Modern CMake simplifies the build process for C++ projects by providing an intuitive scripting language and powerful features to manage dependencies, configurations, and targets efficiently.
Here's a basic example of a CMakeLists.txt file using modern CMake:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
add_executable(MyExecutable main.cpp)
Overview of CMake
What is CMake?
CMake is an open-source, cross-platform tool that automates the build process for software projects. Originating in the early 2000s, CMake has become the de facto standard for C++ projects, owing to its intuitive syntax and flexibility. It simplifies the management of project dependencies, provides built-in testing capabilities, and generates platform-specific build files. By mastering modern CMake for C++, developers can efficiently handle complex builds, making it an essential skill in today's programming landscape.
Key Features of CMake
Modern CMake is equipped with a plethora of features that enhance its utility:
- Cross-Platform Support: CMake enables the same build instructions to be used across various platforms like Windows, macOS, and Linux.
- Integrated Testing: CMake allows for easy integration of testing frameworks, which helps in maintaining code quality throughout development.
- Package Management: It can seamlessly integrate with popular package managers, enabling easy management of dependencies.
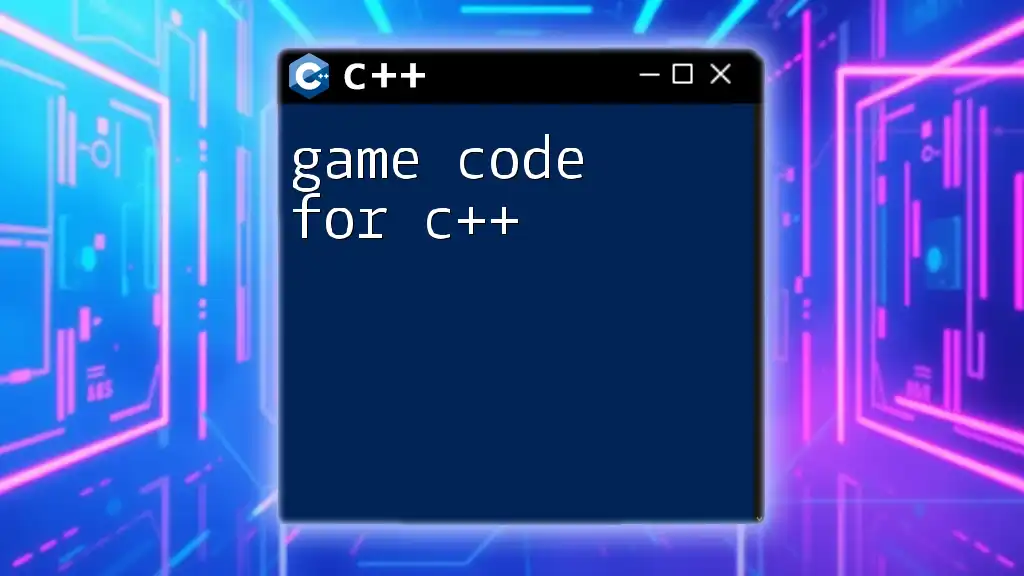
Setting Up CMake
Installation of CMake
To get started with modern CMake for C++, you need to install it. Here are some suitable installation methods based on your operating system:
- Windows: You can install CMake using the installer available on the official CMake website or through package managers like Chocolatey.
- macOS: The easiest method is to use Homebrew:
brew install cmake
- Linux: You can install CMake using APT or another package manager. For Ubuntu, the command is:
sudo apt-get install cmake
Creating a Basic CMake Project
Creating a basic CMake project involves setting up an appropriate directory structure and writing a simple `CMakeLists.txt` file.
-
Directory structure:
MyProject/ ├── CMakeLists.txt └── src/ └── main.cpp
-
Creating the `CMakeLists.txt` file: Here's a simple example of what your `CMakeLists.txt` could look like:
cmake_minimum_required(VERSION 3.10) project(MyProject) add_executable(MyExecutable src/main.cpp)
In this snippet, `cmake_minimum_required` specifies the minimum version required, while `project()` sets the name of the project. The `add_executable()` command defines an executable target built from `main.cpp`.
