A Makefile is a simple way to manage the build process of C++ projects, automating the compilation of multiple files with a specified set of commands.
Here’s a basic example of a Makefile:
# Makefile for a simple C++ project
CC=g++
CFLAGS=-Wall -g
SOURCES=main.cpp utils.cpp
OBJECTS=$(SOURCES:.cpp=.o)
EXECUTABLE=my_program
all: $(EXECUTABLE)
$(EXECUTABLE): $(OBJECTS)
$(CC) $(OBJECTS) -o $@
.cpp.o:
$(CC) $(CFLAGS) -c $< -o $@
clean:
rm -f $(OBJECTS) $(EXECUTABLE)
Understanding Makefiles
What is a Makefile?
A Makefile is a simple way to organize and manage the compilation of programs. It allows developers to automate the build process, specifying how to compile and link different components of your software project. It acts as a configuration file for the `make` utility, which reads the Makefile to execute commands for building your applications.
Why Use a Makefile in C++ Projects?
Using a Makefile significantly simplifies the build process, especially in larger projects. When you don’t use a Makefile, you may find yourself manually compiling each source file and tracking dependencies. This can become tedious and error-prone as your project grows. With a Makefile, you can automate compilation, ensuring that only the necessary files are rebuilt when changes are made, thus saving time and reducing frustration.
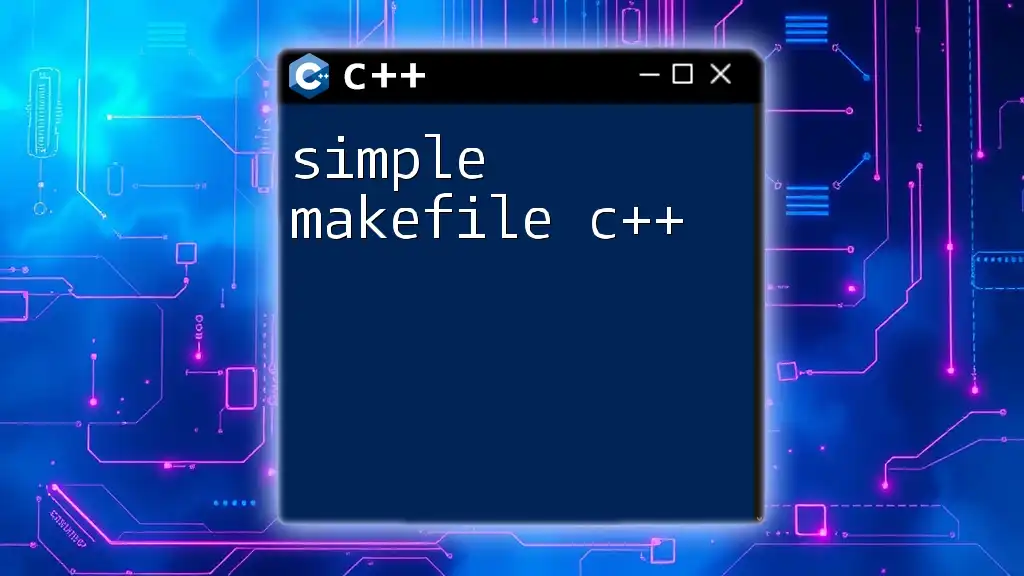
Creating a Simple Makefile for C++
Basic Structure of a Makefile
The structure of a Makefile consists of targets, dependencies, and commands.
- Targets: Typically represent the output files or actions to be taken.
- Dependencies: These are the files that must be updated before the target can be generated.
- Commands: The actual commands executed to generate the target, prefixed by a tab space.
This organization allows you to manage complex builds succinctly.
Example C++ Makefile
Here’s a simple example of a Makefile for a C++ project:
# Simple Makefile Example
CC = g++
CFLAGS = -Wall -g
TARGET = my_program
SOURCES = main.cpp utils.cpp
OBJECTS = $(SOURCES:.cpp=.o)
all: $(TARGET)
$(TARGET): $(OBJECTS)
$(CC) $(OBJECTS) -o $(TARGET)
%.o: %.cpp
$(CC) $(CFLAGS) -c $< -o $@
Explanation of the Example
In this Makefile:
- `CC` defines the compiler being used (in this case, `g++`).
