A C++ software developer is a professional who specializes in designing, implementing, and maintaining applications using the C++ programming language, often focused on performance and efficiency.
Here’s a simple code snippet that demonstrates a basic C++ program to print "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the C++ Language
What is C++?
C++ is a high-level programming language that builds on the foundation of the C language, introducing concepts such as object-oriented programming (OOP), which allows developers to create complex applications more intuitively. It was developed by Bjarne Stroustrup in the early 1980s and has since evolved to support various programming paradigms, including procedural, functional, and generic programming. C++ is widely used in industries ranging from game development and system software to high-performance applications like graphics engines.
Key Features of C++
C++ is known for its powerful features that enable developers to leverage both high-level abstractions and low-level control.
Object-Oriented Programming (OOP) Concepts
C++ facilitates the use of classes and objects, enabling developers to encapsulate data and functions together. This promotes code reuse and enhances software modularity.
For example:
class Animal {
public:
void sound() {
cout << "Animal sound" << endl;
}
};
int main() {
Animal myAnimal;
myAnimal.sound(); // Output: Animal sound
return 0;
}
In this example, `Animal` is a class, and `sound` is a method that belongs to that class. By creating an object of `Animal`, you can call the `sound` method.
Low-Level Manipulation
One of the critical advantages of C++ is its ability to perform low-level manipulation through pointers and references. This allows for efficient memory management, which is essential for performance-critical applications.
Here’s a simple demonstration:
int a = 10;
int* p = &a; // Pointer to a
cout << "Value of a: " << a << endl; // Outputs: 10
cout << "Value via pointer p: " << *p << endl; // Outputs: 10
Here, the pointer `p` holds the address of `a`, showcasing how direct memory access can be achieved with pointers.
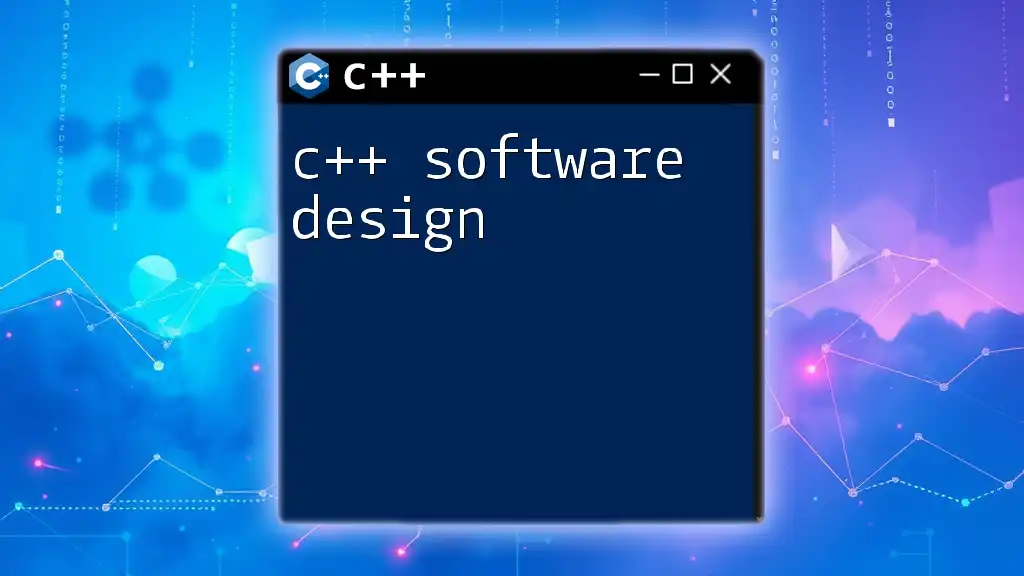
The Role of a C++ Software Developer
Responsibilities of a C++ Developer
A C++ software developer is responsible for writing, optimizing, and maintaining code that meets application requirements. They are tasked with debugging and troubleshooting issues that arise during the software lifecycle. Collaborating with cross-functional teams is another essential aspect of the role, ensuring that project goals are met efficiently through effective communication and cooperation.
Required Skills
Technical Skills
To be successful, a C++ software developer must have a proficiency in the language and a deep understanding of its concepts. Familiarity with development tools and environments, such as Visual Studio or Eclipse, is essential for efficient coding and debugging.
