Foundation classes in C++ are fundamental classes that provide essential functionalities for building applications, often focusing on object-oriented principles and reusable components.
Here's a simple code snippet demonstrating a basic foundation class in C++:
class Shape {
public:
virtual double area() const = 0; // Pure virtual function for area
virtual ~Shape() {} // Virtual destructor
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() const override {
return width * height; // Calculate area of rectangle
}
};
What Are Foundation Classes?
Foundation classes for C++ are integral components that serve as the building blocks of the C++ programming language. They encapsulate essential functionalities that help developers create robust, efficient programs. By understanding these classes, programmers can leverage established patterns and structures, significantly enhancing their productivity.
At its core, a foundation class encapsulates the principles of:
- Abstraction: Hiding complex implementation details while exposing simple interfaces.
- Encapsulation: Bundling data and methods that operate on the data within one unit.
- Inheritance: Allowing new classes to inherit properties and behaviors from existing ones.
- Polymorphism: Facilitating a single interface to represent different underlying data types.
These principles enable better organization and management of code, making foundation classes essential to effective C++ programming.
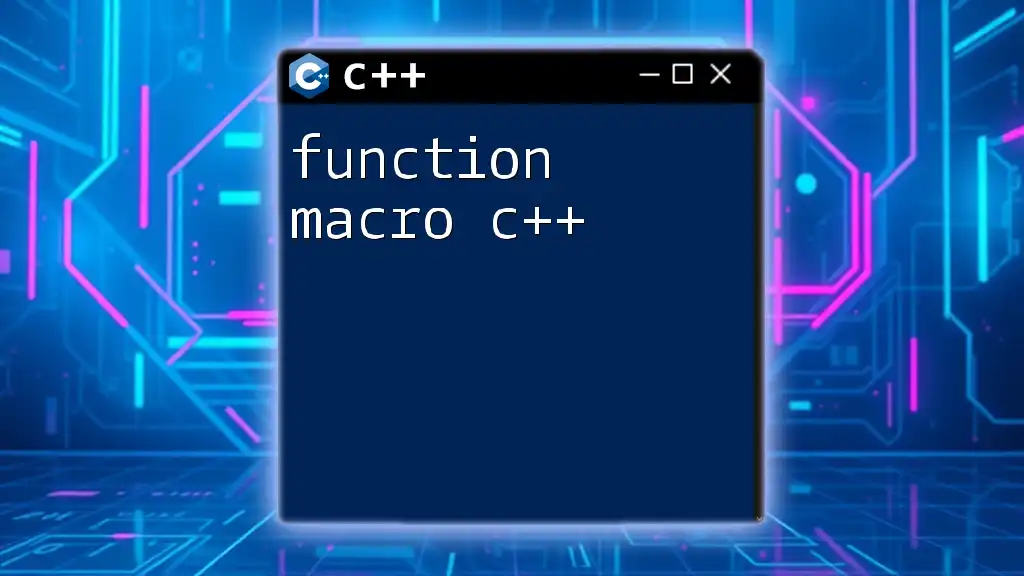
The Role of Foundation Classes in C++
Foundation classes play a pivotal role in C++ programming by providing essential structures and functionalities. They simplify complex tasks by allowing developers to focus on higher-level programming rather than low-level implementation details.
Understanding and utilizing these classes provides several advantages:
- Code Reusability: As foundation classes are designed to be reused across different projects, they help developers save time and effort.
- Improved Program Design: Well-structured foundation classes promote better software architecture, making it easier to maintain and extend programs.
- Increased Efficiency: By using established foundation classes, programmers can take advantage of optimized algorithms and data structures that boost performance.
