In C++, you can declare an array by specifying the type, followed by the name of the array and the size in square brackets, as shown in the example below:
int myArray[5]; // declares an array of integers with 5 elements
Understanding Arrays
What is an Array?
An array in C++ is a collection of variables that are of the same type, stored in contiguous memory locations. The key characteristics of arrays include fixed size and the requirement that all elements must be of a homogeneous data type. This makes arrays a fundamental data structure for storing collections of similar data efficiently.
Benefits of Using Arrays
Using arrays comes with several advantages:
- Memory Efficiency: Arrays occupy a single contiguous block of memory, leading to better cache performance and reduced memory overhead compared to alternatives like linked lists.
- Fast Access to Elements: Elements can be accessed quickly using indices, allowing operations to be performed in constant time, O(1).
- Easy Iteration with Loops: Arrays can be easily traversed with `for` or `while` loops, facilitating operations like searching and sorting.
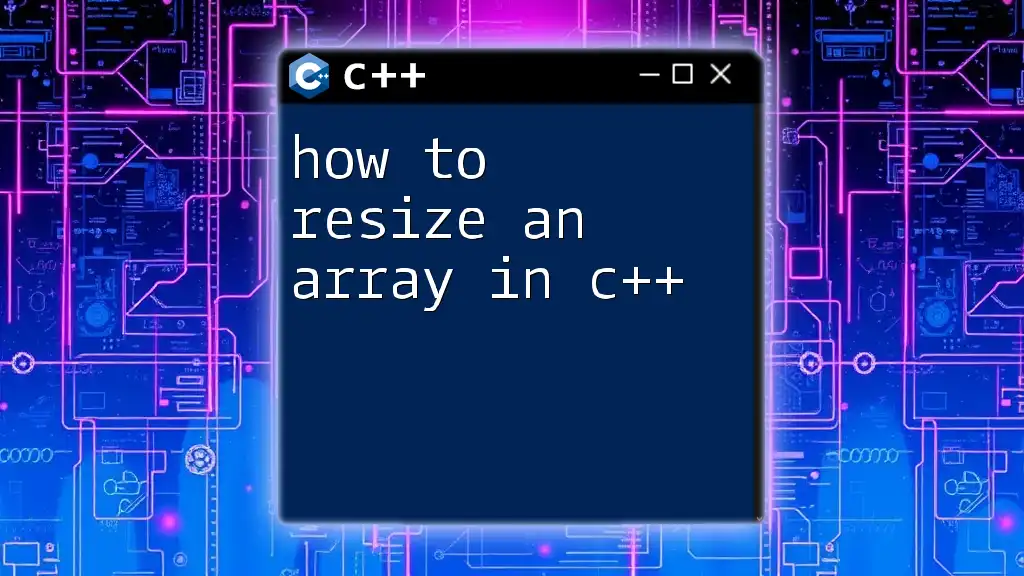
How to Declare an Array
Basic Syntax of Array Declaration
To declare an array in C++, you use the following syntax:
data_type array_name[array_size];
This involves specifying the data type of the array elements, the name of the array, and the number of elements it will contain. For example:
int numbers[5];
In this case, `numbers` is an array that can hold five integers.
Types of Arrays
Single-Dimensional Arrays
A single-dimensional array is the simplest form of array and is essentially a list of elements. The declaration and initialization of a single-dimensional array can be done as follows:
int scores[4] = {90, 85, 88, 92};
To access an element from this array, you can use the index (with indexing starting at 0):
int firstScore = scores[0]; // Retrieves the first element (90)
Multi-Dimensional Arrays
Multi-dimensional arrays extend the concept of arrays to more than one dimension. The most common type is the two-dimensional array, often used for matrix representation. For example:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Accessing elements in a two-dimensional array requires two indices:
int centerValue = matrix[1][1]; // Retrieves the center element (5)
Initializing Arrays
Default Initialization
When you declare an array without initializing it, the elements contain undefined values. However, if you use a static size and declare an array without initial values, its elements are set to zero:
int emptyArray[5]; // All elements are initialized to 0
Manual Initialization
You can also initialize arrays explicitly during declaration, as shown here:
char vowels[5] = {'a', 'e', 'i', 'o', 'u'};
This method allows you to set the specific values for each element at the time of declaration.
