To deallocate an array in C++, use the `delete[]` operator for dynamically allocated arrays to free the allocated memory. Here’s a code snippet demonstrating this:
int* arr = new int[10]; // dynamically allocating an array
// ... use the array ...
delete[] arr; // deallocating the array
Understanding Memory in C++
What is Dynamic Memory?
Dynamic memory allocation plays a crucial role in C++ programming, allowing developers to allocate memory during the runtime of the program rather than at compile time. This is especially useful for arrays when the size may not be known beforehand, or when it needs to be flexible.
In C++, memory is divided into two primary types: stack and heap. Stack memory is automatically managed, but heap memory, where dynamic memory is allocated, requires manual management. Understanding this distinction is essential when learning how to deallocate an array in C++.
The C++ `new` and `delete` Operators
The `new` operator is used to allocate memory dynamically. When creating an array, you would use the `new[]` form, which allocates a contiguous block of memory for your array elements.
The corresponding `delete` operator must be utilized to free the memory previously allocated with `new`. Specifically, when dealing with arrays, you should use `delete[]`, which properly handles the deallocation of all array elements.
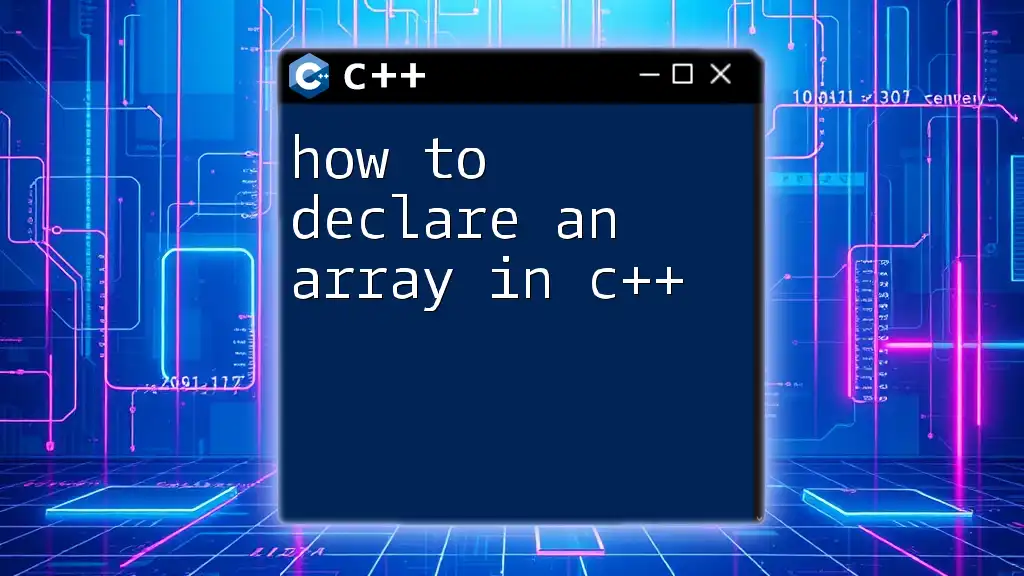
Allocating Arrays in C++
Declaring Arrays
Arrays can be declared statically, where their size must be known at compile time, or dynamically, where they can be allocated during execution. For example:
Static allocation:
int arr[5]; // stack allocated
Dynamic allocation utilizes the `new` operator:
int* dynamicArr = new int[5]; // heap allocated
Dynamic Array Allocation
Dynamic array allocation is essential when the size of the array is not known ahead of time. Here’s how to allocate a dynamic array:
int* myArray = new int[10]; // Allocates an array of 10 integers
This line of code allocates memory for 10 integers on the heap and assigns the starting address of that memory block to the pointer `myArray`.
