C++ application development involves creating software applications using the C++ programming language, leveraging its features for performance and efficiency in various types of projects, such as system software, game development, and real-time simulations.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++: The Basics
What is C++?
C++ is a powerful general-purpose programming language created by Bjarne Stroustrup in the late 1970s. It is an extension of the C programming language, combining the efficiency of C with the advanced features of object-oriented programming (OOP). C++ is widely used in application development across various domains, including system software, game development, and high-performance applications. Key features of C++ include:
- Object-Oriented Programming: Enables you to represent real-world entities as objects, making your code more modular and reusable.
- Templates: Allow for generic programming, which enables the writing of code that works with any data type.
- Standard Template Library (STL): A powerful library of generic classes and functions that provides ready-to-use solutions for common tasks like sorting and searching.
Setting Up the C++ Environment
Choosing an IDE
An Integrated Development Environment (IDE) is crucial for facilitating effective coding. Some popular IDEs for C++ include:
- Visual Studio: Known for its robust features, making it suitable for large-scale applications. However, it is Windows-specific.
- Code::Blocks: A lightweight, open-source option, great for beginners.
- CLion: Offers advanced support for CMake and is cross-platform, but is a paid option.
Installing C++ Compiler
To effectively write and compile C++ code, you need a C++ compiler. Here’s a step-by-step guide to installing GCC (GNU Compiler Collection):
- Install GCC:
- On Windows, you can use MinGW-w64 or Cygwin.
- On macOS, install Xcode Command Line Tools.
- On Linux, use the package manager to install GCC.
- Verify Installation:
Open your command line interface and type:
This should display the installed version of the compiler.g++ --version
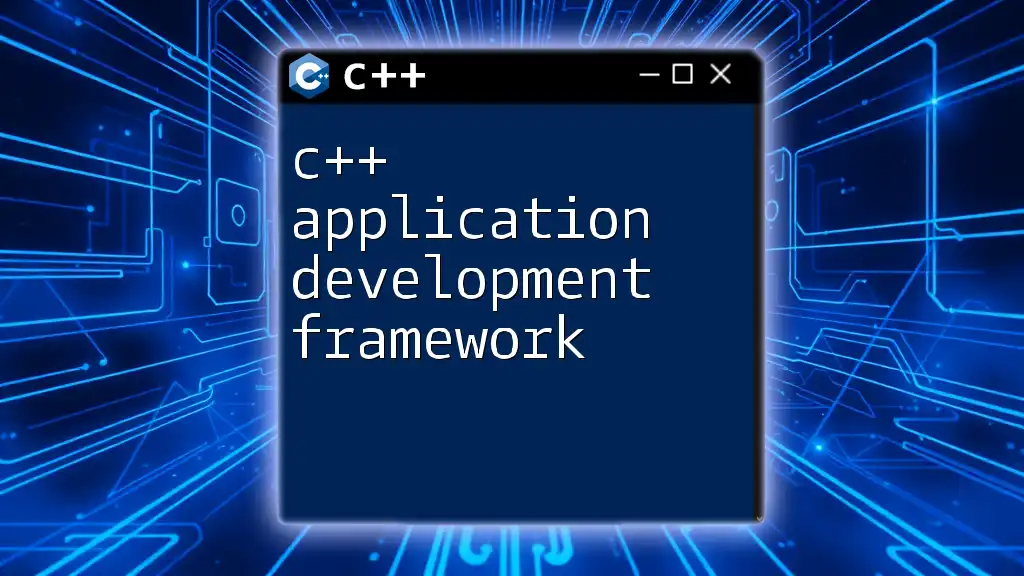
Core Concepts of C++ Application Development
Data Types and Variables
Understanding data types is fundamental to C++ application development. C++ supports various primitive data types, such as:
- `int`: For integers (e.g., `int age = 25;`)
- `float`: For floating-point numbers (e.g., `float height = 5.9;`)
- `char`: For single characters.
C++ also supports complex data types, including arrays and structures. One important distinction to understand is between static and dynamic typing, which impacts how variables are used and memory is managed.
Control Structures
Control structures allow for decision-making and looping in your application.
Conditional Statements
These allow your program to execute certain blocks of code based on specific conditions. Here is a simple `if` statement example:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
Loops
Loops enable you to repeat actions without resorting to redundant code. Consider the following `for` loop example:
for(int i = 0; i < 5; i++) {
cout << i << endl;
}
This loop prints numbers from 0 to 4 and demonstrates C++’s syntax for iterating through code.
