In C++, you can check the data type of a variable using the `typeid` operator from the `<typeinfo>` header, which provides information about the type at runtime.
#include <iostream>
#include <typeinfo>
int main() {
int a = 10;
std::cout << "The data type of a is: " << typeid(a).name() << std::endl;
return 0;
}
Understanding Data Types in C++
In C++, data types are categorized into several groups, each serving a specific purpose in programming. Understanding these types is essential because it influences how operations are performed and how memory is allocated.
Primitive Data Types
- Integers: Whole numbers, which can be signed or unsigned. Examples include `int`, `short`, `long`, and `long long`.
- Floats: Data types used to represent numbers with decimal points. Examples include `float` and `double`.
- Characters: Represents a single character, defined by the `char` type.
- Booleans: A data type that can hold only two values, typically true or false.
Derived Data Types
- Arrays: A collection of elements of the same type. For example, `int arr[10];` creates an array of 10 integers.
- Pointers: Variables that store the memory address of another variable. For example, `int* ptr;` defines a pointer to an integer.
- Functions: In C++, functions can also be treated as data types, allowing for function pointers.
User-defined Data Types
- Structures: A way to group different data types under one name. For example:
struct Person { std::string name; int age; };
- Unions: Similar to structures but can only hold one of its non-static data members at a time.
- Enums: User-defined types consisting of a set of named integral constants, e.g., `enum Color { Red, Green, Blue };`.
- Classes: Fundamental building blocks of Object-Oriented Programming in C++, allowing encapsulation of data and functions.
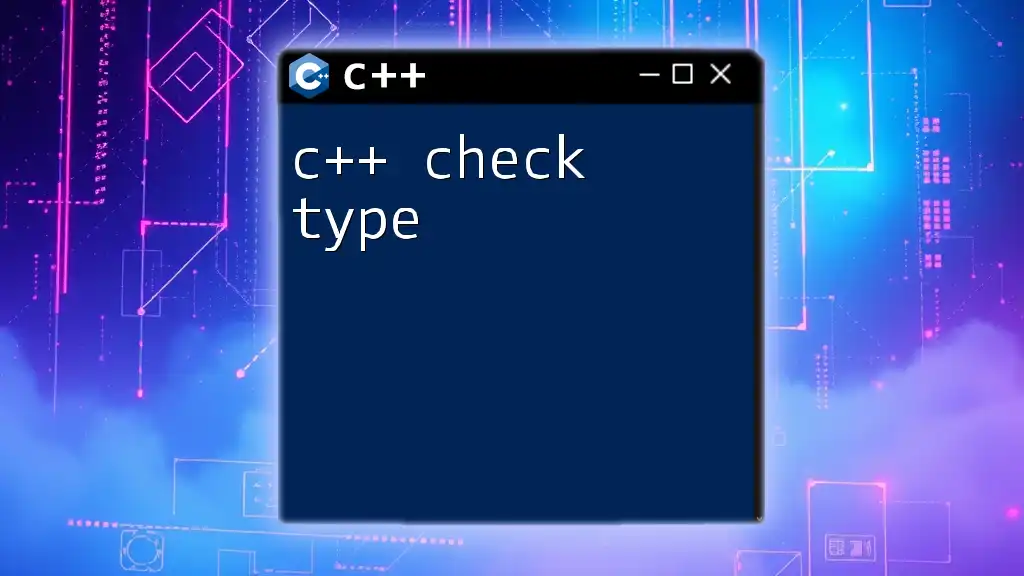
Why Check Data Types?
Checking data types is crucial in programming for several reasons:
- Prevention of Type-Related Errors: Accidentally mixing types can lead to runtime errors. Type checking can catch these issues before they happen.
- Proper Memory Management: Different data types consume different amounts of memory. Understanding data types helps in optimizing resource allocation.
- Code Readability and Maintenance: Explicitly checking for types makes code easier to read, understand, and maintain, as it clarifies the programmer's intentions.
Real-world scenarios illustrate the necessity of checking data types. For example, if a function expects an integer argument but a float is passed, it can lead to unexpected behaviors or crashes.