Soft Skills
Apart from technical aptitude, soft skills play a crucial role. Effective problem-solving abilities allow developers to identify and tackle challenges promptly. Equally important are communication and teamwork, which foster collaboration and enhance project outcomes.
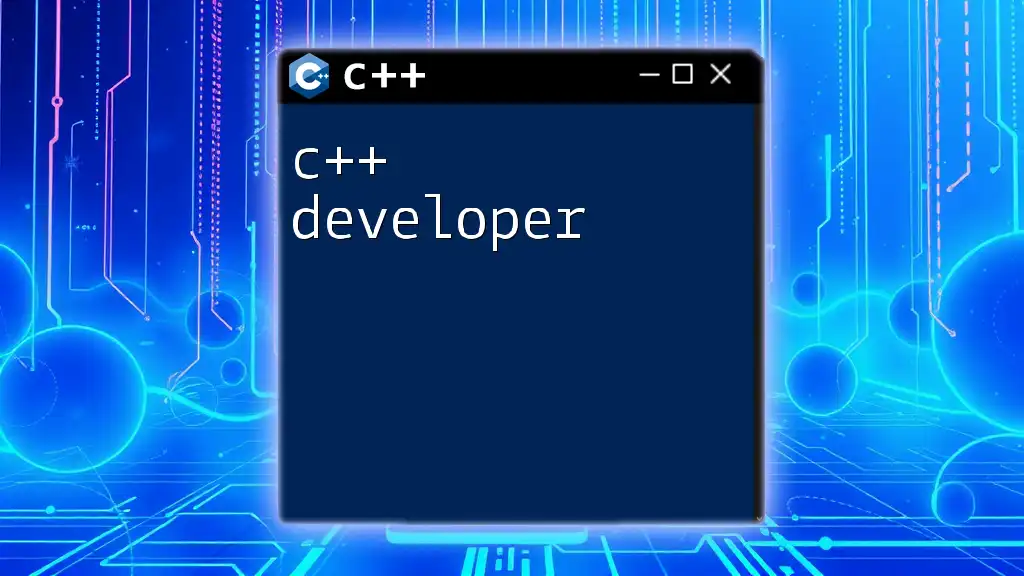
Educational Pathways
Academic Background
Most C++ software developers hold a degree in Computer Science or Software Engineering, emphasizing a solid grounding in programming principles and methodologies. Knowledge of mathematics and algorithms is often a vital component of their education.
Certifications
Acquiring relevant certifications, like those from the C++ Institute or Microsoft, can significantly enhance career prospects. Certifications validate one’s expertise and commitment to professional growth, making candidates more appealing to potential employers.
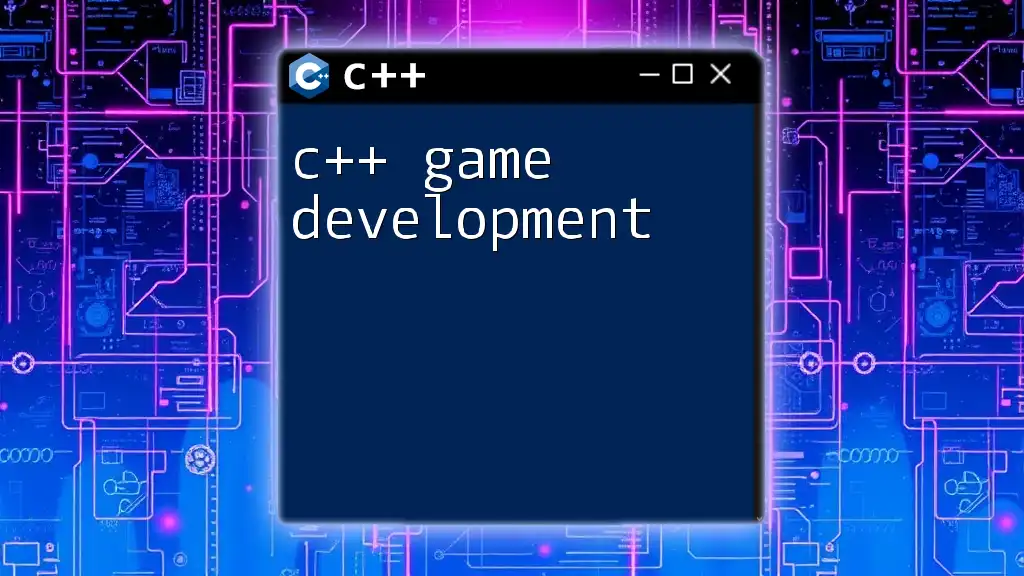
Career Path for C++ Developers
Entry-Level Positions
Common entry-level positions for C++ developers may include junior developer or programmer analyst. Responsibilities at this stage typically involve writing code for specific features or debugging software as part of a larger team.