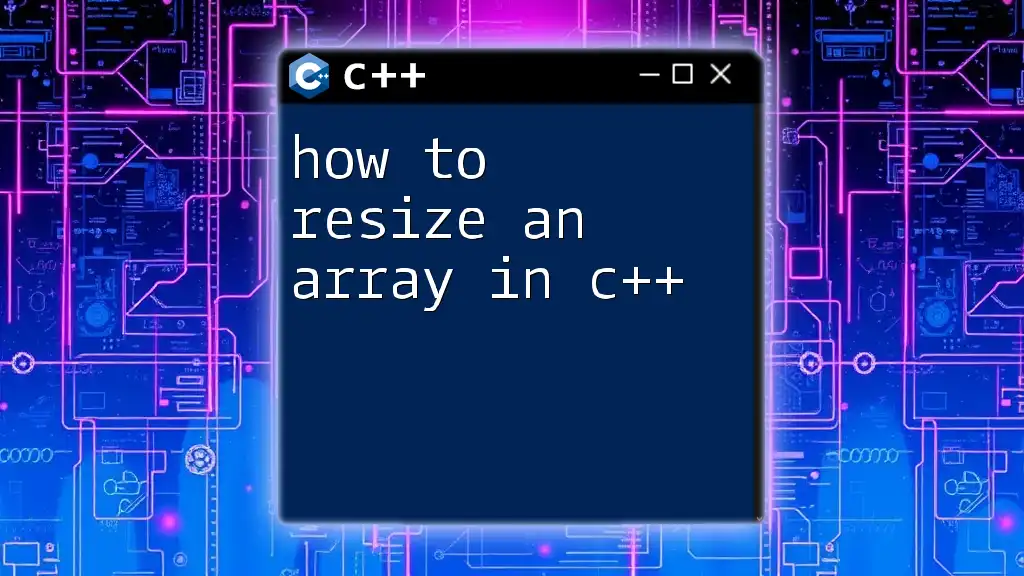
How to Deallocate Dynamic Arrays
The Role of `delete[]`
To properly deallocate a dynamic array, you must use `delete[]` to free the memory that was allocated with `new[]`. Failing to do so leads to memory leaks, which drain system resources over time.
Here’s how to deallocate the dynamic array created in the previous example:
delete[] myArray; // Deallocates memory for the array
Preventing Memory Leaks
A memory leak occurs when a program loses the reference to allocated memory, causing it to remain unreachable and unfreeable. Since the heap does not automatically reclaim memory, not using `delete[]` on dynamically allocated arrays can lead to serious performance issues in long-running applications.
By employing `delete[]`, you ensure that once your program no longer needs the array, the associated memory is properly released back to the system.
Best Practices for Deallocating Arrays
Always Match `new[]` with `delete[]`
It is essential to pair each `new[]` allocation with a corresponding `delete[]` statement. Mismatching can lead to undefined behavior and memory leaks.
For example:
int* myArray = new int[5]; // Allocating
// ... Use the array
delete[] myArray; // Properly deallocating
Setting Pointers to `nullptr`
After deallocating memory, it's crucial to set the pointer to `nullptr` to avoid dangling pointers that could lead to undefined behavior if accessed. This is a good coding practice that minimizes potential errors.
Here’s an example:
delete[] myArray; // Deallocate memory
myArray = nullptr; // Set the pointer to nullptr
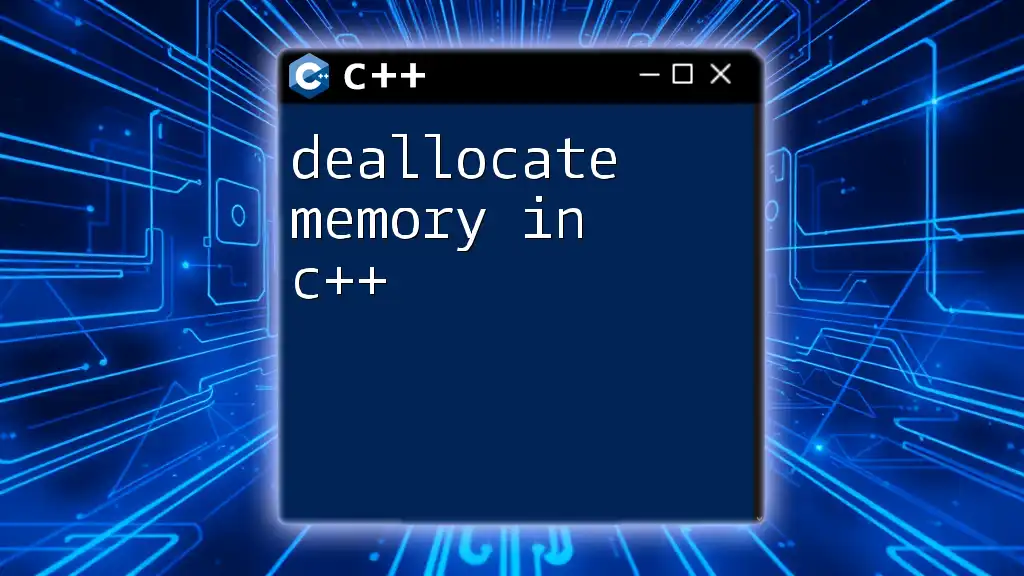
Common Mistakes in Deallocating Arrays
Forgetting to Deallocate
One of the most common mistakes is forgetting to deallocate memory. If you do this, you will create memory leaks, which can accumulate over time, especially in long-running applications.
