A simple Makefile for a C++ project automates the build process by defining rules for compiling source files into an executable.
# Makefile
CC = g++
CFLAGS = -Wall -g
TARGET = my_program
SOURCES = main.cpp utils.cpp
$(TARGET): $(SOURCES)
$(CC) $(CFLAGS) -o $(TARGET) $(SOURCES)
clean:
rm -f $(TARGET)
What is a Makefile?
A Makefile is a special format file used to control the build process of a project in a systematic and efficient way. It defines how to compile and link the program’s source code. The importance of Makefiles in C++ project management cannot be overstated; they simplify the complex process of building applications by automating compilation commands that ensure all source files are updated and compiled correctly.
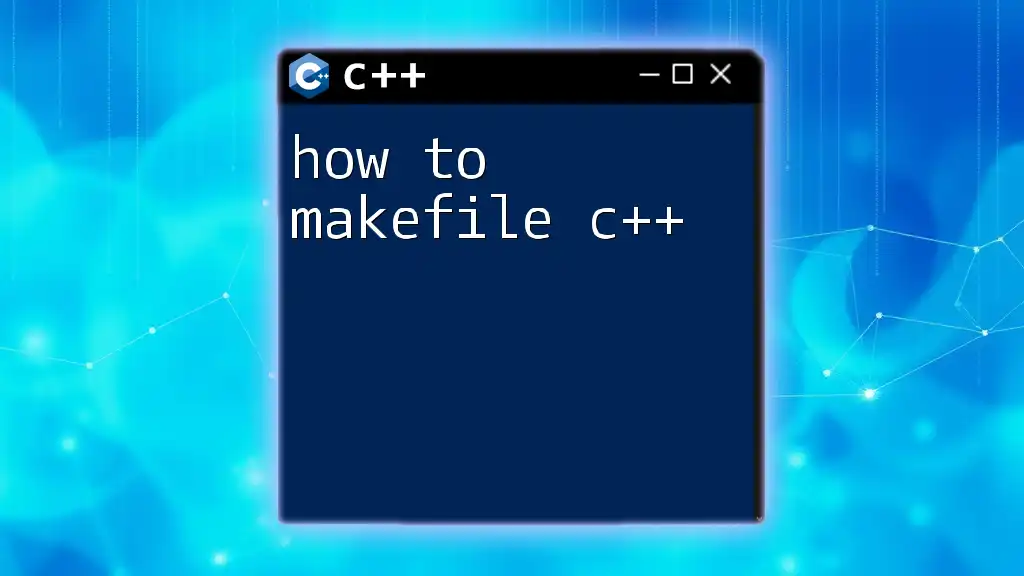
Why Use Makefiles?
Using Makefiles offers several advantages:
- Automation: Makefiles save time by automating the compilation process. Once it’s set up, developers can simply type `make` in the terminal to compile their code.
- Dependency Management: They automatically track which files have changed and only recompile those files, thus minimizing unnecessary build time.
- Clarity: A well-structured Makefile clearly outlines project structure and build rules, making it easier for teams to understand how a project is organized.
Makefiles are prevalent in real-world scenarios, particularly in large-scale software development where managing multiple files and dependencies becomes challenging.