Advancing Your Career
To further their careers, developers should focus on gaining experience and expertise by working on diverse projects and technologies. Networking within the tech community, attending industry conferences, and participating in online forums can also open doors to new opportunities.
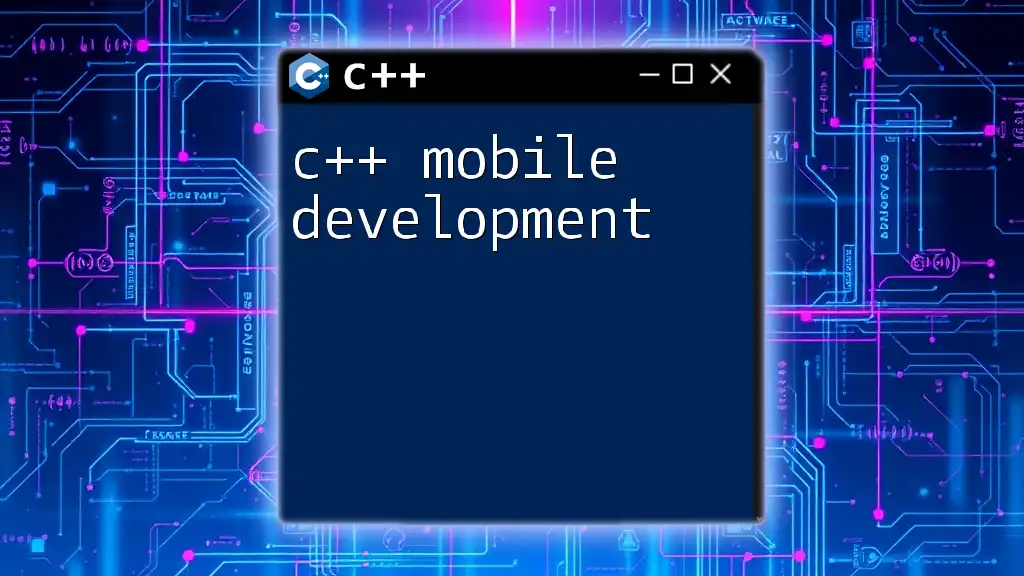
Tools and Technologies for C++ Development
Integrated Development Environments (IDEs)
Popular IDEs, such as CLion or Code::Blocks, simplify the development process by offering features like code completion, debugging, and project management. For instance, when starting a new project in CLion, you can create a main file where you can begin coding right away.
Standard Template Library (STL)
The Standard Template Library (STL) is an invaluable resource that provides a collection of ready-to-use classes and functions for common programming tasks. Using STL reduces development time and improves efficiency by allowing developers to utilize well-tested components.
