In C++, a forward declaration of a class allows you to inform the compiler about the existence of a class before it is fully defined, enabling references or pointers to that class to be used in earlier declarations.
Here’s a code snippet demonstrating a forward declaration:
// Forward declaration of class B
class B;
class A {
public:
void func(B* b); // Pointer to B can be used here
};
class B {
public:
void display() {
// Some implementation
}
};
What is Forward Declaration in C++?
Forward declaration in C++ is a powerful technique that allows you to inform the compiler about the existence of a class, function, or variable before actually defining it. This approach is pivotal, as it enables better organization, reduces compilation times, and avoids issues such as circular dependencies.
Definition of Forward Declaration in C++
A forward declaration acts as a promise to the compiler that a certain class will be defined later in the program. By employing this technique, programmers can declare pointers or references to a class without needing the full class definition at that point in the code.
Purpose of Forward Declaration in Class Design
In C++ class design, forward declaration becomes essential when you want to use a type before it's fully defined. By doing so, you:
- Reduce dependencies: Minimizing the connections between source files can lead to less complex build processes.
- Enhance manageability: Code becomes easier to read and comprehend when unnecessary details are omitted from earlier parts of the code.
Key Benefits of Using Forward Declarations
- Improved Compilation Time: Using forward declarations effectively decreases the number of headers that need to be included, leading to quicker compilation and less bloat.
- Circular Dependency Resolution: Forward declarations can significantly mitigate the risk of circular dependencies, which arise when two classes reference each other.
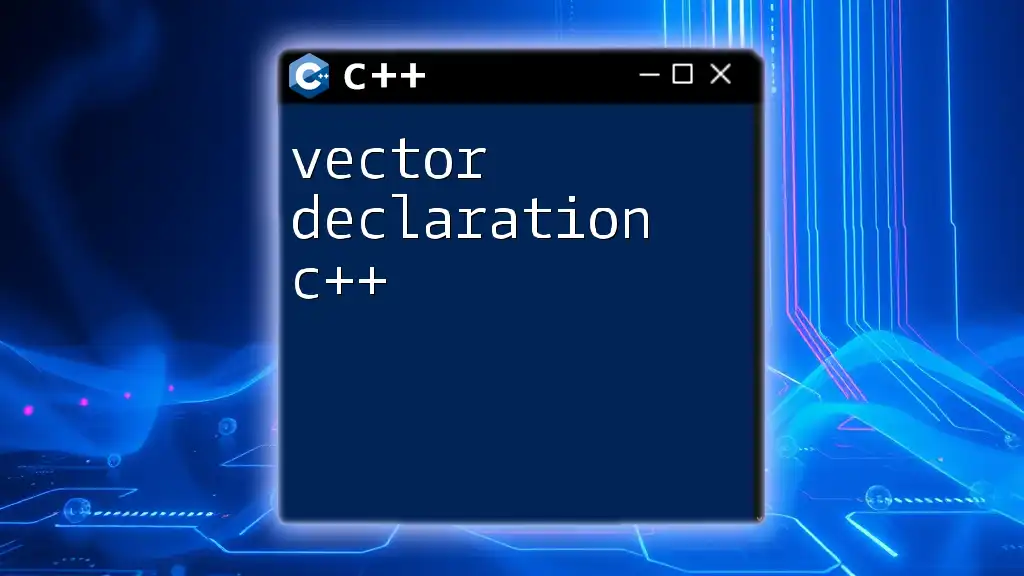
Understanding Class Forward Declaration
What is a Class Forward Declaration?
A class forward declaration specifies the existence of a class without providing the full definition. This is crucial for cases where you want to use pointers or references of that class before defining its complete structure.
Syntax of Class Forward Declaration in C++
The syntax for a class forward declaration is simple:
class MyClass; // Forward declaration
This line of code conveys to the compiler that `MyClass` will be defined later, allowing you to declare pointers or references to it without knowing its details at that moment.
Key Characteristics of Class Forward Declaration
When using forward declarations, it's important to realize the limitations:
- You can declare pointers or references: You may declare pointers to the forward-declared class in another class or function.
- You cannot instantiate objects: Instantiating an object of the forward-declared class is not possible until its definition has been provided.
- Member functions cannot be defined: While you can declare member functions, their definitions cannot be written until the full class definition is available.
