In C++, you can find a character in a string using the `find` method, which returns the index of the first occurrence of the character or `std::string::npos` if the character is not found. Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char ch = 'W';
size_t pos = str.find(ch);
if (pos != std::string::npos) {
std::cout << "Character found at index: " << pos << std::endl;
} else {
std::cout << "Character not found" << std::endl;
}
return 0;
}
Understanding Strings in C++
In C++, strings are primarily represented using the `std::string` class, which is part of the C++ Standard Library. Unlike C-style strings, which use character arrays and end with a null terminator, `std::string` provides a much more flexible and safer representation for managing text.
Basic String Operations
When working with strings in C++, you will often perform common operations such as initializing strings, concatenation, and modification. Here’s a quick reflection on some of these operations:
- Initialization: Strings can be initialized easily using literals or constructors.
- Concatenation: You can concatenate strings using the `+` operator or the `append()` method.
- Modification: Strings can be modified using various methods, such as `replace()` and `erase()`.

Finding a Character in a String
Finding a character in a string is a fundamental operation that enables you to perform various tasks like searching, filtering, or even data validation. Mastery of this skill is crucial for effective string manipulation in C++.
Approaches to Find a Character
In C++, there are several robust methods to find a character in a string, leveraging built-in functions from the Standard Library.
Using std::string::find()
One of the most straightforward ways to find a character in a string is by using the `std::string::find()` method. This method searches for the first occurrence of a specified character and returns its position.
Syntax:
size_t find(char ch) const noexcept;
Example: Basic Usage
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
size_t position = text.find('o');
if (position != std::string::npos) {
std::cout << "'o' found at index: " << position << std::endl;
} else {
std::cout << "'o' not found." << std::endl;
}
return 0;
}
In this example, the output will indicate the index where the character 'o' is found in the string. If the character is not present, the method returns `std::string::npos`, which is a constant representing an invalid position.
Using std::count()
Another useful method involves the `std::count()` function from the `<algorithm>` library. This function counts the occurrences of a specific character within a given range.
Example: Counting Occurrences
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string text = "banana";
char character = 'a';
int count = std::count(text.begin(), text.end(), character);
std::cout << "'" << character << "' appears " << count << " times." << std::endl;
return 0;
}
This example will provide the number of times 'a' appears in the string "banana", showcasing how `std::count()` is beneficial for tallying characters.
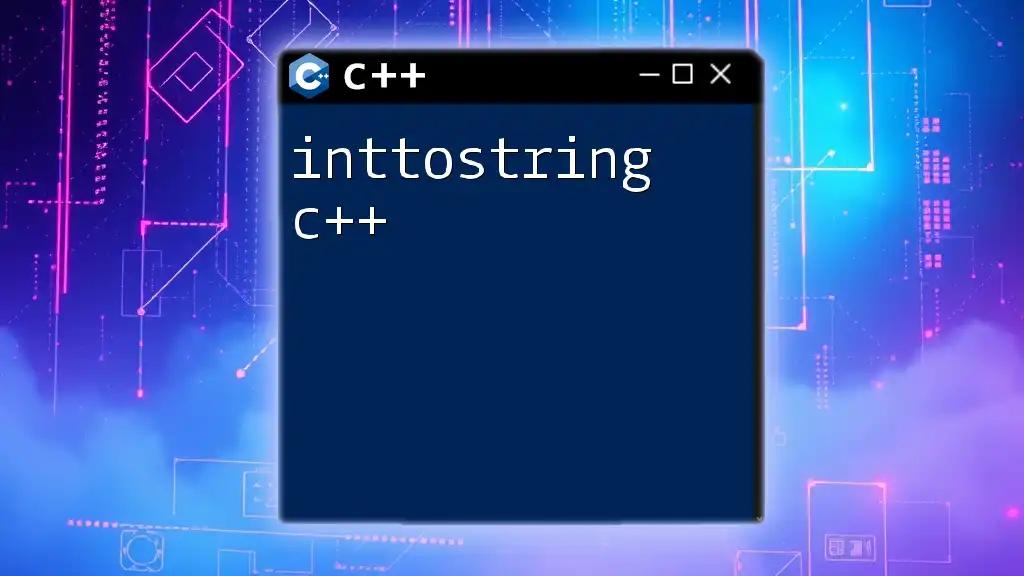
Handling Edge Cases
Searching for characters that do not exist in a string is a common situation that programmers encounter. Proper handling of such cases is essential to prevent errors and ensure the program runs smoothly. Always check if the result from the find operation equals `std::string::npos` to determine if the character was found.
Additionally, case sensitivity is an important consideration when searching for characters. In C++, the character 'A' is distinct from 'a'. If you need to perform a case-insensitive search, you should convert both the string and the character to the same case (either lower or upper) before searching.