Here’s an example of using STL with vectors:
#include <vector>
#include <iostream>
using namespace std;
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
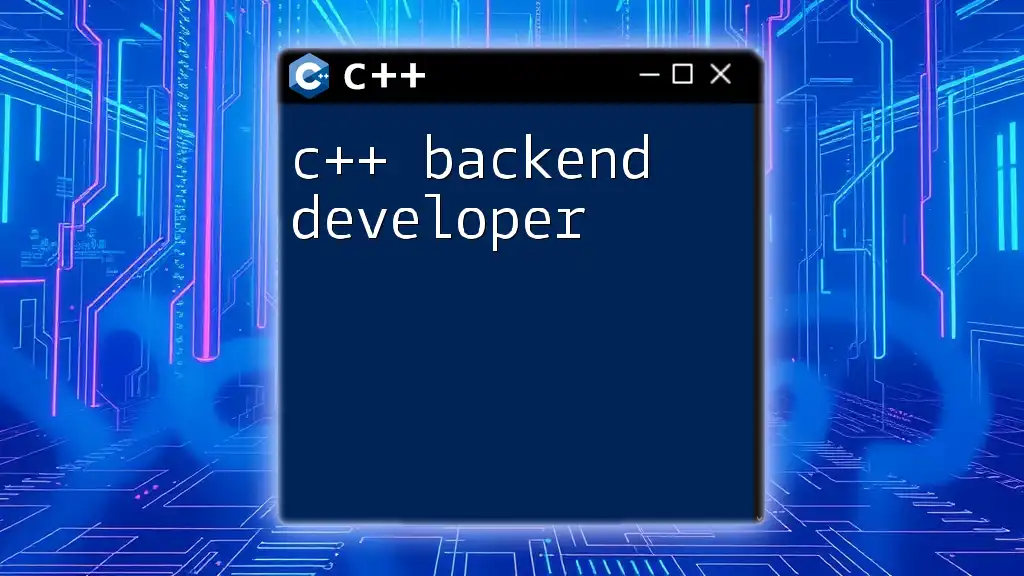
Best Practices in C++ Programming
Writing Clean Code
Writing clean and structured code is paramount. Readability and maintainability help ensure that your code can be easily understood by others or revisited by you in the future. An example of structured versus poorly formatted code can illustrate this difference starkly.
Performance Optimization
Performance optimization is vital in C++ development, especially for applications requiring high efficiency. Techniques such as minimizing resource usage and optimizing algorithms can greatly improve the performance of your applications.
For instance, you can optimize a function using the STL:
#include <vector>
#include <algorithm>
void optimizedFunction(std::vector<int>& data) {
std::sort(data.begin(), data.end()); // Using STL for efficiency
}
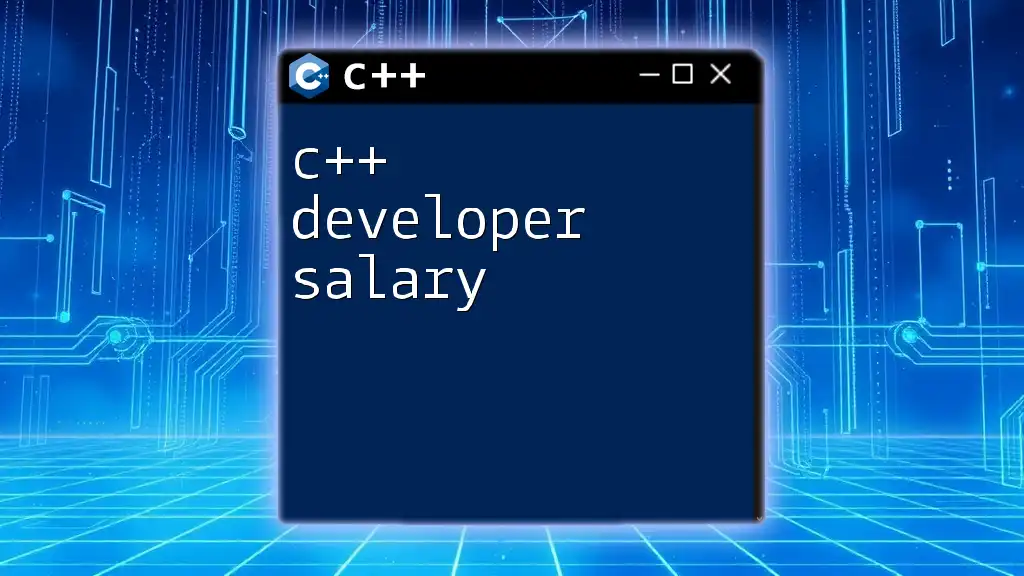
Challenges in C++ Development
Common Pitfalls
C++ developers frequently encounter challenges such as memory leaks and pointer misuse. For example, failing to deallocate memory after usage can lead to memory leaks, severely impacting application performance.
Staying Updated
Given the rapid pace of technological advancement, continuous learning is essential. Developers should regularly engage with new resources, including blogs, tutorials, and online courses, to keep their C++ skills sharp and up-to-date with industry standards.
