The `emplace_back` function in C++ allows you to construct and add an element directly to the end of a `std::vector`, optimizing for efficiency by avoiding unnecessary copies.
#include <iostream>
#include <vector>
struct Point {
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
int main() {
std::vector<Point> points;
points.emplace_back(1, 2); // Directly constructs a Point at the end of the vector.
std::cout << "Point: (" << points.back().x << ", " << points.back().y << ")\n";
return 0;
}
Understanding the Basics
What is `std::vector`?
`std::vector` is a part of the C++ Standard Template Library (STL) and is one of the most widely used dynamic array implementations. Its features include dynamic sizing, contiguous memory allocation, and efficient element access. `std::vector` handles memory management for you, automatically resizing as needed, making it a versatile option for various programming tasks, such as storing collections of objects, managing dynamic datasets, or simplifying memory allocation.
Overview of `push_back`
`push_back` is a member function of `std::vector` used to add an element to the end of the vector. It takes a single object as an argument and makes a copy or moves that object into the container.
Here's a simple example of using `push_back`:
std::vector<int> numbers;
numbers.push_back(5);
numbers.push_back(10);
While `push_back` is simple and effective, it can lead to performance issues when many objects are being added because it often requires making copies. This behavior can be inefficient, particularly with larger objects, as it may interrupt the program flow due to the overhead of copying.
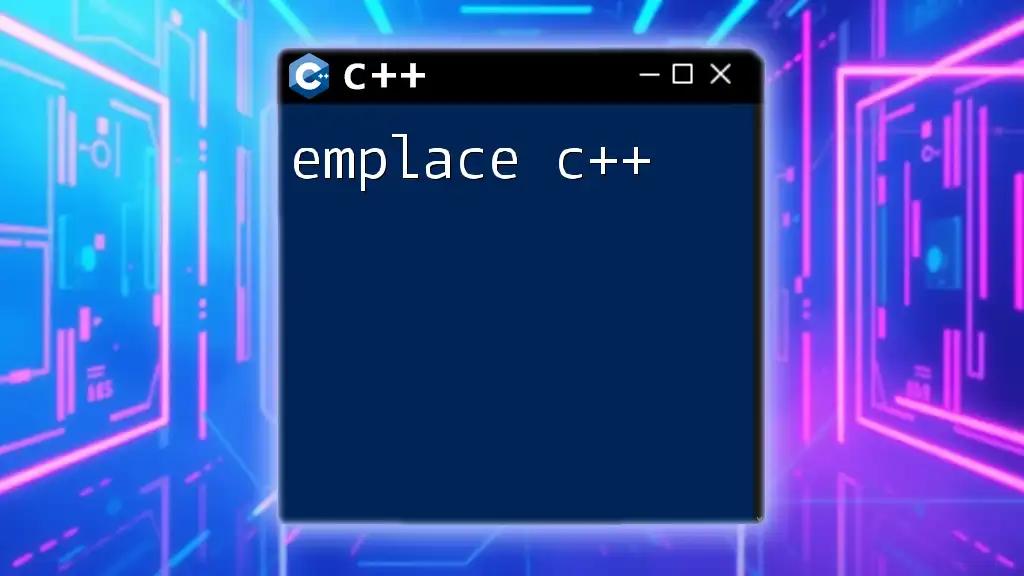
The Functionality of `emplace_back`
Syntax of `emplace_back`
The syntax for `emplace_back` is straightforward. It allows adding a new element to the end of a vector while constructing the element in place, avoiding unnecessary copies:
container.emplace_back(args...);
How `emplace_back` Works
In `emplace_back`, the parameters you provide are forwarded directly to the constructor of the contained type, which means that the element is constructed in the vector's memory rather than being constructed elsewhere and then copied/moved. This leads to more efficient memory usage and potentially improved performance.
When compared to `push_back`, `emplace_back` reduces the overhead associated with copying or moving the object into the vector. In essence, `emplace_back` is a front-end for constructing objects in the right place.
