In C++, you can check if a pointer is null by comparing it to `nullptr`, which helps prevent dereferencing a null pointer that could lead to undefined behavior. Here's a simple code snippet illustrating this:
#include <iostream>
int main() {
int* ptr = nullptr; // Initialize pointer to null
if (ptr == nullptr) {
std::cout << "Pointer is null." << std::endl;
} else {
std::cout << "Pointer is not null." << std::endl;
}
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer is a variable that stores the memory address of another variable. In C++, understanding pointers is crucial because they allow direct memory manipulation, which can lead to efficient programs. However, with great power comes responsibility, especially when it comes to handling null pointers. A pointer that has not been assigned a valid memory address is called a null pointer.
Types of Pointers
In C++, there are several types of pointers:
-
Raw Pointers: These are basic pointers that store addresses directly. For instance, an `int*` points to an integer variable.
-
Smart Pointers: C++11 introduced smart pointers like `unique_ptr`, `shared_ptr`, and `weak_ptr` that help manage memory automatically. They prevent memory leaks and dangling pointers.
Understanding these types is essential as they determine how you manage your memory and check for null values.
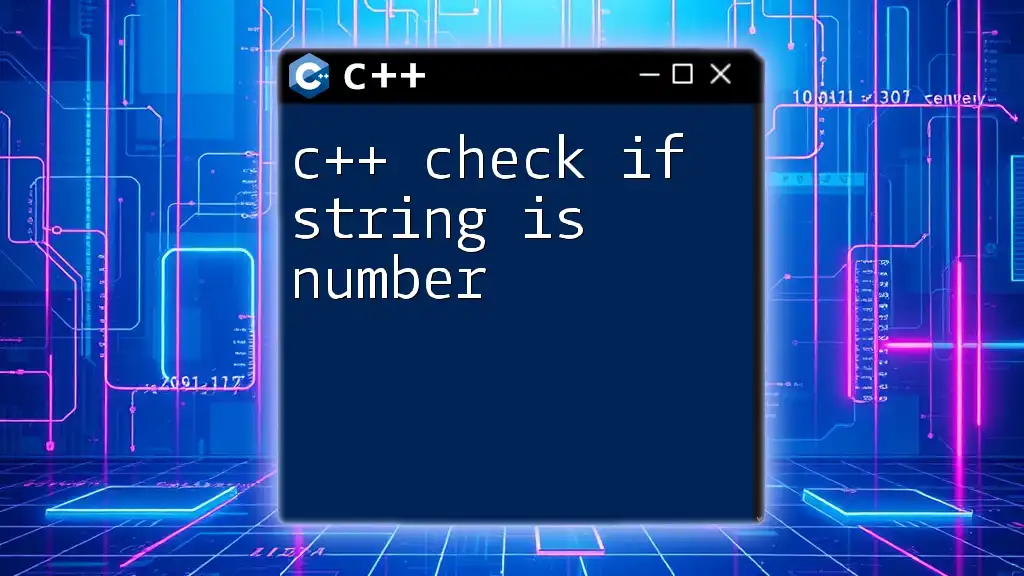
Null Pointers
What is a Null Pointer?
A null pointer is a pointer that does not point to any valid memory location. When a pointer is set to `nullptr`, it signifies that the pointer is not currently assigned to any object. Null pointers serve a critical role in C++ programming, allowing the programmer to check if a pointer is valid before using it.
It is essential to distinguish between null pointers and uninitialized pointers; the latter can point to any random memory address, leading to undefined behavior.
Common Scenarios for Null Pointers
Null pointers frequently crop up in various programming scenarios such as:
-
Dynamic Memory Allocation: When using libraries like `new` or `malloc`, failure to allocate memory may result in a null pointer.
-
Error Handling: Function return types may indicate failure by returning a null pointer, necessitating checks before accessing the pointer.