For instance:
int* myArray = new int[100]; // Allocating
// forgot to delete myArray!
Using `delete` instead of `delete[]`
Using `delete` instead of `delete[]` on a dynamic array can lead to undefined behavior. `delete` is used for single objects and does not properly call destructors for each element in the array.
Example of the mistake:
int* myArray = new int[10]; // Allocating
delete myArray; // Incorrect, should use delete[]
Double Deleting
Double deleting, or attempting to delete the same memory location more than once, can result in program crashes or undefined behavior. If you inadvertently attempt to delete memory that has already been deallocated, it can corrupt the program state.
Example:
int* myArray = new int[5];
delete[] myArray; // First deletion
delete[] myArray; // Error: double deletion
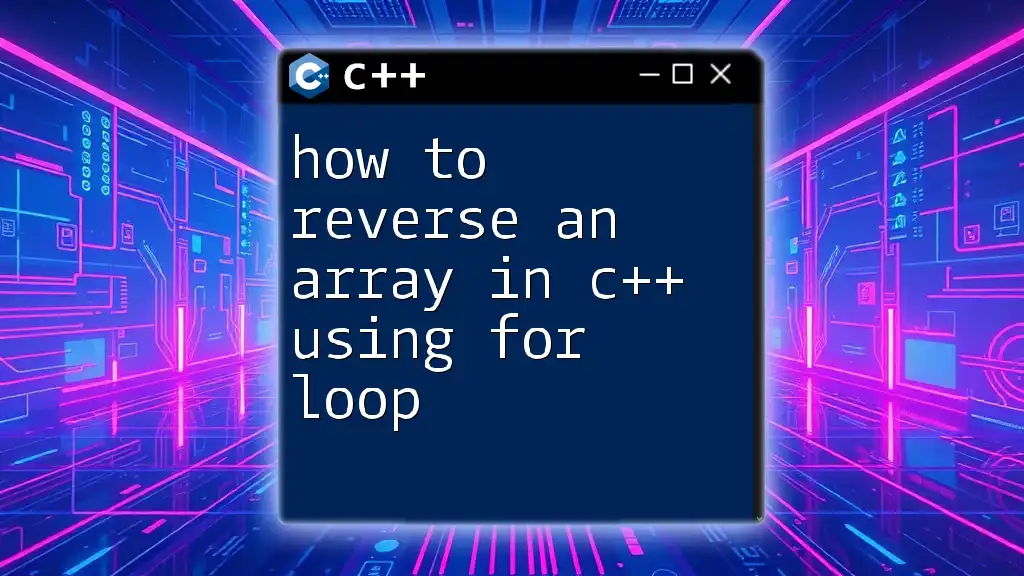
Safe Array Management Techniques
Using Smart Pointers
C++11 introduced smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, which automate memory management and reduce the risk of leaks and dangling pointers. When combined with arrays, these smart pointers can greatly improve safety and readability.
Example using `std::unique_ptr`:
#include <memory>
std::unique_ptr<int[]> myArray(new int[10]); // Automatically deallocates when going out of scope
With `std::unique_ptr`, there’s no need to explicitly call `delete[]` as it gets called automatically when the pointer goes out of scope.
C++ Standard Library Containers
Another effective way to manage arrays is by leveraging C++ Standard Library containers like `std::vector`. These containers handle memory allocation and deallocation for you, minimizing the risks associated with raw pointer management.
Example of using `std::vector`:
#include <vector>
std::vector<int> myArray(10); // Automatically manages array size, memory, and deallocation
Using `std::vector` provides flexibility, safety, and ease of use, making it the recommended approach for managing dynamic arrays in modern C++ code.