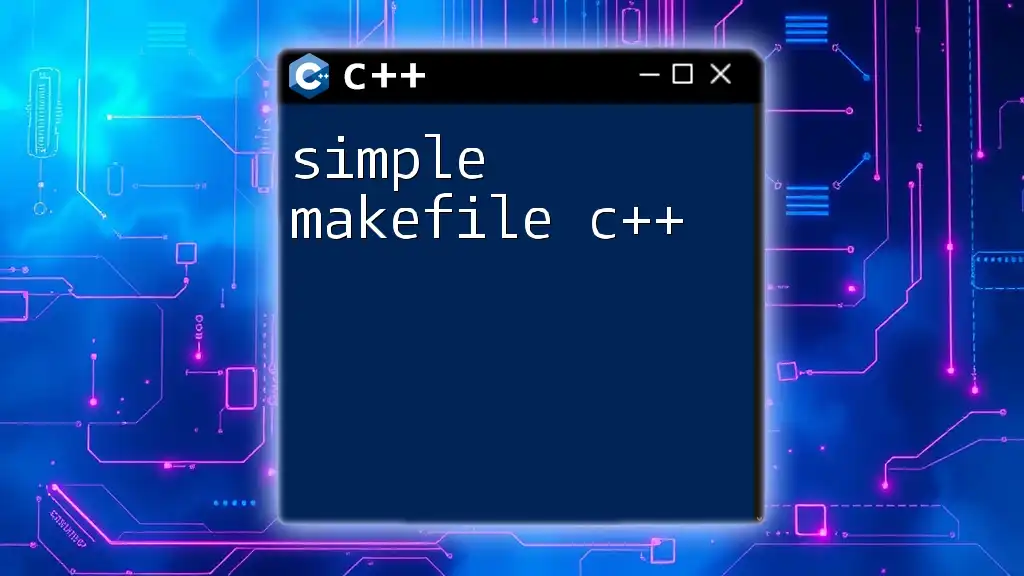
Understanding CMakeLists.txt
Components of CMakeLists.txt
An effective CMake project relies on a well-structured `CMakeLists.txt` file. Understanding its components is crucial.
Project Declaration
Using the `project()` command lets you specify the project's name, version, and the languages used. You can also set properties like:
project(MyProject VERSION 1.0 LANGUAGES CXX)
Specifying Minimum CMake Version
Using `cmake_minimum_required()` ensures your CMake scripts are compatible with your installed CMake version. This is essential as certain features evolve with new releases.
Adding Executables and Libraries
CMake provides commands for creating executables and libraries.
-
Creating an executable:
add_executable(MyExecutable src/main.cpp)
-
Creating a library:
add_library(MyLibrary STATIC src/mylibrary.cpp)
When you define a library, you can create shared or static versions depending on your project needs.
Target Properties
Using `set_target_properties()` allows you to customize various characteristics of your targets. This might include settings for compiler flags, output directory, and C++ standards:
set_target_properties(MyExecutable PROPERTIES
RUNTIME_OUTPUT_DIRECTORY ${CMAKE_BINARY_DIR}/bin
CXX_STANDARD 17
)
Finding Packages
Integrating external libraries can be straightforward with `find_package()`, which finds and configures the necessary libraries.
For example, to link against the Boost library, you can write:
find_package(Boost REQUIRED)
target_link_libraries(MyExecutable Boost::Boost)
In this example, CMake searches for Boost and links it to your executable.
