In C++, the `explicit` keyword is used to prevent implicit conversions for constructors and conversion operators, ensuring that objects are constructed only in explicit ways to avoid unintended behavior.
Here's a code snippet demonstrating its use:
class MyClass {
public:
explicit MyClass(int x) {
// Constructor that prevents implicit conversion
}
};
void func(MyClass obj) {
// Function that takes MyClass as parameter
}
int main() {
MyClass obj(5); // OK: explicit constructor called
// MyClass obj2 = 10; // Error: cannot convert from int to MyClass due to explicit keyword
func(obj); // OK: passing MyClass object
return 0;
}
Understanding the Explicit Keyword in C++
Definition of Explicit
The explicit keyword in C++ serves as a modifier to constructors or conversion operators. It is primarily used to prevent implicit conversions, which can lead to unintentional and often subtle bugs in your code. In simpler terms, an explicit constructor can only be invoked by the compiler if the correct type is explicitly specified.
By marking a constructor as explicit, you assure that the compiler will not attempt to convert types automatically, thus enforcing stronger type safety.
The Role of Explicit in C++
Utilizing the explicit keyword enhances the clarity of your code. When constructors are marked explicit, it is evident to anyone reading the code that certain conversions require a deliberate statement from the programmer.
For instance, when you see an explicit constructor, you understand that passing an argument of a different type is likely to cause a compilation error, prompting the developer to think carefully about their type usage.
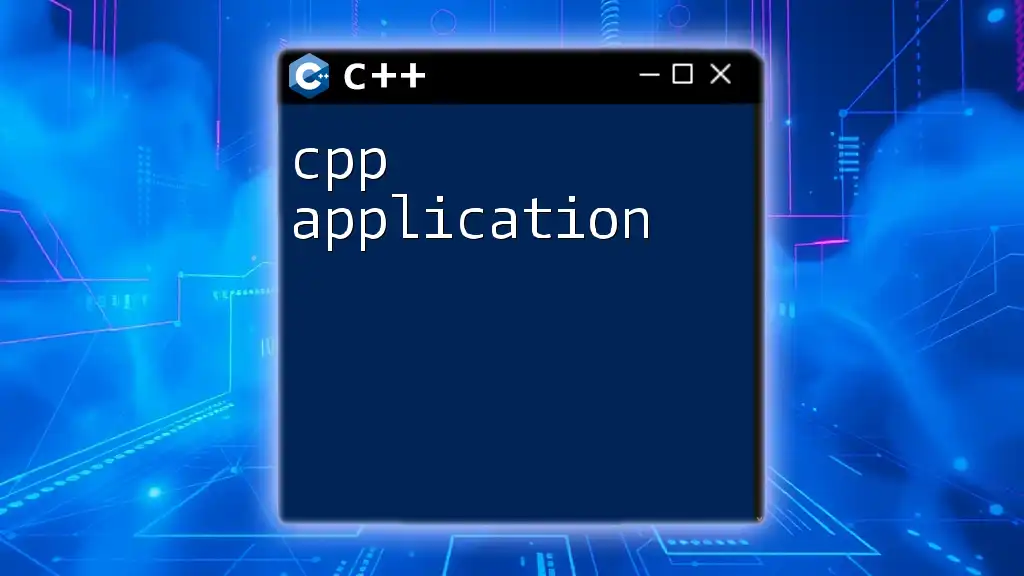
C++ Explicit Constructor
What is an Explicit Constructor?
An explicit constructor is a constructor that is marked with the explicit keyword. This designation prevents implicit conversions, meaning the constructor won't be called automatically for type conversions.
For example, consider a situation where an object of type `MyClass` is expected:
class MyClass {
public:
explicit MyClass(int x);
};
In this scenario, if you try to create `MyClass` using a simple integer without explicitly defining it as a `MyClass` object, it will trigger a compile-time error.
Syntax of an Explicit Constructor
Defining an explicit constructor follows a straightforward syntax pattern. Here’s a basic example:
class ClassName {
public:
explicit ClassName(int arg); // explicit constructor example
};
In this syntax, `ClassName` is a user-defined class and `arg` is an integer parameter. The `explicit` keyword precedes the constructor declaration, indicating that any attempt to convert from `int` to `ClassName` must be made explicitly.
