In C++, you can forward declare an enumeration to allow its use in functions or classes before its full definition, using the following syntax:
enum Color; // Forward declaration
void setColor(Color color); // Function using the forward-declared enum
enum Color { RED, GREEN, BLUE }; // Full definition
Understanding Enums in C++
What is an Enum?
An enumeration (enum) is a user-defined type in C++ that consists of a set of named integral constants. Enums are used to represent a collection of related values, enhancing code readability and maintainability. Here’s the basic syntax for declaring an enum:
enum Color { Red, Green, Blue };
In this example, the enum Color defines three named constants: Red, Green, and Blue.
Benefits of Using Enums
Enums deliver multiple advantages:
-
Improved Code Readability: Enums allow programmers to represent meaningful names instead of arbitrary integers. This makes the code easier to read and understand.
-
Type Safety Advantages: Using enums provides type safety because they restrict the variable to accept only defined constants, reducing the chances of errors.
-
Use Cases for Enums: Enums are commonly used in scenarios like state machines, event handling, or representing categorized data such as colors, directions, or error codes.
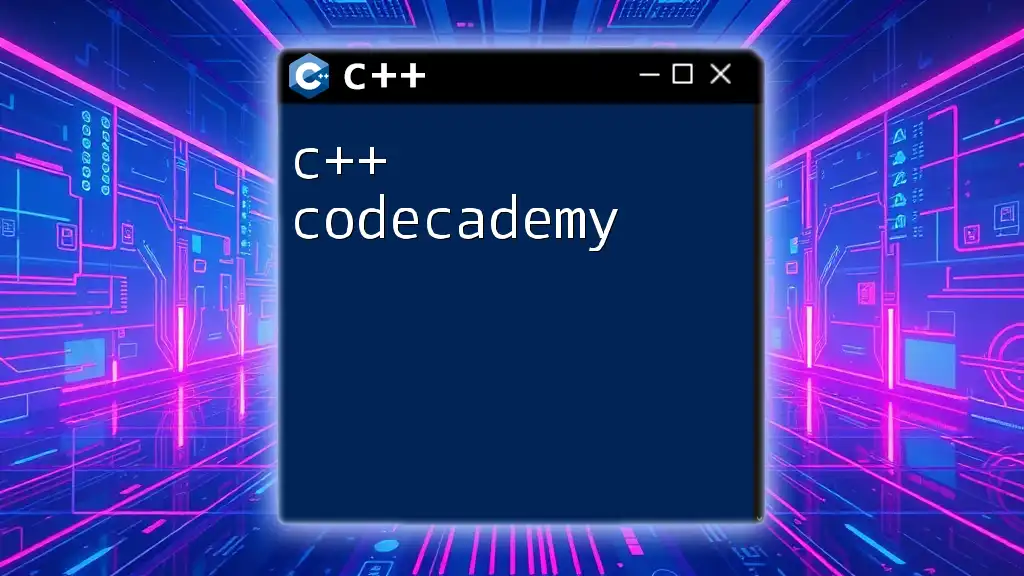
Forward Declaration in C++
What is Forward Declaration?
Forward declaration is a technique in C++ that allows the declaration of a type (such as a class, struct, or enum) before its full definition is available. It helps in managing dependencies in complex codebases.
Syntax of Forward Declaration
The syntax for a forward-declared enum looks like this:
enum class EnumName; // Forward declaration
This line informs the compiler that EnumName is an enumeration type and its definition will be provided later. It's crucial to note that the enum's constants cannot be used until the enum is fully defined.