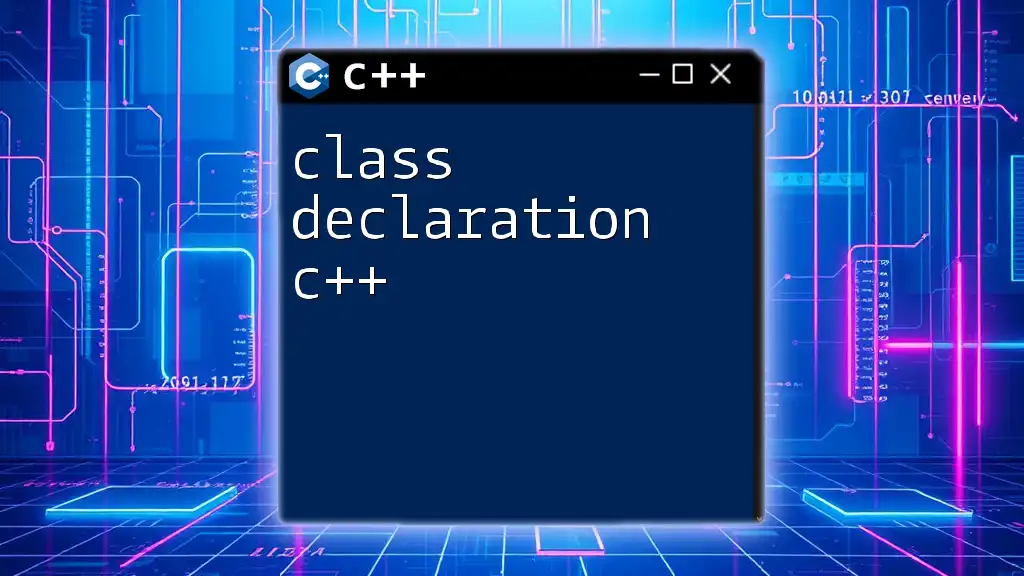
Why Use Forward Declaration?
Improving Compilation Time
Using forward declarations can lead to significant improvements in compilation speed. By decreasing the number of included headers, the compiler has fewer files to parse during compilation. This enhancement is especially beneficial in large projects with intricate interdependencies between classes.
Resolving Circular Dependencies
Circular dependencies can create severe problems in C++ programming. They occur when two or more classes reference each other directly or indirectly. Forward declaration provides a mechanism to break this cycle.
Consider the following scenario:
class ClassA; // Forward declaration
class ClassB {
public:
ClassA* referenceToA; // Using forward declaration to declare a pointer
};
class ClassA {
public:
ClassB instanceOfB; // Full definition of ClassA can reference ClassB
};
With the above approach, `ClassB` can reference `ClassA` without needing its full definition immediately, thus avoiding a circular dependency.
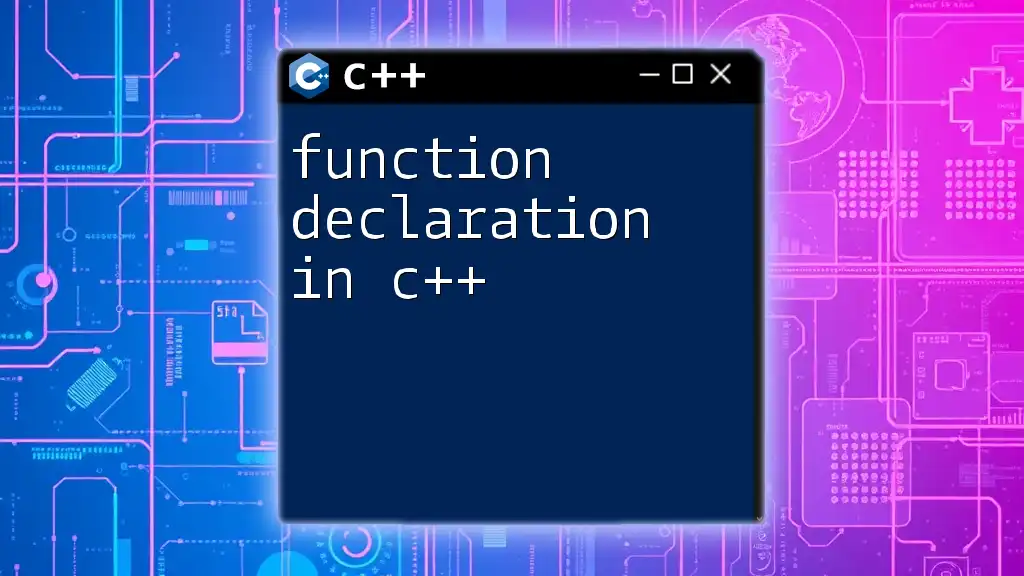
Practical Examples of Forward Declaration
Example 1: Basic Forward Declaration
Here's a straightforward example illustrating a basic forward declaration:
class A; // Forward declaration
class B {
A* a; // Pointer to A, only declared
};
class A {
// Full definition, can be completed later.