- `CFLAGS` specifies the compiler flags, such as `-Wall` for enabling all warnings and `-g` for generating debug information.
- `TARGET` represents the output file that is generated when the build is successful.
- `SOURCES` lists the C++ source files, while `OBJECTS` transforms those source file names from `.cpp` to `.o` (object files).
The `all` target is the default target that gets built first. It calls the `TARGET`, which depends on the object files. The command for linking (`$(CC) $(OBJECTS) -o $(TARGET)`) is executed after compiling all object files.
The rule for compiling an object file is defined using a pattern rule (`%.o: %.cpp`), simplifying the build process by applying the same quality to each source file.
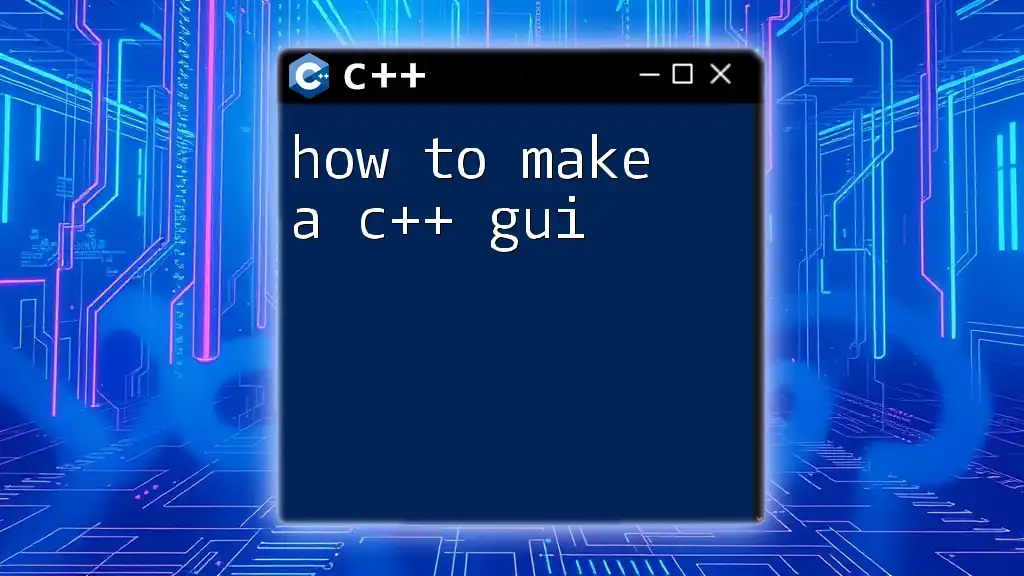
Advanced Makefile Features
Using Variables and Functions
Makefiles support variables, allowing you to reduce repetition and make your Makefile easier to maintain. For example, if you want to change the compiler or flags later, you will only need to update the variable definitions.
# Using variables for easy modifications
CC = g++
CFLAGS = -Wall -O2
Pattern Rules
Pattern rules can enhance readability and efficiency. Instead of writing repetitive rules for each source file, you can use a pattern rule to handle them collectively.
# Example of a pattern rule
%.o: %.cpp
$(CC) $(CFLAGS) -c $< -o $@
This rule states that any `.o` file depends on its corresponding `.cpp` file, allowing Make to infer the commands to compile object files directly.
