In C++, declaring a variable means defining its name and type, enabling it to hold data in your program. Here's an example of a variable declaration in C++:
int age = 25;
What is Declaration in C++?
In C++, a declaration refers to the introduction of a program element, such as a variable, function, or class, along with its name and type. It tells the compiler about the type of entity being declared and allocates necessary resources.
Understanding the distinction between declaration and definition is essential. A declaration simply introduces the element, while a definition provides the actual implementation. For example, while you can declare a variable, the definition would assign it a value.
The Importance of Declaration
The process of declaration is pivotal for several reasons:
-
Memory Management: Proper declarations help the compiler allocate the correct amount of memory for variables and functions, optimizing resource usage.
-
Code Organization: By clearly declaring entities at the beginning of your code, readability is enhanced, allowing other developers (and your future self) to navigate the code more easily.
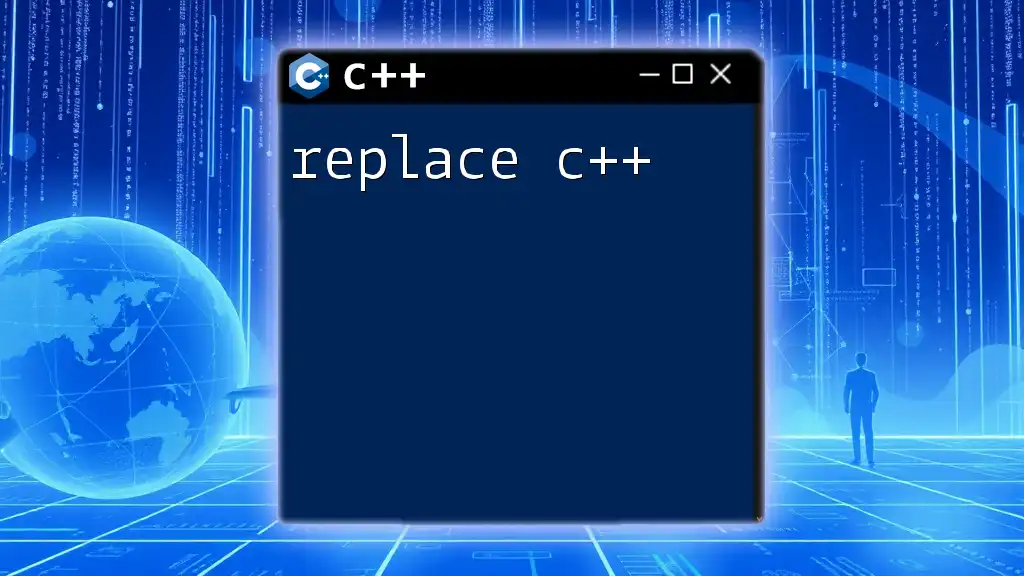
C++ Declaration Syntax
Understanding the syntax of declarations in C++ is essential for effective programming.
Basic Declaration Formats
Variable Declaration
The syntax for declaring a variable is:
data_type variable_name;
For instance:
int age;
float salary;
In this example, we have declared an integer variable named `age` and a floating-point variable named `salary`. It's vital to understand the variety of data types available in C++, such as `int`, `float`, `char`, and `double`, among others, as each serves a specific purpose.
Function Declaration
The syntax for declaring a function is:
return_type function_name(parameters);
For example:
int add(int a, int b);
In this case, we have declared a function named `add` which takes two integer parameters and returns an integer. Here, the parameters are crucial as they define the input the function expects.
Class Declaration
The basic syntax for declaring a class is as follows:
class ClassName {
// member variables and functions
};
An example:
class Car {
public:
void drive();
};
In this example, we declare a class named `Car` with a public member function `drive()`. Classes help us encapsulate data and behaviors together, forming the cornerstone of object-oriented programming.