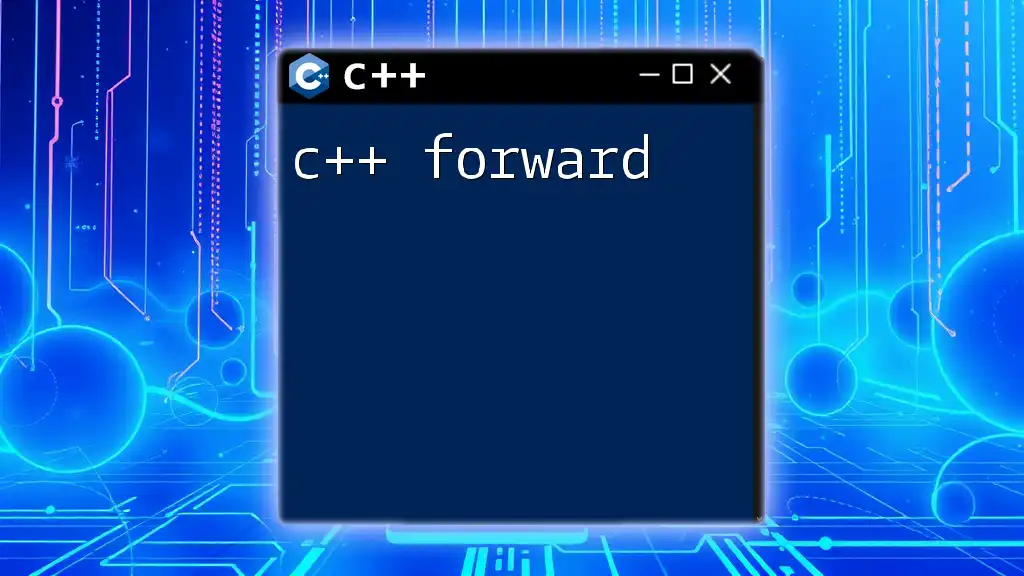
Forward Declaring an Enum in C++
Why Forward Declare an Enum?
Forward declaring an enum helps in:
-
Reducing Dependency: It minimizes interdependencies between headers, aiding in compilation efficiency. This is especially useful in large projects where reducing file inclusions can speed up the build process.
-
Avoiding Circular Dependencies: In complex code structures, forward declarations can resolve circular dependencies that occur when two or more types depend on each other.
How to Forward Declare an Enum
To correctly forward declare an enum, the declaration must be placed in a context where it will be used before its definition is known. Here’s an example:
enum class Direction; // Forward declaration
struct Movement {
Direction direction; // Using the forward-declared enum
};
In the snippet above, Direction is forward declared before being used in the Movement structure.
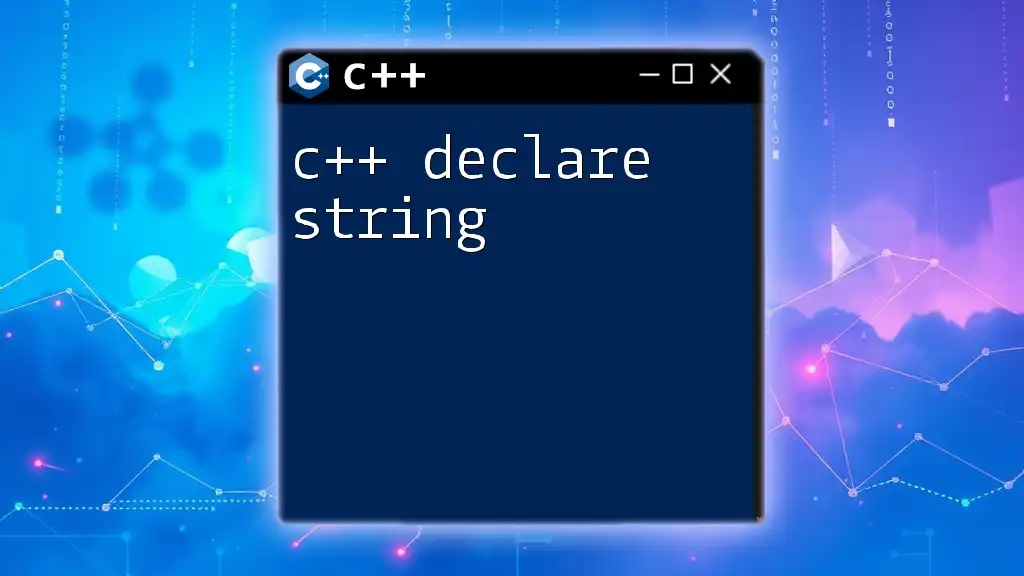
Defining the Forward Declared Enum
Where to Define the Enum
After forward declaration, it’s essential to define the enum in a compilation unit (usually in an implementation or header file) where it will be used. Following proper order helps in avoiding undefined references when accessing enum members.