Functions and Methods
Functions are fundamental in C++. They allow for code reusability and modular design. Here’s how to define a function and demonstrate overloading:
int add(int a, int b) {
return a + b;
}
You can call this function with different sets of integers, and C++ can resolve which version of the function to call based on the parameters passed.
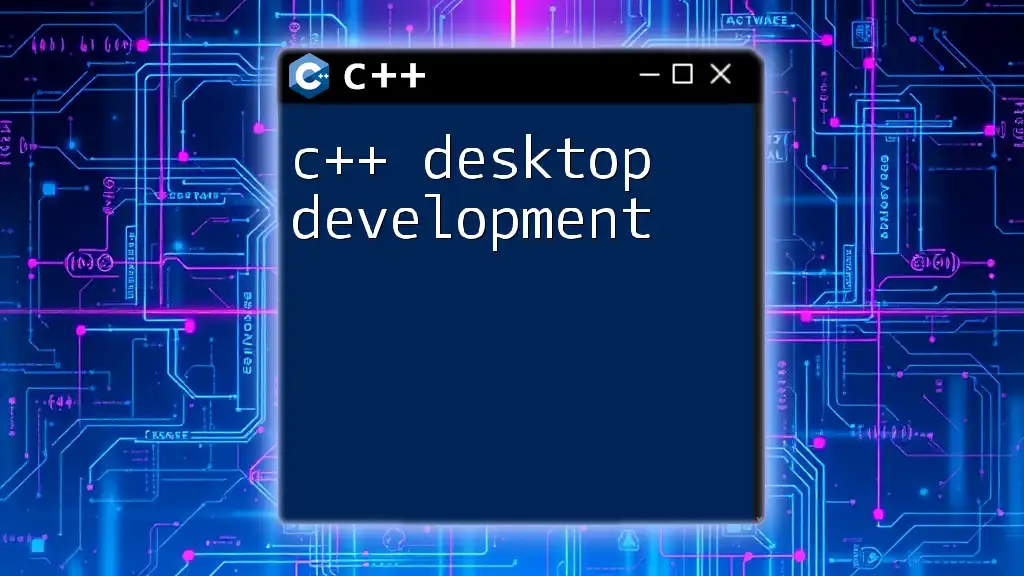
Object-Oriented Programming in C++
Classes and Objects
C++ is widely recognized for its robust OOP capabilities. A class in C++ is a blueprint for creating objects. Here's a basic example:
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
In this example, `Dog` serves as a class, and you can create multiple instances (objects) of `Dog`, each capable of executing the `bark` method.
Inheritance and Polymorphism
C++ supports inheritance, allowing you to create a new class based on an existing class, enhancing code reusability. For example:
class Animal {
public:
virtual void speak() {
cout << "Animal speaks";
}
};
class Cat : public Animal {
public:
void speak() override {
cout << "Meow";
}
};
In this case, `Cat` inherits from `Animal` and can override the `speak` method, showcasing polymorphism—a powerful feature that allows one interface to serve multiple forms.
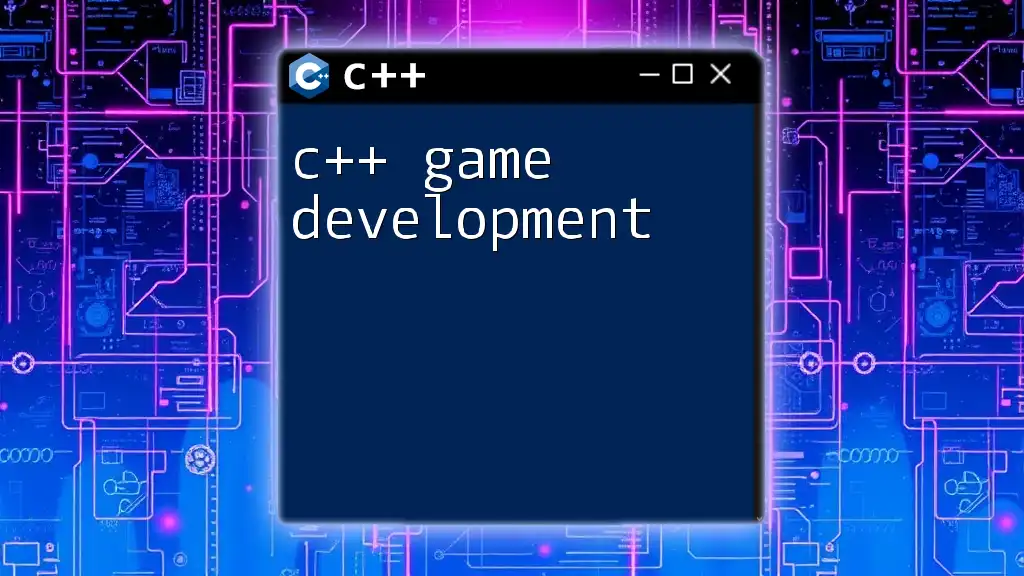
Advanced C++ Features
Templates and Generics
Templates in C++ enhance flexibility and reusability. By utilizing templates, you can write functions that work with any data type without rewriting code. Here’s a simple function template example:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function can now be used with any data type, whether `int`, `float`, or even custom data types.