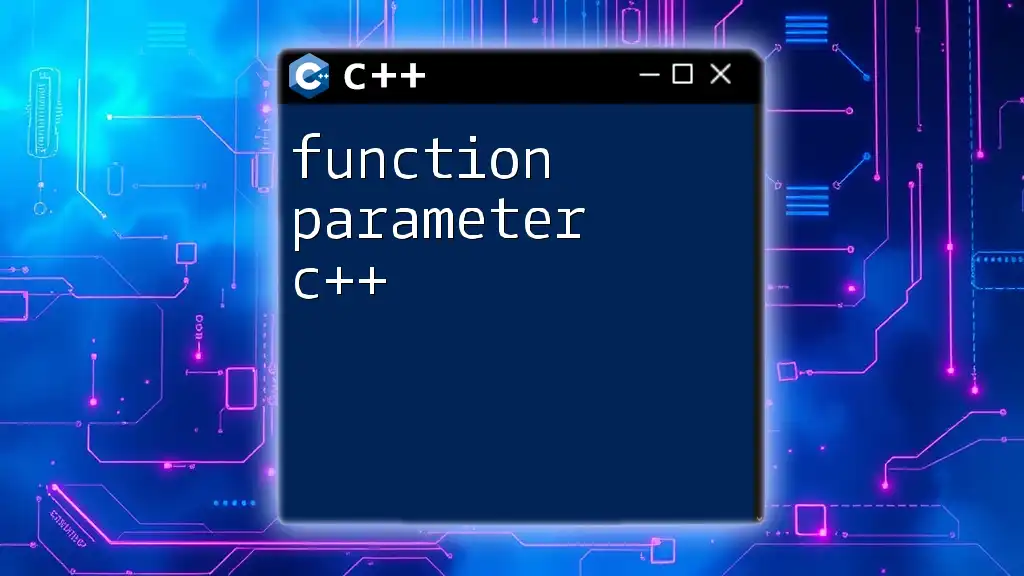
Common Foundation Classes in C++
Standard Template Library (STL) Overview
The Standard Template Library (STL) is a powerful collection of C++ template classes and functions that provides generic algorithms and data structures. It introduces several common foundation classes that enhance programming efficiency.
Containers
Containers are a fundamental component of the STL that stores collections of objects. Here are some widely used container classes:
Vector
A `vector` is a sequence container that manages dynamic arrays. It allows you to store and manipulate data efficiently.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int i : numbers) {
std::cout << i << " ";
}
return 0;
}
Vectors can dynamically resize, providing benefits in terms of both flexibility and performance. They are most suitable when you require random access and frequently add or remove items at the end of the list.
List
In contrast to vectors, `list` provides a doubly linked list implementation. This means that elements can be easily added or removed from anywhere in the sequence without reallocating or moving the entire structure.
#include <list>
#include <iostream>
int main() {
std::list<int> myList = {10, 20, 30, 40};
for (int n : myList) {
std::cout << n << " ";
}
return 0;
}
Lists excel in scenarios where frequent insertions and deletions are required, especially in the middle of the sequence.
Iterators
Iterators are objects that provide a mechanism for traversing containers without exposing their underlying structure. They are essential for working with the elements of containers in a uniform manner.
What Are Iterators?
An iterator acts like a pointer that enables you to navigate through a container's elements. It abstracts away the underlying data structure, allowing you to interact with it through the same interface.
Types of Iterators
There are several types of iterators in C++, including:
- Input Iterators: Allow reading data.
- Output Iterators: Allow writing data.
- Forward Iterators: Can read and write data in one direction.
- Bidirectional Iterators: Can move both forward and backward.
- Random Access Iterators: Allow arbitrary access to elements in constant time.
Here's a basic example of using an iterator:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::vector<int>::iterator it;
for (it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Algorithms
Algorithms are an essential part of the STL and refer to a collection of functions designed to perform operations on containers, such as sorting and searching.
Overview of Algorithms in STL
The STL provides several built-in functions that can manipulate various container types. These algorithms enhance the performance and efficiency of your applications.
Common Algorithm Examples
- Sorting: Arranging elements in a predefined order.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {4, 1, 3, 2};
std::sort(vec.begin(), vec.end());
for (int n : vec) {
std::cout << n << " ";
}
return 0;
}
Sorting helps in efficiently organizing data for any subsequent operations that may occur.
