In C++, you can convert a character to its corresponding ASCII value by simply casting the character to an `int`. Here's a quick example:
#include <iostream>
int main() {
char ch = 'A';
int asciiValue = static_cast<int>(ch);
std::cout << "The ASCII value of " << ch << " is " << asciiValue << std::endl;
return 0;
}
Understanding ASCII Values
What is ASCII?
ASCII (American Standard Code for Information Interchange) is a character encoding standard that allows computers to represent and manipulate text. Developed in the early days of computing, ASCII defines a set of numeric representations for characters—from letters and digits to punctuation marks and control characters. The primary ASCII table contains 128 characters, each corresponding to an integer value from 0 to 127.
The Range of ASCII Values
ASCII values are categorized into two segments:
- Standard ASCII (0-127): This covers the basic English alphabet (both uppercase and lowercase), digits (0-9), and a selection of symbols and control codes.
- Extended ASCII (128-255): This range includes additional characters such as accented letters and graphical symbols. Most modern systems use variations of extended ASCII or other encoding systems, such as UTF-8, which accommodate a greater variety of characters.
For reference, here’s a brief look at some common ASCII values:
Character | ASCII Value |
---|---|
A | 65 |
B | 66 |
a | 97 |
b | 98 |
0 | 48 |
! | 33 |
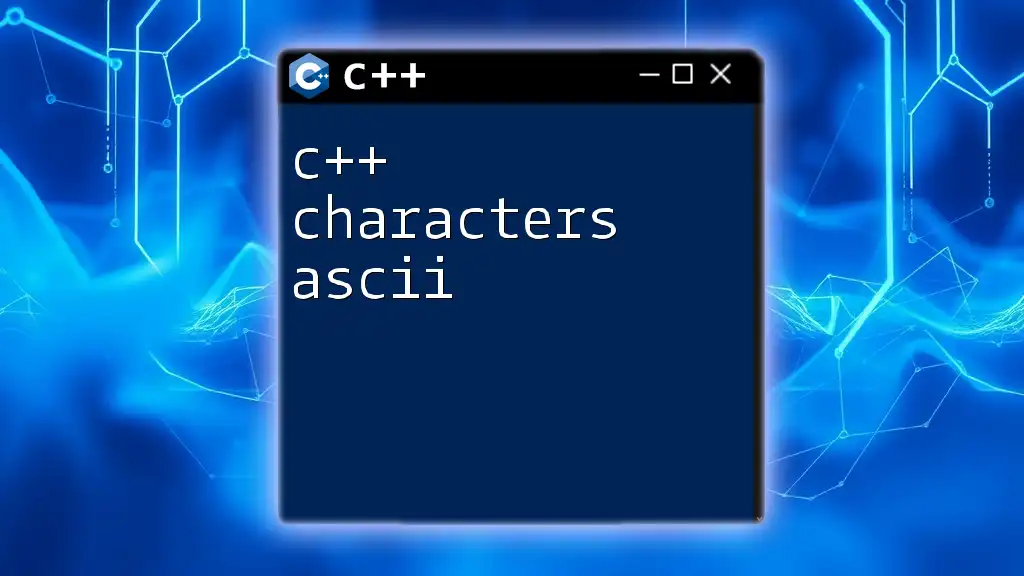
Character Data Type in C++
Defining Characters in C++
In C++, a character is typically represented using the `char` data type. This data type can hold a single character and is an essential building block for string manipulation in the language. Here is how you can declare and use character variables:
char myChar = 'A';
With this declaration, the variable `myChar` is holding the character A.
How to Get ASCII Value of Char in C++
Fetching the ASCII value of a character is straightforward in C++. By using type casting with `static_cast`, you can convert a character to its corresponding integer value. Here’s an example that demonstrates this:
char myChar = 'A';
int asciiValue = static_cast<int>(myChar);
std::cout << "The ASCII value of " << myChar << " is: " << asciiValue << std::endl;
In this example, when you run the program, it will output:
The ASCII value of A is: 65
This simple operation highlights how C++ allows for efficient character manipulation through numeric representations.
