A flowchart in C++ visually represents the logic and sequence of commands in a program, helping to clarify the structure and flow of control.
Here's a simple example of a flowchart represented in C++ code that calculates the factorial of a number:
#include <iostream>
using namespace std;
int main() {
int n, factorial = 1;
cout << "Enter a positive integer: ";
cin >> n;
for (int i = 1; i <= n; ++i) {
factorial *= i; // Multiply factorial by each integer up to n
}
cout << "Factorial of " << n << " = " << factorial << endl;
return 0;
}
What is a Flowchart?
A flowchart is a visual representation of a process, where each step is delineated by symbols that illustrate the operations involved. In programming, flowcharts are instrumental in planning algorithms and illustrating the flow of control within a program. They promote better understanding and analysis, enabling programmers to view the full picture before diving into the code.

Why Use Flowcharts in C++?
Using flowcharts in C++ programming offers several advantages:
- Clarity: Flowcharts allow programmers to visualize how data flows through their code, making logic easier to comprehend.
- Problem Solving: Identifying the steps necessary to achieve a solution becomes simpler when visualized.
- Communication: Flowcharts can serve as an effective communication tool within teams, where complex logic needs to be shared with others who may not be familiar with the code.

Basics of Flowcharting
Key Symbols in Flowcharts
Understanding the symbols that comprise flowcharts is crucial for creating and interpreting them:
- Oval: Used for the Start and End of a process.
- Rectangle: Represents a process or operation.
- Diamond: Indicates a decision point where the flow can branch based on yes/no or true/false conditions.
- Arrow: Directs the flow of the process, connecting symbols and guiding the viewer through the sequence.
How to Read a Flowchart
Reading flowcharts involves following the arrows that guide the flow of steps. Decisions made in the diamond shapes often lead to different paths based on the answers to the posed questions. Recognizing the flow direction helps in understanding how to control program execution in C++, showcasing the dependencies between operations clearly.

Creating Flowcharts for C++ Programs
Steps to Create a Flowchart
When developing a flowchart for your C++ programs, follow these structured steps:
- Define the Process: Clearly articulate what you are trying to achieve with your program.
- Identify Inputs and Outputs: Determine what information needs to be entered or displayed.
- List Out the Steps: Break down the process into manageable actions that can be represented visually.
Example: Flowchart for a Simple C++ Program
Let’s consider a simple C++ program that determines whether a number is even or odd. The flowchart for this logic would look something like this:
+-----------------+
| Start |
+-----------------+
|
v
+-----------------+
| Input a number |
+-----------------+
|
v
+-----------------+
| Is number % 2 == 0? |
+-----------------+
/ \
Yes No
/ \
+----------------+ +--------------------+
| Print "Even" | | Print "Odd" |
+----------------+ +--------------------+
| |
v v
+-----------------------+
| End |
+-----------------------+
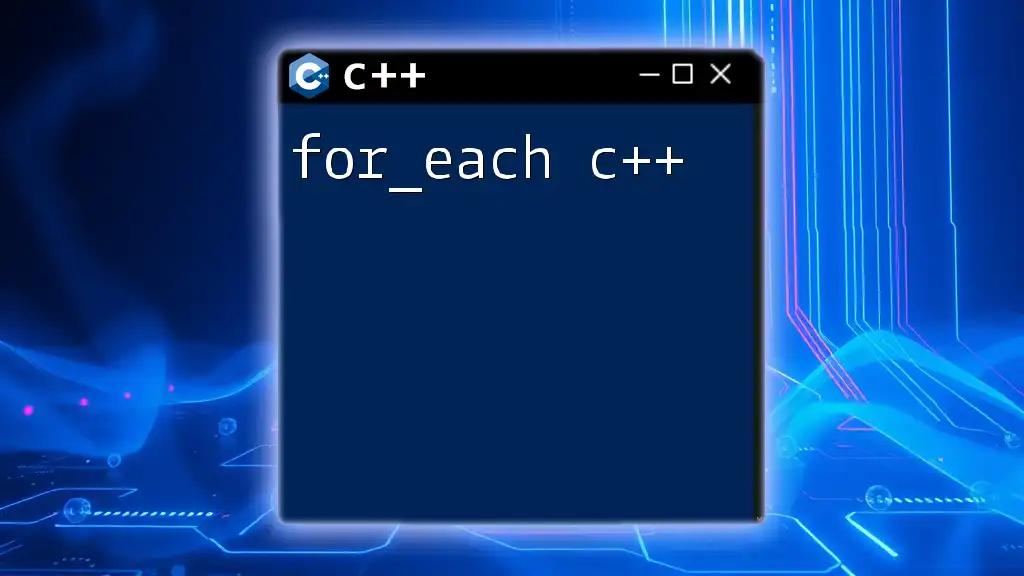
Converting Flowcharts to C++
Translating Flowcharts to Code
Translating a flowchart into C++ code requires a strong grasp of programming logic. Each shape in the flowchart corresponds to specific C++ commands. For our example, here is the corresponding C++ code for the even/odd checker:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (number % 2 == 0) {
cout << "Even" << endl;
} else {
cout << "Odd" << endl;
}
return 0;
}
In this code snippet, the `if` statement directly correlates to the decision diamond in the flowchart, showcasing how flowcharts effectively outline logical patterns.
Common Challenges When Converting
When converting flowcharts to C++, several challenges may arise, including:
- Understanding Logical Conditions: Translating decisions and their conditions into proper syntax needs attention to detail.
- Handling Different Data Types: Recognizing the appropriate data types for input can affect logic and outcomes.
- Flow Control: Ensuring that the flow through the program matches the flowchart can be tricky, requiring careful planning and testing.

