To open C++ files for editing or compilation, you can use a text editor or an Integrated Development Environment (IDE), and in the command line, you can open it with the following command:
g++ -o output_file_name source_file.cpp
Understanding C++ Files
What are C++ Files?
C++ files are essential components of a C++ programming project. They are used to write and organize C++ code, which can then be compiled and executed. The most common file extensions you'll encounter include:
- `.cpp`: This extension denotes a C++ source file that contains the code implementation.
- `.h`: Header files, often used to declare functions, classes, and variables.
- `.hpp`: Similar to header files but specifically for C++.
Structure of C++ Files
To effectively understand how to open C++ files, it’s also beneficial to know their typical structure. Generally, a C++ file consists of:
- Preprocessor directives such as `#include` to include libraries, which are necessary for your code to function.
- Function definitions, including the `main()` function, which serves as the entry point for any C++ program.
Here's an example structure of a simple C++ file:
// Example of a C++ file structure
#include <iostream> // Includes the I/O library
int main() {
std::cout << "Hello, World!" << std::endl; // Outputs text to console
return 0; // Returns 0 to indicate success
}
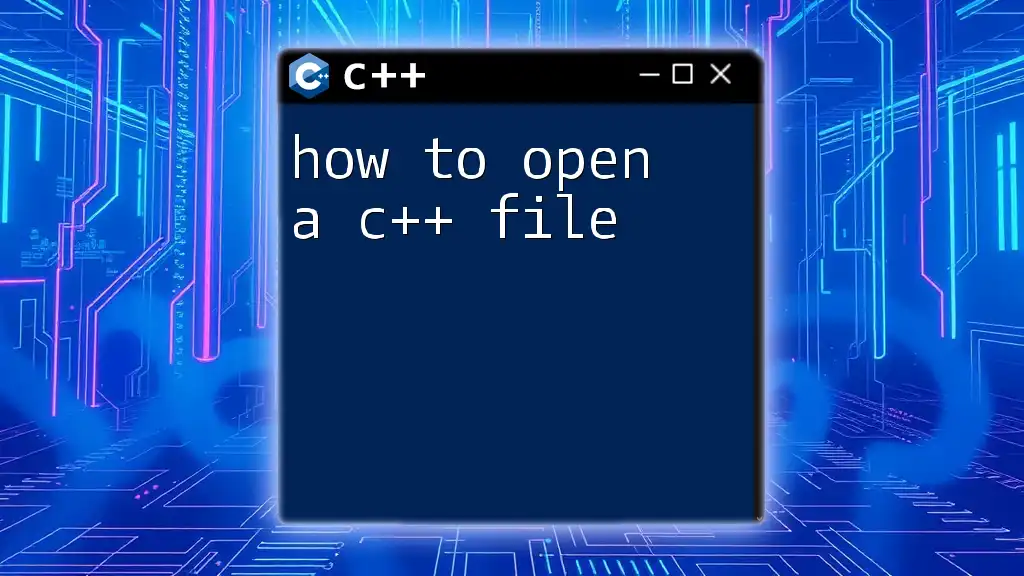
Basic Methods to Open C++ Files
Using a Text Editor
Text editors are lightweight applications suitable for opening and editing C++ files. Here’s how you can open a C++ file:
- Choose a text editor: Options like Visual Studio Code, Sublime Text, or Notepad++ are popular due to their features.
- Install the editor and launch it.
- Open the C++ file:
- In Visual Studio Code, navigate to `File -> Open File...` or press `Ctrl + O`, then select your `.cpp` file.
> Tip: Make sure to explore the editor settings to optimize your coding environment for better productivity!
Using an Integrated Development Environment (IDE)
An IDE offers a comprehensive environment for writing, compiling, and debugging C++ code. IDEs like Code::Blocks, CLion, or Eclipse are all designed to simplify the coding process. Here’s a step-by-step guide to open a C++ file in Code::Blocks:
- Launch Code::Blocks.
- Navigate to `File -> Open...`
- Select your `.cpp` or `.h` file from the file dialog, then click Open.
Using an IDE generally enhances your coding experience because they typically provide features such as auto-completion, error checking, and debugging tools.
