To check if a number is prime in C++, you can iterate through all the numbers up to its square root and verify if it has any divisors other than 1 and itself.
#include <iostream>
#include <cmath>
bool isPrime(int num) {
if (num <= 1) return false;
for (int i = 2; i <= std::sqrt(num); i++) {
if (num % i == 0) return false;
}
return true;
}
int main() {
int number;
std::cout << "Enter a number: ";
std::cin >> number;
if (isPrime(number))
std::cout << number << " is a prime number." << std::endl;
else
std::cout << number << " is not a prime number." << std::endl;
return 0;
}
What is a Prime Number?
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In simple terms, a prime number has exactly two distinct positive divisors: 1 and itself. For instance, the numbers 2, 3, 5, 7, 11, and 13 are prime, while 4, 6, 8, 9, and 10 are not, as they can be divided evenly by numbers other than 1 and themselves.
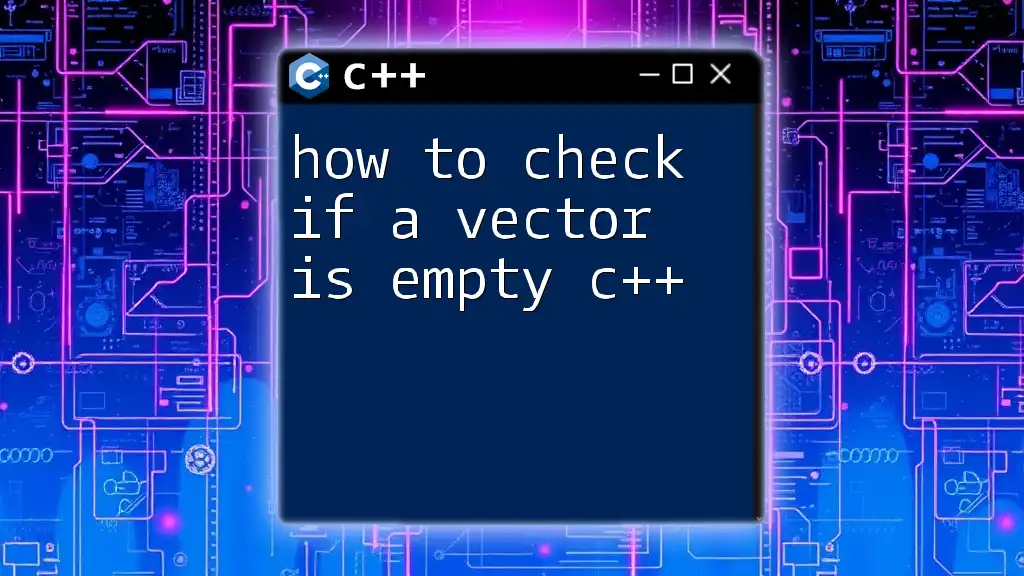
Importance of Identifying Prime Numbers
Understanding prime numbers is crucial in various fields, particularly in cryptography, algorithm design, and number theory. For example, many cryptographic algorithms, such as RSA, rely on the difficulty of factoring large prime numbers. In your coding journey, knowing how to check if a number is prime can also come in handy for loops, conditions, and other programming concepts.
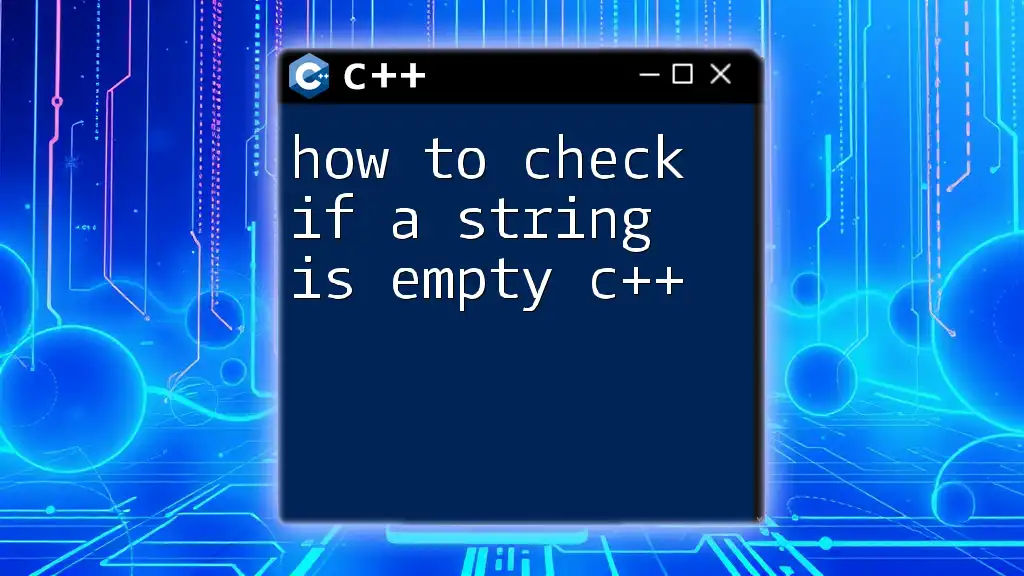
C++ as a Programming Language for Prime Checking
C++ is a powerful programming language that allows you to work directly with memory and perform high-performance computations. Its versatility makes it a preferred choice for implementing algorithms, including those to check prime numbers.
