In C++, a space character can be represented using the `'\ '` escape sequence, and it is commonly used in string outputs to format text.
Here's a code snippet to demonstrate its usage:
#include <iostream>
int main() {
std::cout << "Hello," << ' ' << "world!" << std::endl; // Outputs: Hello, world!
return 0;
}
Understanding the Space Character in C++
What is a Space Character?
The space character is a fundamental aspect of programming in C++. It serves as a separator between characters, words, and commands, making it essential for maintaining clarity and readability in code.
In ASCII, the space character has a decimal value of 32. It is crucial to distinguish between the space character and other whitespace characters such as tabs (`\t`) and newlines (`\n`), as each plays a unique role in formatting and structuring data.
The Role of the Space Character in C++
The space character significantly enhances code readability. For instance, consider the following two snippets:
Without space:
std::cout<<"Hello,World!";
With space:
std::cout << "Hello, World!";
The second version, with appropriate spaces, is much easier to read, clearly indicating the output stream and message. This shows the importance of spaces in improving human comprehension of the code.
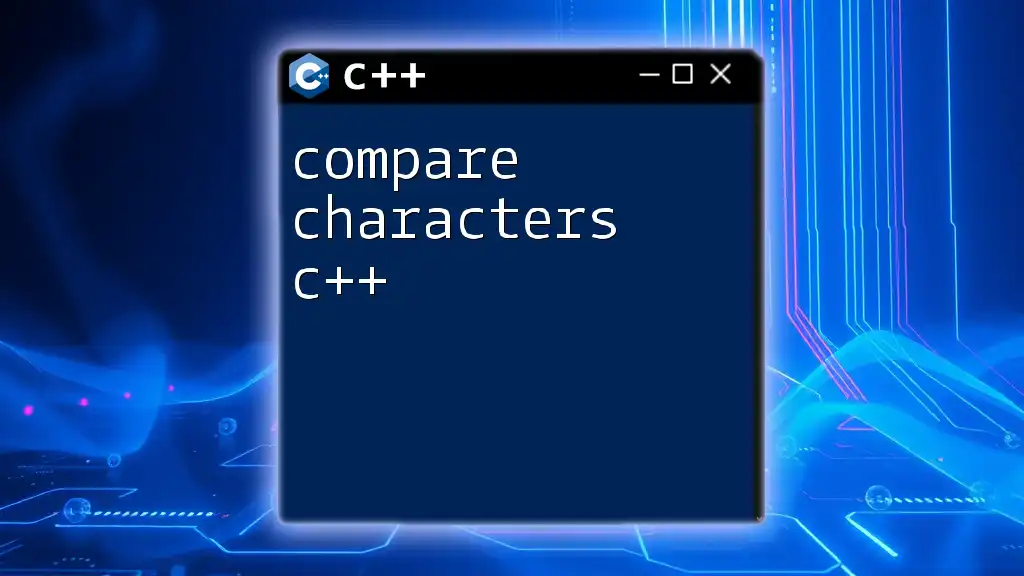
How to Use the Space Character in C++
Inserting Space Characters in Strings
Inserting space characters into strings is straightforward in C++. A string literal can include space naturally, as demonstrated in the code example below:
std::string message = "Hello, World!";
std::cout << "Message: " << message << std::endl;
In this example, the space character is effectively used to separate "Hello," from "World!" within the string.
Using Space Characters for Formatting Output
Formatting the output of your programs is essential for user-friendly interfaces. The space character plays a key role in how data is presented. C++ allows for the use of output manipulators, such as `std::setw`, to manage the placement of space characters. Consider the following code sample:
#include <iomanip>
std::cout << std::setw(10) << "Column" << std::setw(10) << "Value" << std::endl;
In this snippet, `std::setw(10)` ensures that both "Column" and "Value" are aligned and spaced correctly in the output, showcasing how the space character can enhance visual organization.
Space Character in Character Arrays
You can also utilize space characters in C-style strings (character arrays). Here’s an example:
char str[] = "Hello World";
std::cout << str << std::endl;
Here, "Hello World" contains a space character between "Hello" and "World," demonstrating its function in character arrays.