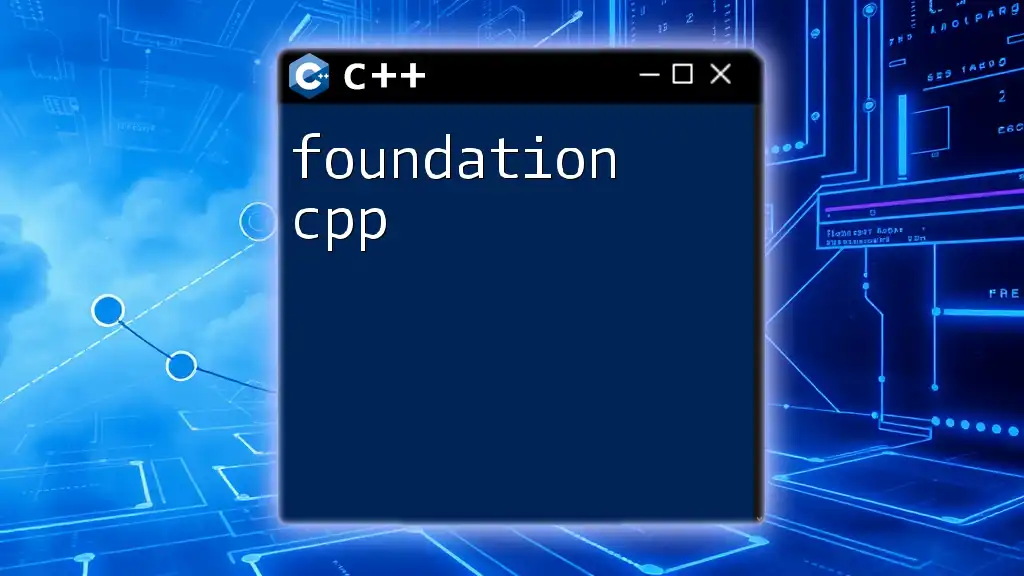
Building Custom Foundation Classes
When to Build Custom Classes
While foundation classes provided by STL cover numerous scenarios, there may be specific cases where custom classes are necessary. These instances arise when a unique data structure or behavior specific to your application is needed.
Designing Your Own Foundation Class
Creating a custom foundation class involves defining its attributes and methods. Here’s how you can create a basic class:
class MyClass {
private:
int value;
public:
MyClass(int v) : value(v) {}
int getValue() { return value; }
};
This simple class encapsulates a single integer value and provides a method to retrieve it. Custom classes enable you to implement tailored functionalities according to your project requirements.
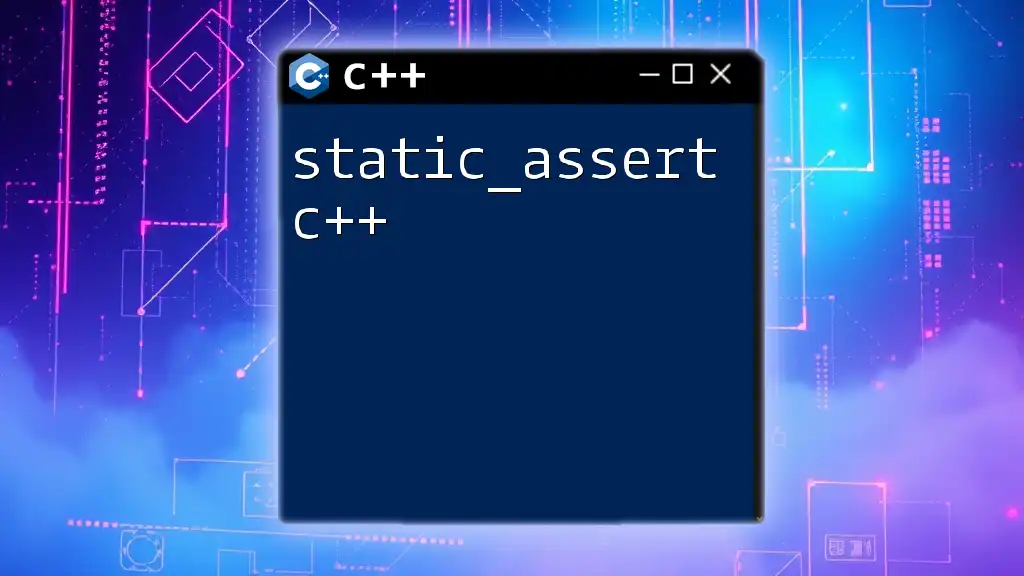
Best Practices Using Foundation Classes
To make the most of foundation classes for C++, consider the following best practices:
Leveraging Existing Libraries: Utilizing the STL and other libraries is critical to writing efficient and maintainable code. It saves development time and leverages optimization done by seasoned library developers.
Avoiding Common Pitfalls: Be mindful of common mistakes, such as incorrect iterator usage or memory leaks. It is essential to grasp the underlying mechanics to prevent these issues.
Memory management is especially vital in C++ since it is a language that requires manual control over resources.