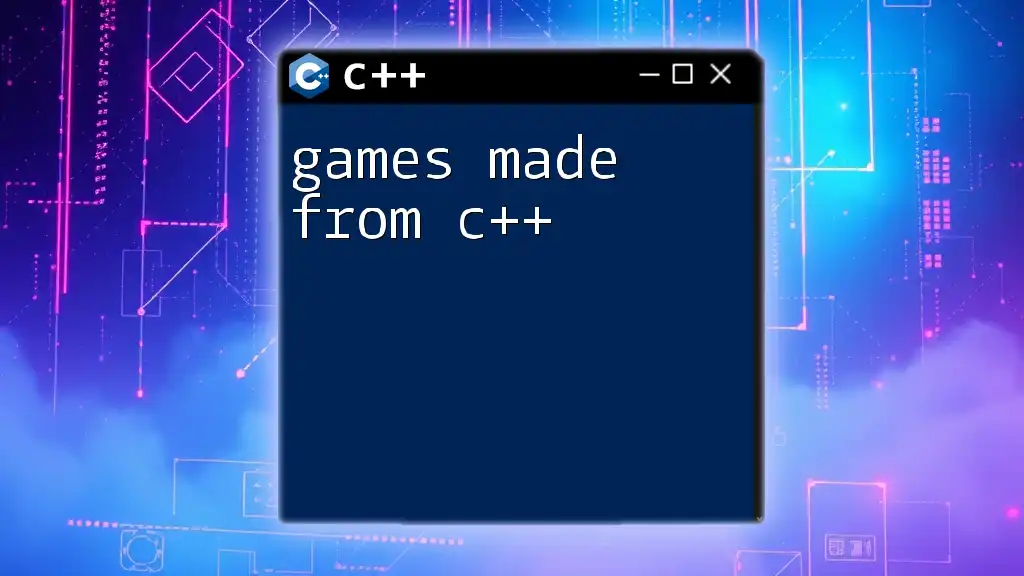
Advanced CMake Usage
CMake Variables and Cache
CMake supports variables, which can significantly simplify and customize your configurations. The `CMakeCache.txt` file retains user-defined variables. You can access and modify these variables to leverage different configurations and options.
CMake Configuration Types
Understanding how to specify build types is vital for managing builds effectively. Typical configurations include Debug, Release, RelWithDebInfo, and MinSizeRel. You can set the build type in your `CMakeLists.txt`:
set(CMAKE_BUILD_TYPE Debug)
This will enable debug symbols and disable optimizations.
Custom Commands and Targets
You can define custom commands in your CMake build system to perform additional tasks. Use `add_custom_command()` for specific actions like file generation or running scripts. Here’s an example:
add_custom_command(
OUTPUT generated_file
COMMAND python script.py
DEPENDS script.py
)
To create a custom target that invokes your executable:
add_custom_target(run ALL COMMAND MyExecutable)
This command will compile your executable and execute it when you run the build.
CMake Functions and Macros
Functions and macros allow you to encapsulate repetitive tasks in a single reusable piece of code. Functions provide variable scoping, whereas macros expand in place. For instance, defining a function to handle custom build logic can streamline your CMake files.
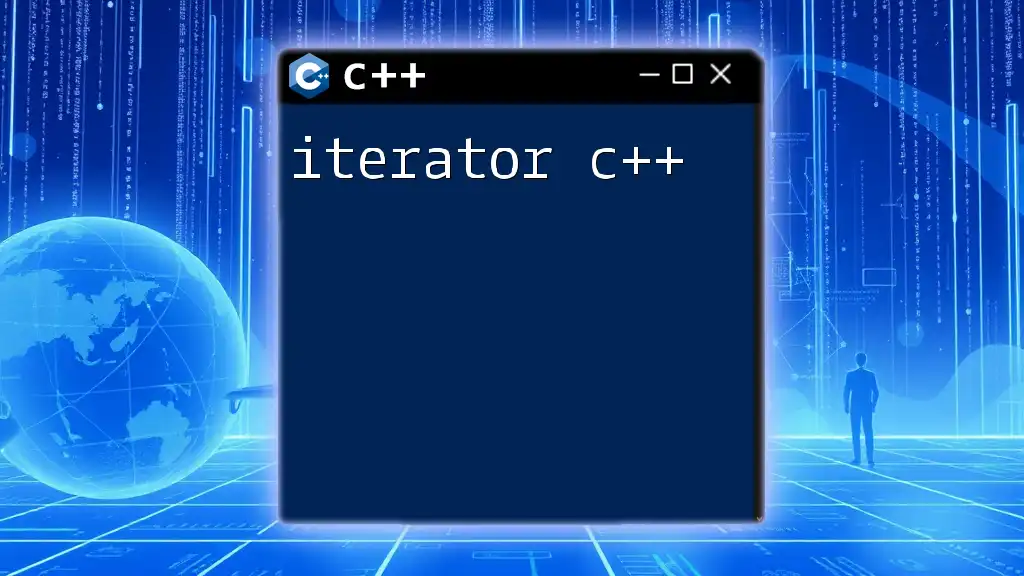
Best Practices for CMake
Organizing CMake Files
As your project scales, organization becomes crucial. Structuring large projects using subdirectories can keep your build configurations manageable. Utilize `include()` to modularize your `CMakeLists.txt` files, extracting common functionalities for maintainability.