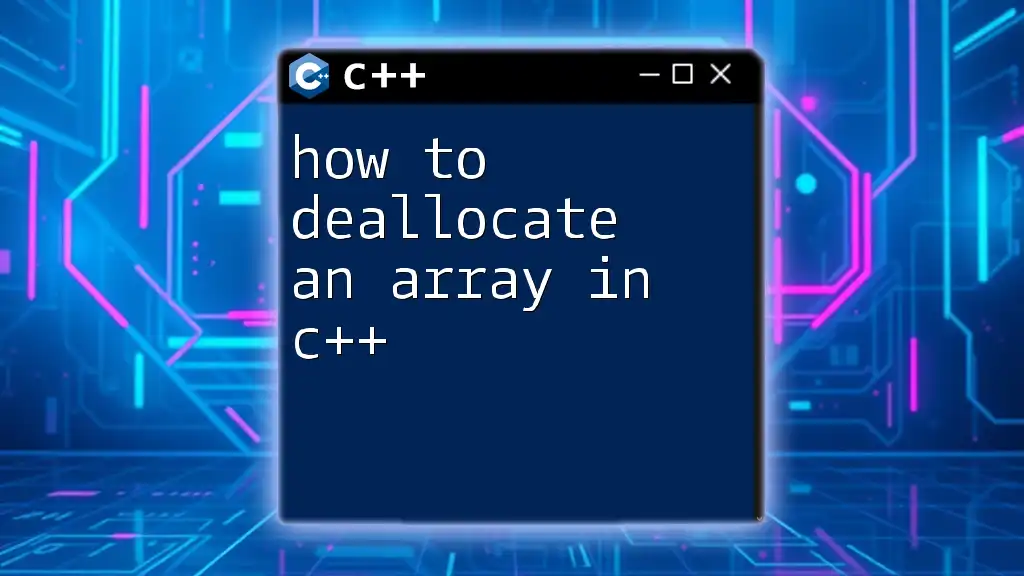
Working with Arrays
Accessing Array Elements
You can read and write the elements of an array by using their indices. Here's how you can do it:
int value = scores[2]; // Accessing the third element
scores[3] = 95; // Modifying the fourth element
In this case, `value` will contain `88` (the third element), and the fourth element of `scores` will now be updated to `95`.
Iterating Through Arrays
To perform operations on each element of an array, loops are essential. A common method is to use a `for` loop. For instance:
for(int i = 0; i < 4; i++) {
cout << scores[i] << " ";
}
This loop will print all the elements of the `scores` array.
Common Mistakes When Declaring Arrays
Array Index Out of Bounds
One common pitfall in working with arrays is accessing an element outside the defined size. Doing this can lead to runtime errors or undefined behavior. Consider this example:
int test[3] = {1, 2, 3};
cout << test[5]; // This will cause an error (index out of bounds)
Always ensure that you are accessing valid indices.
Forgetting to Specify Size
Another common mistake is declaring an array without specifying its size, which is not allowed in C++. For instance:
int uninitializedArray[]; // This line will cause an error
When declaring an array, ensure to specify a fixed size or initialize it explicitly.
