The `emplace_back` function in C++ adds a new element to the end of a vector by constructing the element in place, which can improve performance by eliminating the need for a temporary object.
#include <vector>
#include <string>
#include <iostream>
int main() {
std::vector<std::string> names;
names.emplace_back("Alice"); // Constructs "Alice" directly in the vector
names.emplace_back("Bob");
for (const auto& name : names) {
std::cout << name << std::endl;
}
return 0;
}
What is emplace_back?
Definition of emplace_back
The `emplace_back` function in C++ is a member function of the Standard Template Library (STL) containers, such as `std::vector`. It allows you to add elements to the end of a vector while constructing them in place. This means that instead of creating an object separately and then copying or moving it into the vector, `emplace_back` constructs the object directly in the vector's allocated space. This can lead to performance improvements as it eliminates the need for extra copies or moves.
Key Advantages of using emplace_back
-
Performance: One of the main benefits of `emplace_back` is its performance advantage, especially when passing complex or large objects. `emplace_back` avoids copying or moving by constructing the object in place, which saves time and resources.
-
Construction in-place: Since `emplace_back` constructs the object where it's positioned in the vector, it can lead to better memory management and potentially reduced overhead.
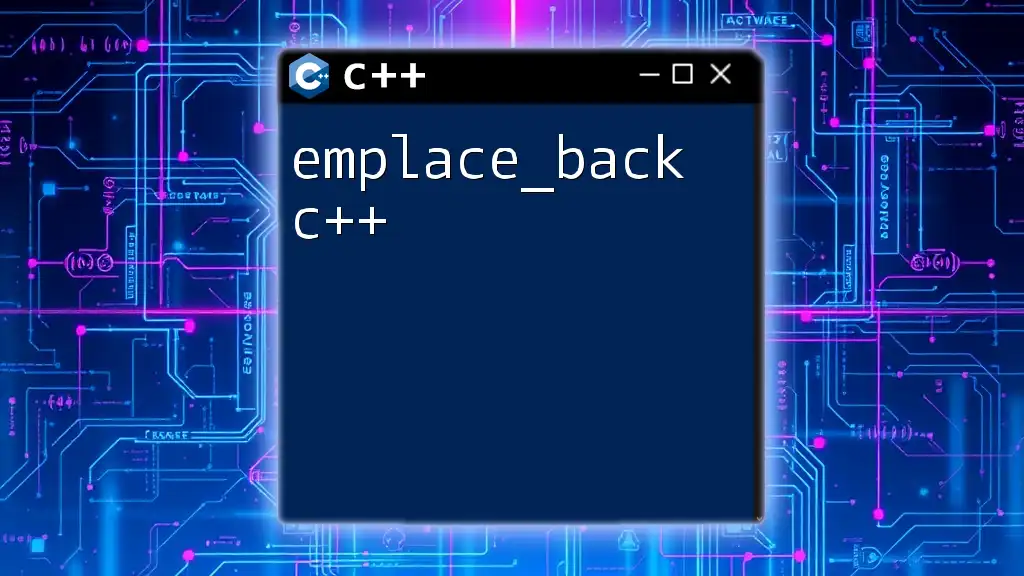
How to Use emplace_back
Syntax of emplace_back
The basic syntax of `emplace_back` is straightforward and looks like this:
vector<Type> vec;
vec.emplace_back(args);
Step-by-Step Guide
Step 1: Include necessary libraries
To work with vectors, you must include the corresponding header file at the beginning of your C++ program:
#include <vector>
Step 2: Define your vector
Define a `std::vector` that will hold your objects. For instance, if you're working with a custom class named `MyClass`, you can declare the vector as follows:
std::vector<MyClass> myVector;
Step 3: Use emplace_back to add an element
You can now use `emplace_back` to add elements directly into the vector:
myVector.emplace_back(arg1, arg2);
In this code, `arg1` and `arg2` are the arguments that will be passed to the constructor of `MyClass`, thus creating the object directly within the vector's storage.