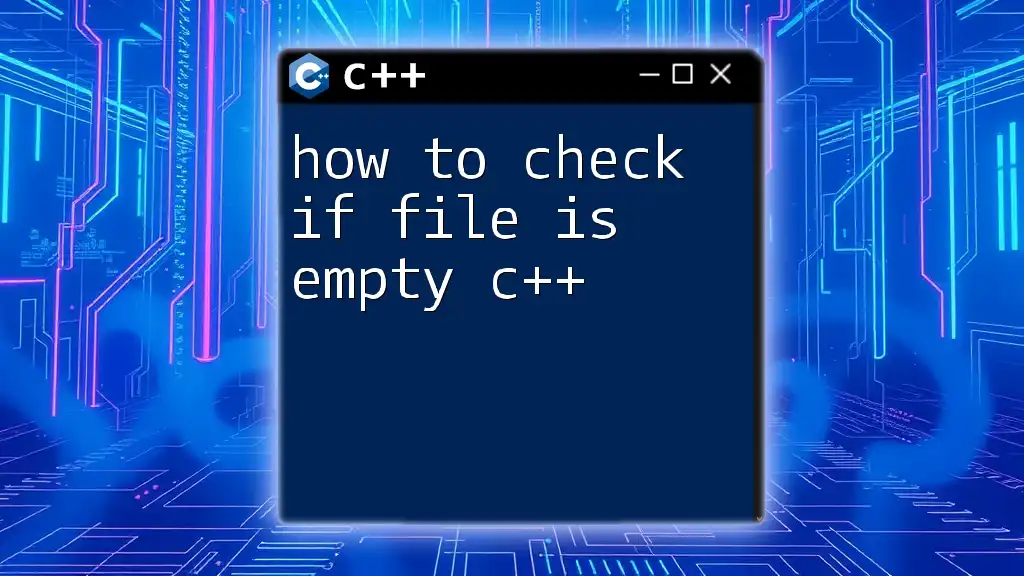
Understanding the Basics of Prime Number Check
What Makes a Number Prime?
To identify whether a number is prime, it is essential to understand the characteristics that make a number prime. All prime numbers are greater than 1 and are only divisible by 1 and themselves. This means that if you can find any integer (other than 1 and the number itself) that divides the number evenly, it cannot be prime.
Basic Approach to Checking Prime Numbers
The naive method to check if a number is prime involves testing divisibility for every integer from 2 up to the number itself. While straightforward, this approach can be inefficient for larger numbers.
Pros and Cons of the Naive Method
- Pros: Simple to understand and implement.
- Cons: Requires many checks, especially for large numbers, leading to performance issues.
