To delete a character from a string in C++, you can use the `erase` method, specifying the position of the character to remove and the number of characters to delete. Here's a simple example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.erase(5, 1); // Deletes the space at index 5
std::cout << str; // Output: HelloWorld!
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters that can store text. The string data type simplifies the handling of character data, which is crucial for many applications that involve user inputs and text processing. C++ provides a built-in string class within the standard library, allowing for convenient manipulation of strings.
Why Manipulate Strings?
String manipulation is essential in various real-world applications, such as parsing user input, formatting output, and processing text data. For instance, if a software application needs to clean up user input for database storage or search functionalities, being able to easily modify strings becomes crucial.
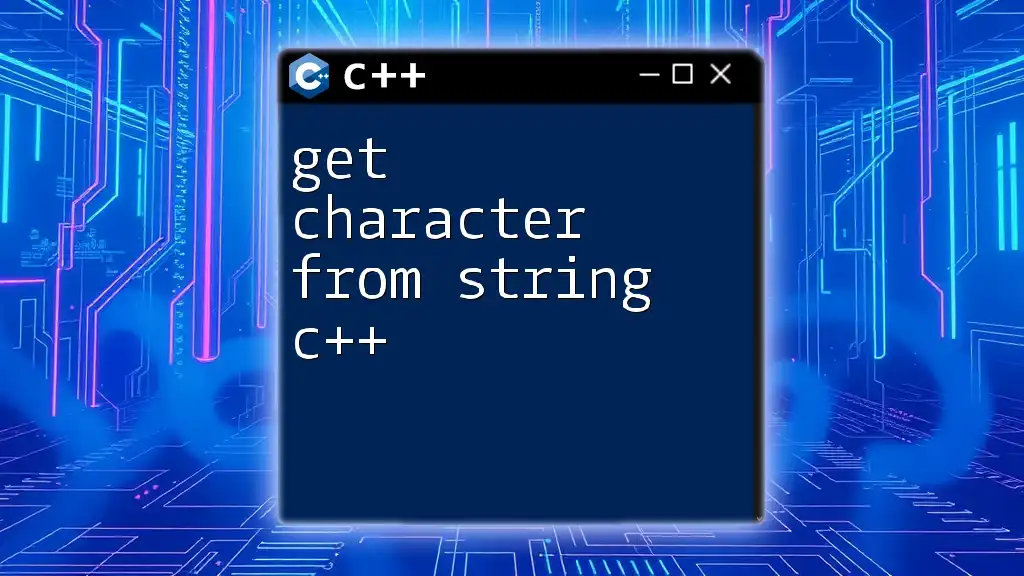
Basic String Operations in C++
Creating Strings in C++
You can create strings in several ways. The simplest way is to use the `std::string` class from the `<string>` header:
#include <iostream>
#include <string>
std::string str = "Hello, World!";
Common String Functions
C++ provides numerous functions to work with strings, such as:
- `length()`: Returns the number of characters in the string.
- `substr(start, length)`: Extracts a substring from the string.
- `find(char)` or `find(string)`: Searches for a character or substring within the string.
These functions form the backbone of effective string manipulation in C++.