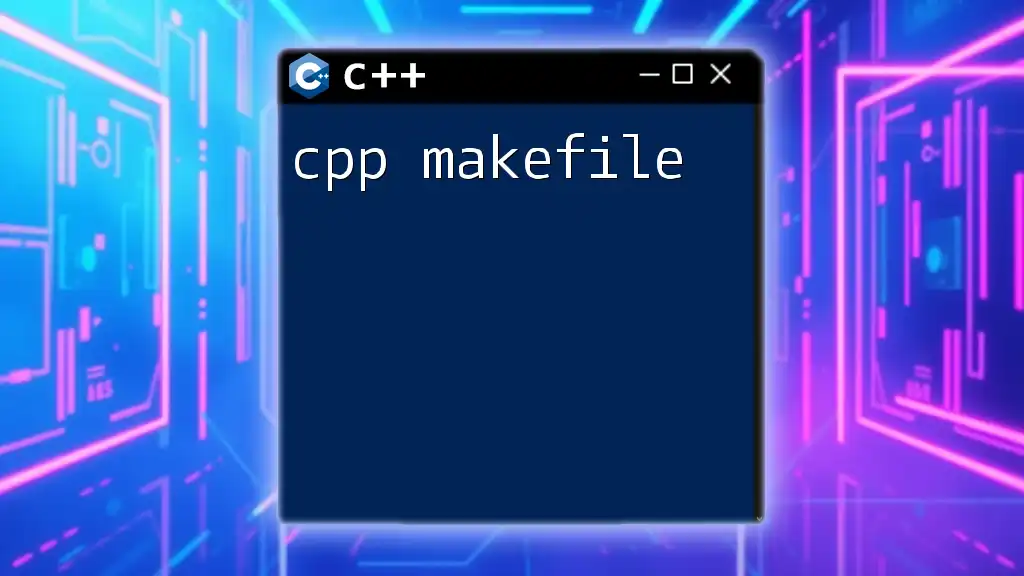
Components of a Makefile
Basic Structure of a Makefile
The basic syntax of a Makefile consists of rules, targets, dependencies, and commands. A typical rule looks like this:
target: dependencies
command
- Target: It is the file that is generated or built (e.g., a binary executable).
- Dependencies: These are the files that the target relies upon (e.g., source files).
- Command: This is what will be executed to produce the target (usually a compilation command).
Example of a Simple Makefile
Here’s an example of a simple Makefile:
# A simple Makefile example
all: main
main: main.o utils.o
g++ -o main main.o utils.o
main.o: main.cpp
g++ -c main.cpp
utils.o: utils.cpp utils.h
g++ -c utils.cpp
clean:
rm -f main *.o
In this example, the `all` target is set to build the `main` target. The `main` target depends on `main.o` and `utils.o`, which are compiled from their respective source files. The `clean` target provides a way to remove the compiled files, ensuring a fresh build next time.
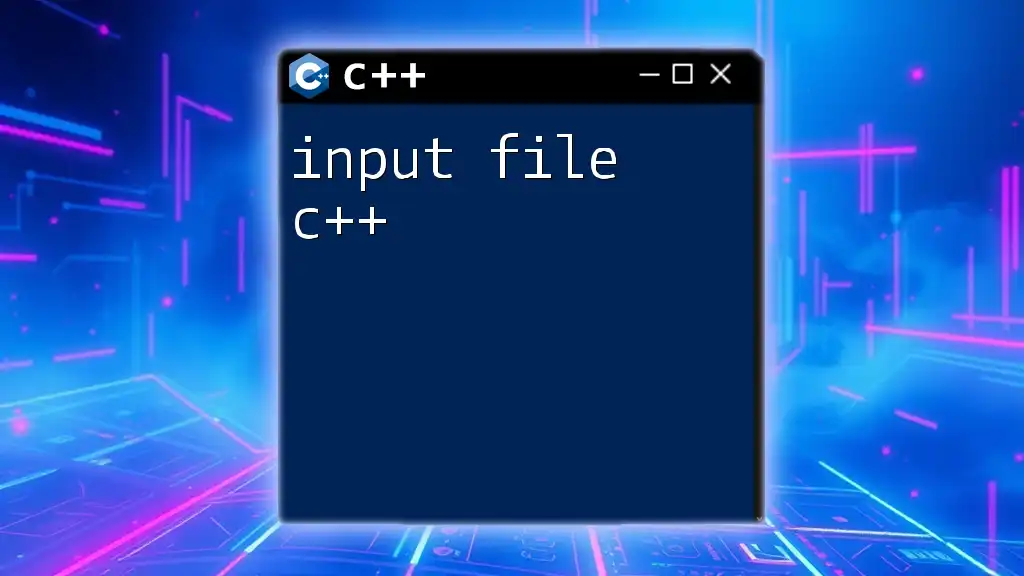
Writing Your First Makefile
Step-by-Step Guide
To create your first Makefile, follow these steps:
- Set up your project directory with a clear structure, placing your `.cpp` files and a `Makefile` in it.