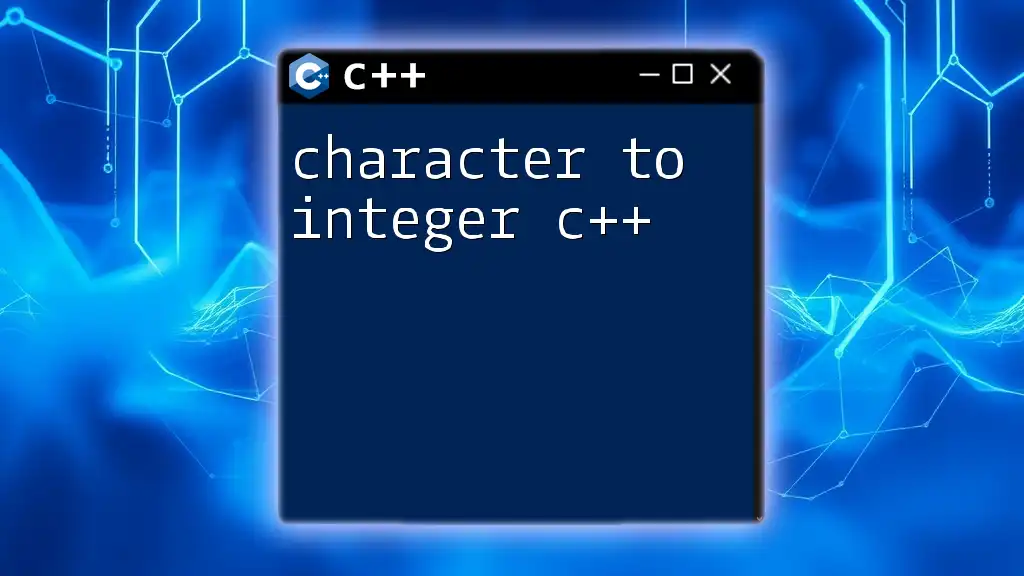
Converting ASCII Values Back to Characters
Understanding Character Conversion
Just as you can convert a character to its ASCII value, C++ also enables you to convert an ASCII value back into a character. This can be particularly useful when processing encoded data or when handling user input.
Example Code Snippet on Conversion
Converting an ASCII integer back to a character involves using `static_cast` again, this time in the reverse operation. Here's a code snippet:
int asciiValue = 65;
char myChar = static_cast<char>(asciiValue);
std::cout << "The character for ASCII value " << asciiValue << " is: " << myChar << std::endl;
Running this code will yield:
The character for ASCII value 65 is: A
This illustrates the ease with which C++ handles conversions between character data types and their integer equivalents.
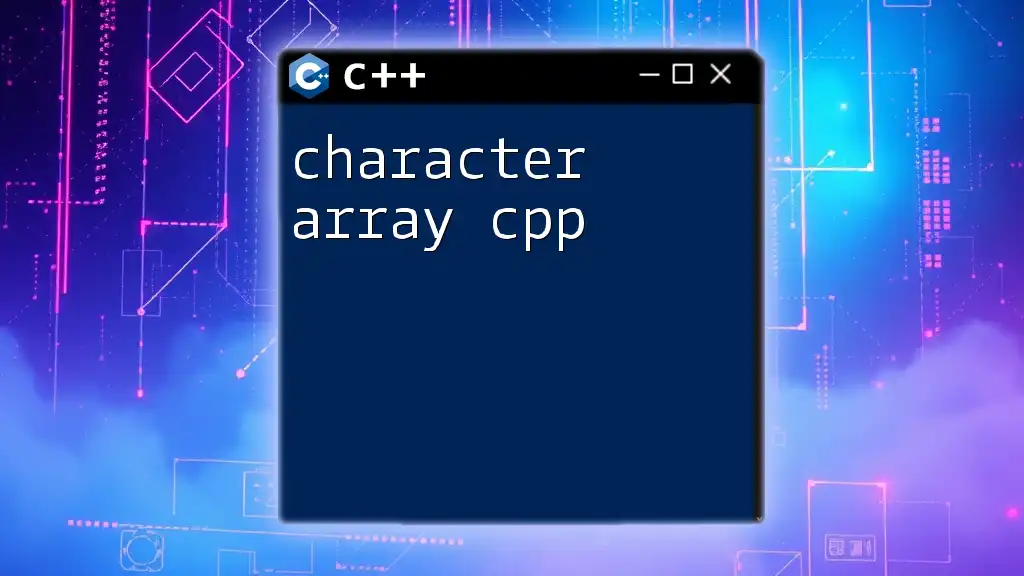
Using ASCII Values in C++ Applications
Common Use Cases
Understanding how to work with ASCII values has practical applications. Here are a few scenarios where ASCII manipulation plays a crucial role:
- Text Encoding: When transmitting or storing data, recognizing and manipulating character encodings can lead to compatibility with various systems.