Real-World Examples of Explicit Constructors
Let’s further illustrate the workings of an explicit constructor with an example:
class MyClass {
public:
explicit MyClass(int x) {
// Constructor logic
}
};
void function(MyClass obj) {}
int main() {
MyClass obj1(10); // OK - explicitly passing an int
// MyClass obj2 = 20; // Error - no implicit conversion allowed
}
In this snippet, `obj1` is constructed correctly because `10` is explicitly passed as an argument. However, the line creating `obj2` results in a compile-time error because it attempts to implicitly convert the integer `20` into a `MyClass` object, a conversion not permitted by the explicit constructor.
Advantages of Using Explicit Constructors
The advantages of using explicit constructors cannot be overstated:
-
Prevention of Unnoticed Conversions: By employing the explicit keyword, you safeguard your code against surprises that come from implicit conversions, thereby reducing potential bugs.
-
Improved Code Maintainability: Code that is explicit in its requirements tends to be easier to maintain. When another developer reads the code, the explicitness of constructors clearly communicates how the types should be treated.
-
Error Minimization: This practice minimizes runtime errors by catching type issues during compile-time, allowing developers to address these problems sooner rather than later.
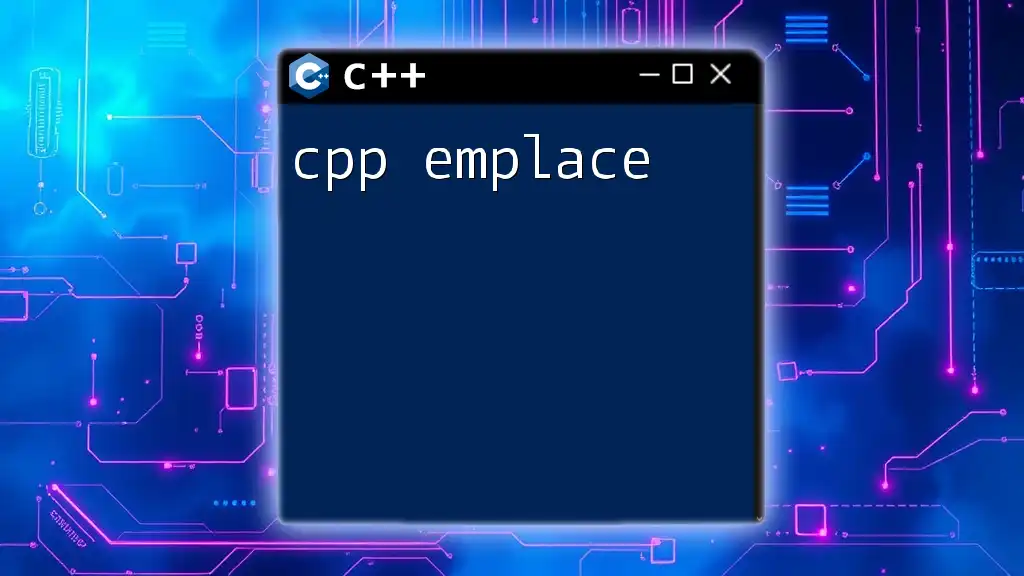
Explicit vs Implicit Conversions
What are Implicit Conversions?
Implicit conversions refer to automatic conversions that the compiler performs. If you have a constructor that is not marked as explicit, the compiler can use it to convert types for you, sometimes without your intention. This can lead to unexpected behavior in your program logic.
Comparing Explicit and Implicit Conversions
-
Explicit Conversions: Require a straightforward approach where the developer must actively convert types, ensuring they are aware of the conversion taking place.
-
Implicit Conversions: Automatically change one type to another, which can be beneficial in some scenarios but poses risks if the conversion is unintended.
Pros and cons:
- Explicit Conversion Pros: Increases code clarity and safety.
- Implicit Conversion Pros: Reduces verbosity and can streamline some coding tasks.
In effect, while implicit conversions can simplify certain syntaxes, explicit conversions enhance clarity and uphold type integrity; the choice often comes down to a question of prioritizing clarity over conciseness.