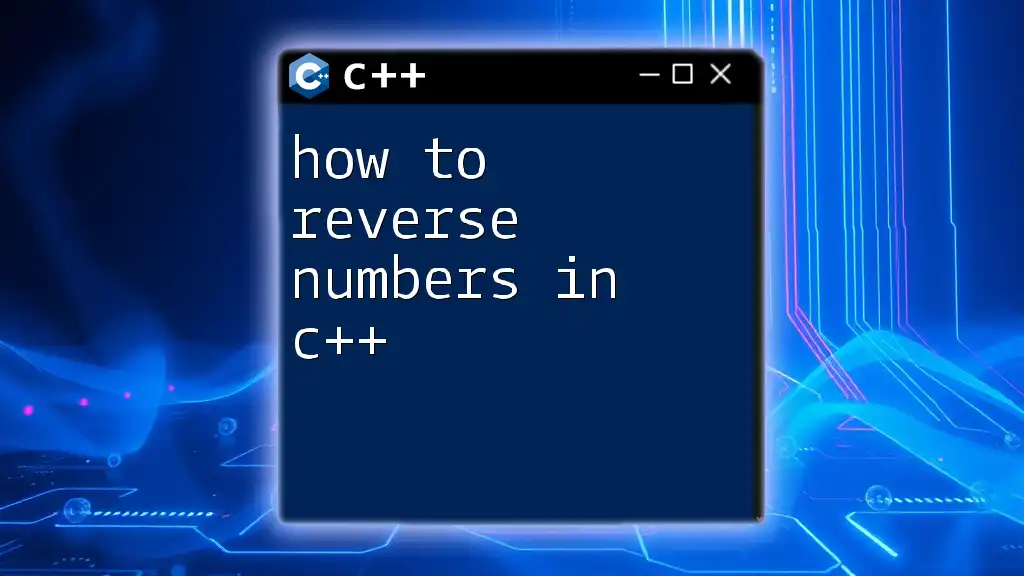
Implementing Prime Check in C++
Writing a Simple Function to Check for Prime
To start, you can create a simple function in C++ that checks if a number is prime. The basic outline would look like this:
bool isPrime(int num);
Code Example
Here’s a straightforward implementation of this function utilizing the naive method:
bool isPrime(int num) {
if (num <= 1) return false; // 0 and 1 are not prime numbers
for (int i = 2; i <= num / 2; i++) {
if (num % i == 0) return false; // divisible by i
}
return true; // num is prime
}
Explanation of the Code
- Return False for Numbers Less Than 2: The function first checks if the number is less than or equal to 1. Since prime numbers are defined only for natural numbers greater than 1, it returns `false` in these cases.
- Loop Through Potential Divisors: The loop iterates from 2 up to `num/2`. If it finds any integer `i` that divides `num` evenly (i.e., `num % i == 0`), it confirms that `num` is not prime and returns `false`.
- Conclusion: If no divisors are found, the function returns `true`, indicating that `num` is prime.
Optimizing the Prime Check Function
Reducing the Number of Iterations
While the naive method works, we can optimize it significantly. Instead of checking all numbers up to `num`, we can limit our checks using the square root of the number.
Revised Code Example
Here’s the improved version of the prime-checking function:
bool isPrime(int num) {
if (num <= 1) return false; // 0 and 1 are not prime numbers
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) return false;
}
return true; // num is prime
}
In this revision, the loop condition checks `i * i <= num`, which significantly reduces the number of iterations, making the function much faster for larger numbers.
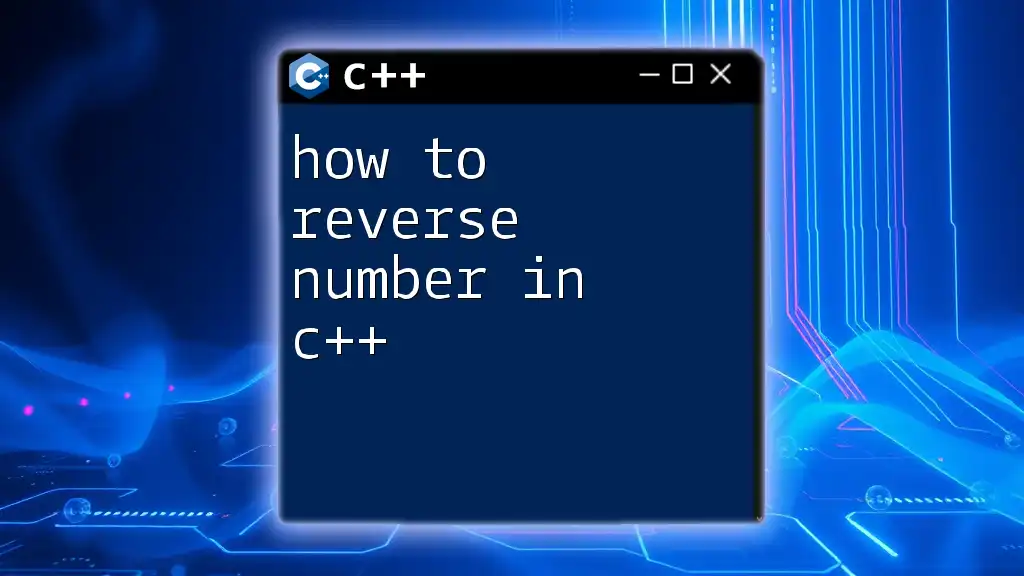
More Advanced Techniques
Using the Sieve of Eratosthenes for Multiple Numbers
For checking the primality of multiple numbers efficiently, the Sieve of Eratosthenes is a powerful algorithm. It identifies all prime numbers up to a specified limit.