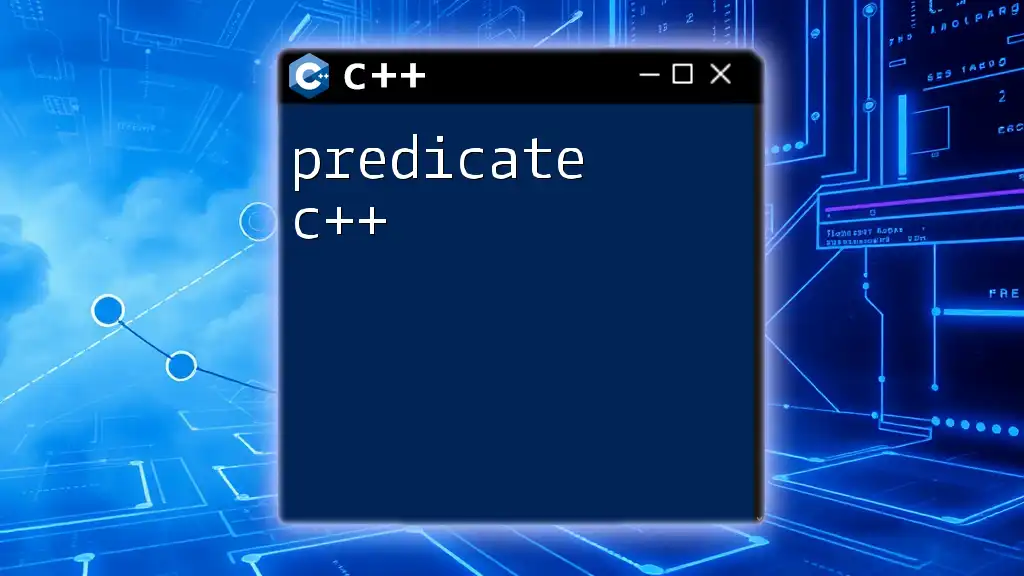
Different Types of Declarations
Variable Declarations
Single Declaration
A variable can be declared individually like so:
int height;
Multiple Declarations
C++ allows multiple variables to be declared in one line:
int length, width, area;
This is particularly useful when the variables are of the same type, as it allows for concise code representation.
Function Declarations
With and Without Parameters
You can declare functions that either require parameters or not:
void showMessage(); // no parameters
int multiply(int a, int b); // with parameters
Understanding how to effectively declare functions is vital for creating modular and reusable code.
Class & Object Declarations
Class Members
When declaring classes, you can organize member variables and functions like this:
class BankAccount {
private:
double balance;
public:
void deposit(double amount);
};
In this example, we declare a class `BankAccount` that has a private member variable `balance` and a public member function `deposit()`. This encapsulation containing both data and methods is a key feature of C++.
Object Creation
You can create an object of the declared class as follows:
BankAccount myAccount;
Now, `myAccount` can use the member functions and data defined within `BankAccount`.
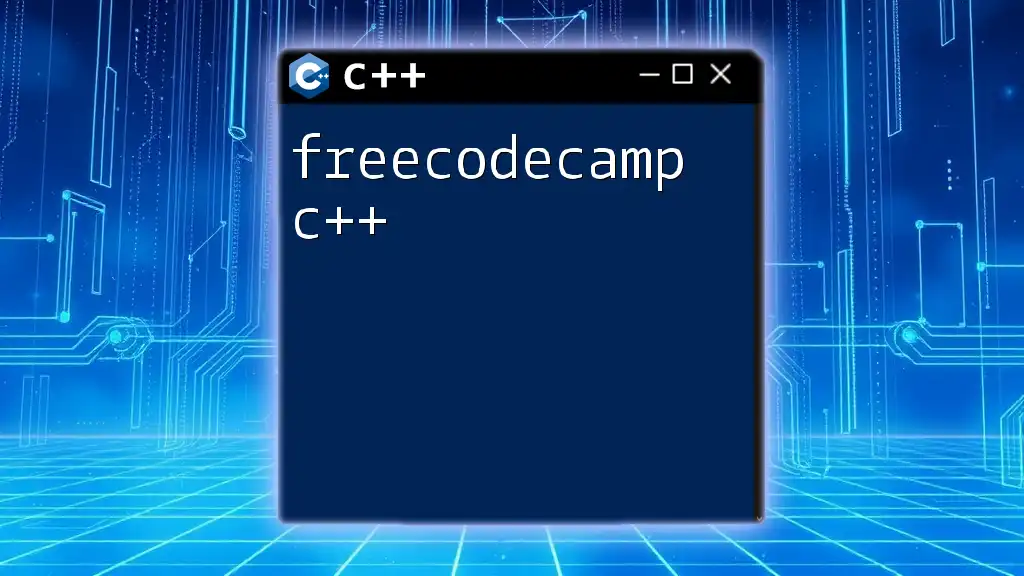
Scope of Declarations
Global vs Local Declarations
Understanding the scope of declarations is vital for cleaner and bug-free code.
-
Global Declarations: Variables declared outside any function and are accessible throughout the program.
-
Local Declarations: Variables declared within a function and are only accessible within that function. An example of a local variable would be:
void exampleFunction() {
int localVar = 10; // only accessible within this function
}
The `extern` Keyword
C++ includes the `extern` keyword which is used to declare a variable that is defined in another file:
extern int count;
This tells the compiler that `count` is defined elsewhere, ensuring that the variable is properly linked.