Standard Template Library (STL)
The Standard Template Library (STL) is a significant feature in C++, offering numerous built-in data structures and algorithms. Key components of STL include:
- Vectors: Dynamic arrays that can resize as needed.
- Lists: Doubly-linked lists.
- Maps: Associative arrays that store key-value pairs.
Here’s an example illustrating the use of STL:
#include <vector>
#include <algorithm>
std::vector<int> numbers = {1, 3, 2, 5, 4};
std::sort(numbers.begin(), numbers.end());
This code snippet showcases a simple array of integers that gets sorted using STL's `sort` function.
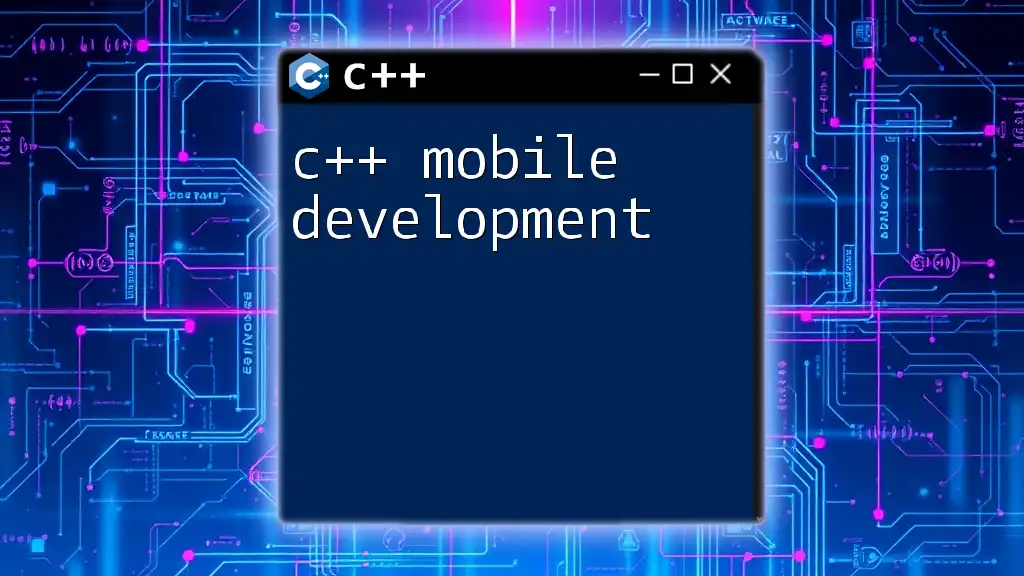
Building a Simple C++ Application
Project Structure
Organizing your project effectively is essential. A well-structured C++ project might include directories like:
- `src`: For source files.
- `include`: For headers.
- `bin`: For compiled applications.
- `tests`: For test cases.
Code Organization and Modularization
Keep your code modular to enhance maintainability. Create separate files for classes and their implementations, following the principle of separation of concerns.
For example, a header file might look like:
// Dog.h
class Dog {
public:
void bark();
};
And the implementation file:
// Dog.cpp
#include "Dog.h"
#include <iostream>
void Dog::bark() {
std::cout << "Woof!" << std::endl;
}
Debugging and Testing
Debugging is crucial in C++ application development. Utilize tools such as gdb for debugging, and apply logging techniques to help trace issues. Furthermore, leveraging unit testing frameworks like Google Test can help ensure your code remains robust. Here’s a simple test case:
TEST(MyTestSuite, MyTest) {
ASSERT_EQ(1, 1);
}
This demonstrates how you can write tests to validate your code's functionalities.