Example: Complete Code Implementation
Here’s a complete example that demonstrates both forward declaration and definition:
// In a header file
enum class Direction; // Forward declaration
struct Movement {
Direction direction;
};
// In a source file
enum class Direction { North, South, East, West }; // Definition
This example shows how we first declare Direction and then define it, using it within the Movement structure.
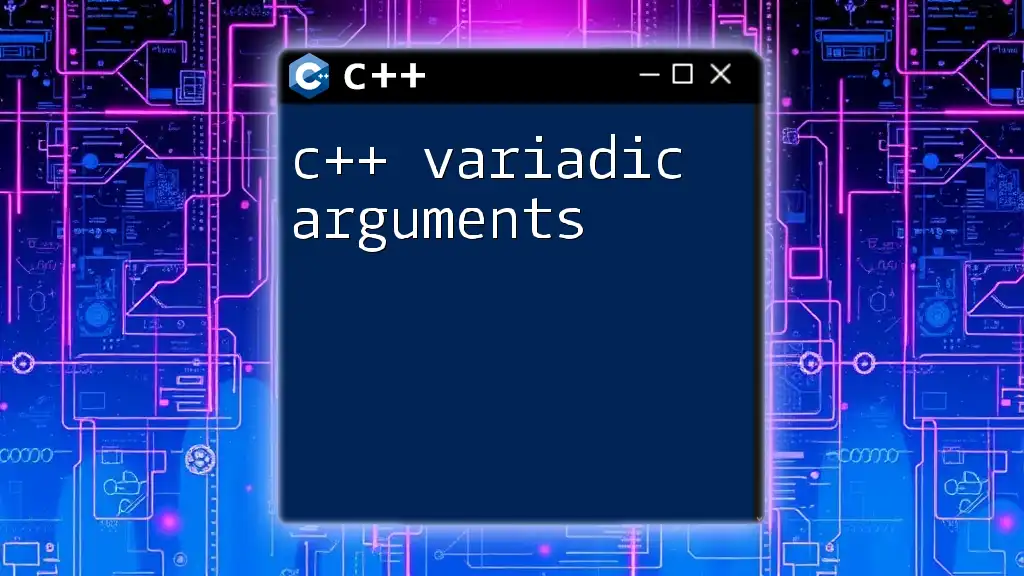
Using Forward Declared Enums
Initializing Enum Variables
After defining the enum, you can use its constants as needed. Here’s how to initialize a forward-declared enum variable:
Movement move;
move.direction = Direction::North; // Using the defined enum
This line of code creates an instance of Movement and sets its direction to North, showcasing straightforward usage of the enum.
Example of Function with Forward Declared Enum
You can also define functions that operate on variables of a forward-declared enum type. Here’s an example function that changes the direction of a Movement object:
void changeDirection(Movement& move, Direction newDirection) {
move.direction = newDirection;
}
This function takes a reference to a Movement object and a new direction, modifying the direction to the provided value.