- Create a new file named `Makefile` in your project root; Makefile names are case-sensitive.
- Define your targets and their dependencies, as shown in the previous example.
Common Makefile Commands
Here are some commonly used commands:
- `make`: This command builds your project according to the default target specified in the Makefile—in this case, it will compile the `main` target.
- `make clean`: This command executes the `clean` target, helping you remove all the object files and binaries that were created during the build process.
- `make [target]`: You can specify a particular target to build if you do not want to compile the whole project (e.g., `make main`).
Tips for Writing Clean and Efficient Makefiles
- Commenting for Clarity: Use comments (`# This is a comment`) to explain what each part of the Makefile does to improve readability.
- Using Variables for Scalability: Introduce variables for commonly used commands, which allows you to make future changes more easily.
- Avoiding Hardcoding Paths: Instead of hardcoding file paths, use variables to make your Makefile adaptable to different project structures.
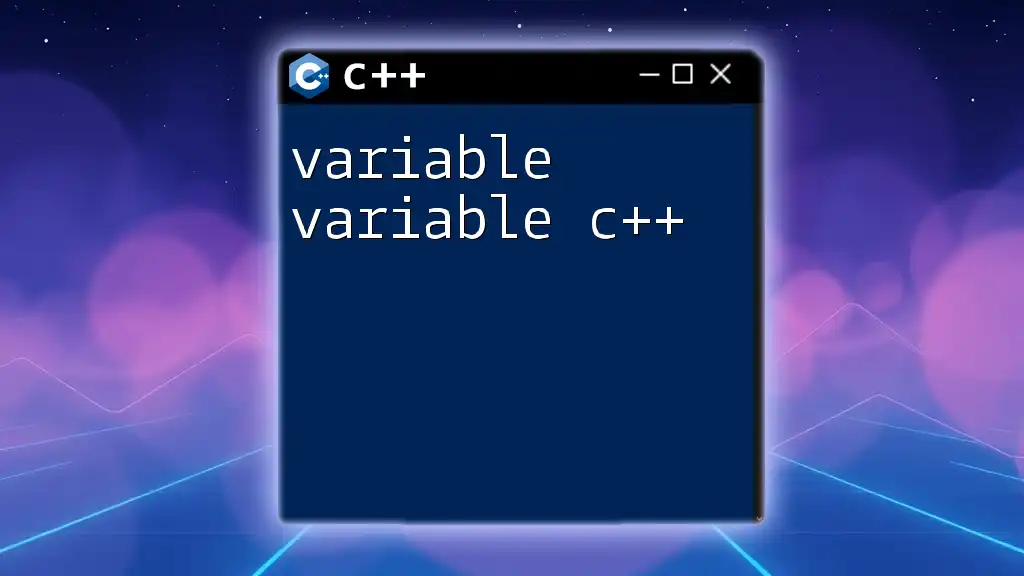
Advanced Makefile Techniques
Using Variables in Makefiles
Variables in Makefiles serve as placeholders for values that improve maintainability. Here’s how you can use them:
CC = g++
CFLAGS = -c -Wall
LDFLAGS =
all: main
main: main.o utils.o
$(CC) -o $@ $^ $(LDFLAGS)
%.o: %.cpp
$(CC) $(CFLAGS) $<
In this example, `CC`, `CFLAGS`, and `LDFLAGS` are variables that store the compiler, compilation flags, and linker flags, respectively. Using the `$@` and `$^` automatic variables makes it easy to refer to the target and prerequisites.
Phony Targets
A phony target is essentially a label for a recipe to be executed without being associated with a file. Using `.PHONY` helps prevent conflicts with files of the same name. Here’s an example:
.PHONY: all clean
clean:
rm -f *.o main
By declaring `clean` as a phony target, Make will execute it every time you type `make clean`, irrespective of file existence.
