C++ is a powerful programming language widely used in the gaming industry for developing high-performance games due to its speed and efficiency.
Here's a simple example of how you might use C++ to create a basic game loop:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
bool isRunning = true;
while (isRunning) {
// Game logic here
std::cout << "Game is running!" << std::endl;
// Simulate frame timing
std::this_thread::sleep_for(std::chrono::milliseconds(100));
// For demonstration, we'll stop the loop after a few iterations
static int count = 0;
if (++count > 10) isRunning = false;
}
return 0;
}
The Importance of C++ in Game Development
C++ is a cornerstone in the gaming industry for multiple critical reasons:
Performance: One of the main advantages of C++ is its ability to execute complex algorithms and graphics rendering quickly. Performance-centric features allow developers to create games that push hardware boundaries, making C++ particularly popular for AAA titles.
Memory Management: Unlike many higher-level programming languages, C++ gives developers fine-grained control over memory allocation. The use of pointers and the ability to manipulate memory directly allows for optimized memory usage, crucial in resource-intensive environments like video games.
Additionally, C++ embodies object-oriented programming principles that facilitate modular and scalable game design. This means developers can create robust, maintainable, and understandable game architectures, which is especially advantageous when collaborating on large projects.
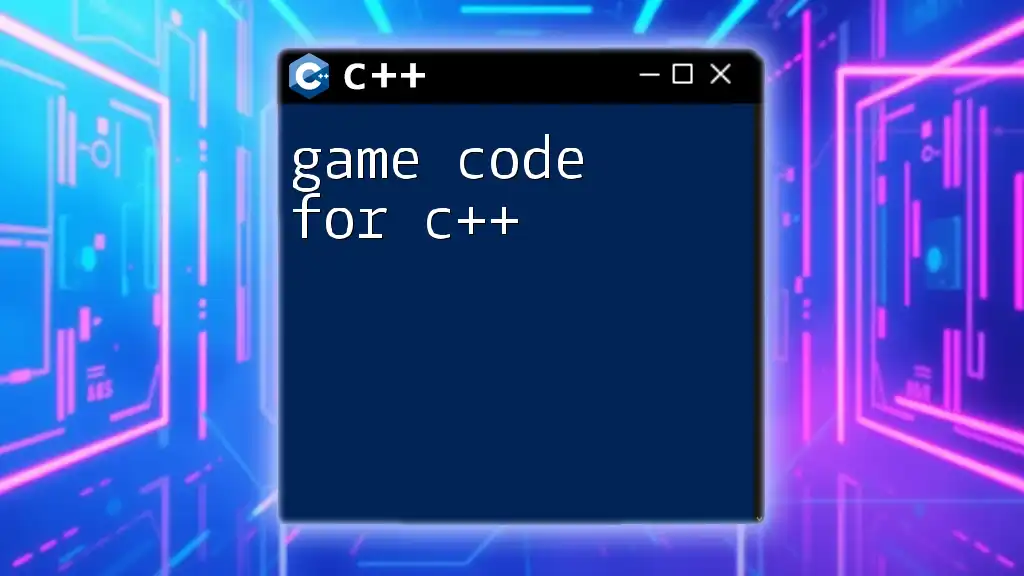
Popular Games Made with C++
A range of widely recognized games, spanning both AAA and indie sectors, showcases the flexibility and power of the language.
AAA Titles
When discussing games made from C++, it’s impossible not to mention some of the remarkable AAA games that have set industry standards:
-
The Witcher 3: Wild Hunt: This critically acclaimed RPG utilizes the REDengine, which is primarily written in C++. The complexity of its world, character interactions, and stunning graphics all benefit from C++'s high-performance capabilities.
-
Call of Duty series: Renowned for its fast-paced action and lifelike graphics, the Call of Duty franchise relies heavily on C++ to manage massive online multiplayer environments and intricate rendering techniques.