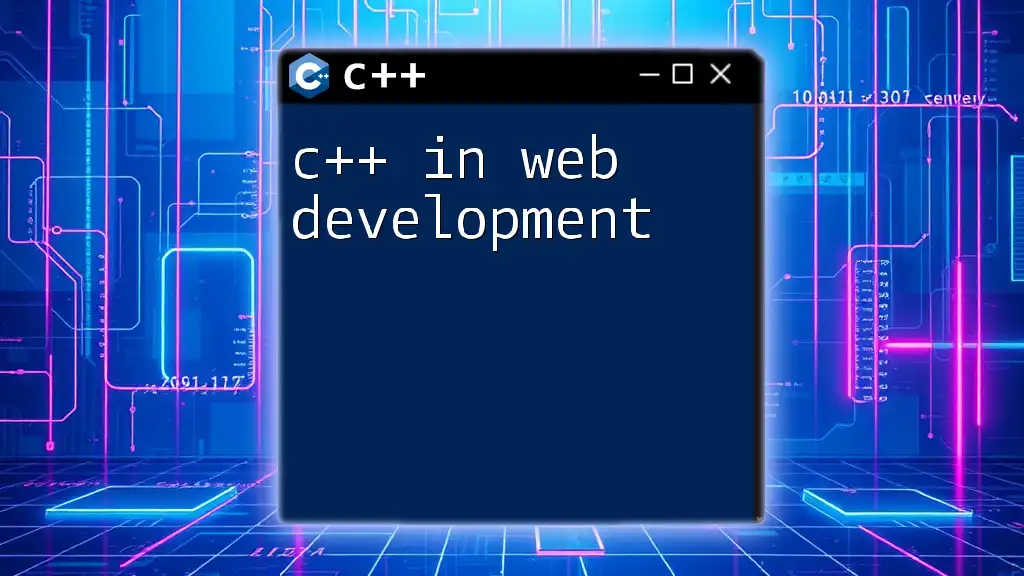
Deployment and Maintenance
Compilation and Build Process
The compilation process converts your C++ code into an executable form. You can use Makefiles or CMake to automate this process. Here’s a simple `CMakeLists.txt` example:
cmake_minimum_required(VERSION 3.10)
project(MyCPlusPlusApp)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyApp main.cpp)
Best Practices for Maintenance
Maintainability is vital in software development. Always document your code to ensure other developers (or your future self) can understand it. Version control tools like Git are indispensable; mastering basic commands (like `git clone`, `git commit`, and `git push`) can significantly streamline your workflow.
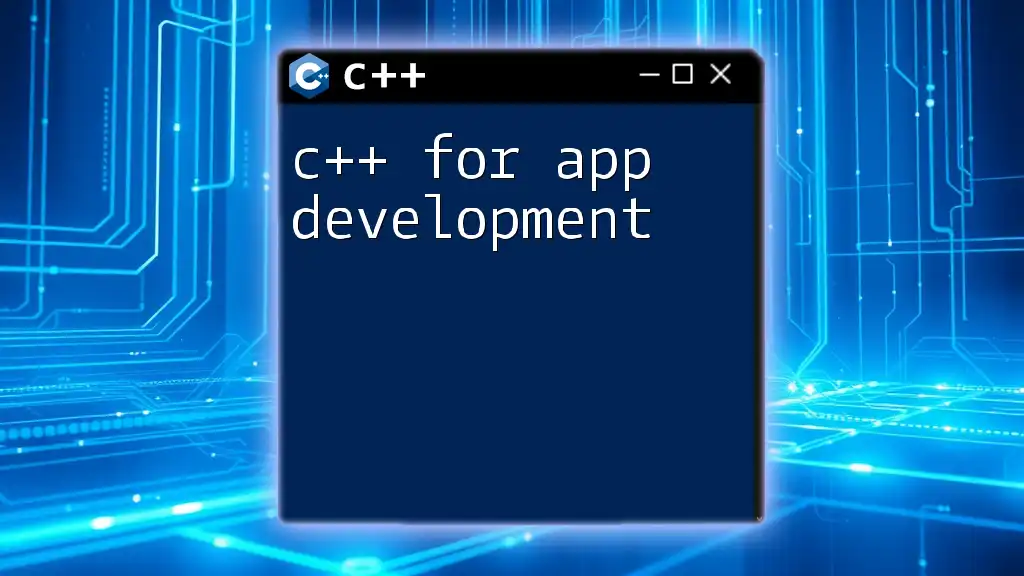
Conclusion
C++ application development is a multifaceted discipline requiring an understanding of core programming concepts, advanced features, and best practices. By mastering these elements, you can fortify your skill set and build high-quality applications. As you continue to immerse yourself in the world of C++, consider exploring more advanced topics, engaging in community forums, or enrolling in specialized courses.