};
In this case, `B` has a pointer to an object of class `A`, which is permitted because `A` is forward-declared.
Example 2: Using Forward Declaration with Member Functions
Forward declarations are particularly useful when passing objects as arguments to member functions. Here's an example:
class Engine; // Forward declaration
class Car {
public:
void setEngine(Engine* e); // Function declaration using forward declaration
};
class Engine {
// Definition of Engine
};
In this scenario, the member function `setEngine` can accept a pointer to `Engine` without requiring the complete structure of `Engine` at that moment.
Example 3: Real-World Use Case
Let’s look at a slightly more complex use case involving multiple classes and forward declarations:
class Order; // Forward declaration
class Customer {
public:
void placeOrder(Order* order); // Declare a method that accepts a pointer to an Order
};
class Order {
// Definition of the Order class
};
Using forward declarations enhances code clarity and organization, allowing the definition of `Order` to be separate from `Customer`.
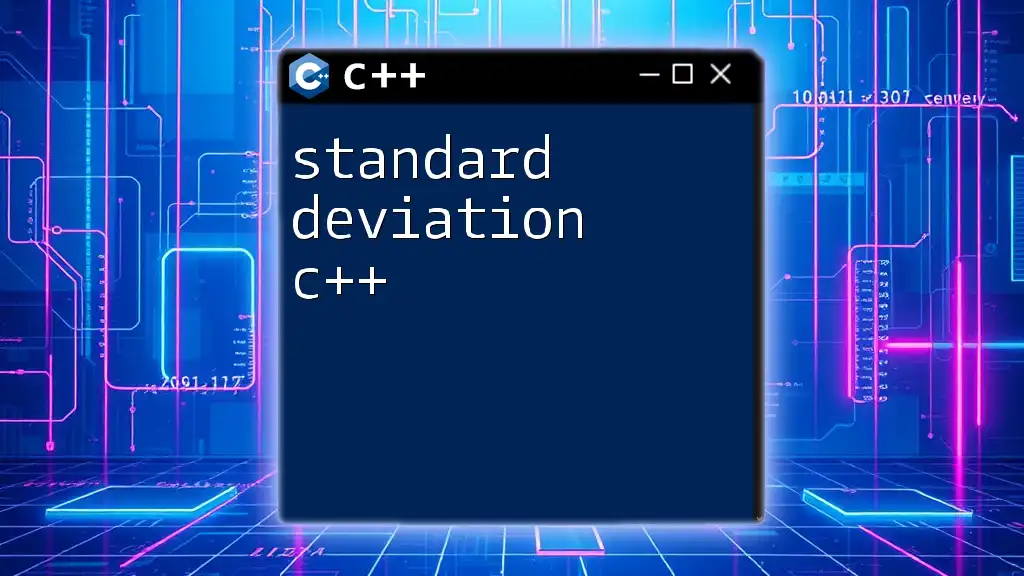
Limitations of Forward Declaration
When Not to Use Forward Declaration
Forward declarations are not universally applicable. Situations where they might lead to confusion include:
- Complex method signatures: If a method's signature requires knowledge of the class's members or behavior, a forward declaration won't suffice.
- Overcomplication: An overuse of forward declarations can lead to less readable code, as it may become unclear what types are being referenced at a glance.
Understanding Scope and Lifetime
Scope issues may arise when using forward declarations. Each declaration exists within the context in which it is declared. It’s crucial to ensure that both the constructors and destructors are defined to prevent undefined behaviors when instances are created.