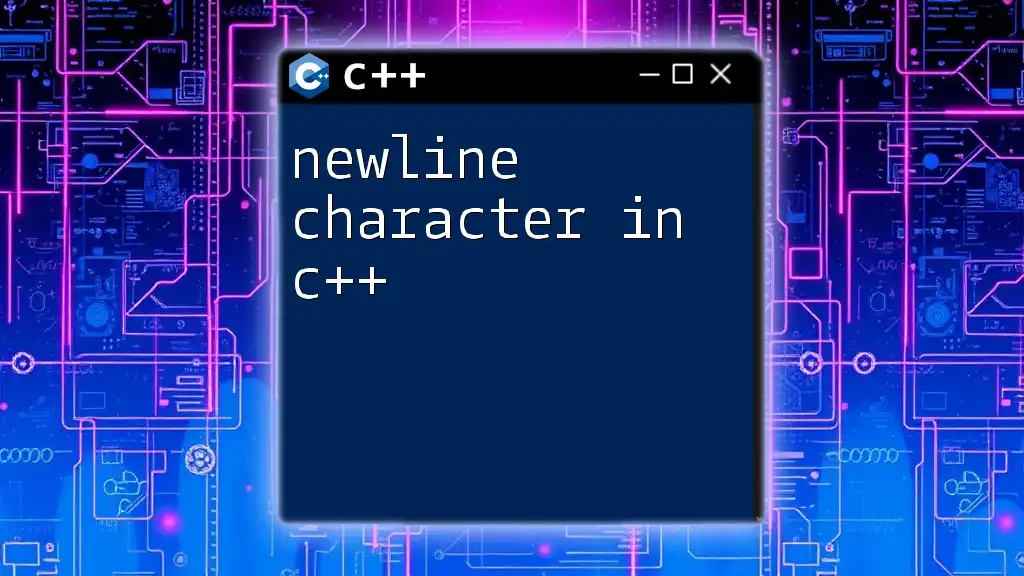
Methods to Delete a Character in a String
Removing a Character by Index
Using the `erase()` Method
The `erase()` method is a powerful way to remove a character from a string by its index.
For example, if you want to delete the comma in "Hello, World!", you would do the following:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
str.erase(5, 1); // Removes the character at index 5
std::cout << str; // Output: Hello World!
return 0;
}
Understanding Parameters of `erase()`
The `erase()` method takes two parameters: the starting index and the number of characters to delete. It modifies the original string by removing the specified characters and can be particularly effective for single-character deletions.
Removing Specific Characters
Using the `remove()` + `erase()` Idiom
If you need to remove all occurrences of a specific character, the `remove()` function in conjunction with `erase()` is very effective.
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
str.erase(std::remove(str.begin(), str.end(), 'o'), str.end());
std::cout << str; // Output: Hell, Wrld!
return 0;
}
Here, `std::remove` shifts the elements that should not be removed to the front of the string, and then `erase()` truncates the string to the new length.
Iterative Approaches
Using a Loop to Remove Characters
For more control, you might iterate through the string and build a new one without certain characters.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string result;
char charToRemove = 'o';
for (char c : str) {
if (c != charToRemove) {
result += c;
}
}
std::cout << result; // Output: Hell, Wrld!
return 0;
}
This method allows you to easily customize which characters to include in the new string.
Using `std::string::find()`
Finding and Deleting Characters
You can also leverage the `find()` function to locate a character before removing it. This can be useful when you need to delete a character based on its context or after checking its existence.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
size_t pos = str.find('W');
if (pos != std::string::npos) {
str.erase(pos, 1);
}
std::cout << str; // Output: Hello, orld!
return 0;
}
The `find()` method returns the index of the first occurrence of the specified character, allowing for targeted deletions.
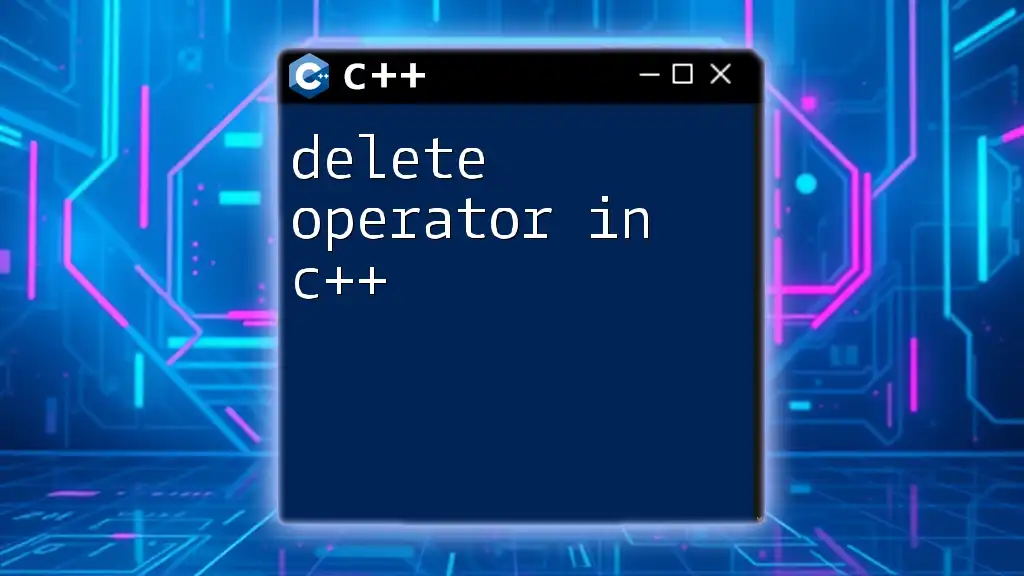
Working With Character Arrays
Delete Char in String using Character Arrays
If you're working with character arrays instead of `std::string`, the approach is slightly different.