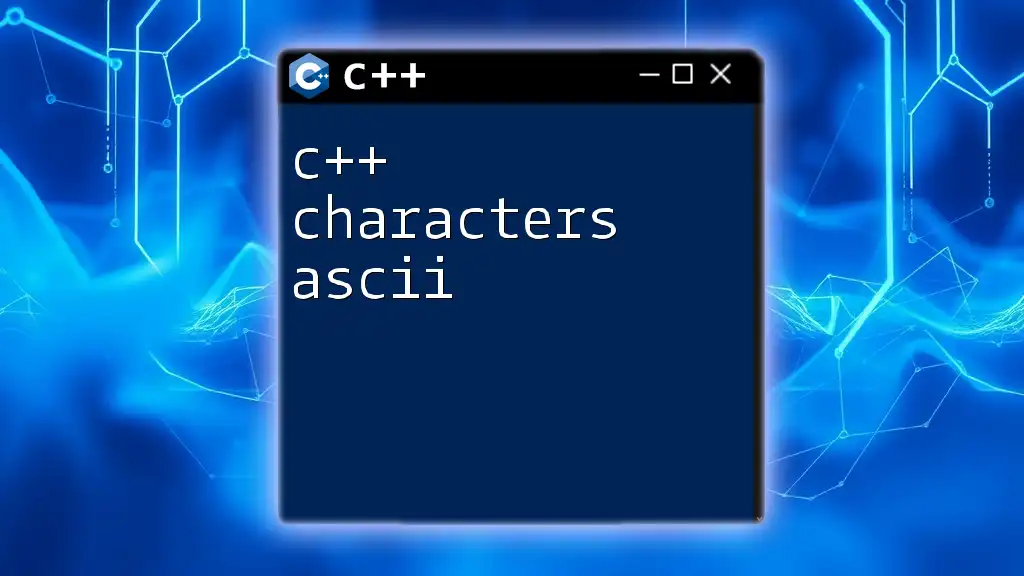
Manipulating Space Characters
Checking for Space Characters in Strings
To work effectively with strings, sometimes it is necessary to check for the presence of space characters. C++ provides the `isspace()` function from `<cctype>`, which can be incredibly useful. The following code iterates through a string and checks for space characters:
for (char c : message) {
if (isspace(c)) {
std::cout << "Space found!" << std::endl;
}
}
In this code, the program will print "Space found!" whenever it encounters a space character, making use of the `isspace()` function to determine its presence.
Removing Space Characters from Strings
In cases where you need to clean up a string by removing all space characters, C++ makes this operation quite straightforward. You can use `std::remove_if` along with a lambda function for this purpose. Here’s an example:
message.erase(std::remove_if(message.begin(), message.end(), ::isspace), message.end());
This snippet erases all space characters, creating a condensed version of the string.
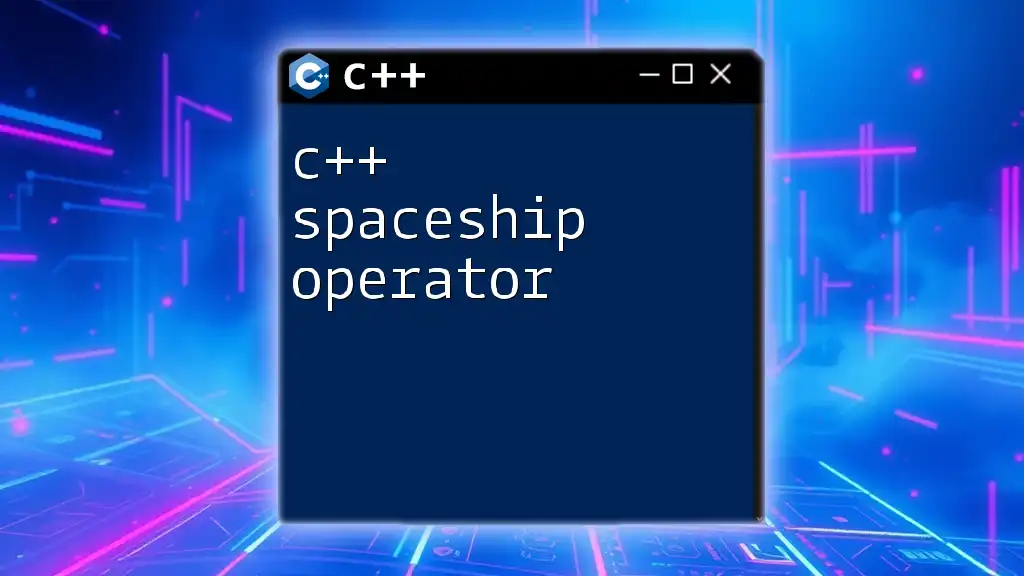
Common Pitfalls and Best Practices
Misunderstanding Whitespace Handling
A common pitfall when working with whitespace, including the space character, is misunderstanding how it is handled in different contexts. For instance, excessive or insufficient spacing around operators and keywords can lead to compilation errors or reduced readability in your source code.
Best Practices for Using Space Characters
To maintain clear, professional-looking code, follow these best practices regarding space character use:
- Consistency: Always follow a consistent style guide for spacing. This could include rules about spacing around operators, after commas, and before opening braces.
