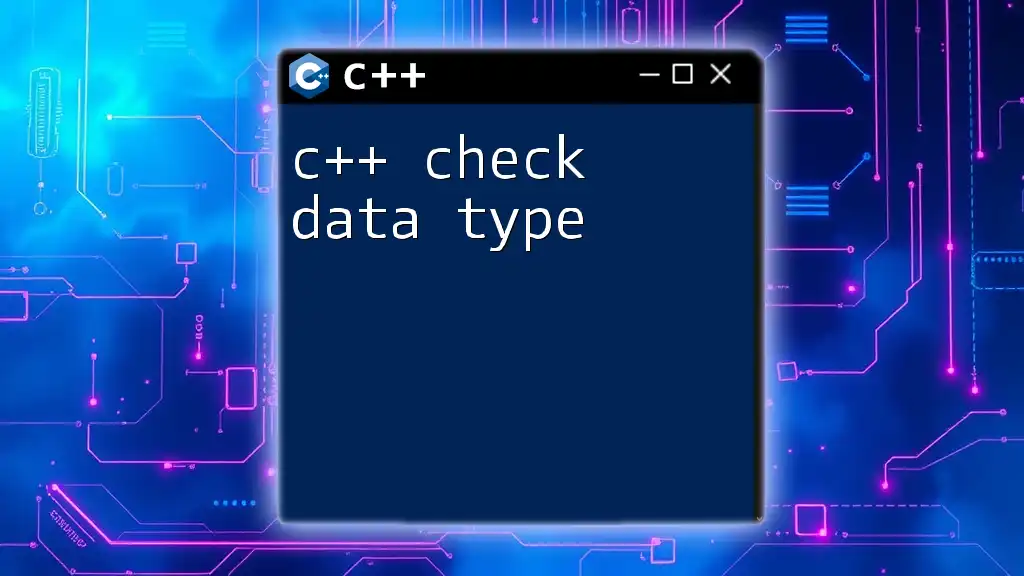
Using `cppcheck`
Now that you have `cppcheck` installed, it’s time to utilize it. The primary use case for `cppcheck` is analyzing C/C++ source files for potential issues.
Basic Command-Line Usage
The command structure for running `cppcheck` is straightforward. Simply navigate to the terminal and run the following command on the desired source code path:
cppcheck path/to/source
For example, if your source code is in a folder named `my_project`, you would execute:
cppcheck my_project/
Command Options
`cppcheck` offers various command-line options to tailor its behavior according to your needs. Here are some common options:
-
`--enable=all`: Enables all checks. This is useful for those wanting comprehensive analysis.
-
`--suppress`: Use this option to ignore specific warnings that you may have deemed irrelevant.
Example of combining options:
cppcheck --enable=all --suppress=missingInclude path/to/source
Output Formats
Understanding the output from `cppcheck` is crucial for effectively diagnosing code issues. By default, `cppcheck` displays results directly in the terminal. A typical warning might look like this:
[path/to/file.cpp:42]: (error) Null pointer dereference
This indicates the specific file and line where the error occurs.
To obtain structured output, you can use the `--xml` option, which generates XML formatted results:
cppcheck --xml path/to/source
The XML output can be integrated into other tools or used for further analysis, making it a versatile option.
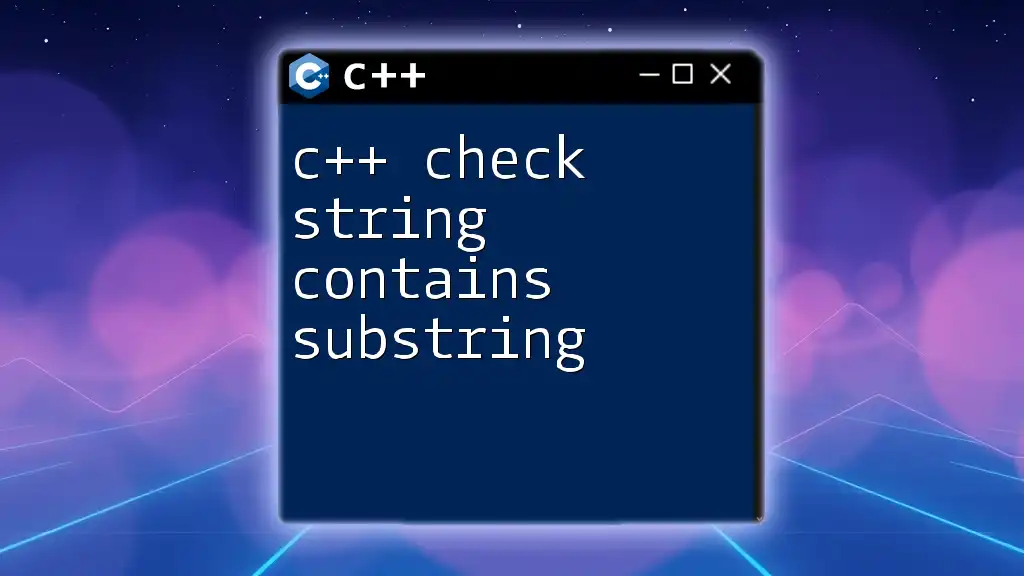
Analyzing Results
Reading and interpreting output from `cppcheck` is essential for effective debugging and code improvement.