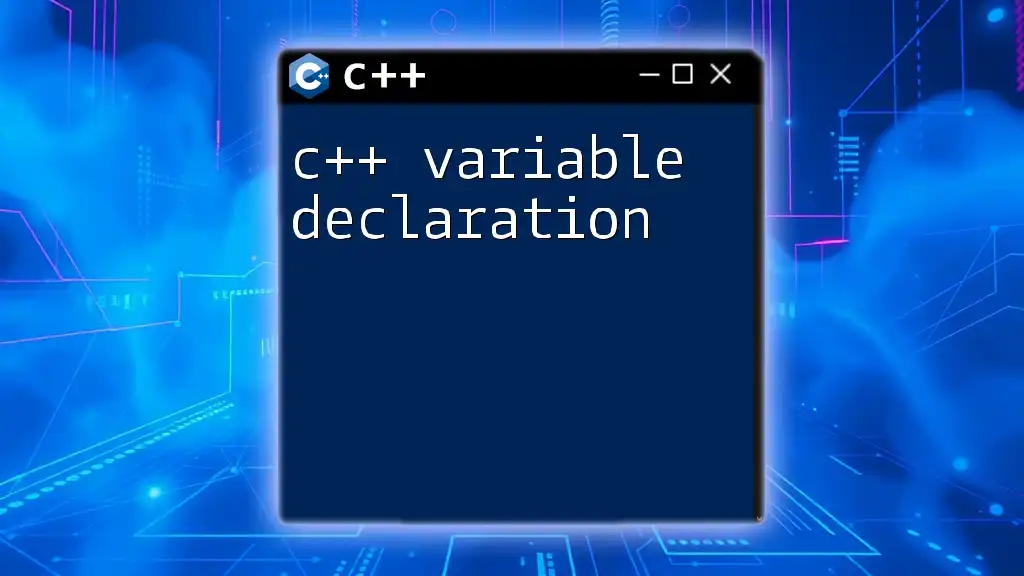
Common Pitfalls and Best Practices
Pitfalls of Forward Declaring Enums
One of the common pitfalls when forward declaring enums is forgetting to provide their definition before they are used. This results in compilation errors indicating that the enum type is unknown or incomplete.
Best Practices for Forward Declarations
Keep a few best practices in mind:
-
Organized Code: Ensure your forward declarations are logically grouped and placed in header files that represent the appropriate context.
-
Minimal Forward Declarations: Limit the use of forward declarations to what is necessary to avoid unnecessary complexity and make your code easier to understand.
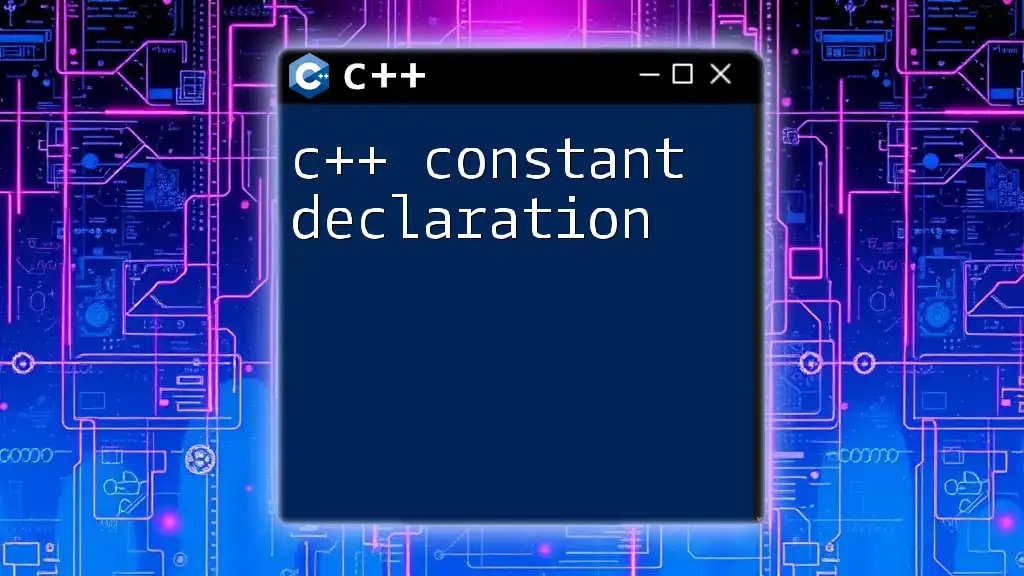
Conclusion
In summary, understanding how to c++ forward declare enum can significantly improve the maintainability of your code. By reducing dependencies and promoting better organization, forward declarations facilitate cleaner and more efficient programming practices. Embracing this technique encourages better design patterns and enhances code readability.