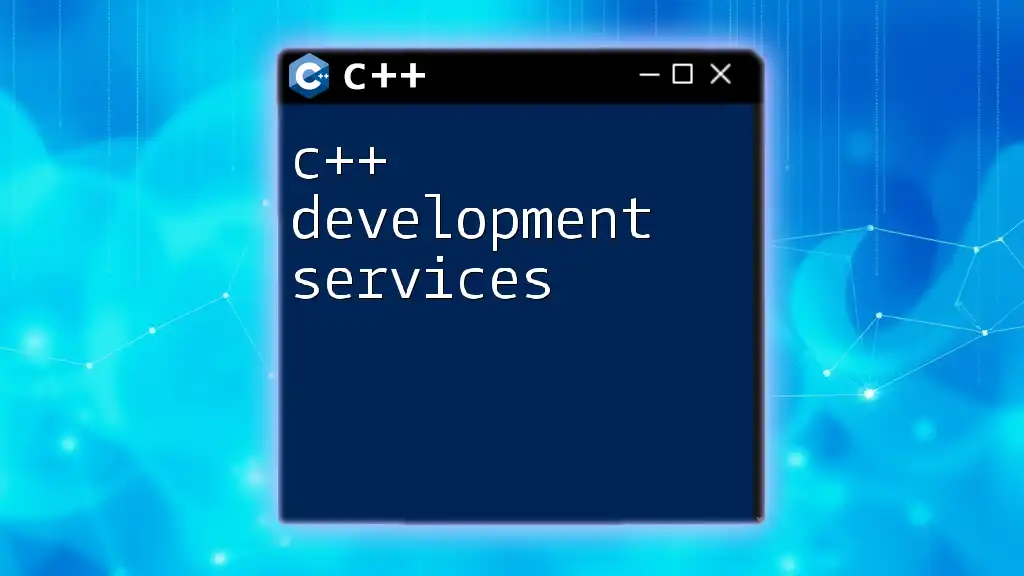
Additional Resources
For continued learning, consider referring to books like "The C++ Programming Language" by Bjarne Stroustrup or taking online courses that dive deeper into C++ concepts and practical applications.
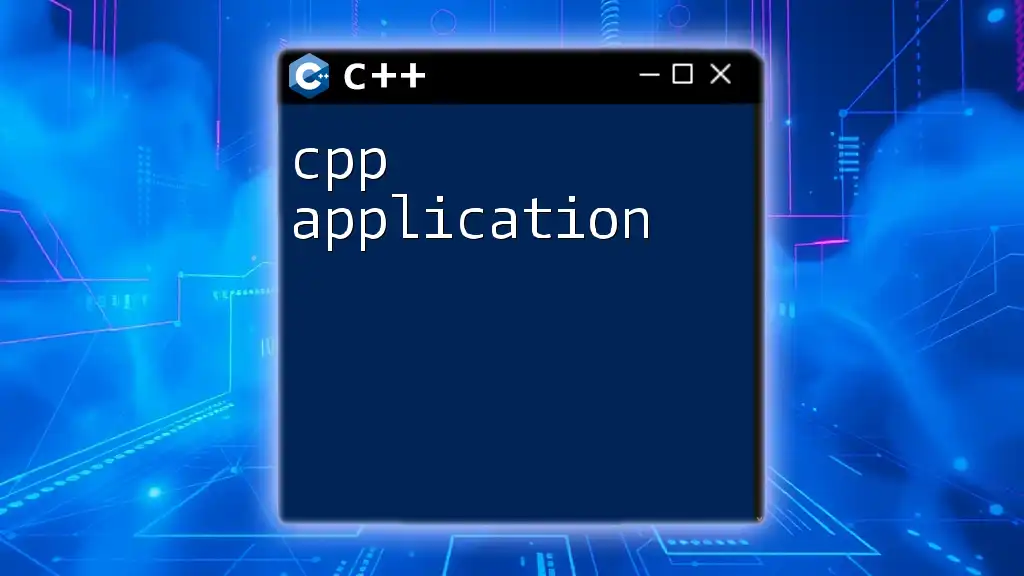
Call to Action
If you're eager to enhance your C++ skills further, subscribe to our newsletter or check out our specialized C++ courses aimed at guiding you in mastering C++ application development!