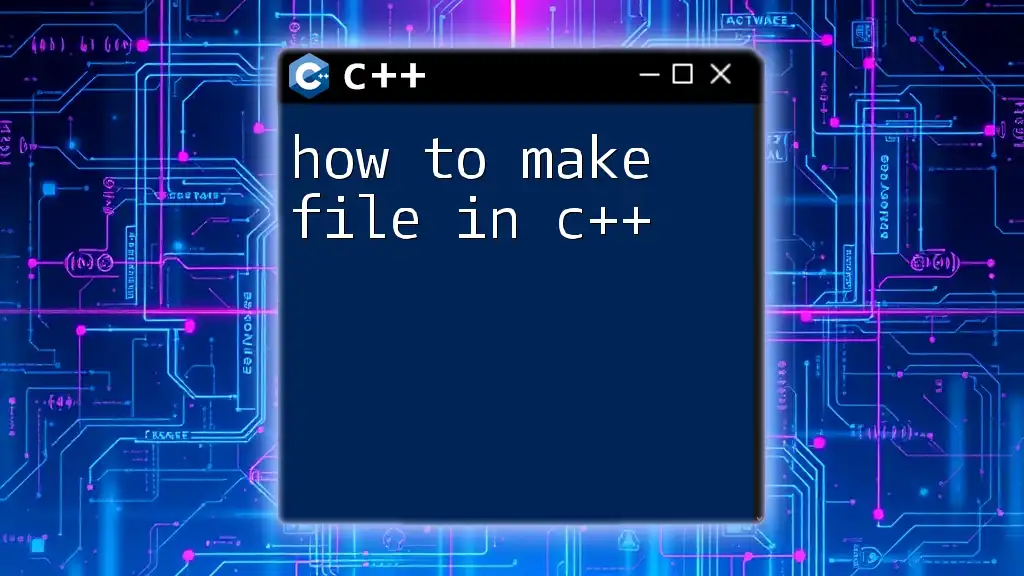
Including Libraries and External Dependencies
Linking with Libraries
Linking libraries increases the power of your applications. You can specify external libraries directly in your Makefile. For instance, if you want to link against the math library, you add it like this:
LIBS = -lm
$(TARGET): $(OBJECTS)
$(CC) $(OBJECTS) -o $(TARGET) $(LIBS)
Managing External Dependencies
Using libraries often introduces dependencies. To simplify this process, many developers utilize tools like `pkg-config`, which can help query the necessary compiler and linker flags for libraries. By integrating `pkg-config` into your Makefile, you can efficiently manage those dependencies.
LIBS = $(shell pkg-config --cflags --libs mylib)
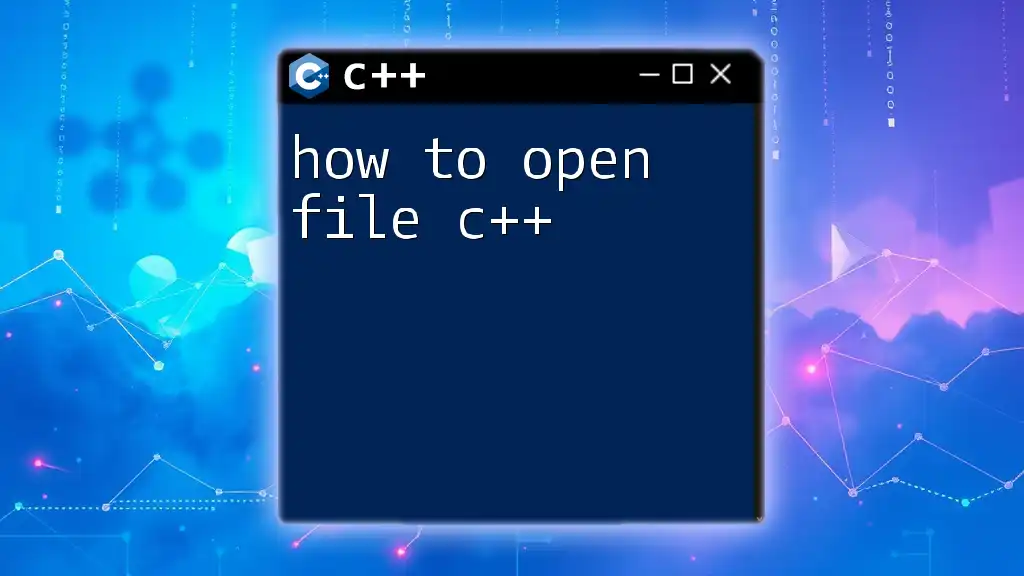
Tips for Writing Efficient Makefiles
Avoiding Common Pitfalls
Common mistakes in Makefiles can lead to inefficient builds. Circular dependencies, for instance, can cause an infinite loop. Always ensure that your target files correctly depend on their prerequisites without looping back on themselves.