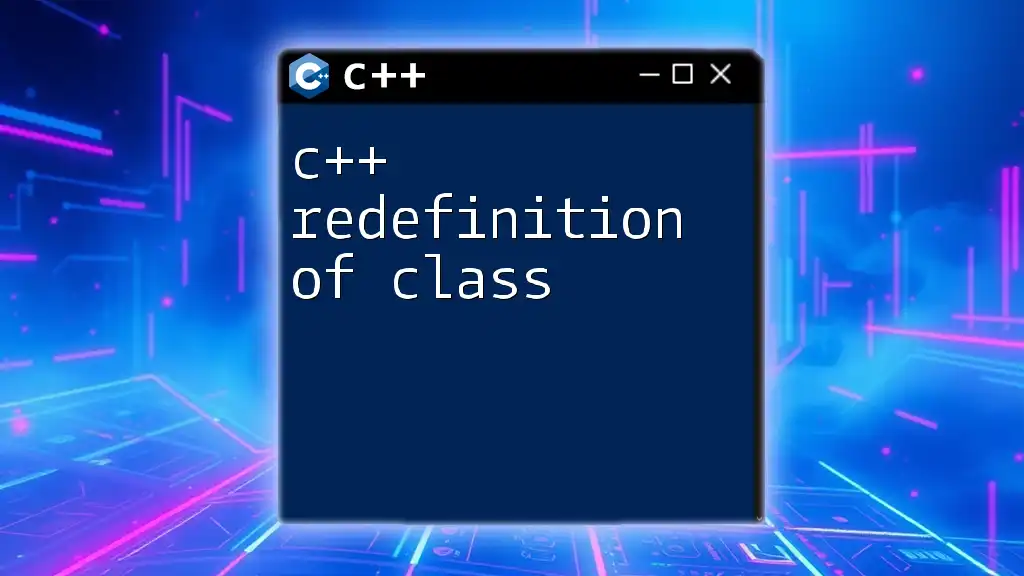
Best Practices for Forward Declaration in C++
Keep Declarations Clear and Understandable
While using forward declarations, it’s vital to maintain clarity. Commenting on each forward declaration can aid other developers in understanding the code better. For instance:
class A; // Forward declaration. A will be defined later.
This simple comment provides clarity about the future definition of the class.
Use Forward Declarations Where Appropriate
Striking a balance is essential. Use forward declarations strategically in situations where they enhance code cleanliness and avoid circle dependencies. Avoid using them excessively; sometimes, a full class definition may provide more immediate clarity for the reader.
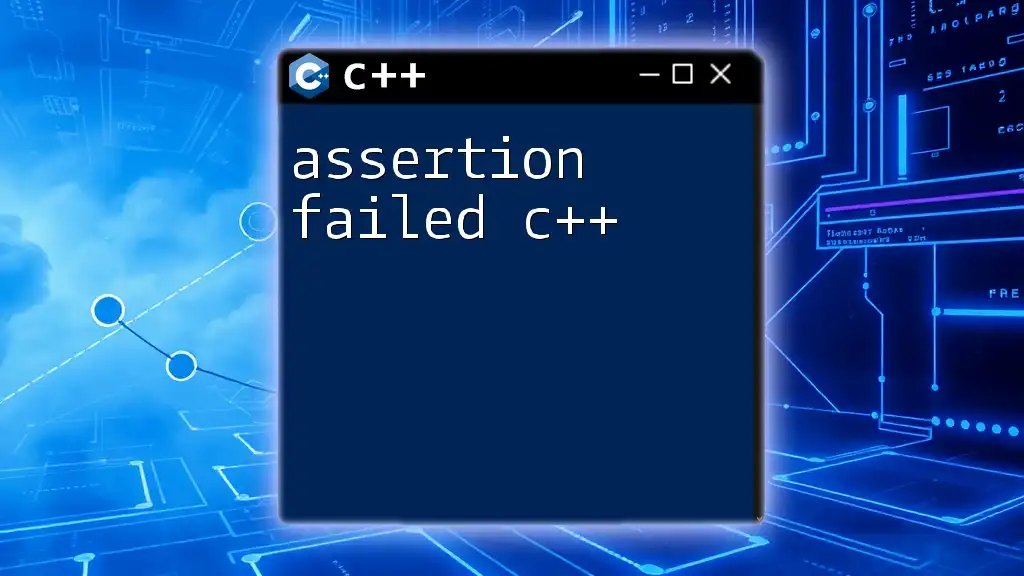
Conclusion
The forward declaration of class in C++ is a vital technique that enhances code organization, reduces compilation times, and mitigates the risk of circular dependencies. Understanding when and how to use forward declarations is integral for any C++ developer looking to write efficient, maintainable code. With practice, mastering forward declarations can lead to more robust and clearer C++ applications.