Here's an example of how to delete a character in a character array:
#include <iostream>
int main() {
char str[] = "Hello, World!";
char charToRemove = 'o';
int j = 0;
for (int i = 0; str[i]; i++) {
if (str[i] != charToRemove) {
str[j++] = str[i];
}
}
str[j] = '\0'; // Null-terminate the array
std::cout << str; // Output: Hell, Wrld!
return 0;
}
In this example, each character is inspected, and only those not matching `charToRemove` are copied into a new position in the array.
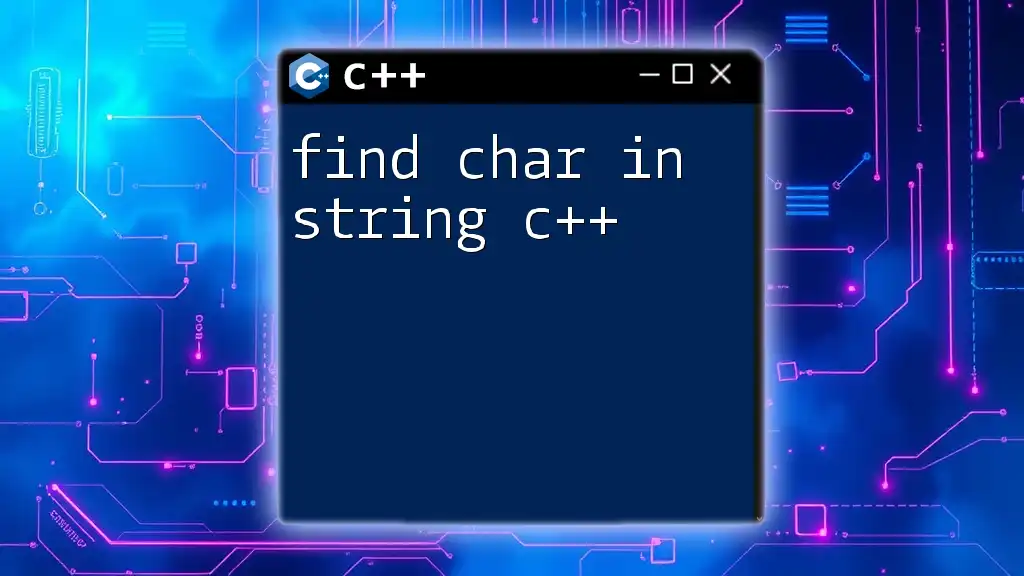
Performance Considerations
Time Complexity of Different Methods
The time complexity of various string manipulation methods can vary significantly. For instance, using `find()` followed by `erase()` is generally efficient, while looping through the string might result in higher complexity if done incorrectly.
When to Use Each Method
- Use `erase()` for precise indexing.
- Use the `remove() + erase()` idiom for removing all occurrences of a character.
- Use loops for more customized deletion logic.
