In C++, an empty character is represented by `'\0'`, which is the null terminator indicating the end of a string or an empty character.
Here's a code snippet demonstrating the usage of an empty character:
#include <iostream>
int main() {
char emptyChar = '\0'; // Assigns an empty character
std::cout << "The ASCII value of the empty character is: " << int(emptyChar) << std::endl;
return 0;
}
What is an Empty Character?
In C++, the term "empty character" may refer to the concept of a character that represents nothing or is perceived as blank. This definition can be misleading, as it might lead one to confuse it with the null character. To clarify, an empty character isn't fundamentally a character that has no value; rather, it can be thought of as a placeholder or an indication of an absence of visible characters.
In C++, characters can be represented in various ways, including individual characters and character arrays. Understanding how "empty" relates to these types is important for proper use and manipulation of data structures in your programs.
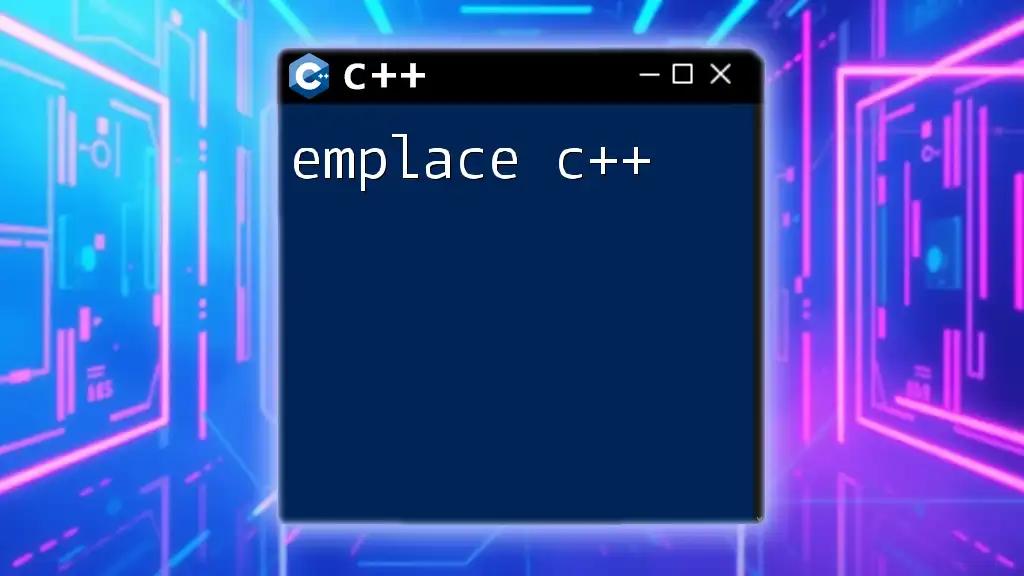
The Concept of Null Characters
What is a Null Character?
At the core of discussing an empty character lies the null character, denoted as `'\0'`. This character acts as a terminator for strings in C++ and is essential when working with character arrays. It signifies the end of a string, allowing functions to determine where the string data terminates. Importantly, while an empty character may sometimes evoke thoughts of nothingness, it's the null character that plays a critical role in string handling in C++.
Uses of Null Characters in C++
Null characters have two primary uses in C++: they terminate character strings and help manage memory effectively. When dealing with a character array, placing a null character at the end allows functions like `strlen()` or `strcpy()` to know when to stop reading, thus preventing out-of-bounds access.
Here's a simple code snippet demonstrating this concept:
char str[10] = "Hello";
str[5] = '\0'; // Null-terminating the string
In this example, `Hello` is followed by a null character, marking the end of the string in the array.
