To check if a character is a number in C++, you can use the `isdigit` function from the `<cctype>` library. Here's how you can do it:
#include <cctype>
#include <iostream>
int main() {
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a number." << std::endl;
} else {
std::cout << ch << " is not a number." << std::endl;
}
return 0;
}
Understanding Character Types in C++
What is a Char in C++?
In C++, the `char` data type is used to store single characters. It occupies one byte of memory and can hold any of the values defined by the ASCII or Unicode standards. Understanding how characters are represented in C++ is crucial for performing operations like checking if a character is a number.
Character Encoding
Characters are represented using encoding systems such as ASCII (American Standard Code for Information Interchange) or Unicode. In the ASCII encoding scheme, characters have specific numerical values. For example, the numeral '0' is represented by the value 48, '1' is represented by 49, and so on, up to '9' which is represented by 57 in ASCII. Recognizing these representations helps in effectively checking if a character is a number in C++.
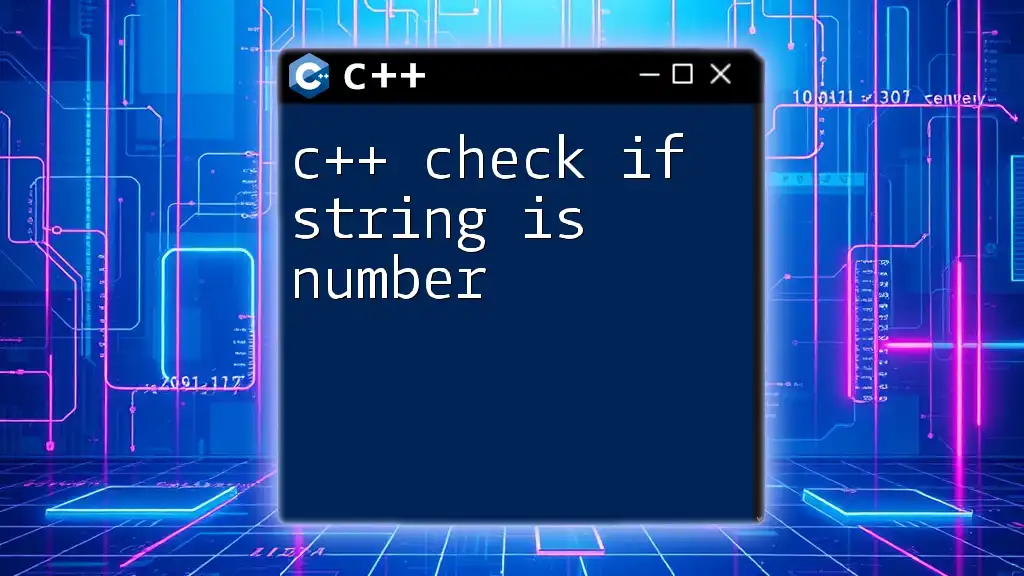
Methods to Check if a Character is a Number
Using `isdigit() Function
One of the simplest ways to check if a character is a number in C++ is by using the `isdigit()` function, which is included in the `<cctype>` library. This function checks if a given character is a decimal digit.
Example:
#include <iostream>
#include <cctype>
int main() {
char ch = '5';
if (isdigit(ch)) {
std::cout << ch << " is a number." << std::endl;
} else {
std::cout << ch << " is not a number." << std::endl;
}
return 0;
}
In this example, the code checks if the character stored in the variable `ch` is a digit. If it is, it prints that the character is a number. The function `isdigit()` is straightforward and easy to use, making it an excellent choice for simple character validation tasks.
Using ASCII Value Comparison
Another method to check if a character is numeric involves comparing its ASCII value. This method simply checks whether the character falls within the range of '0' to '9'.
Example:
#include <iostream>
int main() {
char ch = '3';
if (ch >= '0' && ch <= '9') {
std::cout << ch << " is a number." << std::endl;
} else {
std::cout << ch << " is not a number." << std::endl;
}
return 0;
}
In the code snippet above, the logic explicitly tests whether the character `ch` lies between the ASCII values for '0' and '9'. This method is efficient and avoids function call overhead.
Using Regular Expressions (C++11 and later)
For more complex scenarios, such as when checking against various patterns, C++ offers a powerful feature: regular expressions. Introduced in C++11, the `<regex>` library allows developers to perform pattern matching.
Example:
#include <iostream>
#include <regex>
int main() {
char ch = '7';
std::regex number_regex("[0-9]");
if (std::regex_match(std::string(1, ch), number_regex)) {
std::cout << ch << " is a number." << std::endl;
} else {
std::cout << ch << " is not a number." << std::endl;
}
return 0;
}
In this example, `std::regex_match` is used to compare the single-character string against the regex pattern `[0-9]`, which represents any digit. While this method is flexible, it may not be the most efficient for simple checks compared to `isdigit()` or ASCII comparisons.