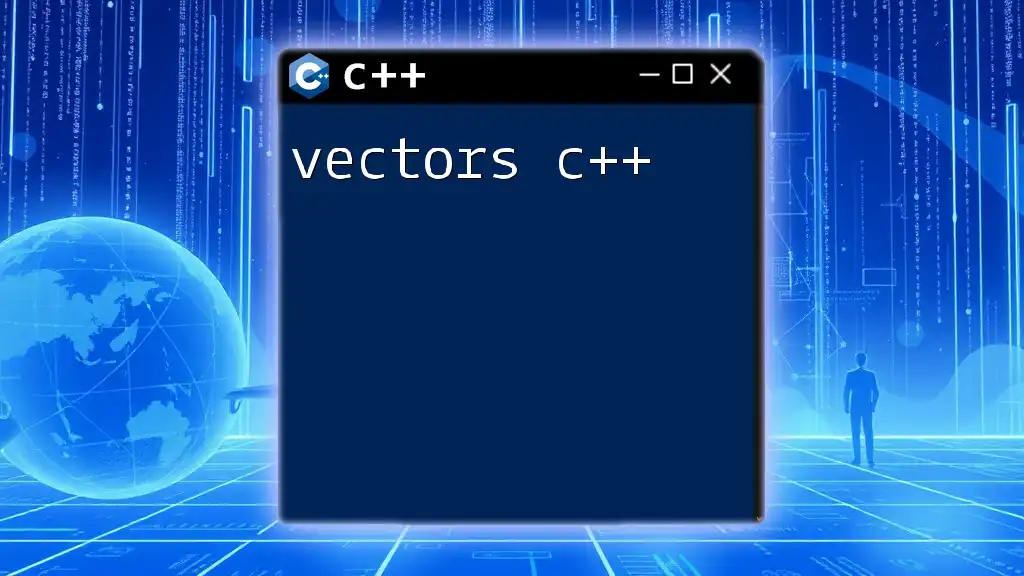
Initialization vs Declaration
While a declaration introduces the variable, initialization assigns it a value.
For example:
int number = 10; // initialization
Here, we have both declared `number` as an integer and simultaneously initialized it with a value of `10`.
Key Differences
These differences highlight the significance of initialization, as it is often necessary to assign values to variables upon declaration to avoid using uninitialized variables, which can lead to undefined behavior.
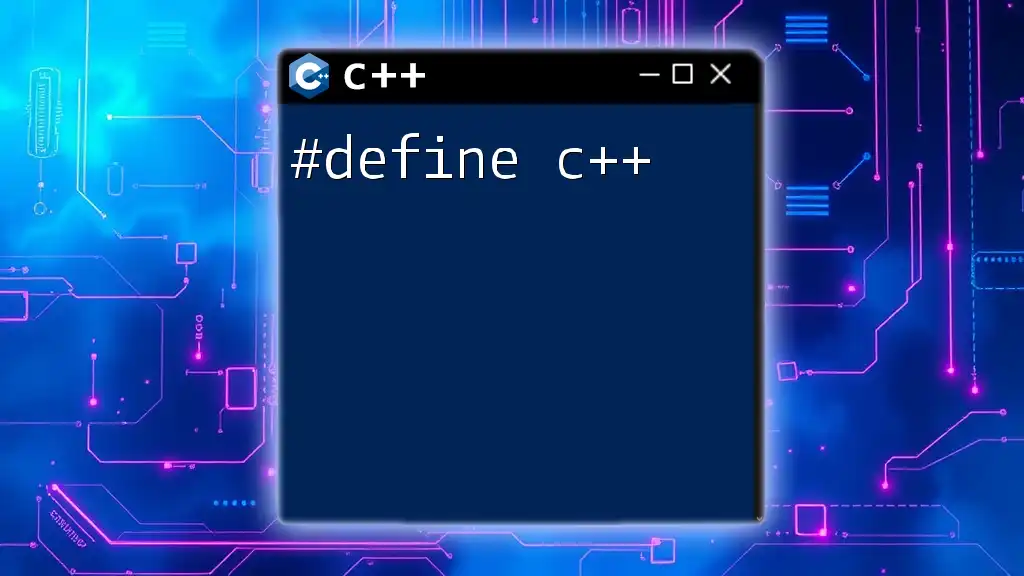
Best Practices for Declarations
Clarity and Readability
In C++, naming conventions play a crucial role in code clarity. Meaningful variable names that describe their purpose can dramatically enhance readability. Instead of using a vague name like `x`, use something descriptive like `accountBalance`.
Minimizing Errors
Being mindful of common mistakes in declarations can significantly reduce bugs in your code. For instance, watch for typos in variable names and ensure consistency in data types across declarations.