Advanced Flowchart Techniques for C++
Using Flowcharts for Complex Logic
As problems become more intricate, so do flowchart representations. Creating flowcharts for complex logical structures enhances clarity. For instance, a flowchart evaluating grades based on score might look like this:
+-----------------+
| Start |
+-----------------+
|
v
+---------------------+
| Input student score |
+---------------------+
|
v
+---------------------+
| Is score >= 90? |
+---------------------+
/ \
Yes No
/ \
+----------------+ +---------------------+
| Print "A" | | Is score >= 80? |
+----------------+ +---------------------+
/ \
Yes No
/ \
+----------------+ +---------------------+
| Print "B" | | Is score >= 70? |
+----------------+ +---------------------+
/ \
Yes No
/ \
+----------------+ +----------------+
| Print "C" | | Print "F" |
+----------------+ +----------------+
|
v
+-----------------------+
| End |
+-----------------------+
This flowchart breaks down the evaluation of scores, leading to the print statements and validating the decision-making processes in a structured way. Each decision point flows logically, demonstrating how to implement this in C++.
Agile Development and Flowcharts
Integrating flowcharts within the Agile development framework can facilitate better iterative development processes. As requirements evolve, flowcharts can adapt quickly, allowing programmers to visualize changes in logic. This visual approach enables teams to collaborate effectively, ensuring everyone understands the current structure and flow.
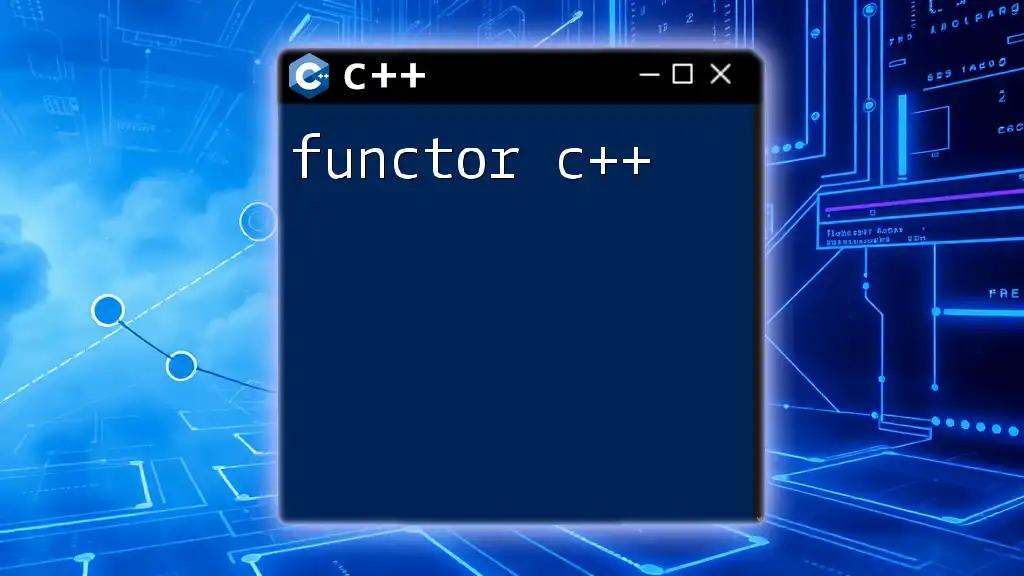
Tools for Creating Flowcharts
Software Options for Flowchart Creation
Many tools are available for creating flowcharts, with some popular options including Lucidchart, Microsoft Visio, and Draw.io. Each software offers various features tailored to different user needs. When selecting a flowchart tool, consider the following:
- User-friendliness and accessibility
- Collaboration features for teamwork
- Integrations with coding platforms and IDEs

Conclusion
Flowcharts serve as powerful tools within the realm of C++ programming. They facilitate clarity of thought and provide a blueprint from which developers can translate logic into code seamlessly. By visualizing the flow of information, programmers can enhance their problem-solving skills and significantly improve productivity.
Encouraging the use of flowcharting techniques in C++ programming is paramount for both novice and experienced developers alike. Dive into the resources available for learning C++ and start utilizing flowcharts to maximize your coding efficiency.

Additional Resources
To deepen your understanding of flowcharts and C++ programming, consider exploring comprehensive tutorials, guides, books, and online courses dedicated to these subjects. These resources can significantly enhance your programming proficiency, ensuring your success in the realm of coding.