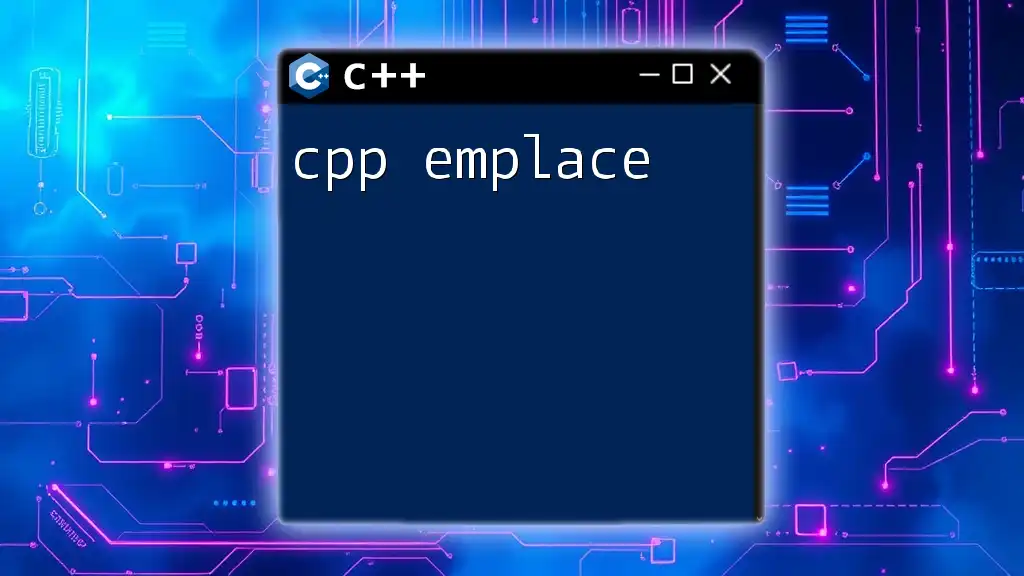
Practical Example: Using emplace_back in Real-World Situations
Defining a Custom Class
Let's consider a simple class named `Point` designed to represent a point in a 2D space:
class Point {
public:
Point(int x, int y) : x(x), y(y) {}
int x, y;
};
Using emplace_back with the Custom Class
Now, let's create a vector of `Point` objects and add some points using `emplace_back`:
std::vector<Point> points;
points.emplace_back(1, 2);
points.emplace_back(3, 4);
In the example above, two `Point` objects are being constructed directly in the `points` vector. When `emplace_back` is called, it calls the constructor of `Point` with the given arguments right where the space is allocated in the vector. This leads to efficient memory usage since there are no unnecessary copies or moves.
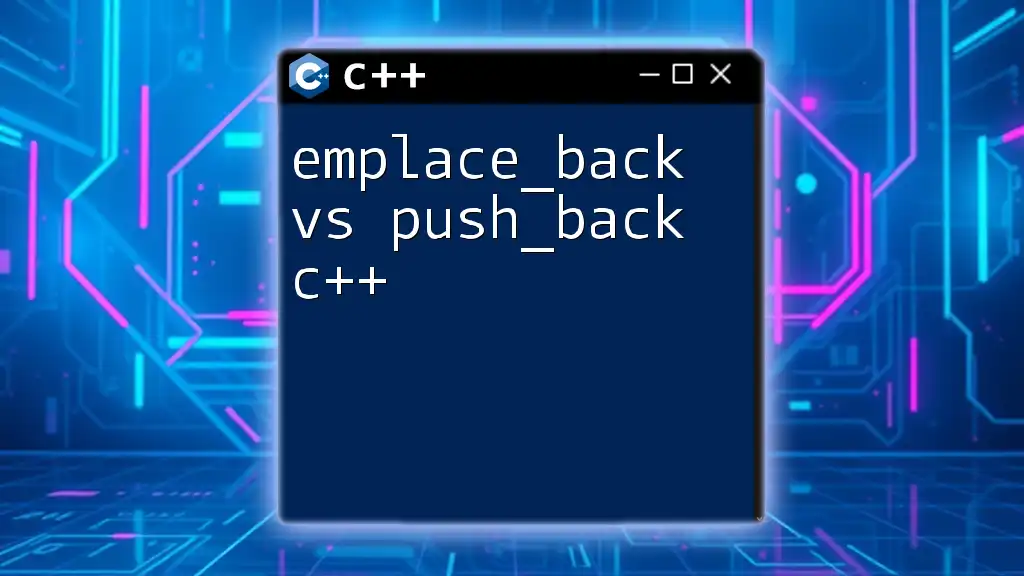
Differences Between emplace_back and push_back
Overview of push_back
The `push_back` function also adds elements to the end of a vector; however, it requires you to create the object beforehand.
myVector.push_back(MyClass(arg1, arg2));
In this case, a temporary object is created and then moved or copied into the vector. While `push_back` works perfectly in many scenarios, it incurs a bit more overhead than `emplace_back` when dealing with large or complex objects.