Another useful convention is to declare non-file targets using `.PHONY`, which prevents `make` from confusing them with files:
.PHONY: clean
clean:
rm -f $(OBJECTS) $(TARGET)
Best Practices for Maintainability
To enhance maintainability:
- Comment your Makefile liberally, explaining the purpose of each section.
- Organize large Makefiles by breaking them into separate files and using `include` directives to manage complexity.
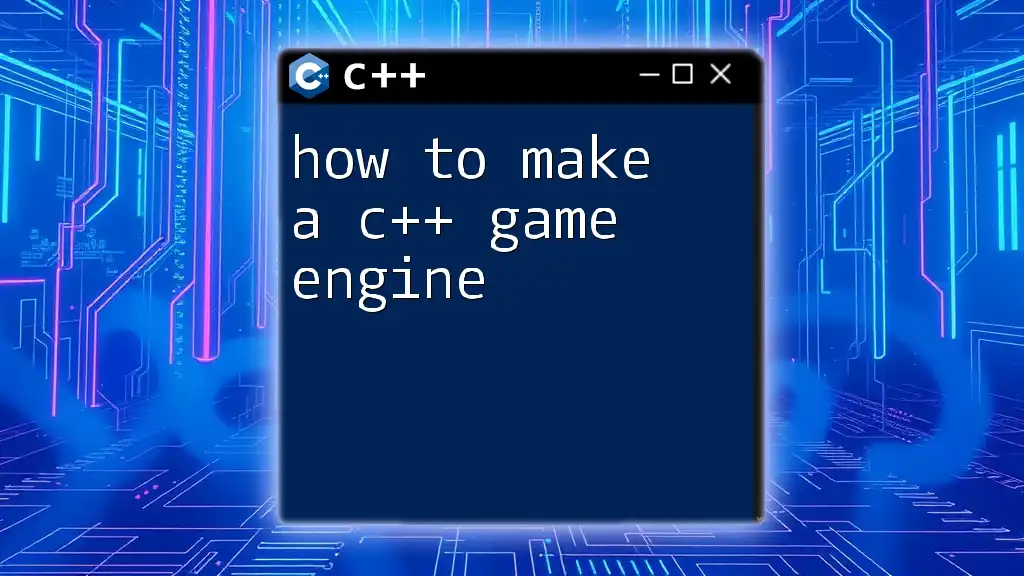
Testing and Debugging Your Makefiles
Running Your Makefile
To execute your Makefile, run the command `make` in the terminal. The default target (first target defined) will be processed, compiling the project. You can also specify targets directly:
make clean
This command removes generated files, allowing you to regenerate your build from scratch.
Debugging Tips
When you encounter issues, use the verbose flag for more detailed output:
make VERBOSE=1
This helps identify the commands that `make` is executing, thus facilitating faster troubleshooting.