Handling Dependencies
Properly managing third-party libraries is fundamental in modern CMake for C++. Use `fetch_content` or the `ExternalProject` module to handle dependencies effectively, ensuring decisions align with project goals.
Cross-Platform Considerations
When developing cross-platform applications, account for differences in file paths, library locations, and compiler specifics. CMake’s built-in functions can help you handle these variations gracefully, enabling a smoother build experience.
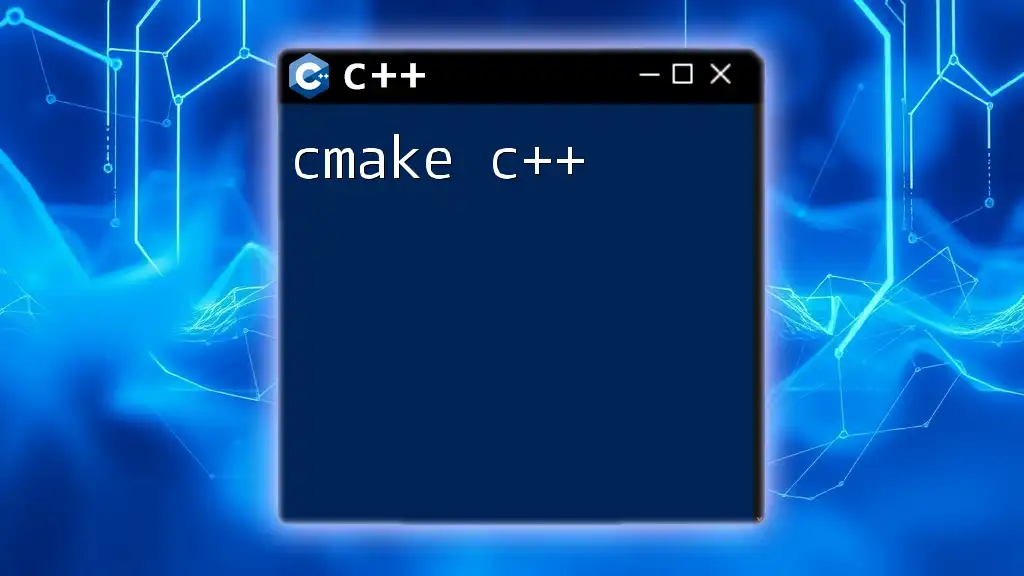
CMake in Continuous Integration (CI)
Integrating CMake with CI/CD Tools
Modern development often includes automated building and testing processes. Integrating CMake with CI/CD platforms such as GitHub Actions, Travis CI, or GitLab CI can streamline your workflow. Create YAML configuration files that define the build process using CMake.
Running Tests with CMake
Embedding testing frameworks into your CMake projects enhances code reliability. For instance, integrating Google Test with CMake is straightforward:
enable_testing()
add_subdirectory(test)
This configuration turns your tests into targets within the build system, allowing for automated testing.