Explanation of the Algorithm
The algorithm works by iteratively marking the multiples of each prime starting from 2. As it progresses, the unmarked numbers remaining in the list are primes.
Code Implementation
Here’s how you can implement the Sieve of Eratosthenes in C++:
#include <iostream>
#include <cstring>
void sieveOfEratosthenes(int n) {
bool prime[n + 1];
memset(prime, true, sizeof(prime));
for (int p = 2; p * p <= n; p++) {
if (prime[p] == true) {
for (int i = p * p; i <= n; i += p)
prime[i] = false;
}
}
for (int p = 2; p <= n; p++)
if (prime[p])
std::cout << p << " ";
}
Application and Benefits
The Sieve of Eratosthenes is particularly efficient for generating a list of prime numbers. If you need to find primes up to a large number, this method is preferable due to its lower time complexity compared to checking each number individually.
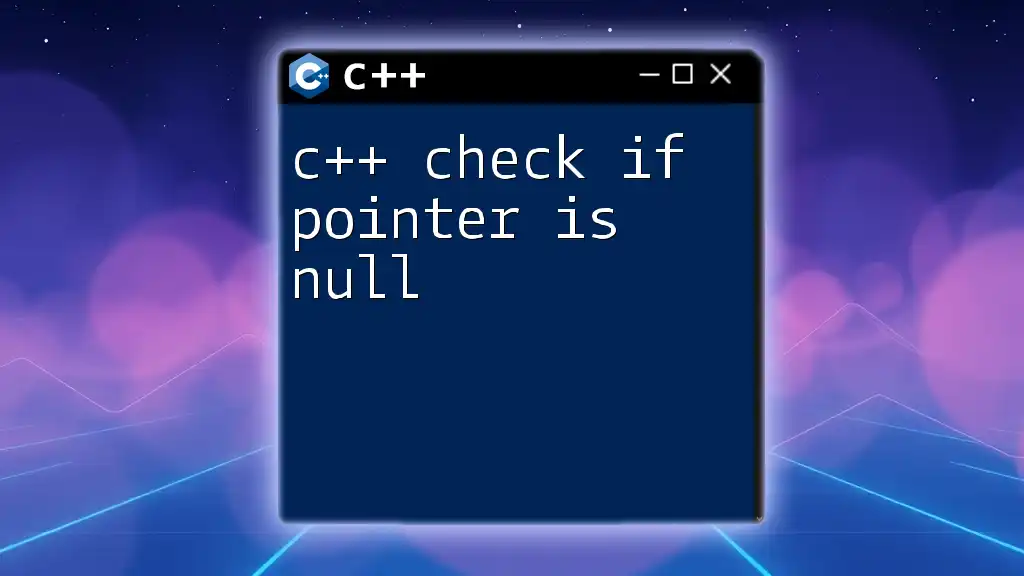
Testing the Prime Check Function
Creating Test Cases
Testing is a crucial step in programming to ensure your functions are working correctly. Various test cases can help confirm the expected functionality.
Example Test Cases
Here’s how you can test your `isPrime` function:
std::cout << isPrime(11) << std::endl; // Expected: true
std::cout << isPrime(15) << std::endl; // Expected: false
std::cout << isPrime(1) << std::endl; // Expected: false
Using Assertions
Assertions can be integrated into your code to ensure that your functions return the correct outputs during development.
#include <cassert>
assert(isPrime(2) == true);
assert(isPrime(4) == false);
By embedding assertions, you maintain code reliability and catch potential errors early in the development process.
