To check if a file is empty in C++, you can use the `std::ifstream` class to open the file and then use the `std::ifstream::peek()` method to determine if there are any characters to read.
#include <fstream>
#include <iostream>
bool isFileEmpty(const std::string& filename) {
std::ifstream file(filename);
return file.peek() == std::ifstream::traits_type::eof();
}
int main() {
std::string filename = "example.txt";
if (isFileEmpty(filename)) {
std::cout << "The file is empty." << std::endl;
} else {
std::cout << "The file is not empty." << std::endl;
}
return 0;
}
Understanding File Handling in C++
What is File Handling?
File handling in C++ refers to the process of interacting with files on the disk. This enables programs to read data from and write data to files, allowing for persistent data storage beyond the program's runtime. C++ provides a set of classes for file operations, including `ifstream` for reading and `ofstream` for writing.
Why Check If a File is Empty?
Before performing operations on a file, checking if a file is empty is crucial. An empty file may lead to unintended consequences, such as:
- Runtime errors: Attempting to read from an empty file could lead your program to crash or produce invalid results.
- Data integrity: If your logic depends on file content, processing an empty file might compromise the correctness of your program.
- User experience: Returning appropriate messages to users when files are empty fosters a better interaction with your application.

Methods to Check if a File is Empty
Using `std::ifstream`
Opening a File
Before checking if a file is empty, you need to open it for reading using `std::ifstream`. Here’s how to include the necessary header files:
#include <iostream>
#include <fstream>
The `std::ifstream` class is designed for reading files. It helps create an input file stream, allowing you to read data from an existing file.
Checking File Size
One effective method to check if a file is empty in C++ is by utilizing the file size. You can achieve this using the `seekg()` and `tellg()` methods, which navigate through the file. Here’s how it works:
std::ifstream file("example.txt");
file.seekg(0, std::ios::end);
if (file.tellg() == 0) {
std::cout << "File is empty." << std::endl;
}
- `seekg(0, std::ios::end)`: This command moves the file pointer to the end of the file.
- `tellg()`: It retrieves the current position of the file pointer. If it's at 0, the file is empty.
Using this method is straightforward and effectively checks if there is any content in the file.
Using `std::ifstream::peek()`
The `peek()` Method
Another handy approach is leveraging the `peek()` method, which allows you to check the first character in the file without removing it from the stream. This method is beneficial for assessing the state of the file quickly.
Here’s a code snippet that demonstrates how to use `peek()`:
std::ifstream file("example.txt");
if (file.peek() == std::ifstream::traits_type::eof()) {
std::cout << "File is empty." << std::endl;
}
In this case:
- `peek()` reads the next character in the stream.
- If it returns `std::ifstream::traits_type::eof()`, this indicates that the end of the file has been reached, confirming the file is empty.
Note: This method is particularly useful when combined with others for robust file handling checks.
Checking Errors with `std::ifstream::fail()`
File Open Validation
It's critical to first validate if the file was opened successfully before performing any operations. The `fail()` method can help identify any issues with file opening:
std::ifstream file("example.txt");
if (!file) {
std::cerr << "Error opening file." << std::endl;
return;
}
This piece of code checks for any errors during the file opening stage, such as if the file does not exist.
Combining Checks
Combining the open validation with the emptiness check creates a reliable mechanism for file handling:
if (file.fail() || file.peek() == std::ifstream::traits_type::eof()) {
std::cout << "File is either not opened or empty." << std::endl;
}
This combines two essential checks:
- If the file opened correctly.
- If the file is empty.
Example Program
To put everything into practice, here’s a complete example that incorporates the aforementioned methods in C++. The program checks if a specified file is empty:
#include <iostream>
#include <fstream>
void checkFileEmpty(const std::string &filePath) {
std::ifstream file(filePath);
if (!file) {
std::cerr << "Error opening file." << std::endl;
return;
}
if (file.peek() == std::ifstream::traits_type::eof()) {
std::cout << "File is empty." << std::endl;
} else {
std::cout << "File is not empty." << std::endl;
}
}
int main() {
checkFileEmpty("example.txt");
return 0;
}
In this example:
- The `checkFileEmpty` function attempts to open the file.
- It checks for successful opening, evaluates emptiness, and provides user feedback correspondingly.
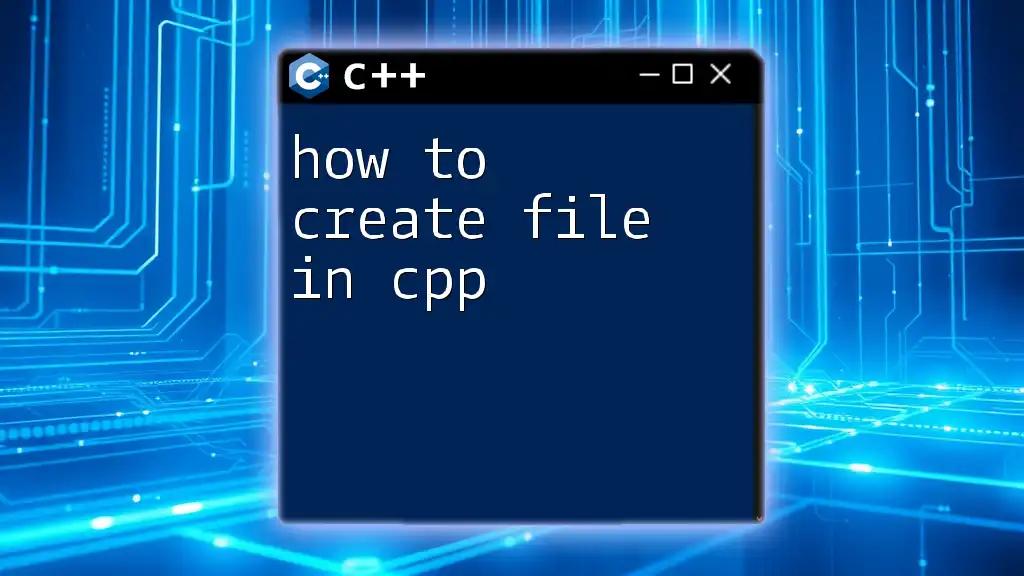
Conclusion
In summary, understanding how to check if a file is empty in C++ is a fundamental skill that can prevent runtime issues and enhance data integrity. By leveraging methods such as file size checks, the `peek()` function, and validating file opening, you can create robust programs that handle files efficiently.
Practicing these techniques will help you become proficient in file handling, allowing for more complex and manageable data-driven applications.

Additional Resources
To further enrich your knowledge on file handling in C++, consider exploring additional resources such as:
- The C++ Standard Library documentation.
- Tutorials focusing on advanced file operations.
- Community forums where practical applications of file handling are discussed.
By investing in your understanding of these concepts, you will certainly become a more effective C++ developer!