Understanding `cppcheck` Output
The output consists of several severity levels, including error, warning, and style. Each term denotes the nature of the issue found.
For example, a typical output appears like this:
[path/to/file.cpp:25]: (warning) Possible memory leak: 'ptr'
This states that there is a potential memory leak on line 25 of `file.cpp`, specifically involving the variable `ptr`.
Debugging with `cppcheck`
Resolving issues reported by `cppcheck` involves several steps. It is advisable to prioritize warnings based on their severity. Start with critical issues such as memory leaks and null pointer dereferences, as these can lead to application crashes or undefined behavior.
For instance, consider the following problematic code that raises a null pointer dereference warning:
int* ptr = nullptr; // This will raise a warning
// Code continues...
To fix this, you can incorporate a check:
if (ptr != nullptr) {
// Safe to dereference ptr
}
By addressing these warnings, you can enhance the stability and reliability of your code.
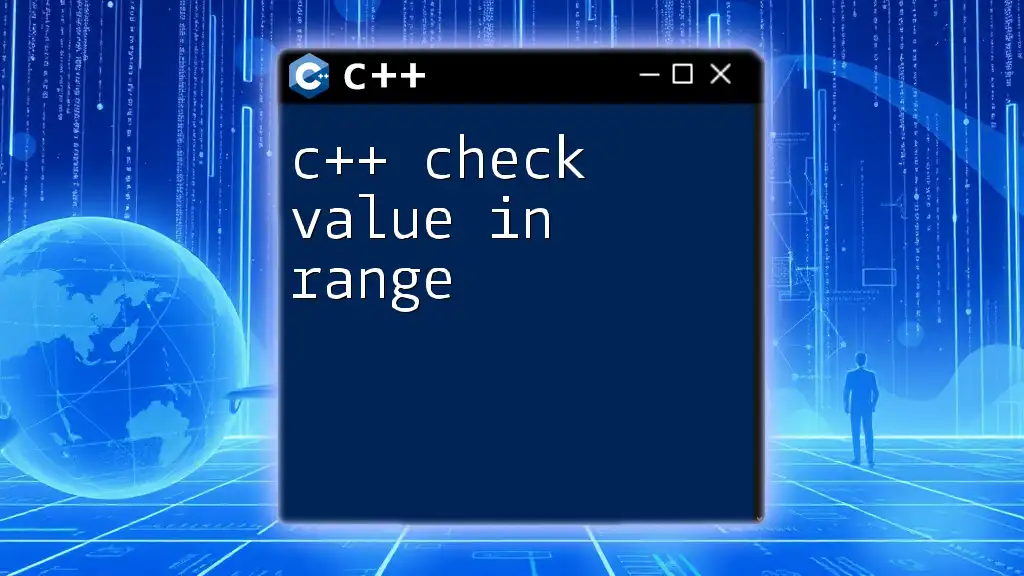
Features of `cppcheck`
Types of Checks
`cppcheck` performs several checks covering a broad range of scenarios. Notably, it investigates for:
- Common Checks: Including memory leaks, undefined behaviors, and error-prone API usage.
- Style Checks: Such as trailing whitespace and naming conventions.
Configuring `cppcheck`
For tailored analysis, you can use a `.cppcheck` configuration file. This allows you to define custom suppression rules or adjust which checks to enable.
Example of a simple `.cppcheck` configuration:
suppress: missingInclude
This line tells `cppcheck` to ignore warnings about missing includes when analyzing your code.