Indie Games
C++ isn't just for large-scale projects; it also holds a significant place in the indie game scene:
-
Celeste: This platformer demonstrates how C++ can power intricate mechanics and graphics without requiring an enormous budget. Its tight controls and sophisticated physics engine are great examples of what can be achieved with C++.
-
Fez: A unique puzzle-platformer, Fez showcases cross-platform compatibility, highlighting C++'s ability to integrate with various systems efficiently.
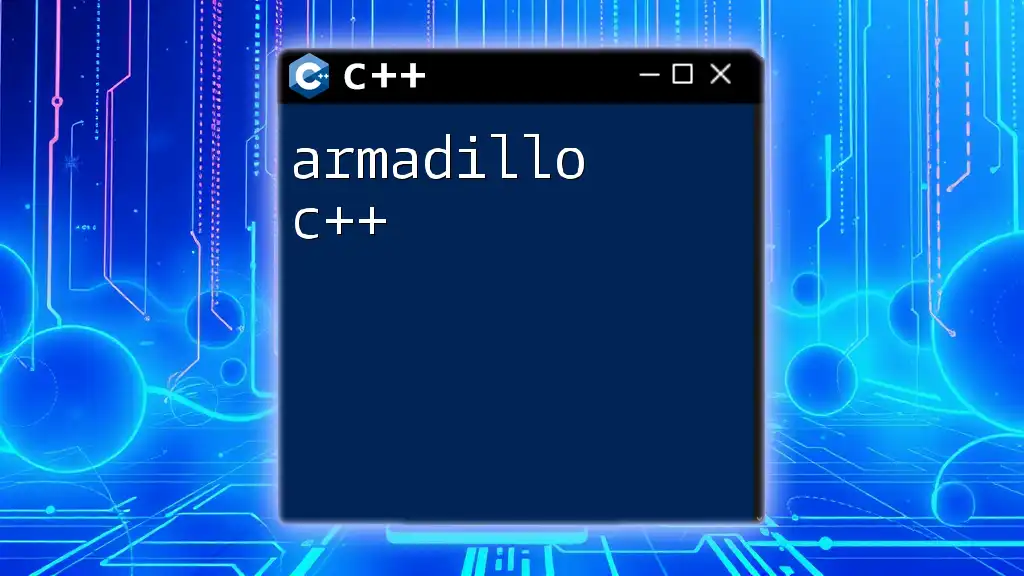
Game Engines Built with C++
C++ also plays a pivotal role in the creation of many game engines that developers use:
Unreal Engine
Unreal Engine, one of the most popular game engines in the world, is primarily built with C++. It offers extensive tools for visual scripting and is leveraged for photorealistic graphics and complex game mechanics.
For example, here's a simple C++ snippet demonstrating how to spawn an object in Unreal Engine:
void AMyActor::SpawnMyObject()
{
FVector SpawnLocation = GetActorLocation() + FVector(0, 0, 50);
FRotator SpawnRotation = FRotator(0, 0, 0);
GetWorld()->SpawnActor<AMySpawnedObject>(MySpawnedClass, SpawnLocation, SpawnRotation);
}
CryEngine
CryEngine is another powerful game engine that leverages C++. Known for its stunning visual fidelity, it gives developers the tools to create realistic environments and physics.
Unity (with C++)
Unity may primarily use C#, but it allows developers to implement performance-critical components using C++. This combination provides the best of both worlds: ease of development and high-performance execution when necessary.