- Readability: Use spaces generously to enhance readability, making your code easier to understand for yourself and others.
Referencing established coding style guides can provide additional context and rules for proper use of space characters and formatting in your C++ code.
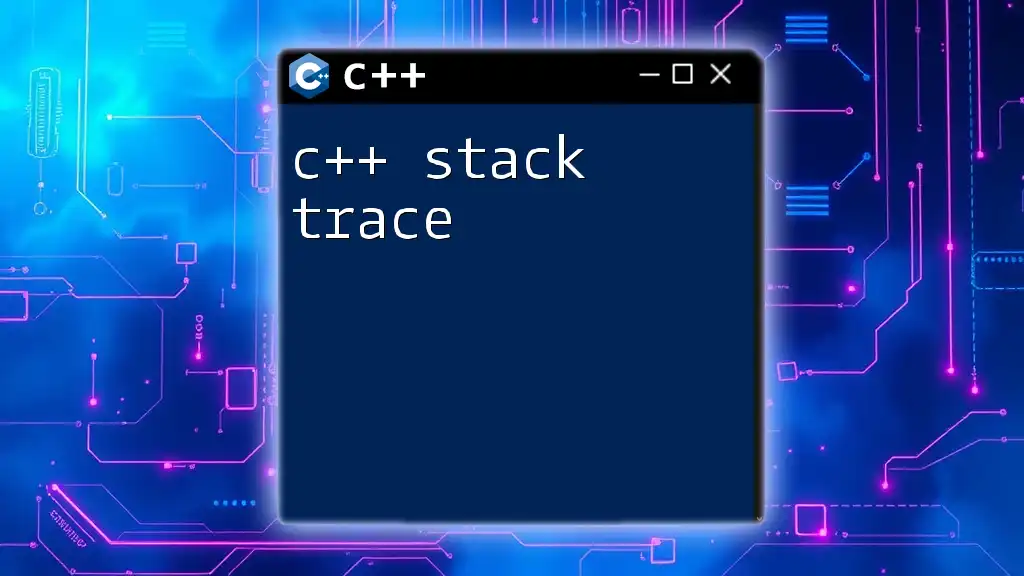
Conclusion
Understanding the C++ space character is vital for any programmer aiming to write clear, effective code. From inserting spaces in strings to manipulating and removing them, the space character plays an essential role in structuring data. By mastering its use, you can significantly improve the quality and readability of your programming, making your code more maintainable and user-friendly.
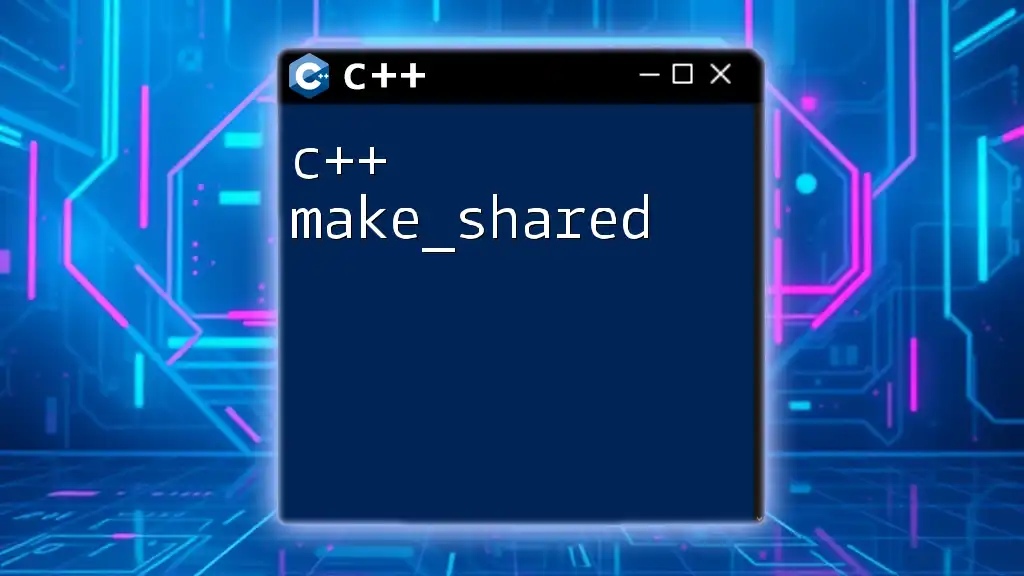
Additional Resources
For further learning, explore online documentation, tutorials, and coding communities that offer in-depth insights and practice opportunities for mastering C++, with a focus on effectively using space characters. Recommended books on C++ programming can also provide valuable context and examples to deepen your understanding.