Pattern Rules
Pattern rules provide a concise way to specify how to make multiple targets using a single rule template, reducing redundancy. Consider this example:
%.o: %.cpp
$(CC) $(CFLAGS) $<
This rule specifies how to compile any `.cpp` file into a corresponding `.o` file, streamlining the build process for larger projects.
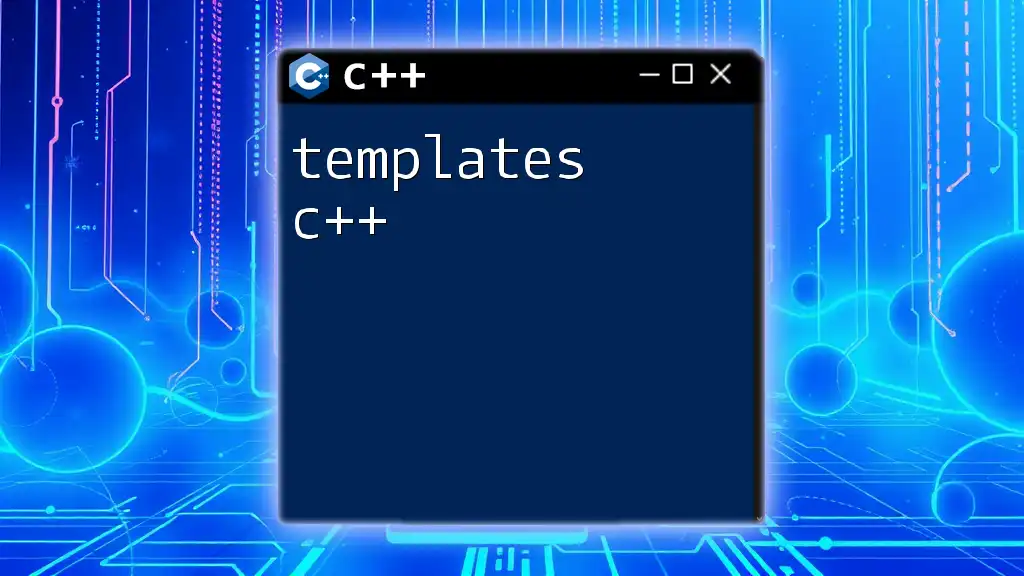
Debugging Makefiles
Common Errors in Makefiles
When working with Makefiles, syntax errors can lead to confusing build failures. Common pitfalls include:
- Missing colons: Errors can occur if you forget the colon after specifying a target.
- Incorrect assumptions about dependencies: Failing to specify a dependency leads to not recompiling the necessary files.
Debugging Tips
To debug Makefiles effectively, consider the following tips:
- Use `make -n`: This command performs a dry run, showing which commands would be executed without actually running them. This is useful for verifying that the correct commands are set to run.
- Check for lost dependencies: Make sure that all prerequisites are correctly defined to avoid unexpected behavior.