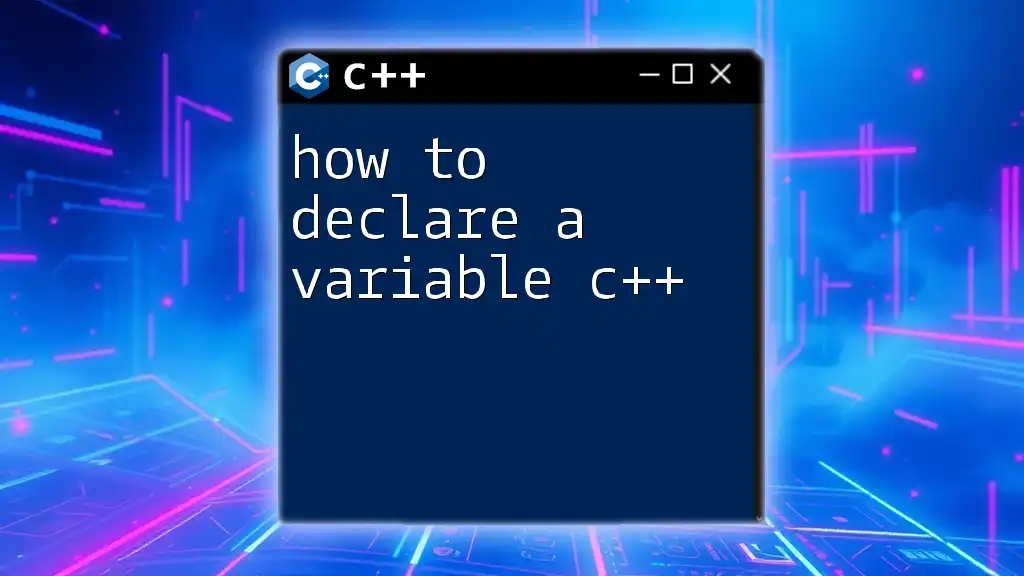
Advanced Topics
Using Arrays with Functions
Arrays can be passed to functions in C++, allowing for modular programming. The syntax for passing an array is as follows:
void printArray(int arr[], int size) {
for(int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
}
You would then call `printArray(scores, 4);` to output the `scores` array.
Dynamic Arrays
When the size of an array needs to be determined at runtime, dynamic arrays come in handy. You can use the `new` keyword to allocate memory dynamically:
int* dynamicArray = new int[n];
Remember to free the memory allocated for dynamic arrays using `delete[]` once you're done using them:
delete[] dynamicArray;
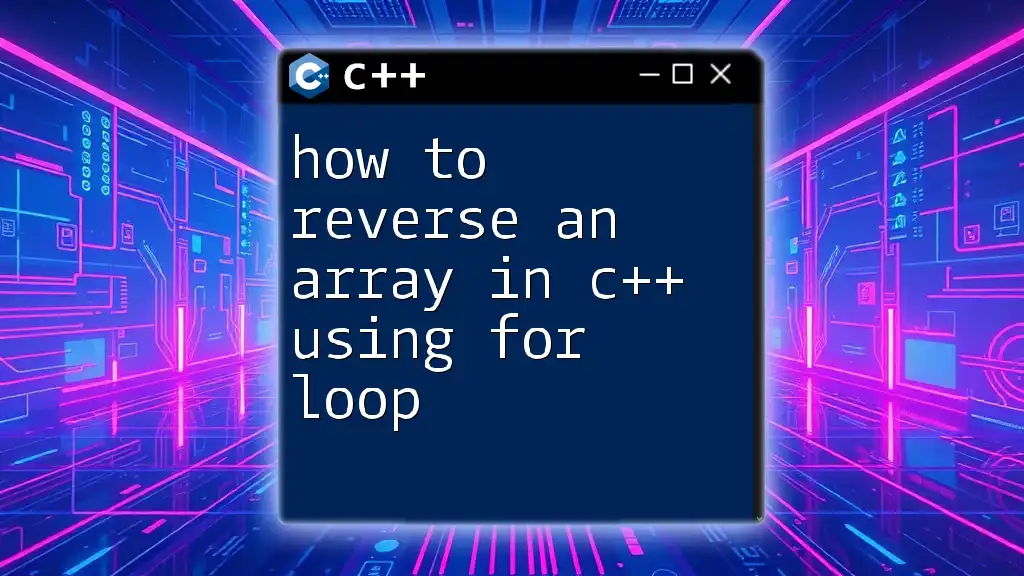
Conclusion
Arrays are a fundamental part of programming in C++, enabling efficient storage and manipulation of collections of data. Understanding how to declare an array in C++, its various types, initialization methods, and common pitfalls is crucial for every programmer.
By mastering these concepts, you'll enhance your programming skills and be well-prepared to tackle more advanced data structures and algorithms.