- Sorting Characters: ASCII values can be used to sort characters easily, as integer values are naturally ordered.
- Data Analysis: In applications that process text, such as search engines or data mining, analyzing character frequencies can yield insights.
Example Application—Character Counting
To further illustrate the application of ASCII values, consider a simple program that counts how many times each ASCII character appears in a string:
std::string str = "Hello World!";
int count[256] = {0};
for (char c : str) {
count[static_cast<int>(c)]++;
}
for (int i = 0; i < 256; ++i) {
if (count[i] > 0) {
std::cout << "Character " << static_cast<char>(i) << " occurs " << count[i] << " times." << std::endl;
}
}
In this code, a string is analyzed character by character, and the occurrences of each character are tallied using an array. This simple counting algorithm can be adapted for various text analysis tasks, demonstrating the practical utility of ASCII values in programming.
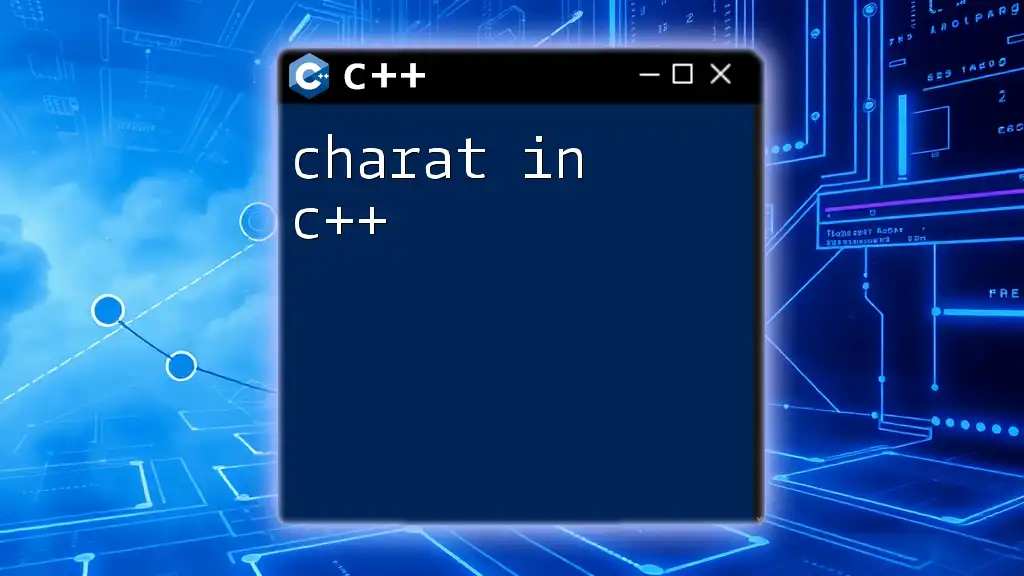
Debugging Common Issues with ASCII Conversions
Pitfalls in ASCII Conversions
When dealing with ASCII conversions, developers may encounter several pitfalls. Common mistakes include:
- Incorrect Type Casting: Forgetting to cast types may lead to unexpected results, especially when converting between characters and integers.