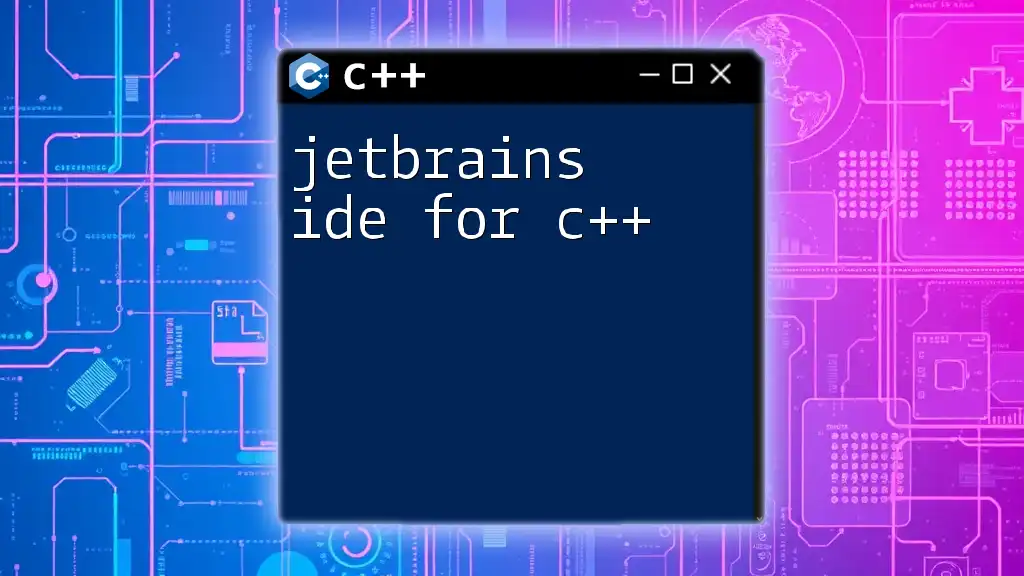
Developing a Simple Game: A Practical Example
Understanding the essence of games made from C++ often involves actual practice. Let's create a simple game—a Pong clone—to illustrate.
Choosing an IDE and Setting Up
Developers can choose various IDEs like Visual Studio or Code::Blocks to set up their projects efficiently. Both of these tools are beginner-friendly and offer robust support for C++ game development.
Sample Game: Pong Clone
Game Design Overview: The classic Pong game involves two paddles and a ball, where players attempt to score by getting the ball past their opponent.
Code Snippet: Initial Setup
Starting with a simple console output, let’s begin with the basic structure:
#include <iostream>
using namespace std;
int main()
{
cout << "Welcome to Pong!" << endl;
// Initialize game elements here
return 0;
}
Implementing Game Logic
Next, you'd move into player movement logic and collision detection. Each player would control a paddle, so handling input effectively is essential. You could use keyboard input to move the paddles up and down, checking for key presses each frame.
Rendering with a Graphics Library
To render graphics, we might integrate SDL (Simple DirectMedia Layer). Here’s how to set up the SDL window:
#include <SDL.h>
int main(int argc, char* argv[])
{
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Pong", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, 0);
// Main loop and rendering here
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
This simple block initializes the SDL library and creates a window where our game will be displayed. The main loop would handle rendering, input, and game updates.