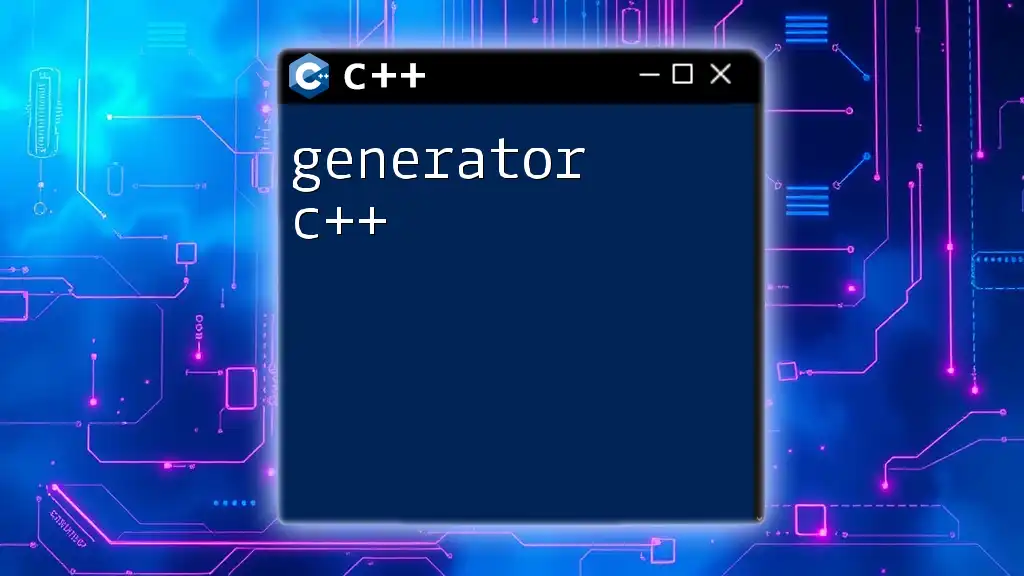
Conclusion
The Future of CMake in C++
Mastering modern CMake for C++ development will place you at the forefront of software engineering practice. Its evolving features and community support indicate a bright future, making it essential for aspiring developers to gain proficiency in CMake.
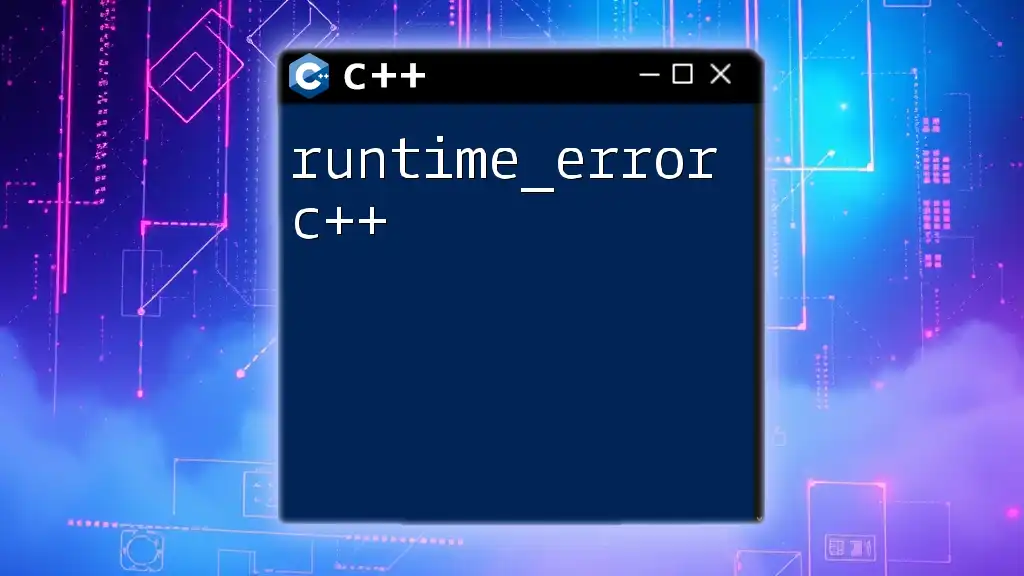
Additional Resources
Recommended Books and Documentation
For further learning, check out the official CMake documentation and consider picking up books that delve into advanced CMake techniques and project management.
Community and Forums
Engaging with the wider C++ and CMake community through forums, Q&A sites, and discussion boards can provide insights and assistance as you expand your understanding of this powerful tool.