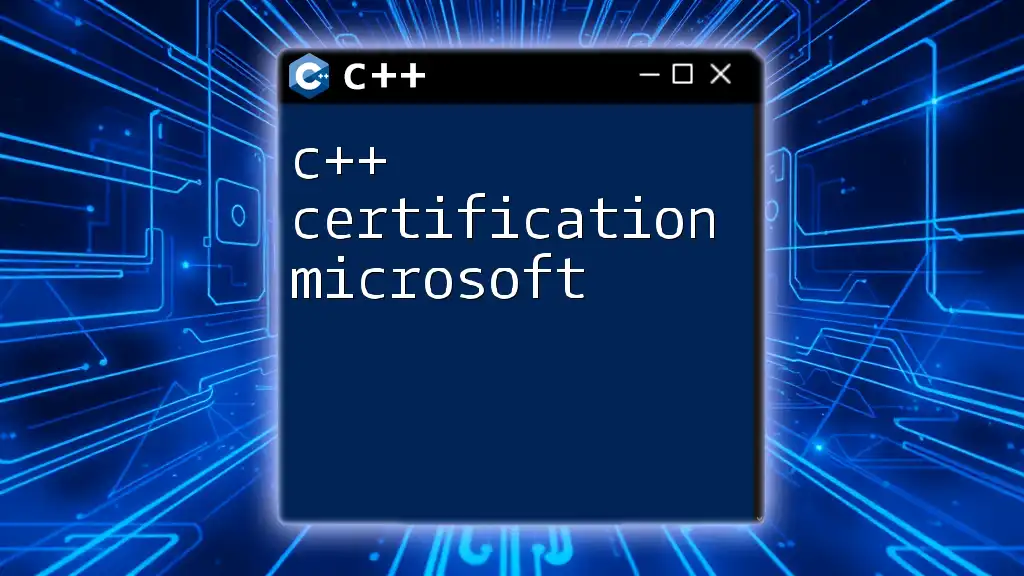
Common Pitfalls in Character Validation
Misunderstanding Character Types
One common mistake lies in confusing character types. For instance, you may find yourself trying to check an `int` type directly, which can lead to unexpected results. Always ensure that you are working with actual `char` variables when making comparisons.
Regions of Undefined Behavior
Be cautious of scenarios that could lead to undefined behavior, such as passing non-character data types to functions expecting `char`. Always use proper validation techniques and type checking before performing operations on characters.
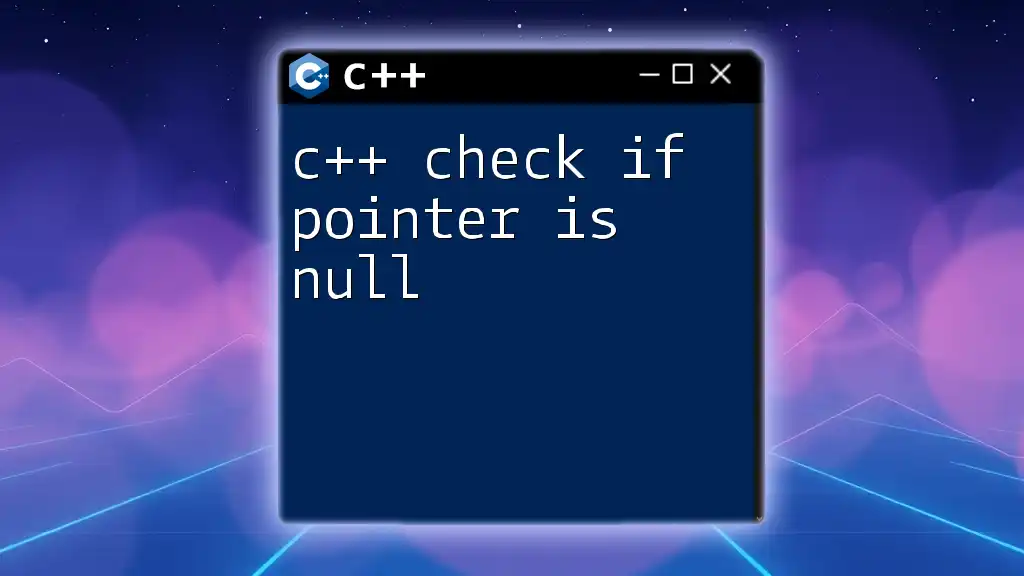
Conclusion
Validating characters in C++ is a foundational skill that enhances your programming ability and fortifies your applications against errors. From using the built-in `isdigit()` function to leveraging ASCII value comparisons and regular expressions, several techniques allow you to efficiently and effectively check if a character is a number. Experiment with these methods in your projects and reinforce your understanding of character validation in C++.