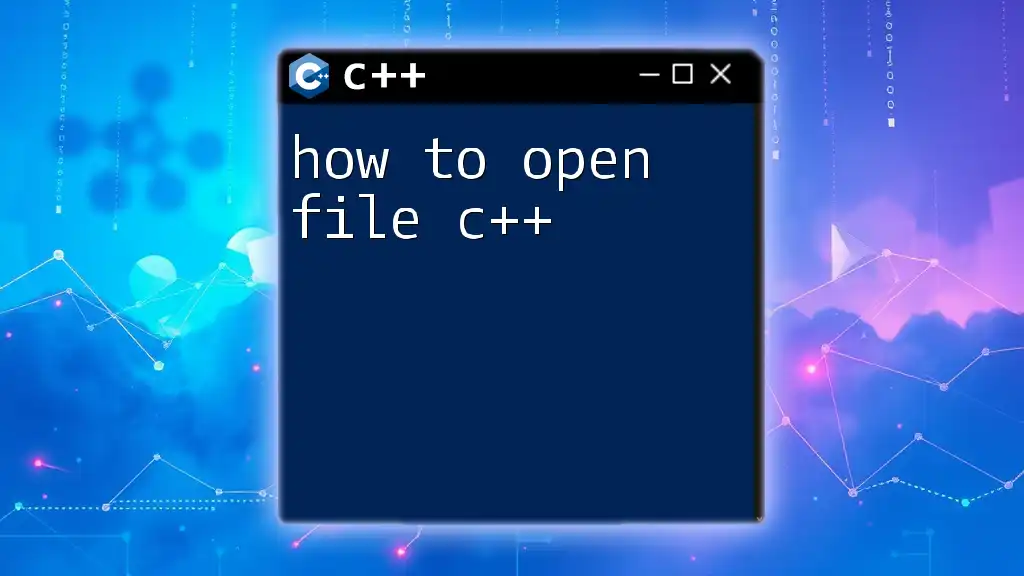
Advanced Methods to Open C++ Files
Command Line Interface (CLI)
For developers who prefer a more hands-on approach, you can also open C++ files via the command line. This method is especially useful for quick edits or when working on remote servers.
-
Windows: Use the following commands to navigate to your file's directory and open it:
cd path\to\your\folder start filename.cpp
-
Linux/Mac: The commands are slightly different:
cd /path/to/your/folder open filename.cpp
This method provides a quick way to access files without navigating through GUI menus.
Using Version Control Systems
If your C++ files are stored in a version-controlled repository (e.g., Git), you can clone the repository and open your files easily. Here’s how:
-
Clone the Git repository:
git clone https://github.com/your-repo.git
-
Navigate into the cloned directory:
cd your-repo
-
Open the desired C++ file using your preferred editor, e.g., with Visual Studio Code:
code your-file.cpp
This ensures you have the latest version of your code and can efficiently manage changes.
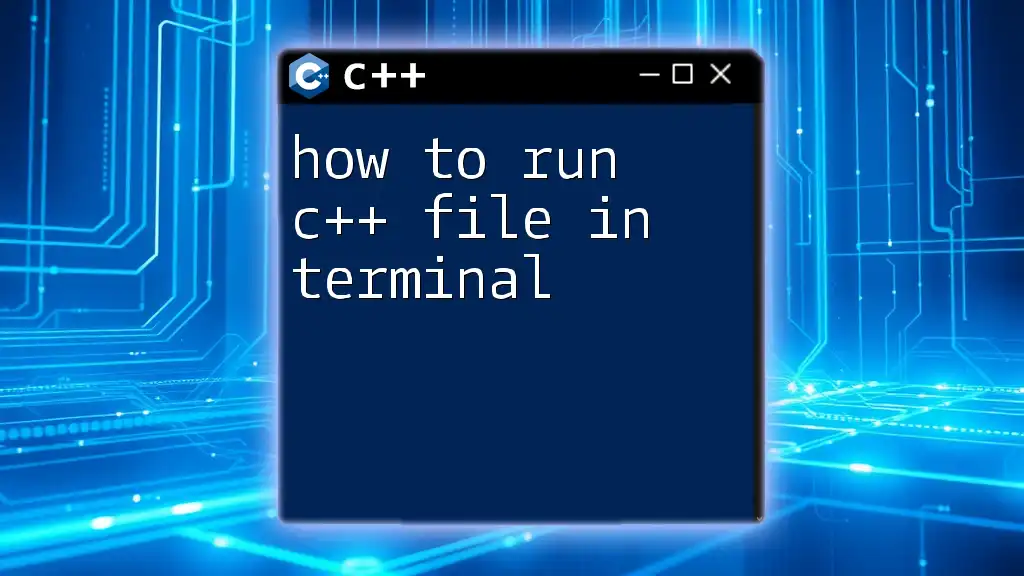
Editing and Saving Opened C++ Files
Basic Editing Techniques
Once you have the C++ file open, you can begin editing. Some essential tips for writing quality C++ code include:
- Consistent indentation for improved readability.
- Use of comments to explain complex logic or algorithms.
- Adherence to naming conventions for functions and variables.
Saving Your Changes
In any coding environment, saving changes regularly is crucial. Use the following guidelines based on the environment you are using:
- In most text editors, you can simply hit `Ctrl + S` to save your file.
- For IDEs, the same shortcut usually applies, and it is a good practice to make use of the auto-save feature if available.