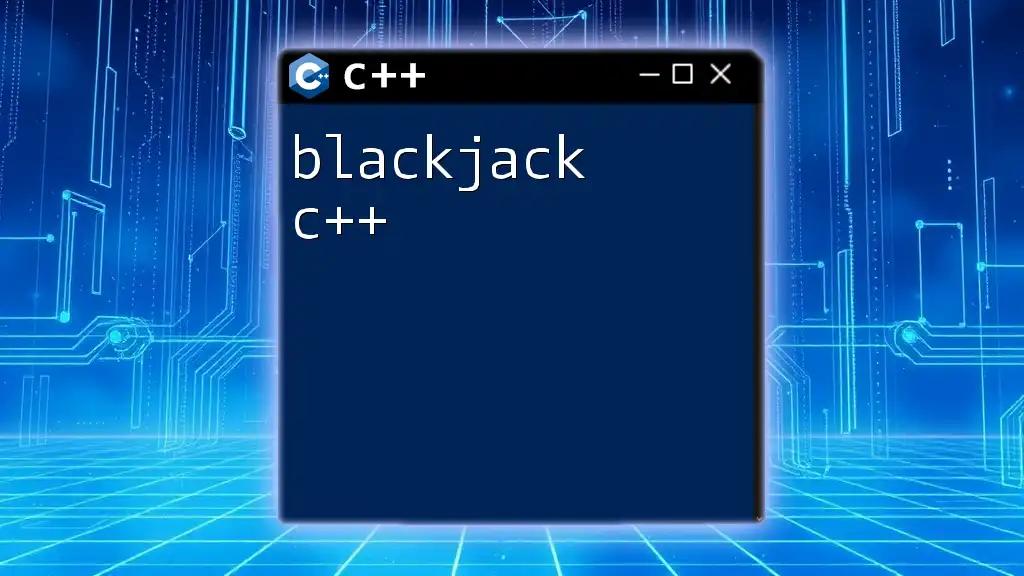
Benefits of Using `emplace_back`
Improved Performance
One of the largest benefits of `emplace_back` is performance enhancement. By constructing the element directly in the container, you eliminate the extra copy or move operations required by `push_back`. This becomes particularly evident when working with large objects.
Example: Consider this structure representing a two-dimensional point:
struct Point {
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
Using `emplace_back`, you can directly create a `Point` object in the vector without making a temporary copy:
std::vector<Point> points;
points.emplace_back(1, 2); // constructs Point(1, 2) directly in the vector
Cleaner and More Efficient Code
Using `emplace_back` not only results in better performance but also promotes cleaner and more efficient code. When you construct objects directly inside the vector, your code becomes more concise.
Example of cleaner code with `emplace_back`:
class Person {
public:
std::string name;
int age;
Person(std::string name, int age) : name(name), age(age) {}
};
std::vector<Person> people;
people.emplace_back("Alice", 30);
people.emplace_back("Bob", 25);
This usage is not only more efficient but also easier to read and maintain.
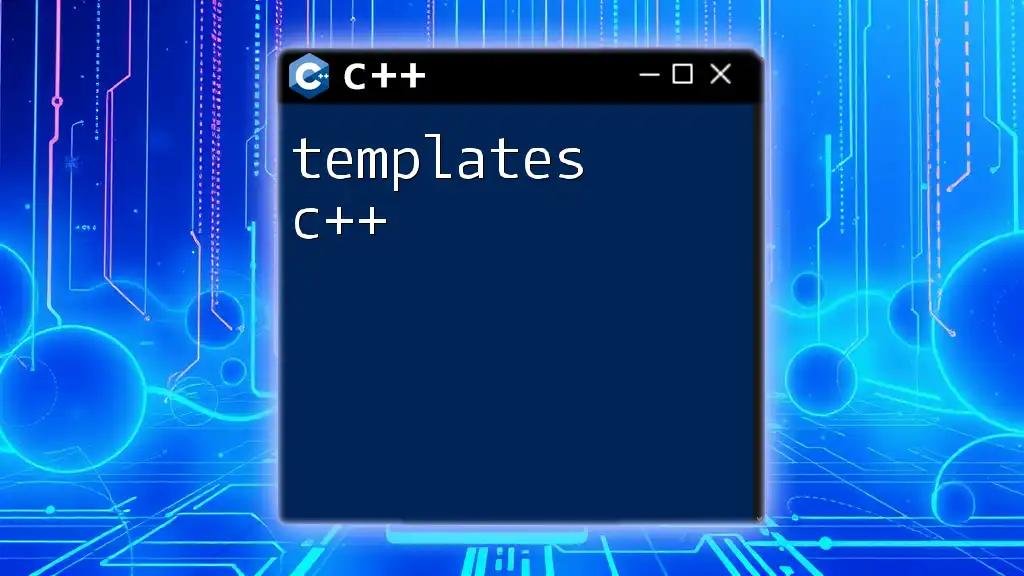
Practical Examples
Example 1: Storing User-Defined Types
Using `emplace_back` with user-defined types can drastically improve efficiency and readability. The `Person` class example demonstrates how simple it is to create objects directly inside the vector.