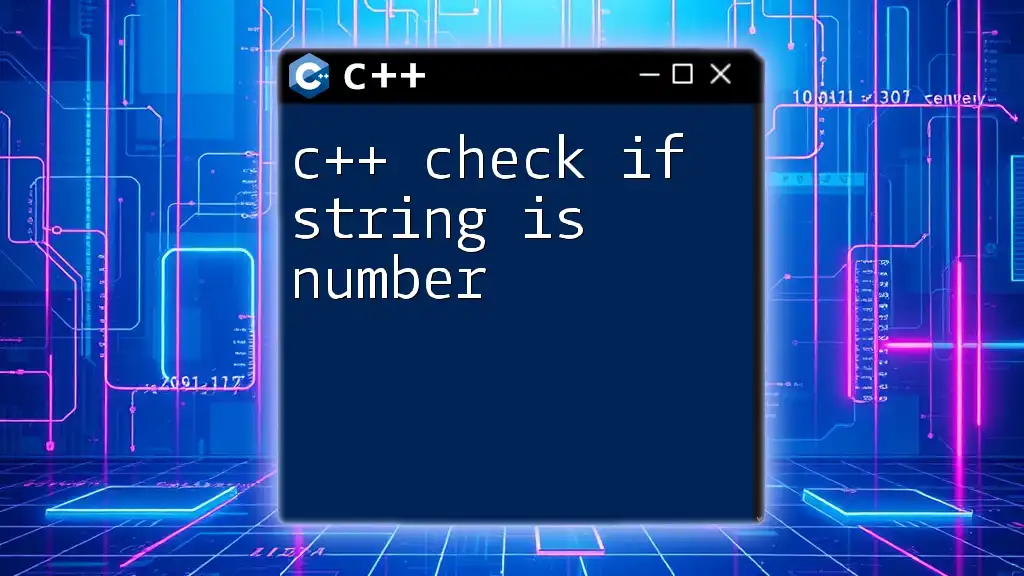
Advanced Usage
Integrating with CI/CD Pipelines
To ensure your code remains error-free during the development cycle, integrating `cppcheck` into your Continuous Integration/Continuous Deployment (CI/CD) pipeline is highly beneficial. This automates the checking process, ensuring every change is analyzed before merging.
Here’s a simple GitHub Actions job definition that runs `cppcheck` on your code:
jobs:
cppcheck:
runs-on: ubuntu-latest
steps:
- name: Checkout
uses: actions/checkout@v2
- name: Run cppcheck
run: cppcheck --enable=all --xml path/to/source
This ensures that every push or pull request activates `cppcheck`, allowing you to catch issues immediately.
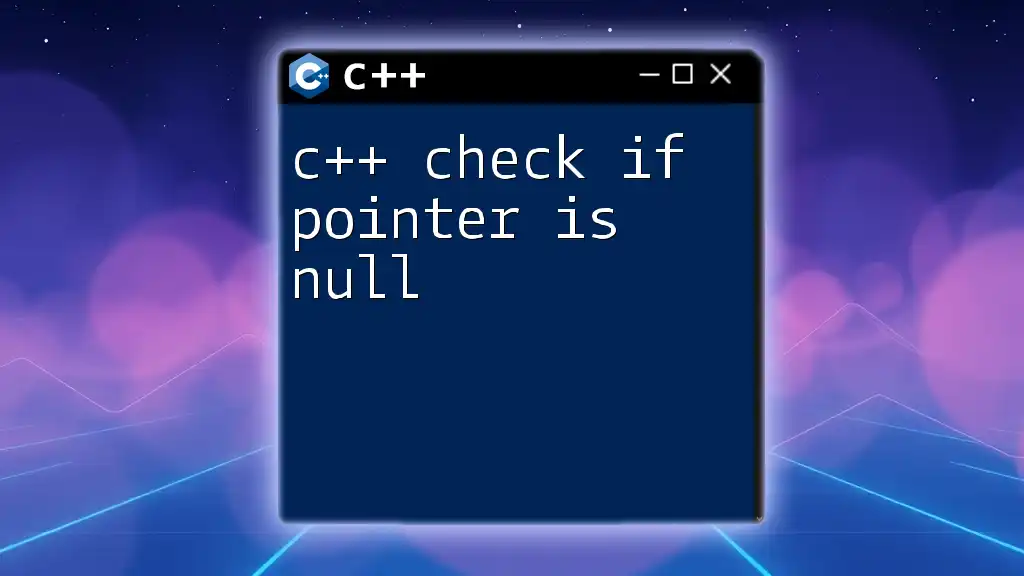
Common Pitfalls and Misconceptions
While `cppcheck` is a powerful tool, understanding its limitations is essential. Many developers mistakenly believe that `cppcheck` can catch all issues that might arise in their code. However, it’s crucial to remember:
-
Scope of `cppcheck`: It may not find logical errors in your code, especially those that occur only at runtime.
-
Limitations Compared to Other Tools: Unlike dynamic analysis tools such as valgrind or AddressSanitizer, `cppcheck` does not execute your code. It’s primarily a static analysis tool.