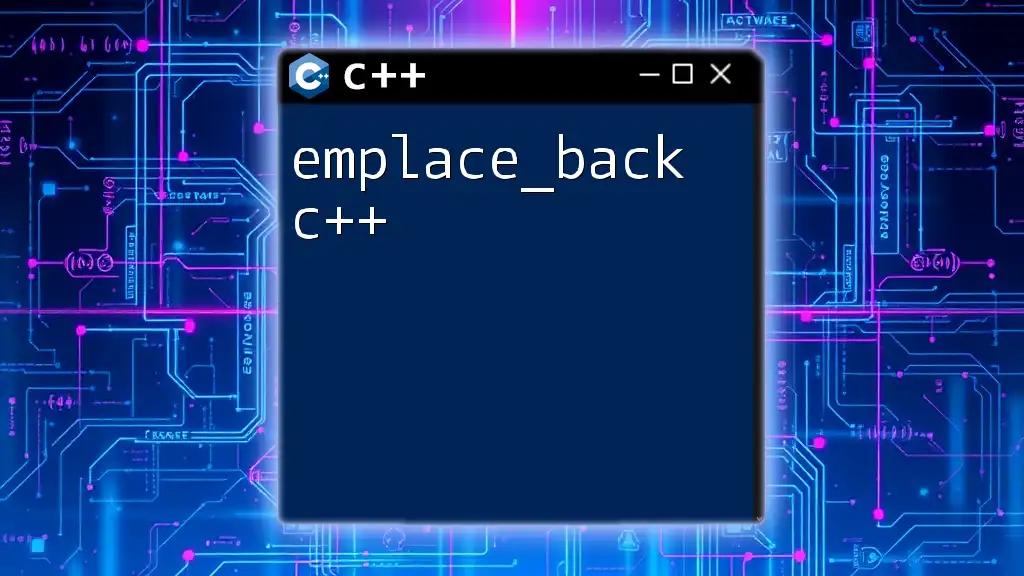
Creating an Empty Character
Empty Character in Character Literals
In C++, you can declare an empty character using single quotes. Although it sounds contradictory, an "empty character" can refer to a space character:
char emptyChar = ' '; // A space character
While this space character may not appear "empty" in a visual sense, it occupies a position in memory and can serve various purposes in string manipulation or formatting.
Using an Empty Character in Strings
The presence of empty characters can significantly alter string operations. For instance, appending an empty character to a string allows for maintaining structure without visibly altering the output:
std::string myString = "Hello";
myString += ' '; // Appending an empty space
In this example, a space character is added to `myString`, which will visually represent an empty character when printed but will still affect string length and structure in memory.
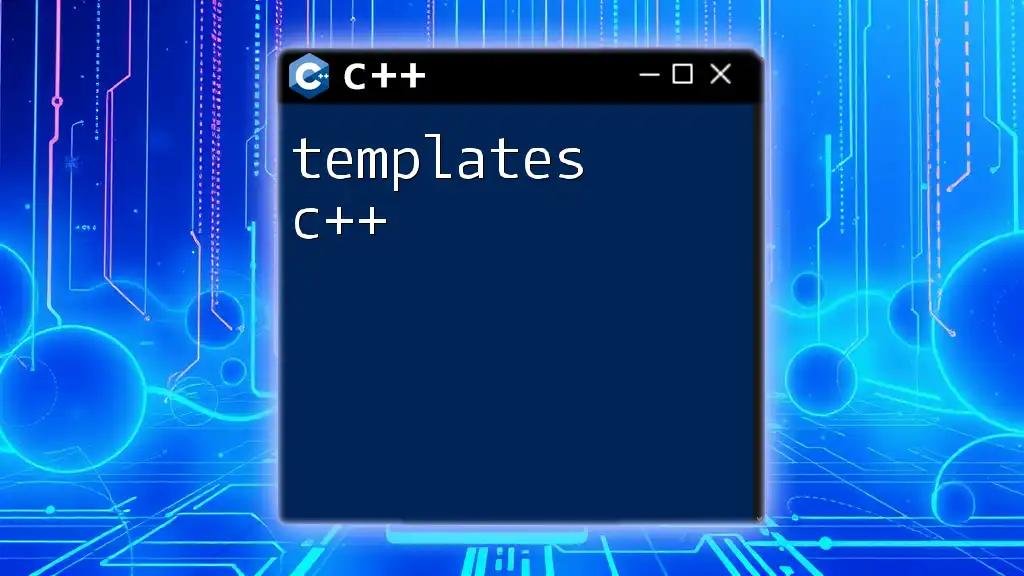
Practical Applications of Empty Characters in C++
String Manipulation
Empty characters are particularly useful when manipulating strings. By incorporating empty characters or spaces, developers can adjust user interfaces, manage input, and format output accordingly.