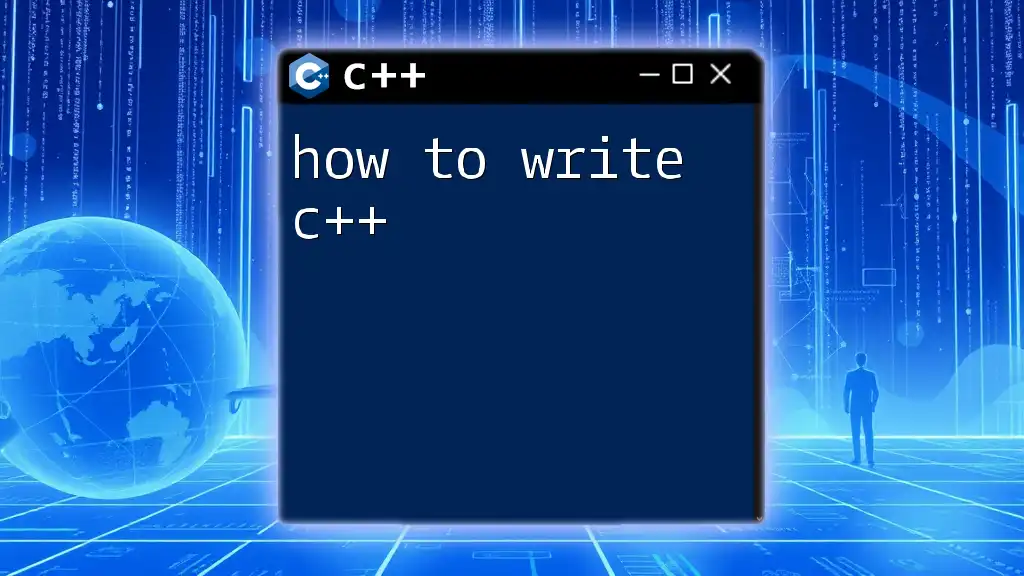
Conclusion
Recap of Important Concepts
Throughout this guide, we’ve explored how to create a Makefile for C++, covering basic structures, advanced features, and best practices. Makefiles streamline your build process, especially in larger projects by managing dependencies and automating tasks.
Additional Resources
For more in-depth information, refer to the GNU Make documentation and other resources available online. There are also various tools available for visualizing and managing complex Makefile scenarios.
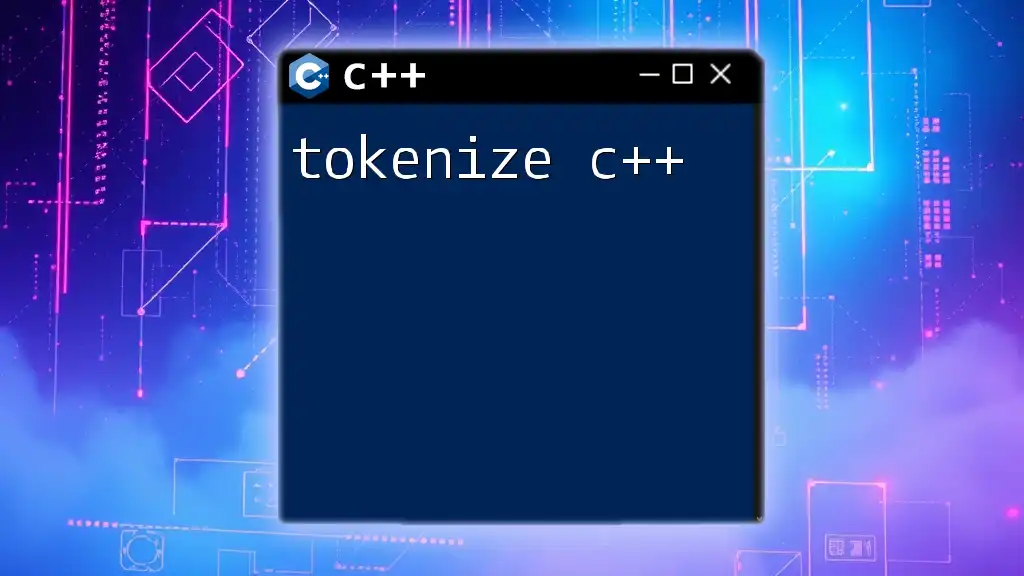
Call to Action
Don’t hesitate to implement Makefiles in your C++ projects! Start small, experiment, and gradually apply more advanced concepts as you grow more comfortable. Share your experiences and improvements with the community, and together we can enhance our understanding and use of Makefiles in C++ development.