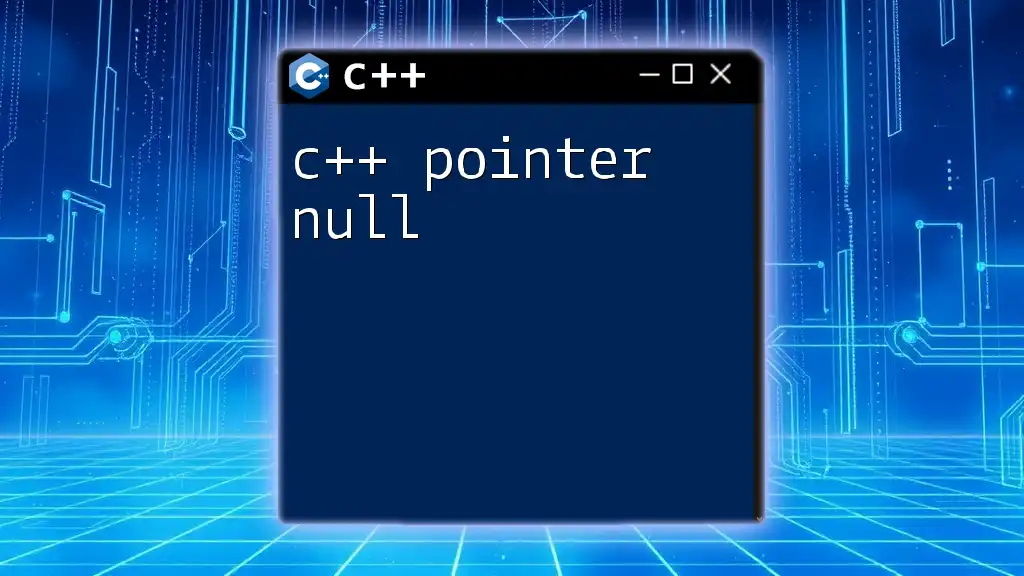
Checking for Null Pointers
How to Check if a Pointer is Null in C++
When you need to check if a pointer is null in C++, you can adopt several methods. Each of the following ways has its use cases and advantages.
Using `nullptr`
Starting from C++11, the standard introduced `nullptr`, a type-safe null pointer constant. It is preferred because it eliminates ambiguity.
int* ptr = nullptr;
if (ptr == nullptr) {
// Pointer is null
}
Using `NULL`
Before C++11, programmers commonly used `NULL`, which is defined as `0` in C. However, using `NULL` can lead to confusion in certain contexts.
int* ptr = NULL;
if (ptr == NULL) {
// Pointer is null
}
Using `0`
Using `0` explicitly to denote a null pointer is an older method and is generally less preferred nowadays due to type safety issues.
int* ptr = 0;
if (ptr == 0) {
// Pointer is null
}
Best Practices for Pointer Checks
To ensure robust coding practices, adhere to the following:
-
Always Check for Null: Before dereferencing a pointer, ensure it is not null.
-
Combine Conditions Wisely: You may often check other conditions alongside a null check. This practice enhances program security.
-
Embrace Modern C++ Features: Using smart pointers and automatic memory management can help minimize the risks associated with null pointers.
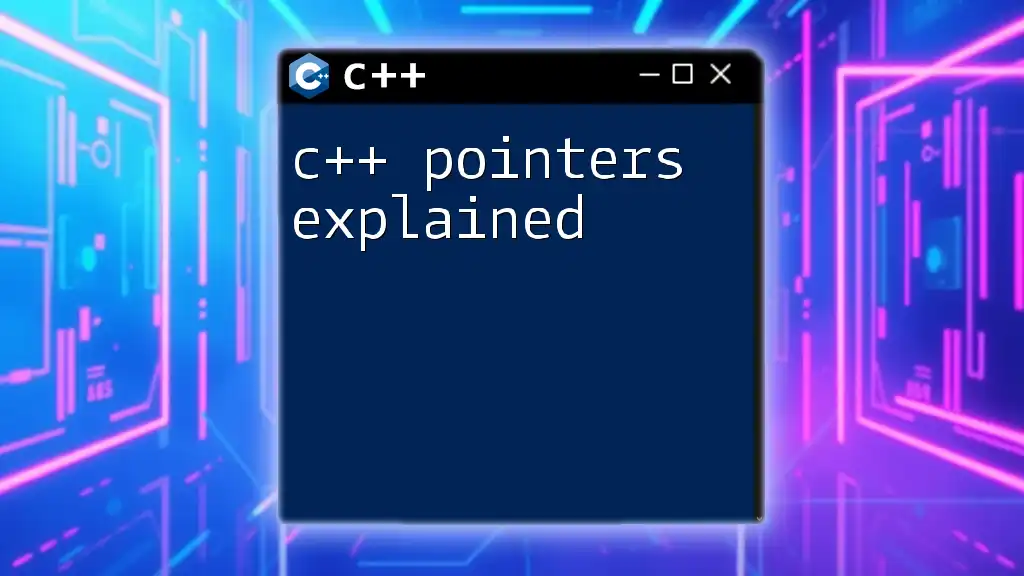
Consequences of Dereferencing Null Pointers
What Happens If You Don't Check?