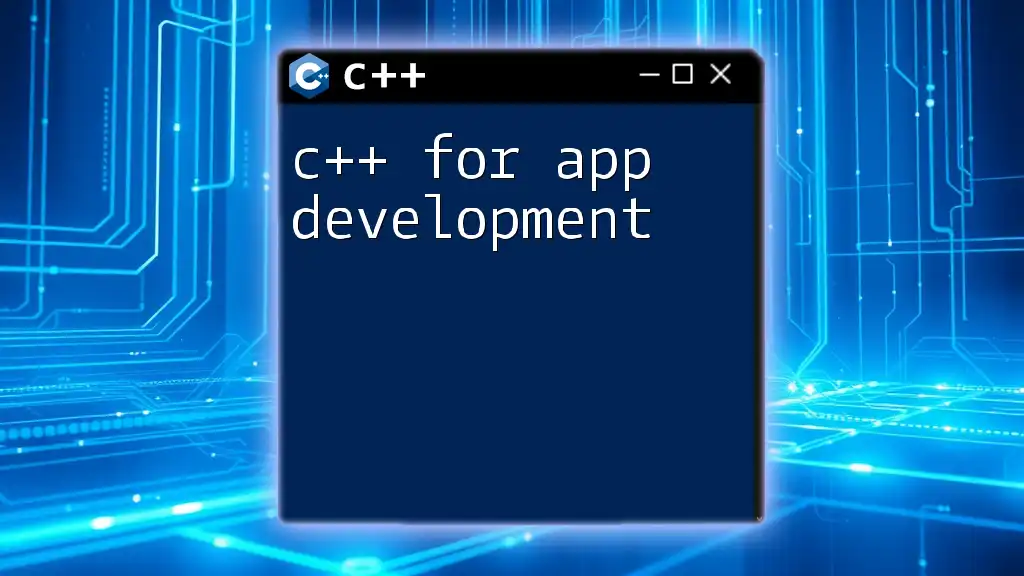
Conclusion
Becoming a successful C++ software developer involves mastering a robust language, acquiring the right skills, and continuously adapting to new challenges. With dedication and a commitment to learning, you can excel in this dynamic field and unlock numerous career opportunities.
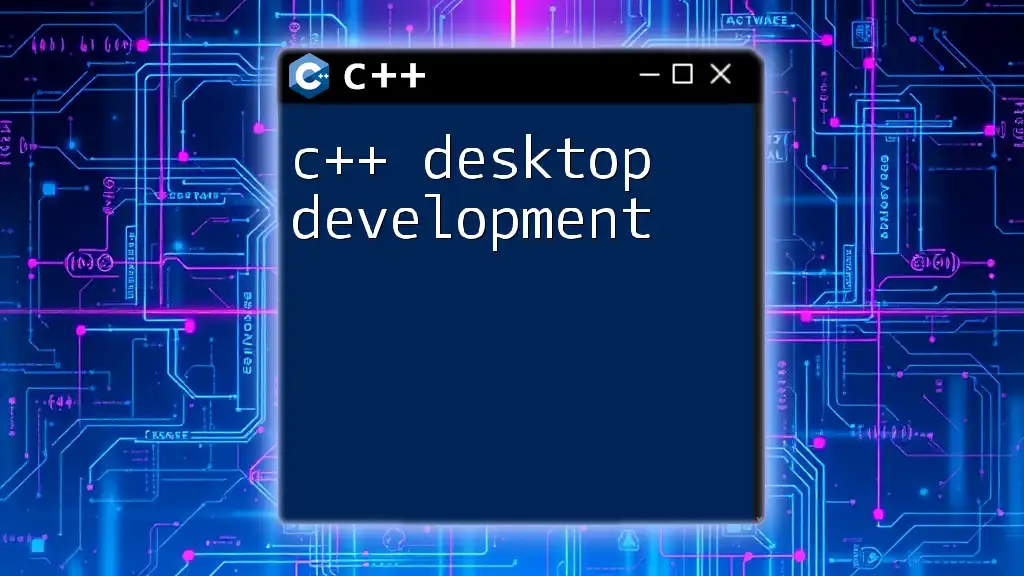
Additional Resources
For further exploration, consider reading engaging books and enrolling in courses specifically designed for C++ programming. Participating in online communities like forums and discussion groups can also enhance your understanding and provide additional support on your journey in C++ development.