class Person {
public:
std::string name;
int age;
Person(std::string name, int age) : name(name), age(age) {}
};
std::vector<Person> people;
people.emplace_back("Alice", 30);
people.emplace_back("Bob", 25);
Example 2: Using `emplace_back` with Standard Library Types
`emplace_back` can also be effectively used with STL types. For instance, consider using `std::pair` to store key-value pairs within a vector:
std::vector<std::pair<int, std::string>> pairs;
pairs.emplace_back(1, "One");
pairs.emplace_back(2, "Two");
This showcases how `emplace_back` streamlines the code structure by constructing the pairs directly within the vector.
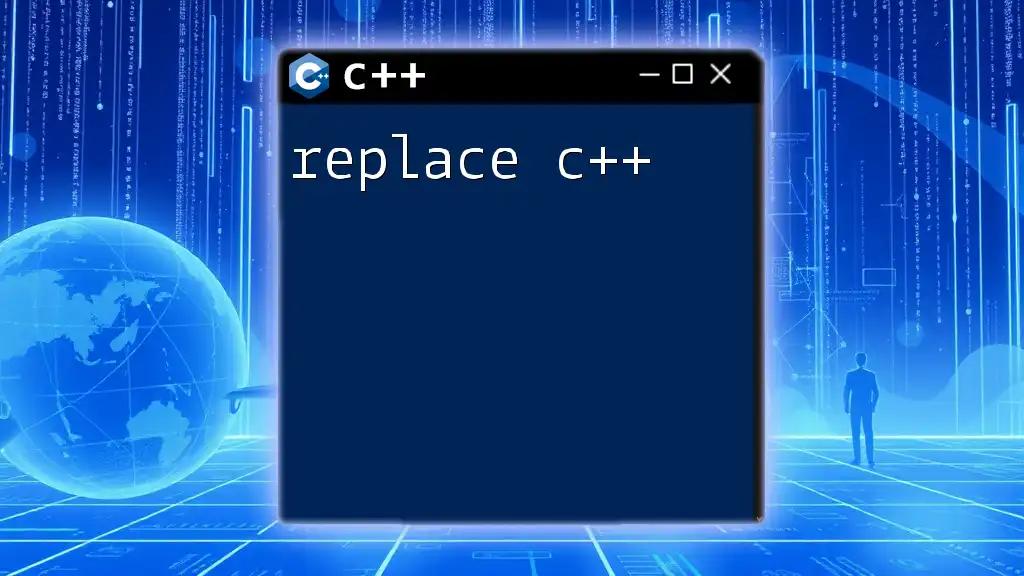
Potential Pitfalls
When to Avoid `emplace_back`
Despite its advantages, there are circumstances where using `emplace_back` is not recommended. If your object is of a type that cannot be moved or copied (e.g., a unique pointer with exclusive ownership), using `emplace_back` can result in compilation errors.
Common Mistakes
Many developers mistakenly believe that `emplace_back` will always provide better performance. It’s crucial to understand your use case; if your vector's contents are being managed through references or pointers, the benefits of `emplace_back` may diminish. Always evaluate the performance in the context of the overall application.