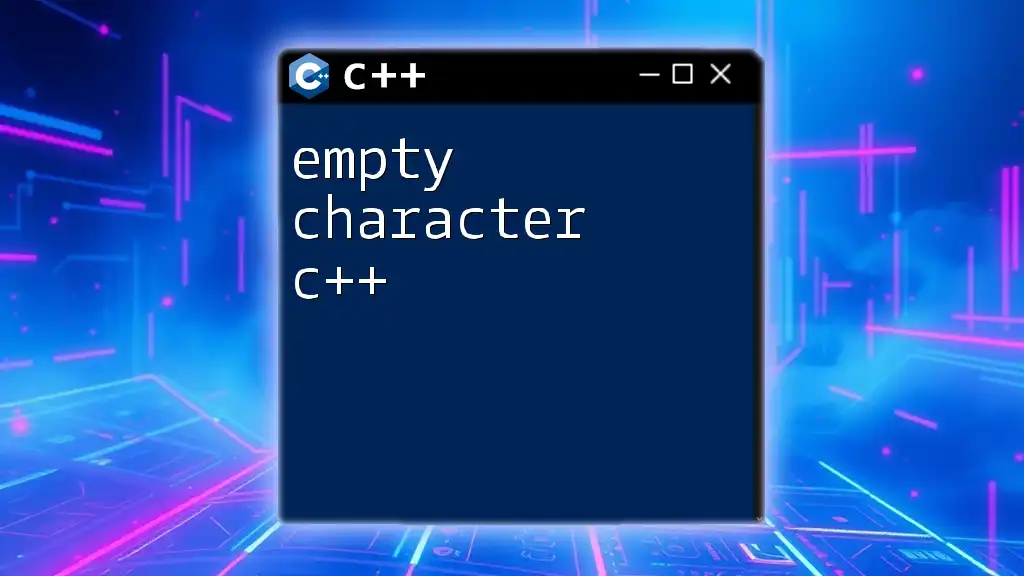
Common Mistakes and How to Avoid Them
Accessing Invalid Indices
A common mistake is trying to access an index that doesn't exist in the string. Always ensure that your indices are within the valid range.
Modifying String Length During Iteration
When deleting characters while iterating, be cautious, as it can lead to skipping characters or accessing out-of-bounds memory. The previous examples illustrate how to handle this safely.
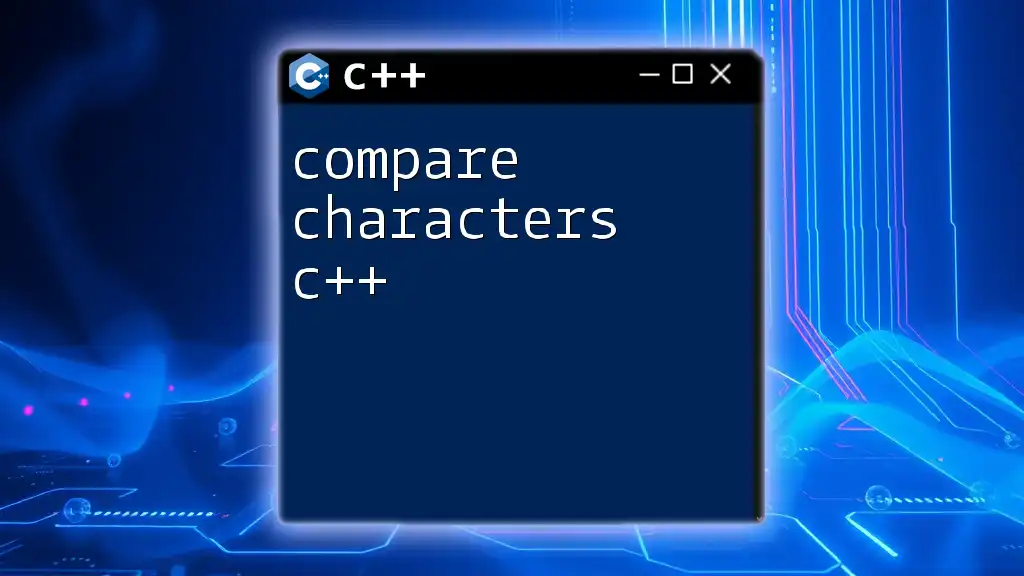
Conclusion
Manipulating strings in C++ is a fundamental skill for any software developer. By utilizing methods such as `erase()`, `remove()`, and loops, you can effectively delete a character in string C++ while keeping your code clean and efficient. Experimenting with these techniques allows for greater control over string data, enhancing your programming capabilities.