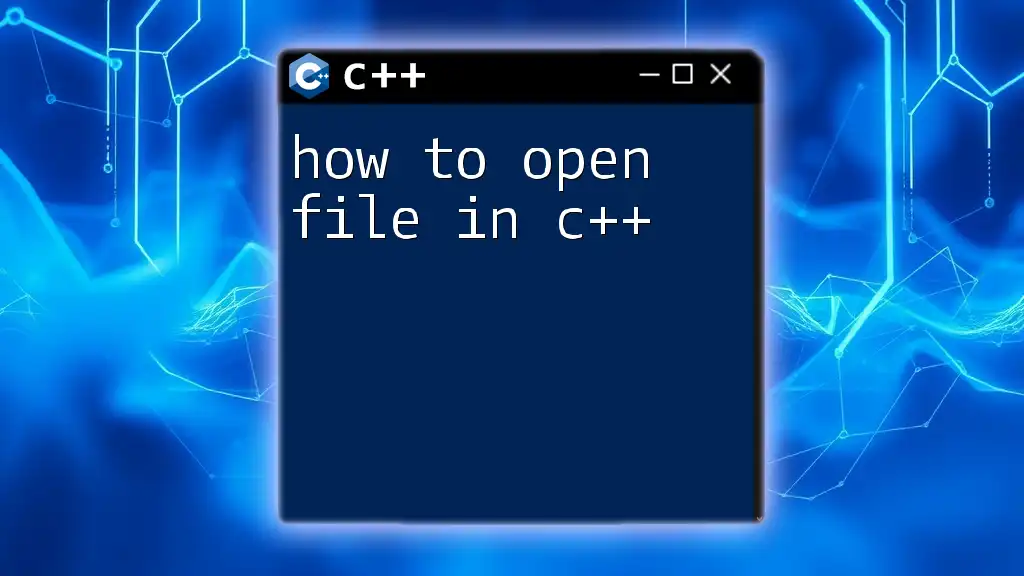
Troubleshooting Common Issues
File Not Found Errors
One common issue when trying to open C++ files is encountering a "File Not Found" error. This could happen due to:
- The file not being in the specified directory.
- Typos in the file path or name.
To resolve this, double-check the path and ensure that the file exists in the location you are trying to access.
File Permission Issues
If you're unable to open a C++ file due to permission errors, it may be that your user account lacks the necessary permissions. To change file permissions:
- On Windows, right-click the file, select Properties, go to the Security tab, and modify the permissions as needed.
- On Linux/Mac, you can change permissions using the command:
chmod 755 filename.cpp
This command sets the file to be readable and executable.
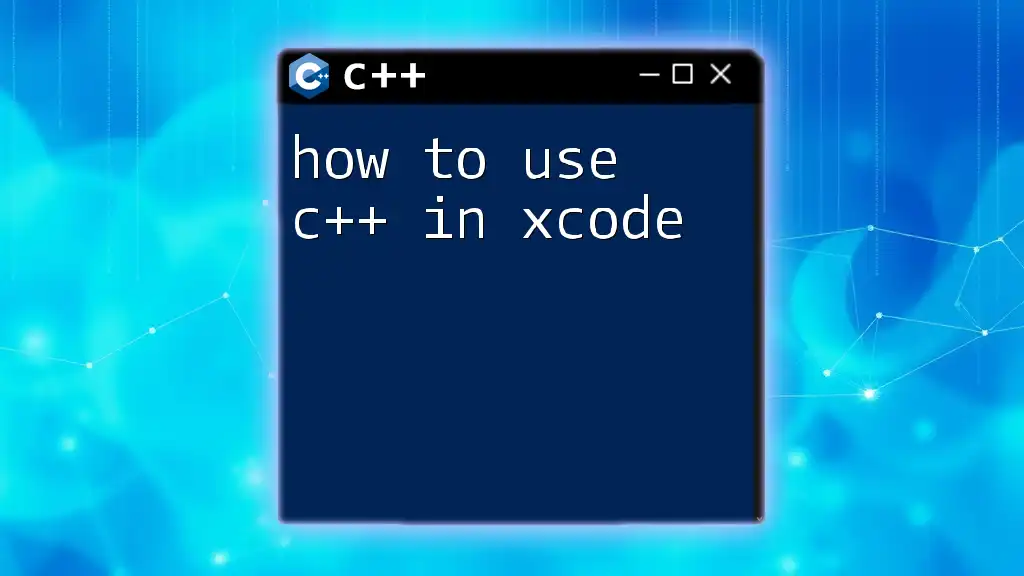
Conclusion
Now that you have a comprehensive understanding of how to open C++ files, you can explore various methods according to your preferences and needs. Whether you choose a simple text editor, a robust IDE, or the command line, the ability to navigate your C++ environment efficiently is key to becoming a proficient programmer. Don't hesitate to practice these skills and delve into other relevant C++ resources as you continue your learning journey.