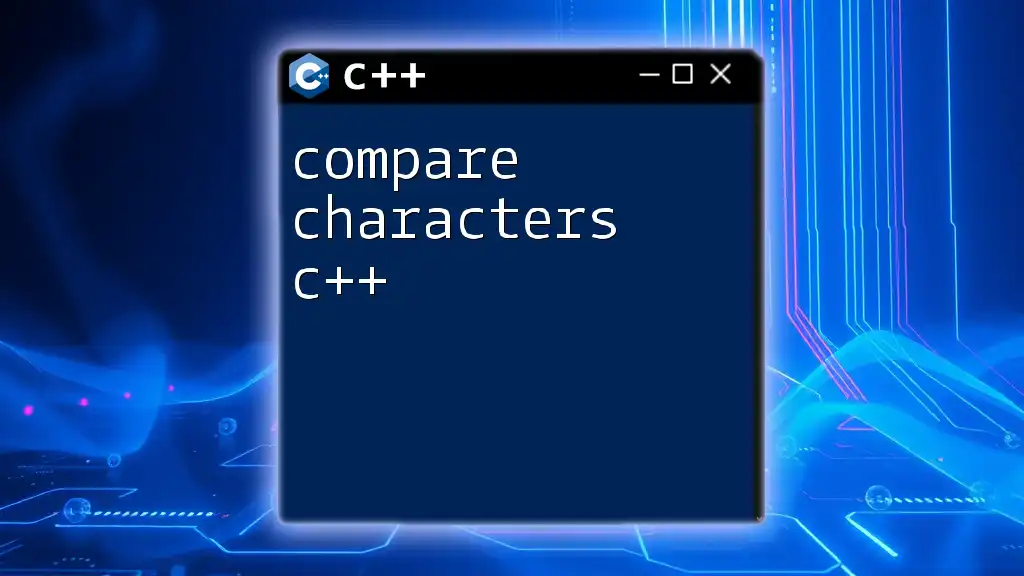
Additional Resources
For those looking to deepen their knowledge beyond this guide, consider exploring technical books, documentation, and online forums. Engaging in platforms where programmers gather and share insights can provide invaluable perspectives on effective C++ programming practices.
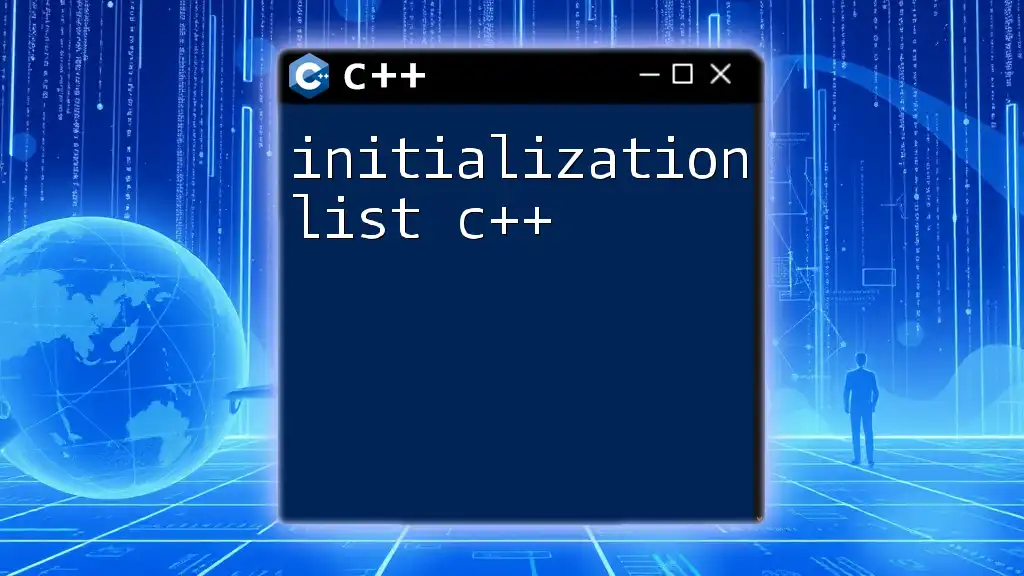
Call to Action
I encourage you to engage with forward declarations in your coding projects. Try implementing them in your designs and share your experiences or any questions you may have! Through collaborative learning, we can enhance our understanding of C++ together.