- Out-of-Range Values: Providing an invalid ASCII value (less than 0 or greater than 255) can lead to undefined behaviors in your program.
- Character Encodings: Misunderstanding the difference between ASCII and other encodings such as UTF-8 could lead to subtle bugs when handling strings.
Troubleshooting Tips
To avoid these issues, consider the following tips:
- Always double-check your type casts when converting between characters and their ASCII values.
- Familiarize yourself with the ranges of values for the character types you are using. This knowledge will help you avoid out-of-range errors.
- Utilize debugging tools to step through your code, particularly when handling character data, to ensure that values are what you expect them to be.
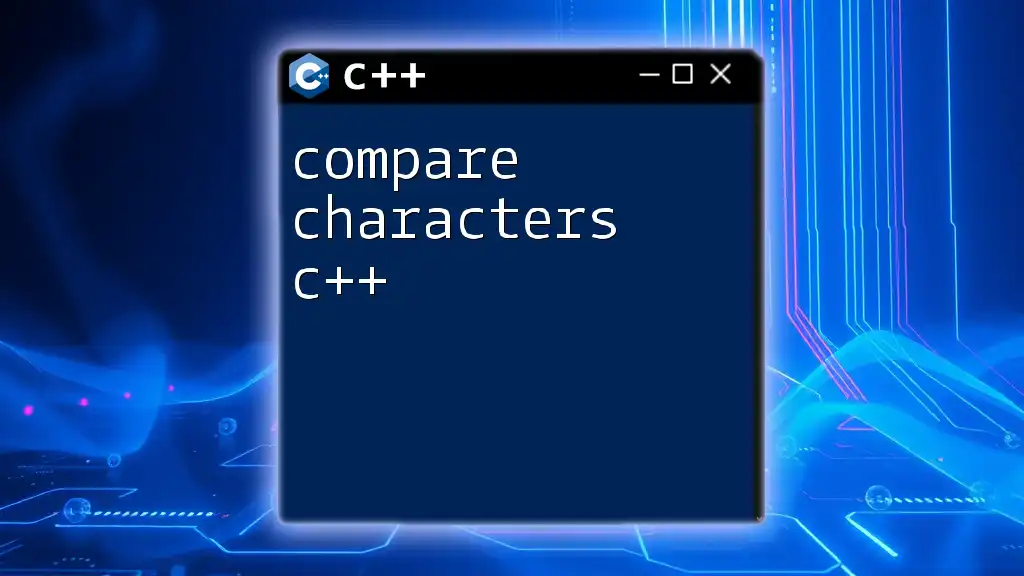
Summary
In summary, understanding how to perform character to ASCII conversions in C++ can greatly enhance your programming skills. From basic data handling to more complex text processing applications, mastering these operations is essential. Practice these concepts regularly to solidify your understanding and improve your efficiency as a developer.