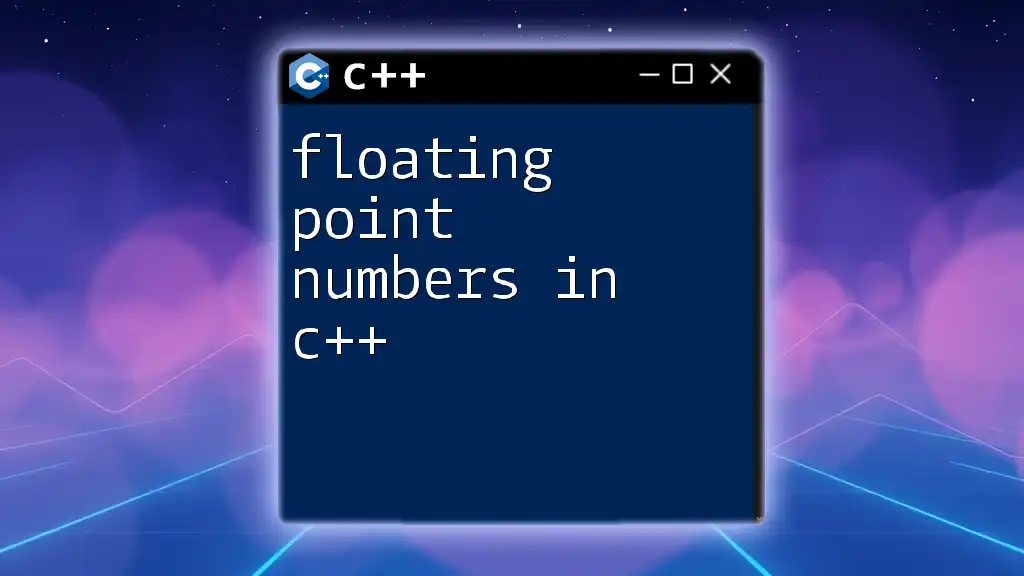
Conclusion
In this guide, we covered how to check if a number is prime in C++ using different methods—ranging from a basic prime-checking function to more advanced algorithms like the Sieve of Eratosthenes. With the knowledge gained here, we encourage you to explore further and practice what you have learned. Engaging with coding communities or enrolling in C++ command courses can deepen your understanding and skills. Happy coding!
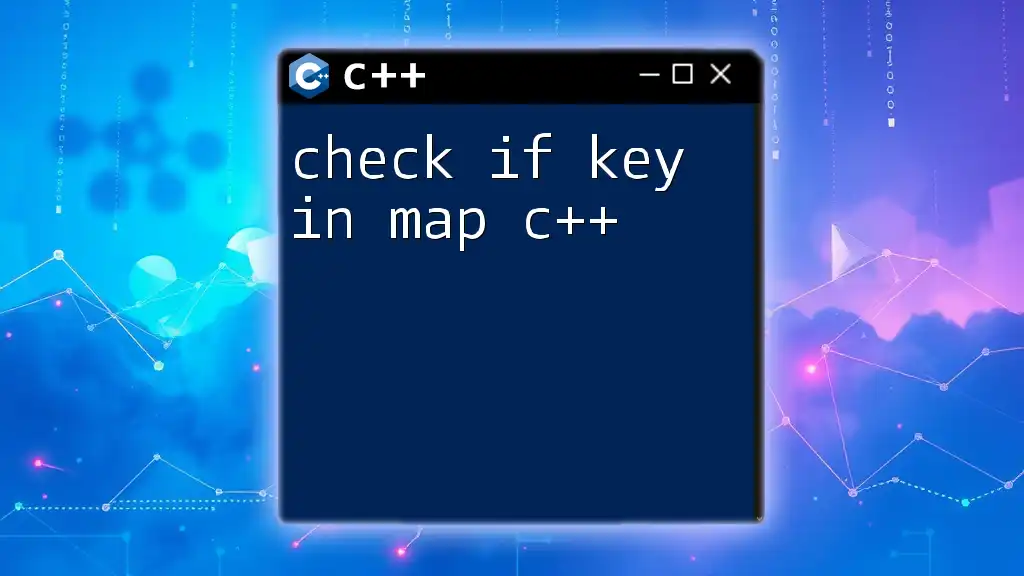
Additional Resources
For further reading, consider exploring books on algorithms and data structures, reputable programming forums, and comprehensive C++ documentation to broaden your knowledge.