When to Use `cppcheck` in Your Workflow
Integrating `cppcheck` into your workflow is advantageous when you are actively developing code. Regular checks can help maintain code quality, prevent issues from escalating, and facilitate better practices among team members.
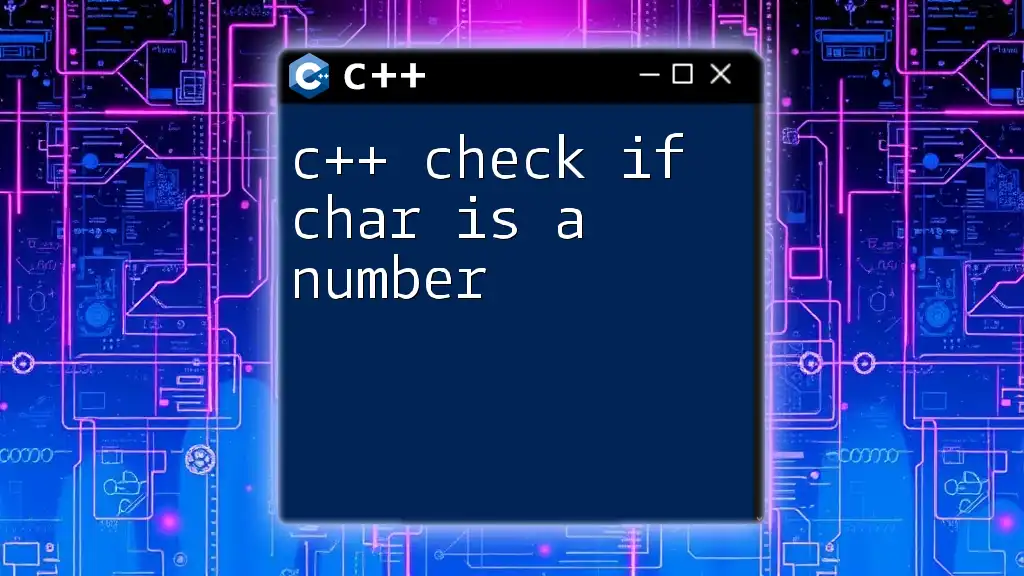
Conclusion
In summary, `cppcheck` is a robust tool for maintaining code quality in C and C++ projects. Its ability to identify bugs, undefined behavior, and style issues makes it invaluable for developers. By incorporating `cppcheck` into your programming practices, you not only enhance code robustness but also promote a culture of diligence and quality throughout your development team.
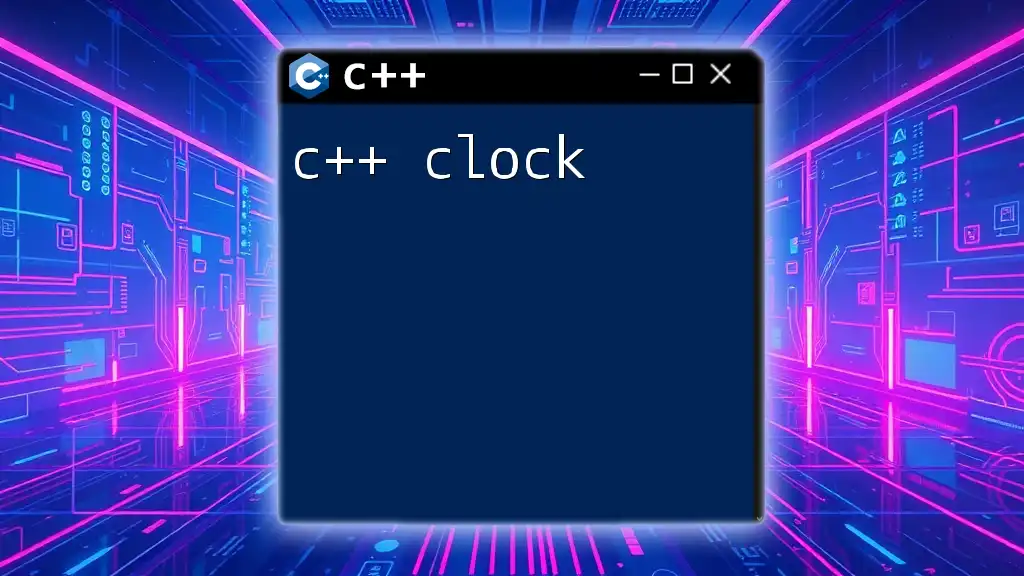
Additional Resources
For more comprehensive information, consider exploring the official `cppcheck` documentation and participating in community forums for support and best practices. This will further enhance your understanding and use of static code analysis in your projects.