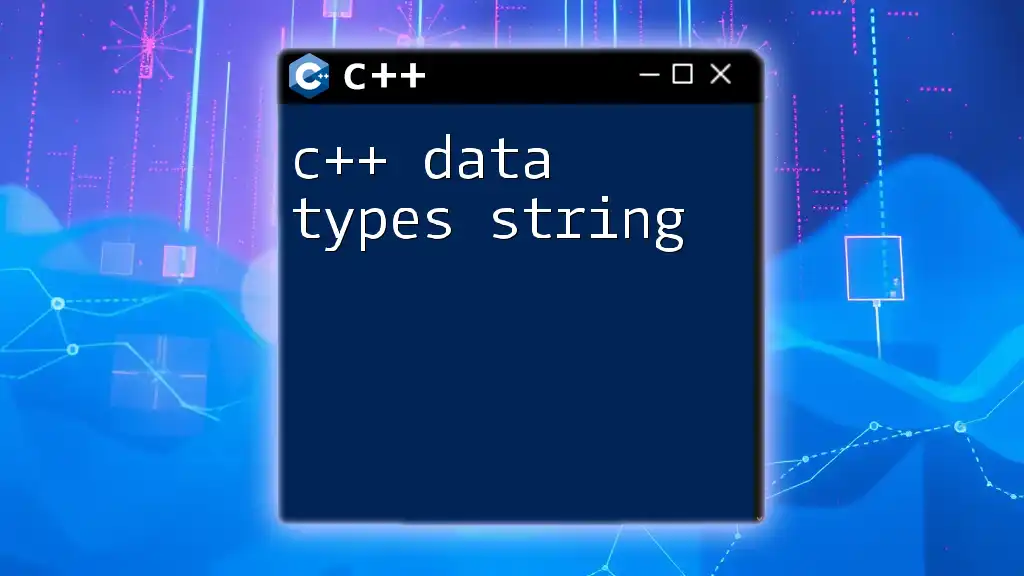
Methods to Check Data Types in C++
Typeid Operator
The `typeid` operator provides a way to determine the type of an expression at runtime. Here’s how it works:
#include <iostream>
#include <typeinfo>
int main() {
int a = 5;
std::cout << "The type of a is: " << typeid(a).name() << std::endl;
return 0;
}
In this example, using `typeid(a).name()` retrieves the type of variable `a`, which will output something like `i` for `int`, depending on the compiler. This method is straightforward and useful for debugging purposes.
Using std::variant for Type Safety
`std::variant` is a powerful feature introduced in C++17 that allows you to create a type-safe union. With `std::variant`, you can check which type is currently held by the variant.
#include <iostream>
#include <variant>
int main() {
std::variant<int, float, std::string> myVar = 10;
if (std::holds_alternative<int>(myVar)) {
std::cout << "myVar holds an int." << std::endl;
}
return 0;
}
In this code, `std::holds_alternative<int>(myVar)` checks if `myVar` currently holds an `int`. This adds a layer of type safety over traditional unions, preventing runtime errors.
Custom Function for Type Checking
Creating a custom function allows for a reusable approach to checking types throughout your codebase. Here's an example:
#include <iostream>
#include <typeinfo>
template<typename T>
void checkType(T variable) {
std::cout << "The type of the variable is: " << typeid(variable).name() << std::endl;
}
int main() {
checkType(123);
checkType(45.67);
return 0;
}
This `checkType` function uses templates, enabling it to accept any data type while providing a clear output of the variable's type, showcasing the power of C++ templates in type-checking scenarios.
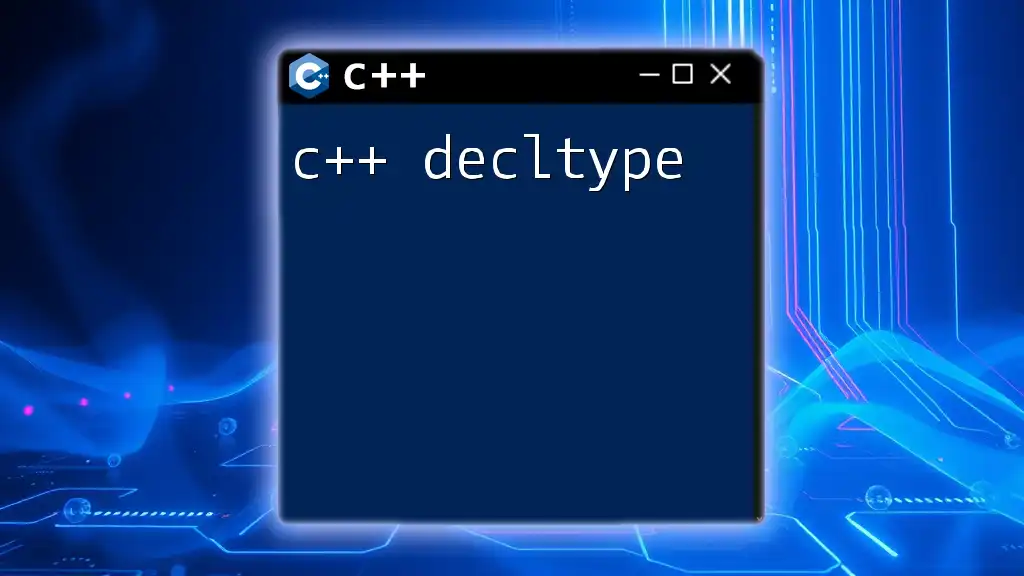
Additional Tools for Type Checking
Using Libraries
There are several libraries available that facilitate better type checking in C++. Two popular libraries are:
- Boost Type Traits: This library provides a set of template classes that help query and manipulate types at compile time, allowing you to enforce type constraints directly in your code.
- GSL (Guideline Support Library): Maintained by the C++ Core Guidelines team, GSL helps improve code safety and provides various utilities for type management and safety checks.