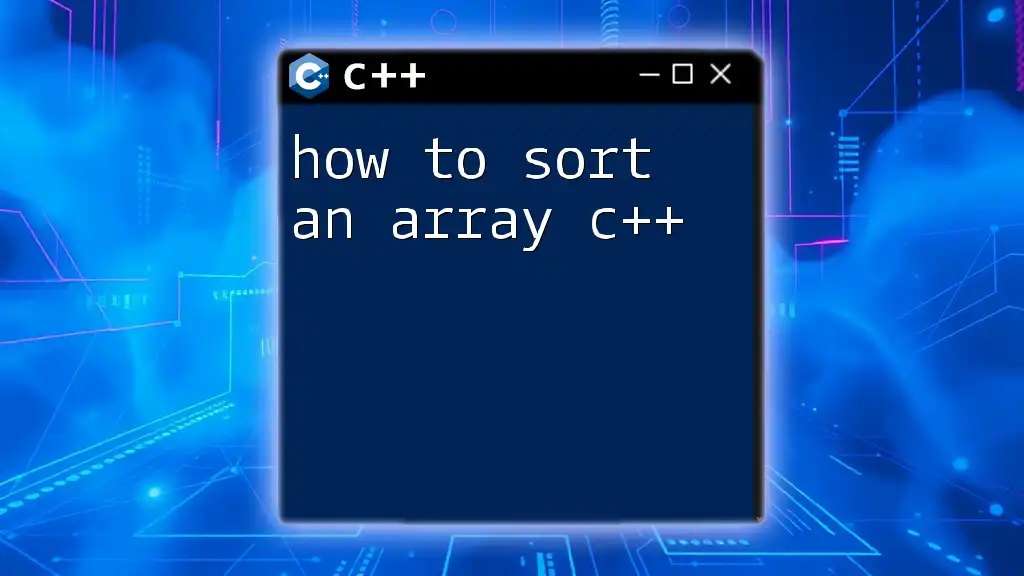
Conclusion
Understanding how to deallocate an array in C++ is an essential skill for effective memory management in your programs. By following best practices and using modern techniques like smart pointers and standard containers, you can significantly reduce the risk of memory leaks and bugs associated with manual memory management. Always remember to pair your dynamic allocations with proper deallocations and employ safeguards such as nullifying pointers to ensure robust C++ applications.
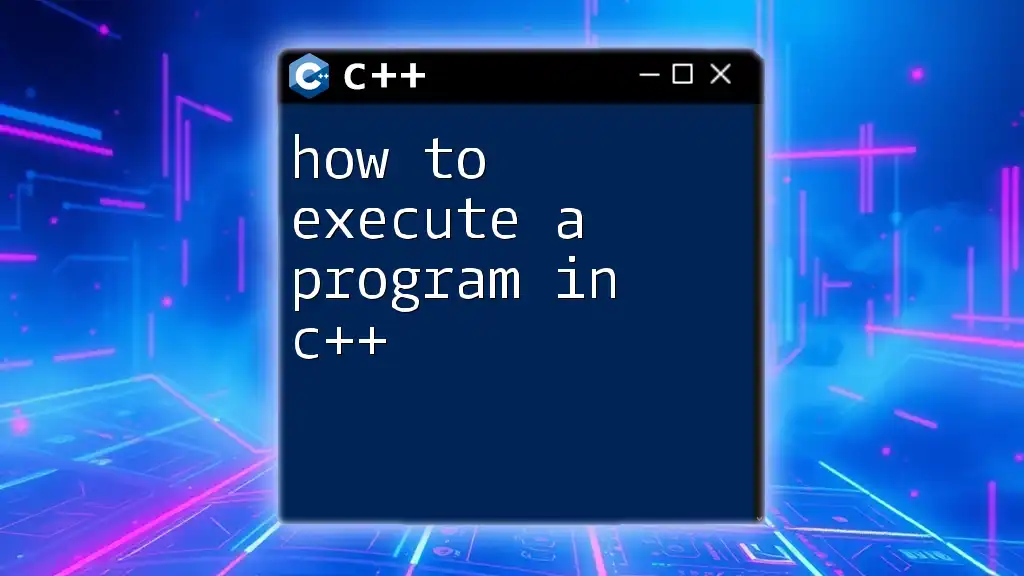
Additional Resources
For further reading and resources on C++ memory management, consider looking into textbooks, online courses, or dedicated C++ programming websites that offer comprehensive tutorials on the topic. Exploring these materials will deepen your understanding and proficiency in efficient memory management practices in C++.