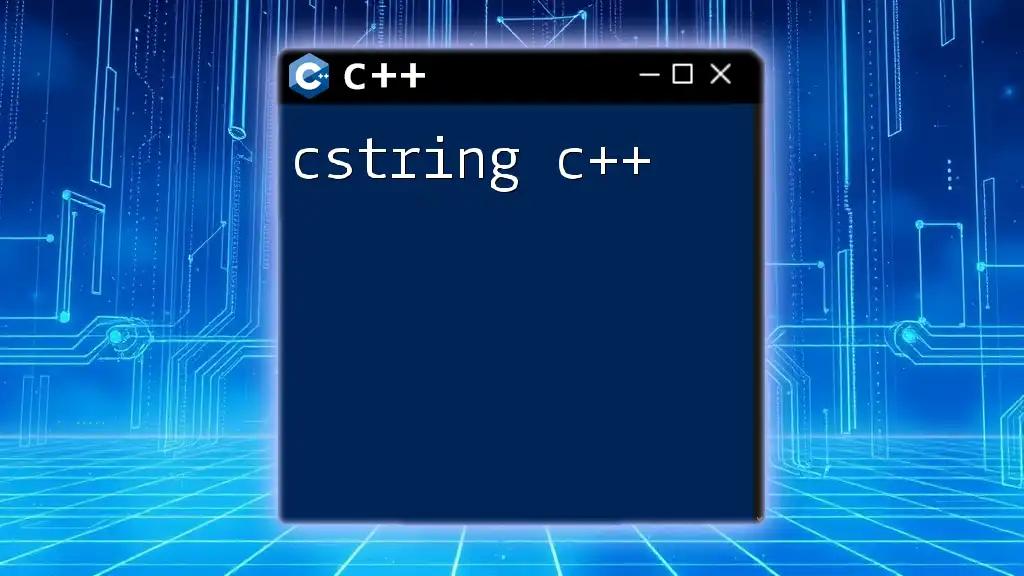
Advanced Techniques
Using Regular Expressions
For more complex search requirements, you can leverage the `<regex>` library. Regular expressions allow for pattern-based searches, which can be significantly more powerful than simple character searches.
Example: Regex Search
#include <iostream>
#include <string>
#include <regex>
int main() {
std::string text = "Hello, World!";
std::regex pattern("o");
bool found = std::regex_search(text, pattern);
std::cout << "Character 'o' found: " << (found ? "Yes" : "No") << std::endl;
return 0;
}
In this example, the `std::regex_search()` function checks for the presence of the character 'o' in the string "Hello, World!", demonstrating how regex can add versatility to character searches.
Performance Considerations
When choosing a method to find a character in a string, it's essential to consider efficiency. The performance of different methods can vary depending on their underlying implementation:
- `std::string::find()` has a time complexity of O(n), where n is the length of the string, as it may need to check each character.
- `std::count()` also operates in O(n) time complexity since it iterates through the entire string to count occurrences.
- Regular expressions may have an overhead due to their complexity but can lead to more powerful searching capabilities in more extensive, pattern-based needs.
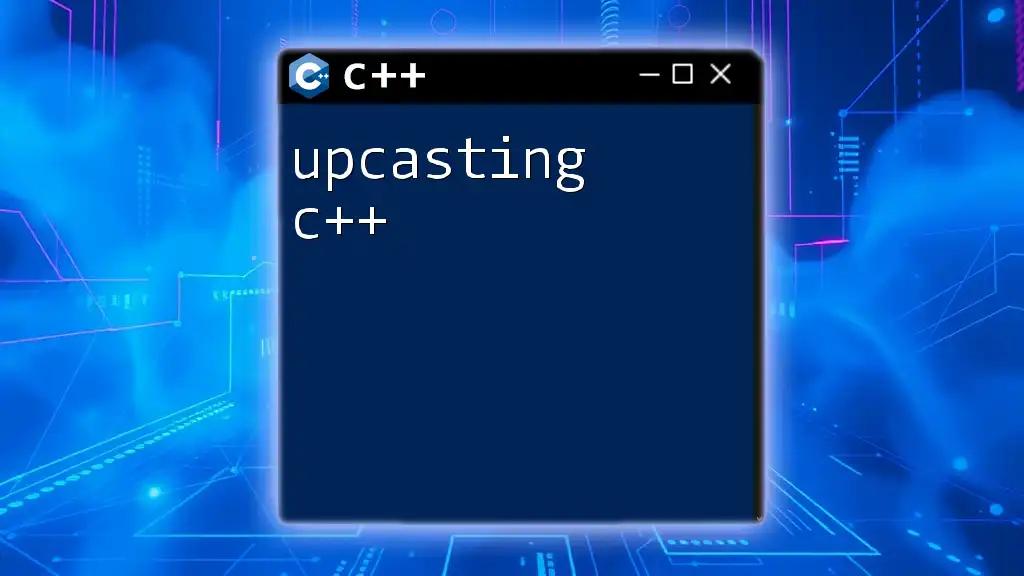
Conclusion
In conclusion, finding a character in a string using C++ is an essential skill that can enhance your programming proficiency. By leveraging built-in functions like `std::string::find()` and `std::count()`, or using advanced techniques such as regular expressions, you can perform character searches effectively and efficiently.
I encourage you to explore different examples and practice finding characters in various strings to solidify your understanding of this essential topic. Don't hesitate to share your own experiences or ask questions—engagement will help deepen your mastery of C++.

Additional Resources
For further exploration, consider checking out recommended books and reliable websites. Also, refer to the [C++ documentation for `std::string`](https://en.cppreference.com/w/cpp/string/basic_string) and the `<algorithm>` library to expand your knowledge on string manipulation methods.
If you have experiences or questions about how to effectively "find char in string c++", feel free to reach out and share!