Consider the following example where an empty character (or a space) is utilized:
std::string example = "Hello ";
example += ""; // Still "Hello "
Even though the empty string appended here does not change the visible content of `example`, it illustrates how empty characters could be introduced in more complex scenarios without altering the existing information.
Input and Output Operations
When dealing with user input, an understanding of how empty characters operate is essential. For instance, when you read characters from standard input, an empty character can be captured:
char ch;
std::cin >> ch; // Can still accept ' ' as input
In this example, if the user inputs a space, it is treated as a valid character and is assigned to `ch`. This behavior demonstrates how empty characters interact with standard input and shows the importance of handling such cases gracefully.

Common Mistakes with Empty Characters
Misunderstanding The Concept
One common mistake when beginners discuss empty characters is the conflation of empty characters with null characters. It's crucial to approach the two concepts with clarity:
- Empty Character: A character that does not display any visible representation (like a space).
- Null Character: A terminator indicating the end of a string that cannot be visually represented.
Failing to understand this difference can lead to bugs in string handling and data manipulation.
Debugging Issues
When developers mishandle empty and null characters, it can result in subtle but frustrating bugs. For example, if a string is not null-terminated, subsequent operations may read past the intended data, causing undefined behavior or crashes.
To debug issues effectively, always ensure that character arrays are properly null-terminated. Additionally, pay attention to how empty characters influence string length and indexing when using them in calculations or algorithms.
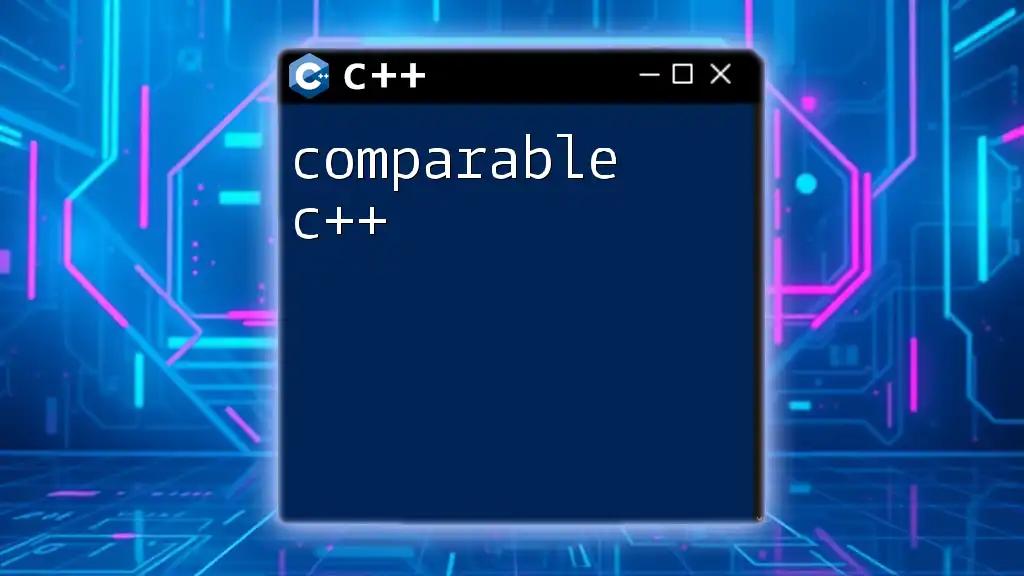
Conclusion
Understanding the concept of an empty character in C++ is vital for developers looking to manipulate strings and characters correctly. Whether you're dealing with string termination using null characters or utilizing space characters for formatting, grasping these fundamentals will enhance your coding skills and enable you to write cleaner, more efficient code.
Take the time to practice these concepts in your own projects, and don't hesitate to consult additional resources for deeper understanding as you delve into C++ character manipulation.