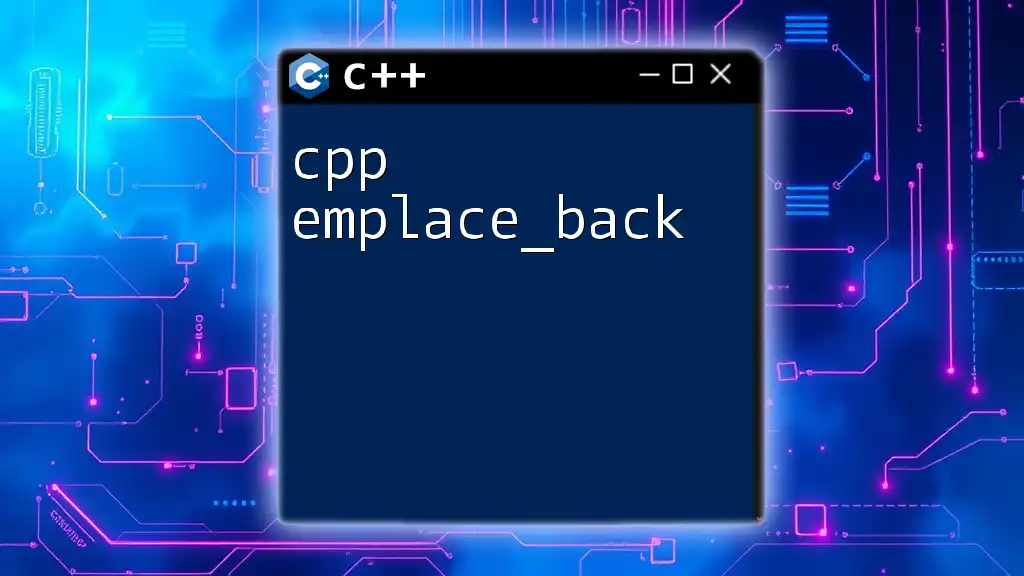
Best Practices for Using Explicit in C++
When to Use the Explicit Keyword in C++
Employing the explicit keyword is generally recommended under the following conditions:
- When creating single-argument constructors that can be invoked using a different type.
- For conversion operators to prevent automatic type changes that could lead to bugs.
It’s a good practice to review your classes and determine if marking any of your constructors as explicit would enhance code safety.
Testing and Debugging
To ensure your code does not inadvertently allow for unwanted implicit conversions, make use of the following techniques:
-
Unit Testing: Write unit tests to check for expected behavior and ensure that constructors are behaving as intended.
-
Static Analysis Tools: Utilize static analysis tools that can highlight potential implicit conversion issues within your code base.
These methods not only help maintain a clean code base but also promote a culture of vigilance towards type integrity.
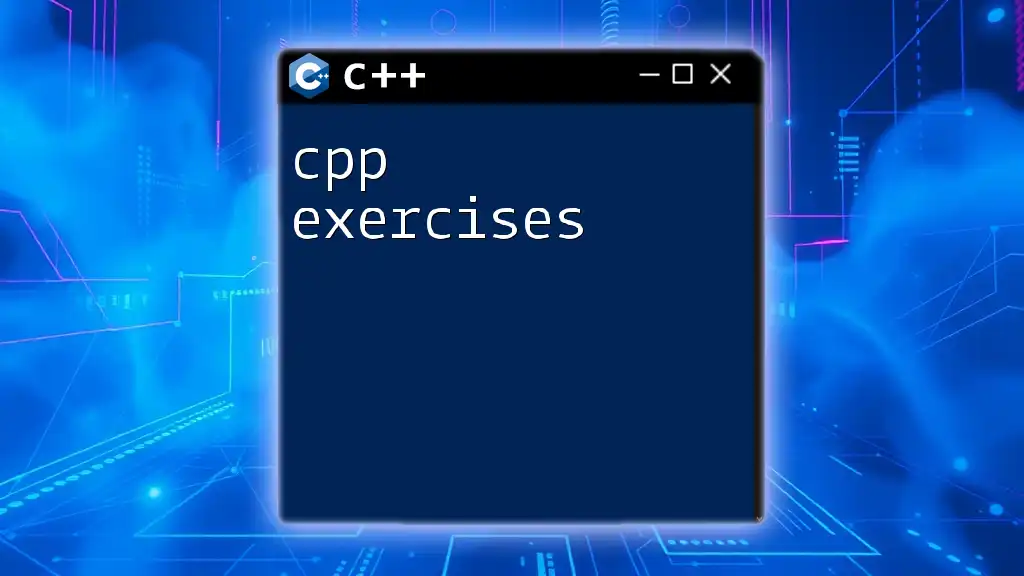
Conclusion
The cpp explicit keyword serves as a powerful tool in the C++ programmer’s arsenal. Through the use of explicit constructors, developers can enforce type safety, maintain the clarity of their code, and ultimately reduce the chances of encountering bugs caused by unintentional type conversions. Applying the explicit keyword where appropriate can reinforce strong coding practices, benefitting both individual developers and teams alike.
As you implement your newfound knowledge on explicit constructors and conversions, remember to embrace these techniques in your everyday coding tasks to enhance the performance and reliability of your C++ applications.