Failing to check whether a pointer is null can lead to undefined behavior, which may cause unexpected crashes or security vulnerabilities in your program.
Consider the following example, where a null pointer is dereferenced:
int* ptr = nullptr;
std::cout << *ptr; // This will lead to a crash
Debugging Null Pointer Dereferencing
When debugging null pointer issues, consider using tools like Valgrind or GDB. These tools can help you identify problematic areas in your code by tracing back where the pointer became invalid, helping you correct the logic.
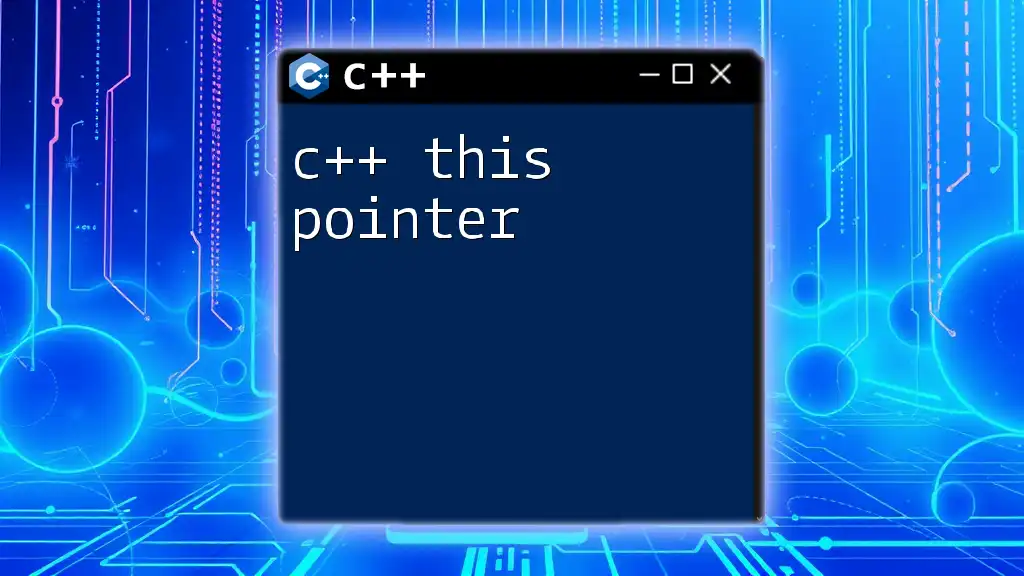
Summary
In this article, we've navigated the concept of pointers in C++, particularly focusing on the crucial task of checking for null pointers. Key points to remember include the importance of using `nullptr`, the differences between `NULL` and `0`, and best practices that can safeguard your applications against null dereferencing issues.