Memory and Performance Implications
Using `emplace_back` allows you to minimize memory fragmentation and reduce the overall number of object copies. This can significantly boost performance, especially when your vector holds non-trivial objects that require non-trivial constructors or destructors.
For example, in performance-critical applications, such as graphics or real-time systems, choosing `emplace_back` over `push_back` can lead to smoother execution and lower latency.
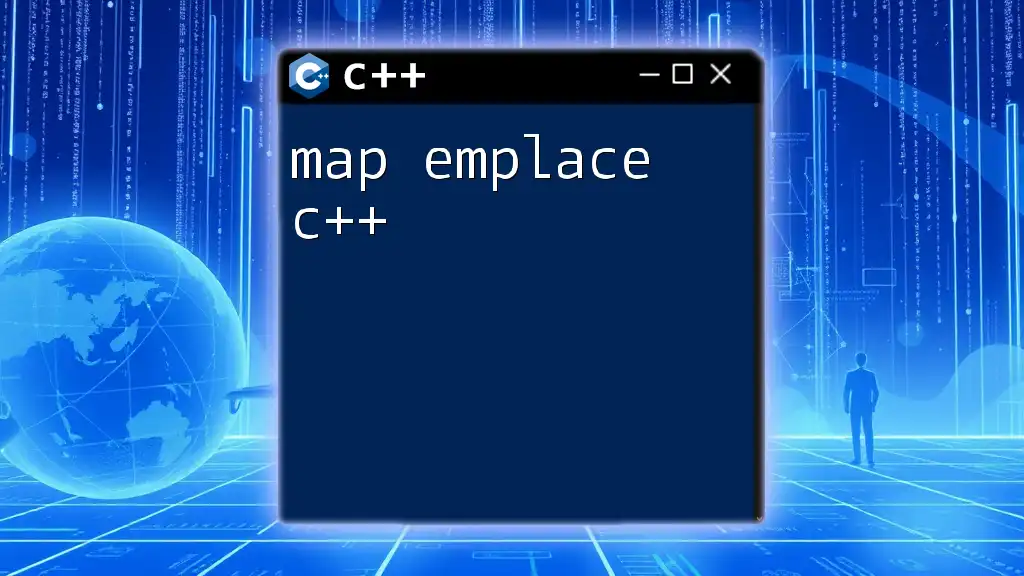
Best Practices for Using emplace_back
When to Use emplace_back
Use `emplace_back` when:
- You are creating objects that have non-trivial constructors (i.e., constructors that initialize resources).
- You want to improve performance by minimizing copies.
- You prefer simpler syntax, as you don’t have to create temporary objects in advance.
Avoid the Pitfalls
Avoid common mistakes such as:
- Not passing the right arguments corresponding to the constructor of the element type. If your arguments are mismatched, you may face compilation errors.
- Trying to call `emplace_back` with an object type that cannot be constructed in-place due to missing constructors.