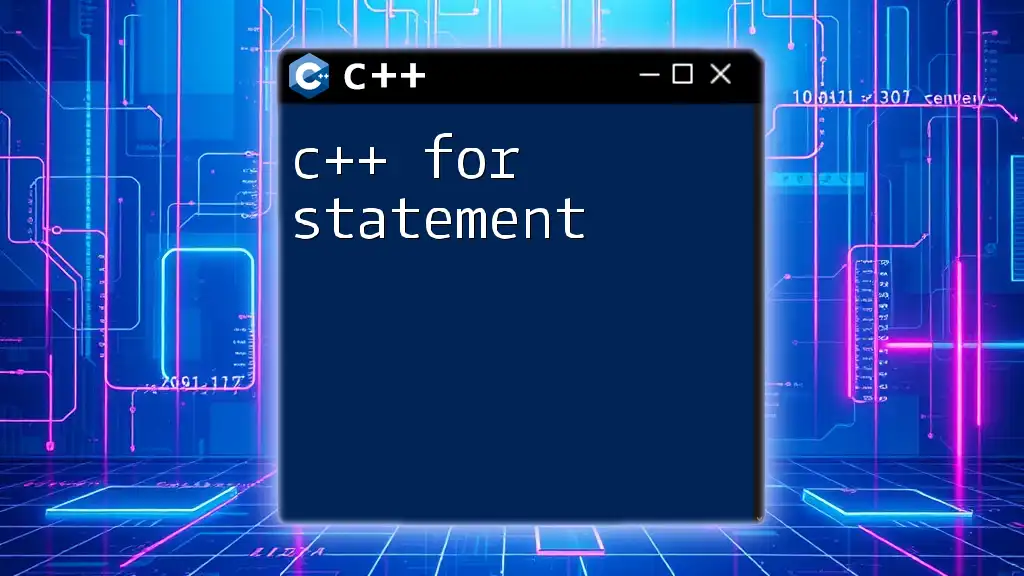
Further Reading and Resources
For more insights on C++ enums and associated best practices, explore additional resources, tutorials, and community forums dedicated to C++ programming.
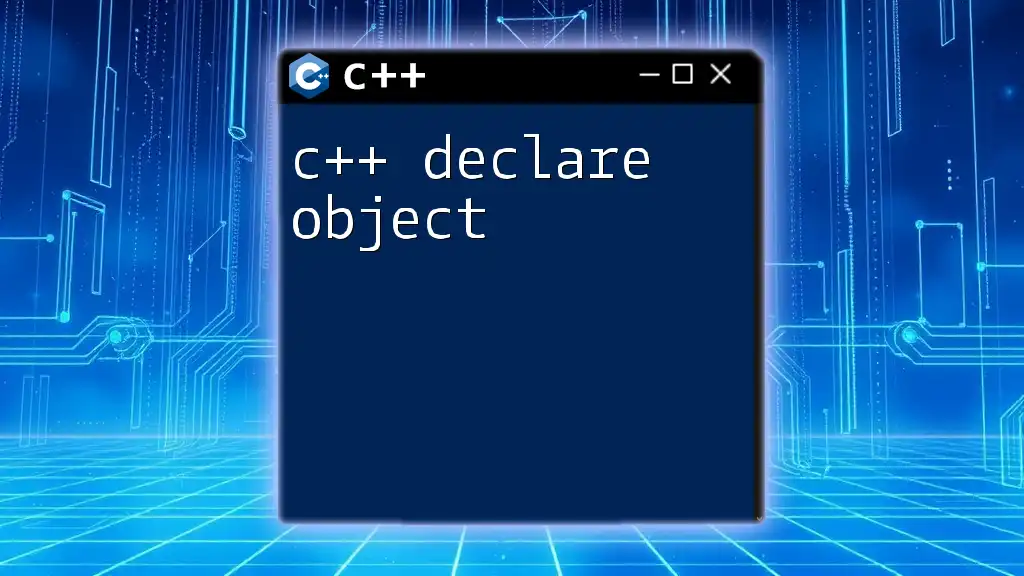
FAQs
What happens if you forget to define a forward-declared enum?
Forgetting to define a forward-declared enum leads to compilation errors where the compiler indicates that it cannot find the type or members of the enum, causing your code not to compile.
Can you forward declare an enum in a namespace?
Yes, you can forward declare an enum within a namespace. The syntax is as follows:
namespace MyNamespace {
enum class MyEnum; // Forward declaration within a namespace
}
This allows for better structure and organization in larger projects.