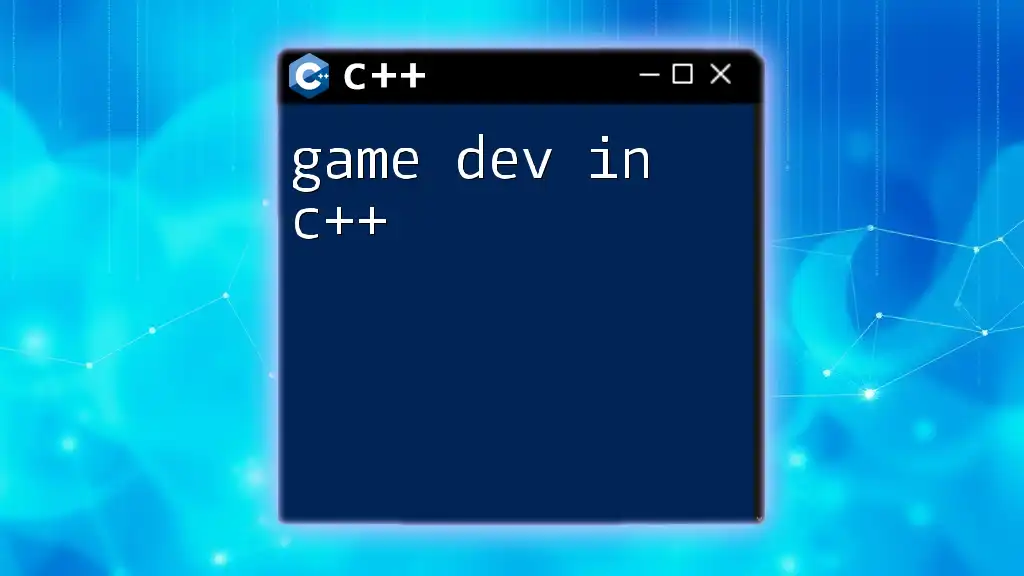
Benefits of Learning C++ for Game Development
Grasping C++ offers numerous advantages for aspiring game developers:
Skill Enhancement: Learning C++ opens doors to job opportunities in a competitive industry. It is often a requirement for positions in game development, especially with companies that develop AAA titles.
Versatility and Control: C++ provides greater control over hardware and performance optimizations, making it an ideal choice for developers striving to elevate game experiences.
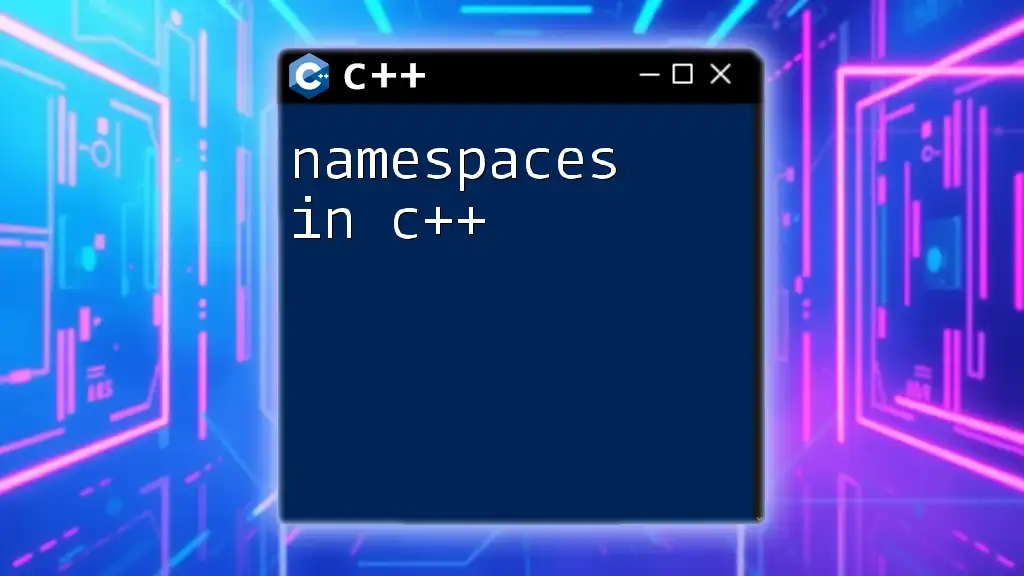
Conclusion
In conclusion, games made from C++ highlight the language's versatility, performance, and extensive capabilities in crafting advanced gaming experiences. By engaging with C++, not only can developers create high-performing games, but they can also explore vital career paths in a dynamic industry. Embracing the power of C++ can be a game-changer on both a personal and professional level.