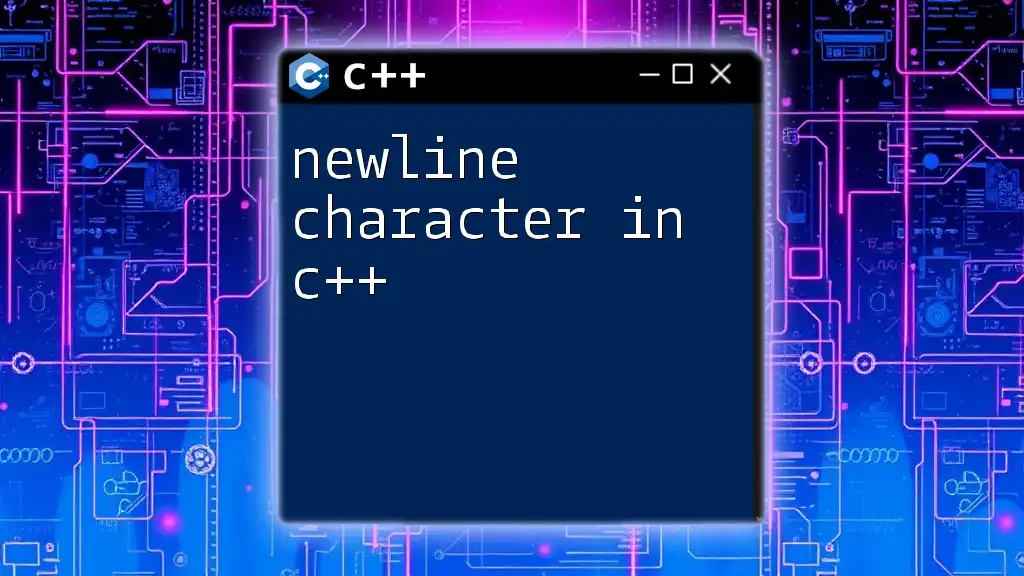
FAQs
What are the ASCII values for lowercase and uppercase letters?
The ASCII values for letters are as follows:
- A-Z: 65-90 (uppercase)
- a-z: 97-122 (lowercase)
Can ASCII values represent special characters?
Yes, ASCII values also represent special characters, including punctuation marks and control characters. For example, the ASCII value for the exclamation mark (!) is 33 and for a newline character, it is 10.
How to find ASCII value in other programming languages?
Most programming languages have similar functionality to C++ for working with ASCII values. For instance, in Python, you can use the `ord()` function to get the ASCII value of a character (e.g., `ord('A')` will return 65), and in Java, you can cast a char to an int.
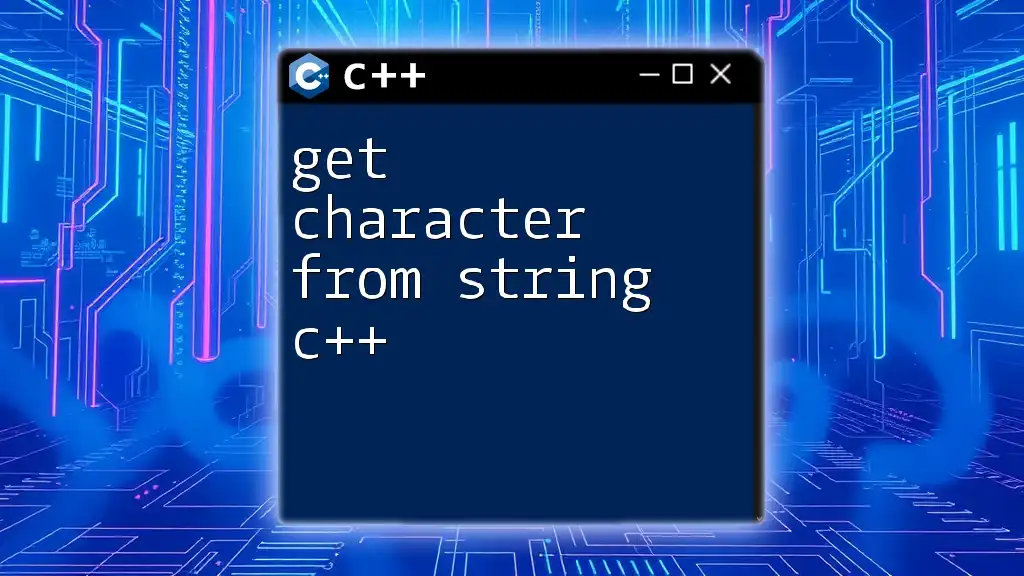
Conclusion
We have covered the critical aspects of converting characters to ASCII values in C++. By leveraging these techniques and principles, you can enhance your programming capabilities and tackle a variety of tasks involving character manipulation. Consider enrolling in our course to explore more about using C++ commands effectively!