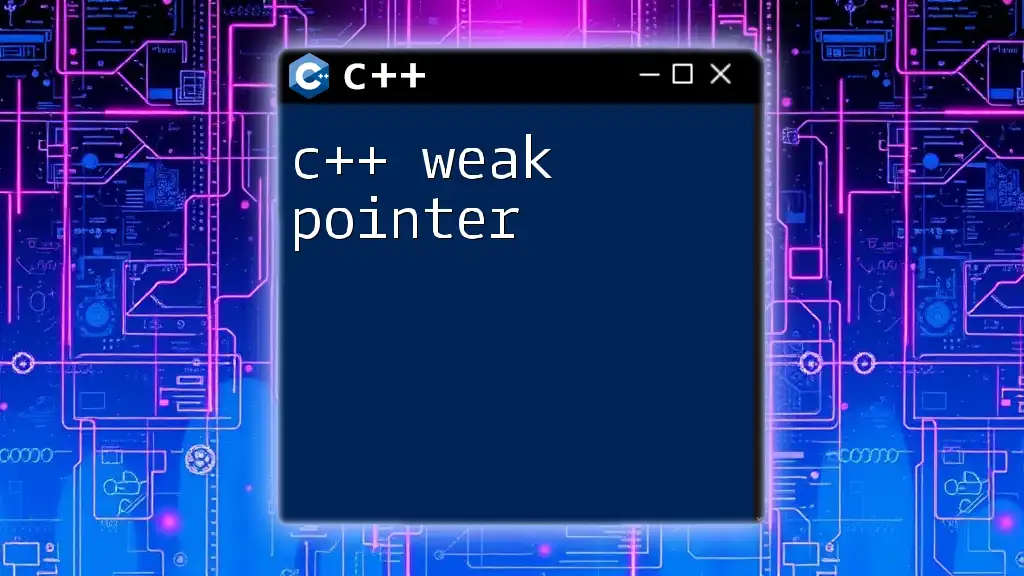
Further Learning Resources
For those interested in deepening their knowledge of C++, consider exploring the following resources:
-
Books like "C++ Primer" by Lippman, Lajoie, and Moo for foundational knowledge.
-
Online courses on platforms like Coursera or Udemy for practical, hands-on experience.
-
The official C++ documentation to clarify language features and functions.
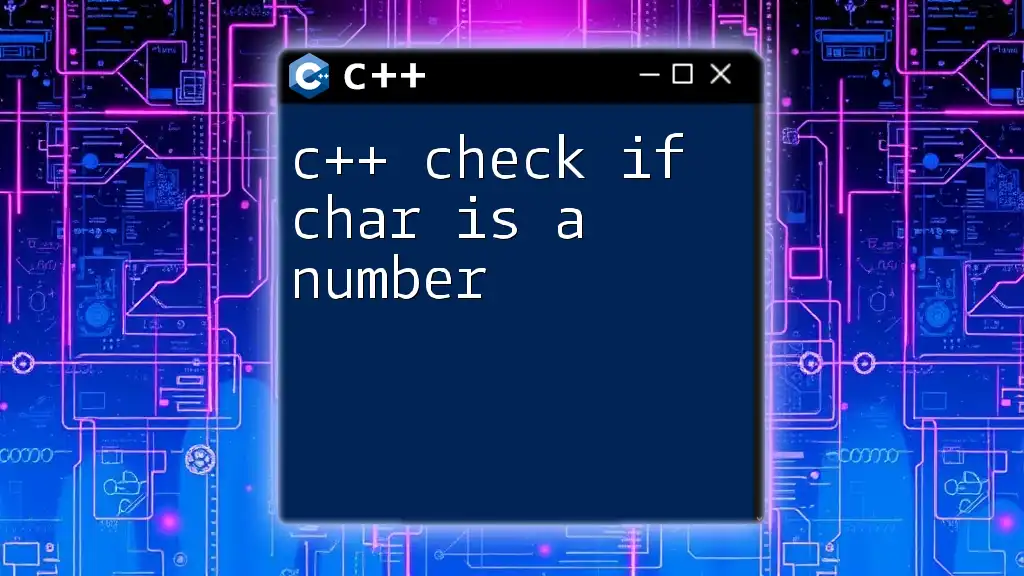
Conclusion
Remember, effectively managing pointers and checking for null values are essential skills for any C++ programmer. I encourage you to practice these techniques in your projects and share your experiences or questions regarding null pointers in C++.