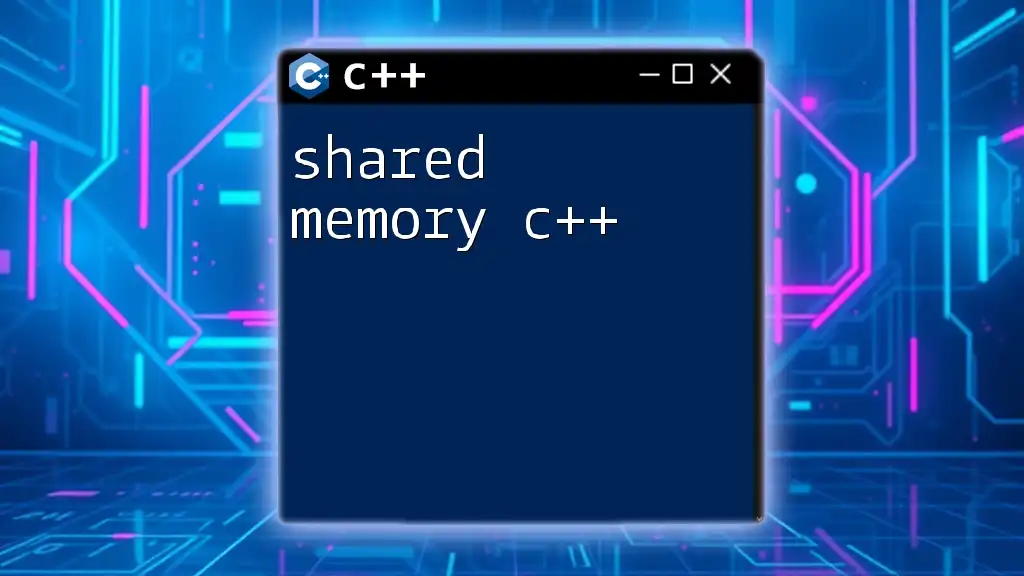
Call to Action
We encourage our readers to share experiences, insights, or queries regarding their journey into game development using C++. Let’s build a community where we can support and learn from each other!
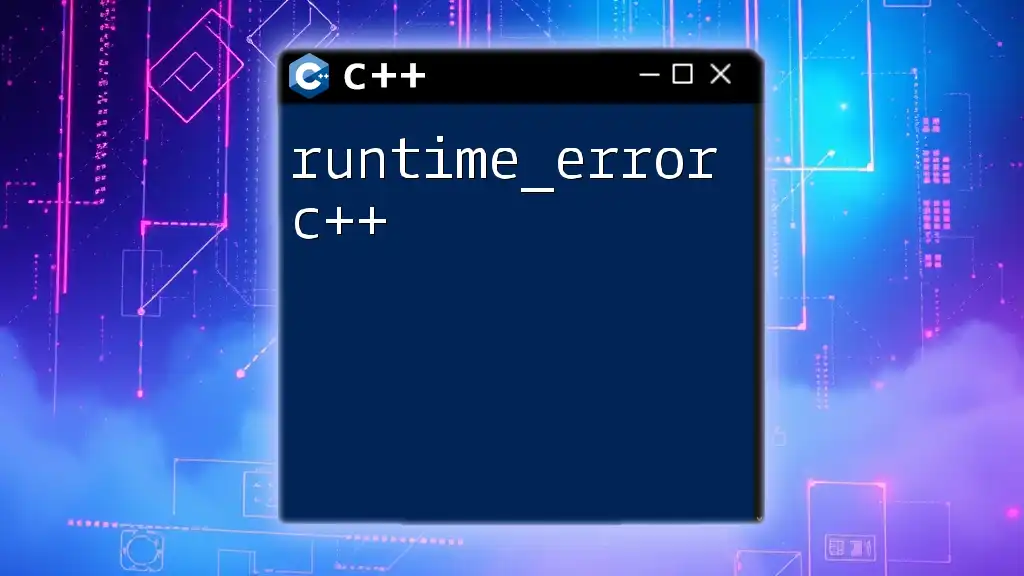
Additional Resources
For further learning, check out various tutorials, online courses, and forums dedicated to mastering C++ in game development. Expand your knowledge and practical skills to thrive in this exciting field!