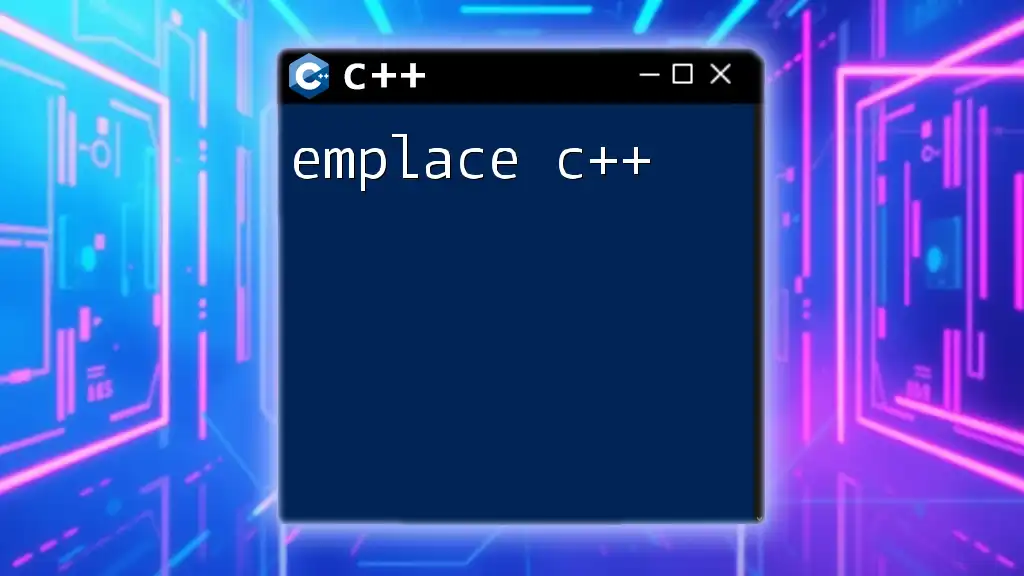
Conclusion
In conclusion, understanding how to use cpp emplace_back effectively is critical for optimizing your C++ code. It not only enhances performance by constructing objects in place but also minimizes overhead related to object copying. As you work on your C++ projects, consider incorporating `emplace_back` in scenarios where its advantages shine. Mastering this can lead to more efficient and cleaner code, especially in complex applications.
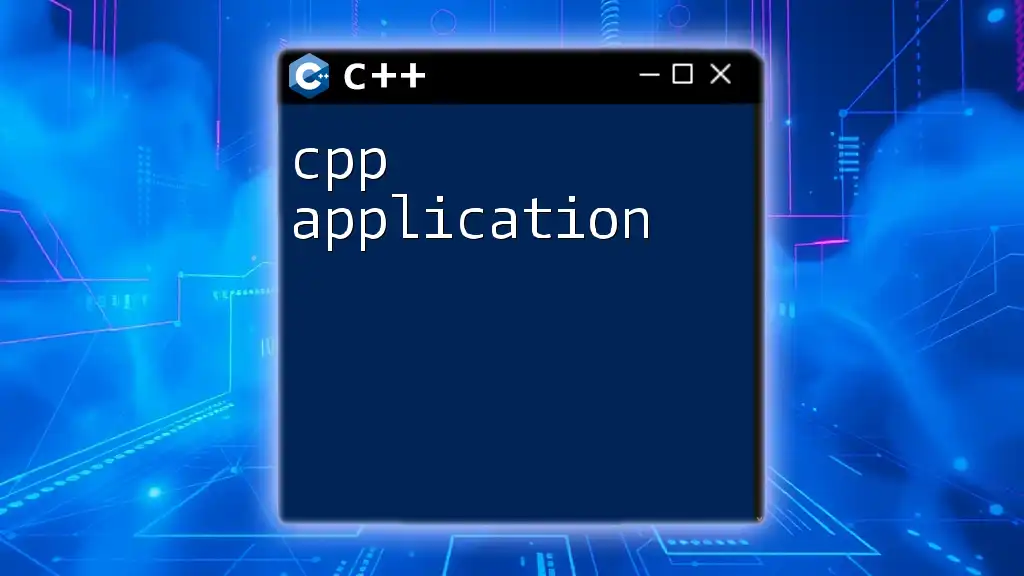
FAQs about emplace_back
What type of container supports emplace_back?
`emplace_back` is primarily used with sequence containers like `std::vector`, `std::deque`, and `std::list`. These containers allow you to construct elements directly in the location where they will reside, which is key to leveraging `emplace_back` effectively.
How does emplace_back handle move semantics?
When you use `emplace_back`, it constructs the object in the target location within the vector. Move semantics will come into play if you pass a movable type as an argument, thereby transferring ownership of resources seamlessly without copying.
Can emplace_back throw exceptions?
Yes, `emplace_back` can throw exceptions if the constructor of the object being created throws. It is essential to handle exceptions gracefully in your code when working with `emplace_back` to ensure your program remains robust. Overall, it is imperative to understand the nuances of using `emplace_back` to ensure optimal results in your C++ programming endeavors.