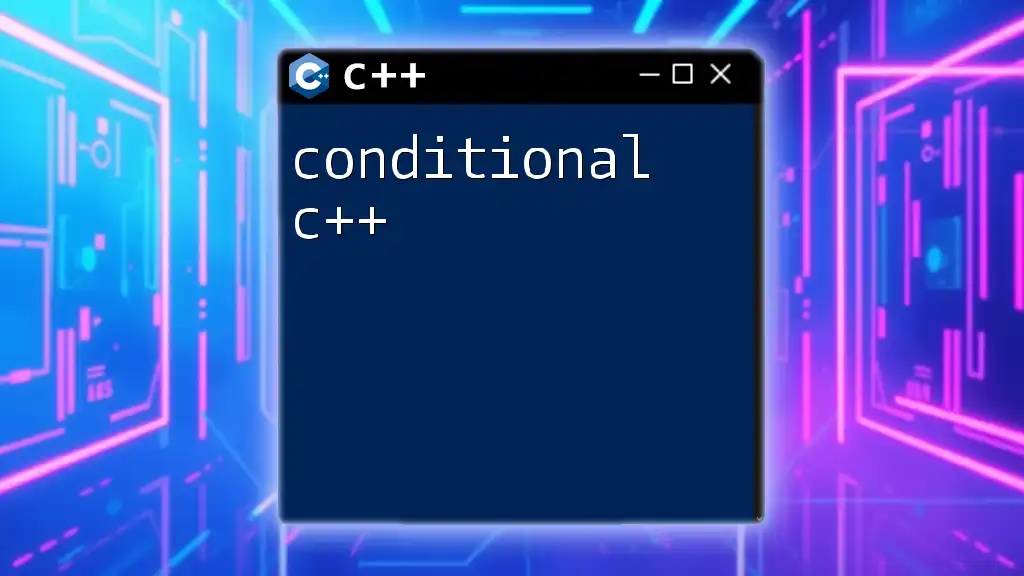
Performance Considerations
Performance in C++ can significantly vary based on the choice of data structures and algorithms.
Evaluating Communication Overhead in Foundation Classes
Improper usage of foundation classes can lead to communication overhead. It’s essential to evaluate the efficiency of your chosen class, particularly in scenarios where performance is paramount.
Benchmarking and Analysis
To optimize your code, employing benchmarking techniques can help you assess and analyze the performance of different foundation classes. Identifying bottlenecks or inefficiencies permits developers to refactor or replace components, ultimately leading to improved application performance.
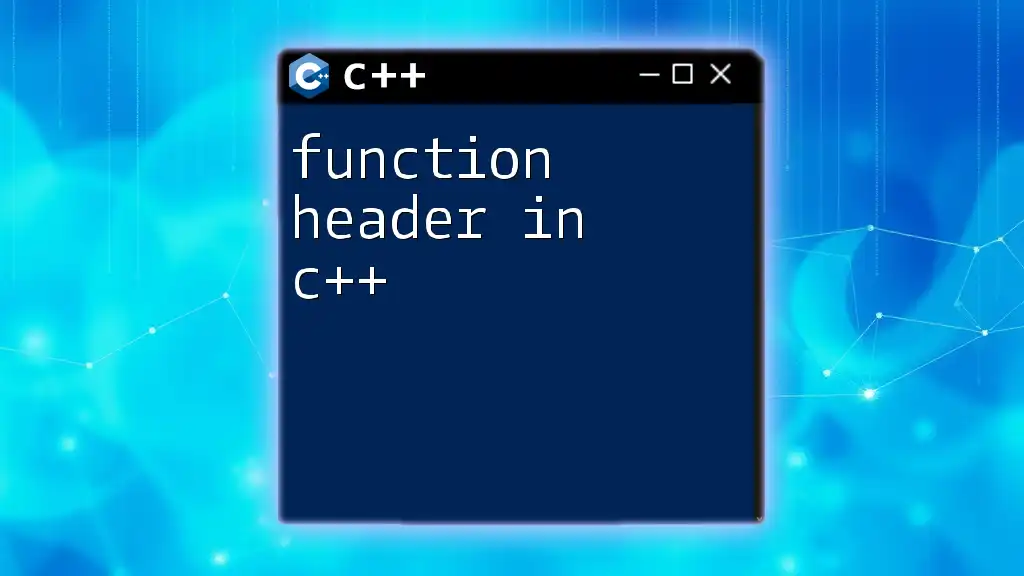
Conclusion
The exploration of foundation classes for C++ reveals their substantial role in simplifying development and enhancing program design. By leveraging these classes and understanding their core principles, developers can create robust and efficient applications. As you grow in your C++ programming journey, consider diving deeper into custom implementations, optimizing performance, and further utilizing the powerful capabilities offered by the standard libraries.