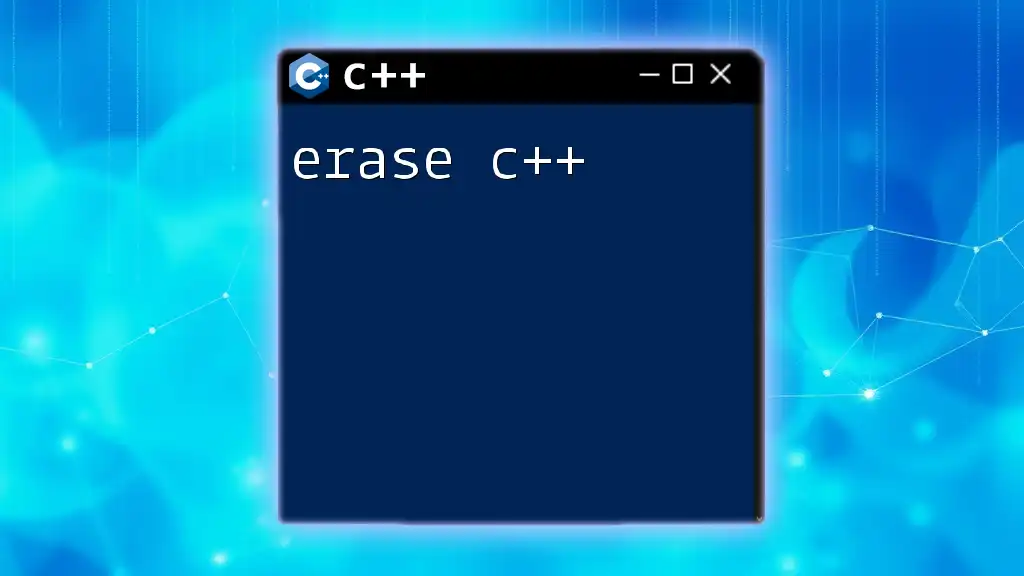
Common Pitfalls to Avoid
Several pitfalls can occur during the declaration process:
-
Overlapping Scope Issues: Always be aware of where variables are declared. If a local variable shares the same name as a global variable, the local variable takes precedence in that scope.
-
Misunderstanding Function Declarations vs Definitions: Many beginners confuse the two. Remember, a declaration tells the compiler about the function, while a definition provides its body.
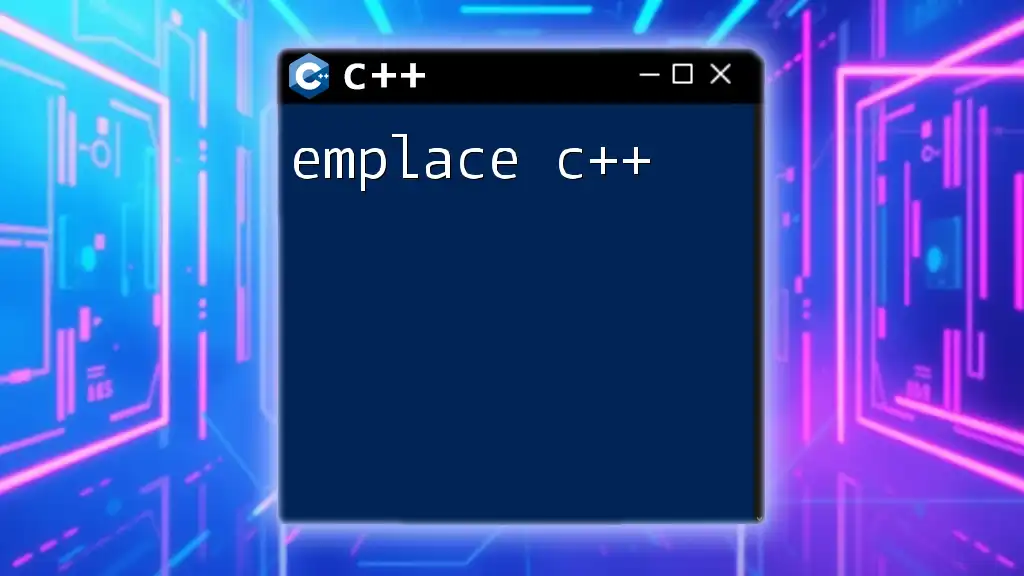
Conclusion
By mastering the concept of how to declare C++, you set a solid foundation for effective programming. Each type of declaration—variables, functions, and classes—contributes towards making your code efficient, organized, and understandable. Make sure to practice these concepts regularly to enhance your skill set and programming knowledge.