Example of improper use leading to errors:
std::vector<std::unique_ptr<MyClass>> myVector;
myVector.emplace_back(std::make_unique<MyClass>()); // Correct: Can be used
myVector.emplace_back(MyClass()); // Error: Cannot copy, causes issues
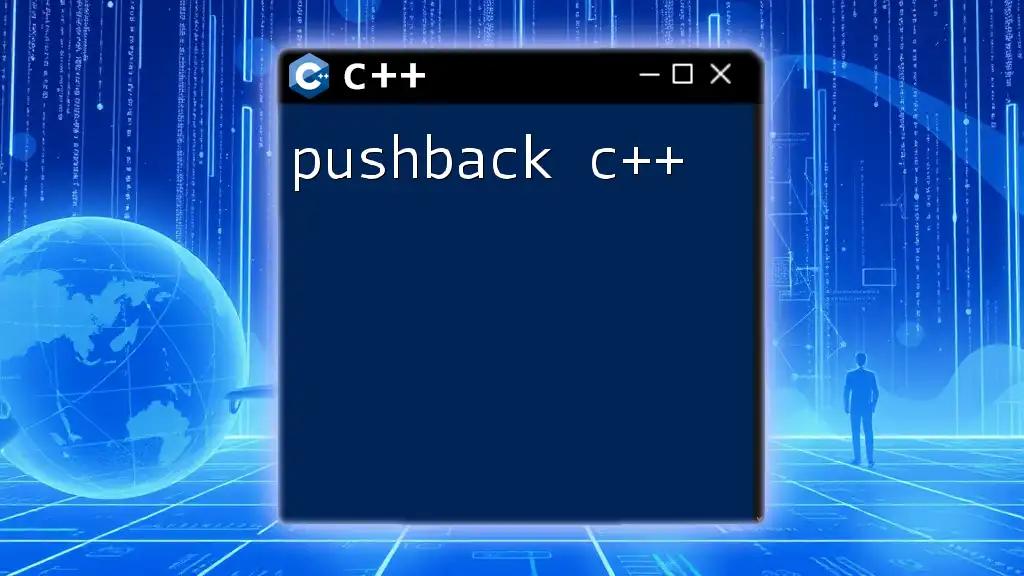
Conclusion
In summary, `emplace_back c++` is a powerful tool that allows developers to construct objects directly in a vector, significantly enhancing performance and code readability. The efficiency gained from eliminating unnecessary copies can lead to improved application runtime, especially in performance-sensitive applications.
As you continue your journey in mastering modern C++, incorporate `emplace_back` into your methodology and experience the benefits it brings. Embrace this feature, experiment with various types, and watch how it can clean up your code and improve efficiency in your projects.
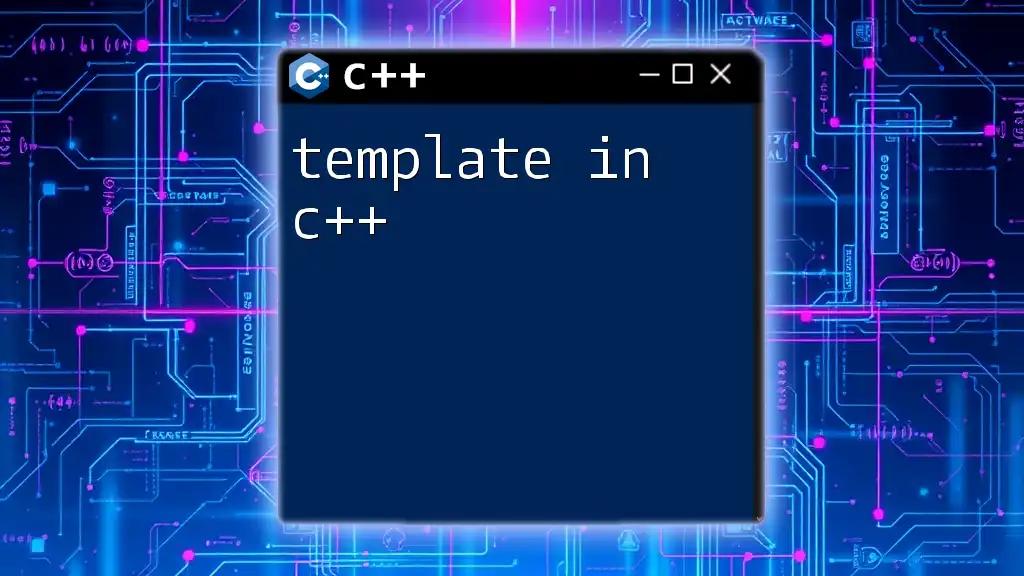
Additional Resources
To further enhance your understanding and skills, consider exploring advanced C++ features and best practices through the following resources:
- Online C++ tutorials and courses
- Recommended readings on modern C++ design patterns
- Community forums where you can engage in discussions about C++ topics and best practices

FAQs
What is the difference between `emplace_back` and `push_back`?
`emplace_back` constructs an object in-place within the vector, while `push_back` makes a copy of an existing object.
Can `emplace_back` be used with different STL containers?
Yes, `emplace_back` is typically applicable to many standard containers like `std::list` and `std::deque`, though implementation details may vary.
Is `emplace_back` safe with multithreading?
Using `emplace_back` in a multithreading context requires proper synchronization (e.g., mutex locks) to prevent data races when multiple threads access the same vector.