These libraries enhance the way developers can handle type information, making their code more robust and maintainable.
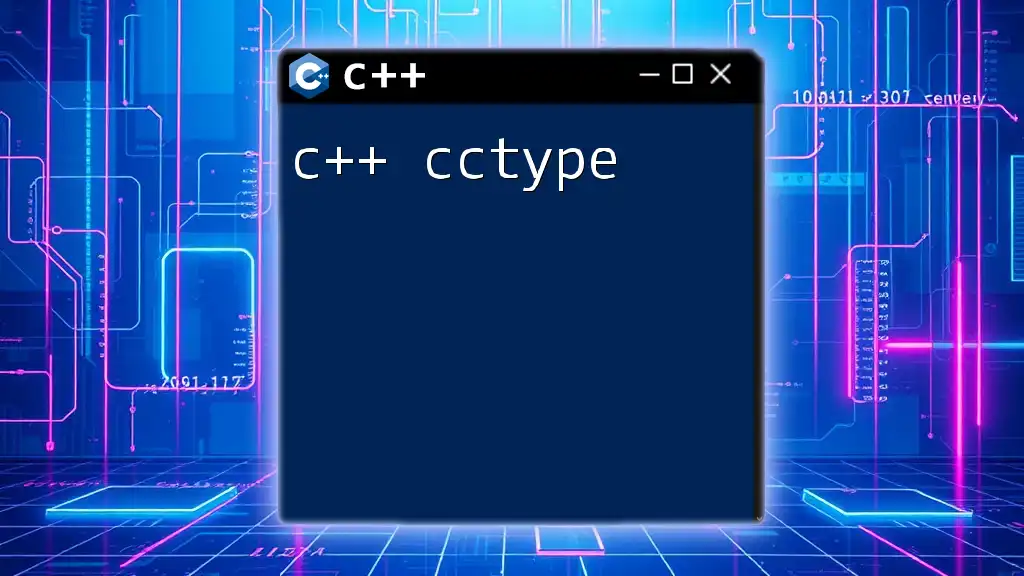
Best Practices
When checking data types in C++, here are some best practices to follow for effective programming:
- Always Use Explicit Type Definitions: This prevents confusion about what kind of data is being processed and ensures that correct types are passed between functions.
- Avoid Relying Heavily on Type Checking in Performance-Critical Code: Type checks can introduce overhead. Use them judiciously, especially in performance-sensitive areas of your code.
- Make Use of C++11 and Later Features for Type Safety: Use features like `std::unique_ptr`, `std::shared_ptr`, and `std::variant` to help manage types and memory without unnecessary complexity.
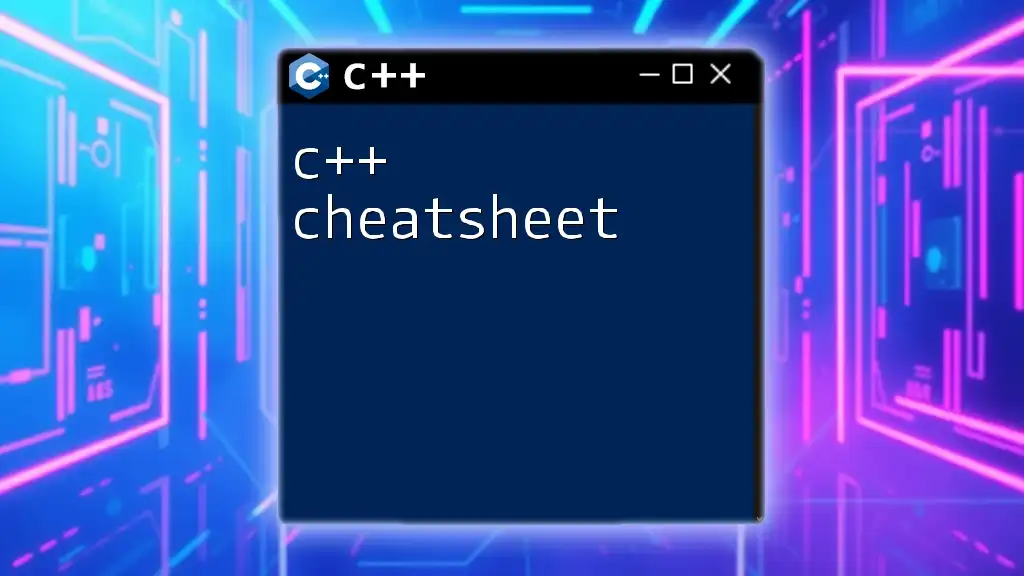
Conclusion
Understanding how to c++ check data type is an integral part of developing efficient and error-free applications. By leveraging the tools and techniques discussed, developers can enhance their code's robustness and maintainability. It's essential to practice checking types with examples to reinforce these concepts deeply.
In your journey to master C++, remember that every small practice increases your proficiency. Embrace the challenges and keep learning!