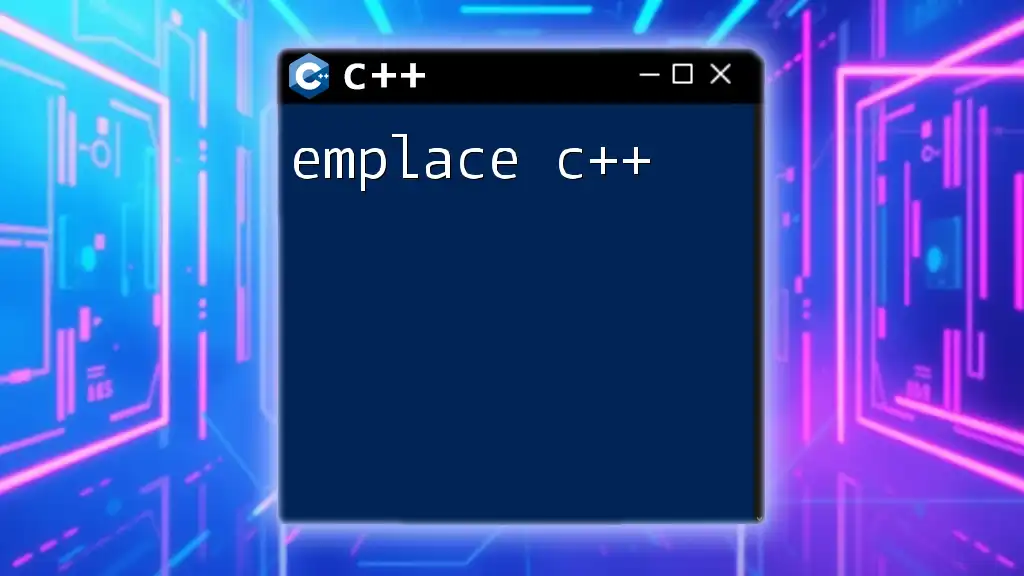
Best Practices for Using Makefiles in C++
Organizing Large Projects
For larger C++ projects, structuring your Makefile properly is crucial. Keep related source files in subdirectories and use recursive Makefiles if necessary, or handle multiple directories with a single Makefile by specifying paths.
Documentation and Comments
Keeping your Makefile maintainable means adding comments that explain the rationale behind complex parts of your script. Documentation makes it easier for you and others to modify the Makefile in the future, facilitating smoother collaboration within development teams.
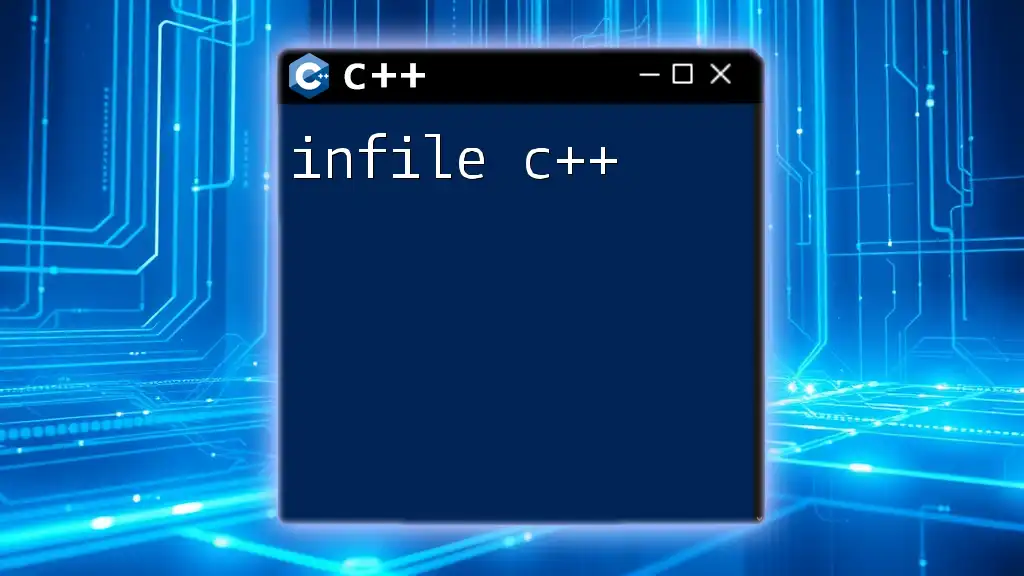
Conclusion
To summarize, a simple makefile c++ provides a streamlined and automated way to manage the build process of your C++ projects. Understanding its components, employing advanced techniques, and adhering to best practices will enhance your development experience.
Further Resources
For a more in-depth understanding, consider referring to official documentation and community forums, where you can explore advanced Makefile functionalities and solarize best practices within the context of C++ development.
Get Started with Your Own Makefile
Now that you have a comprehensive understanding of Makefiles, experiment with building your own! Start small, build your projects, and watch as your efficiency in C++ development skyrockets. If you have questions or would like to share your experiences, feel free to leave a comment below!