In C++, memory allocated with `new` must be deallocated using `delete` to prevent memory leaks.
int* myArray = new int[10]; // Allocate memory
// ... use the array ...
delete[] myArray; // Deallocate memory
Understanding Memory Allocation and Deallocation
Memory management is a crucial aspect of programming in C++. Memory allocation refers to the process of reserving a block of memory for variables or objects during the program execution, while deallocation is the process of freeing that memory space once it is no longer needed. Properly managing memory is essential for preventing resource leaks and ensuring your program runs efficiently.
In C++, memory is primarily divided into two types: stack memory and heap memory. Stack memory is automatically managed by the compiler; it's where local variables are stored and deallocated automatically when they go out of scope. In contrast, heap memory is manually managed by the programmer. It requires explicit allocation and deallocation, which typically makes it more complex but also offers greater flexibility for dynamic memory requirements.
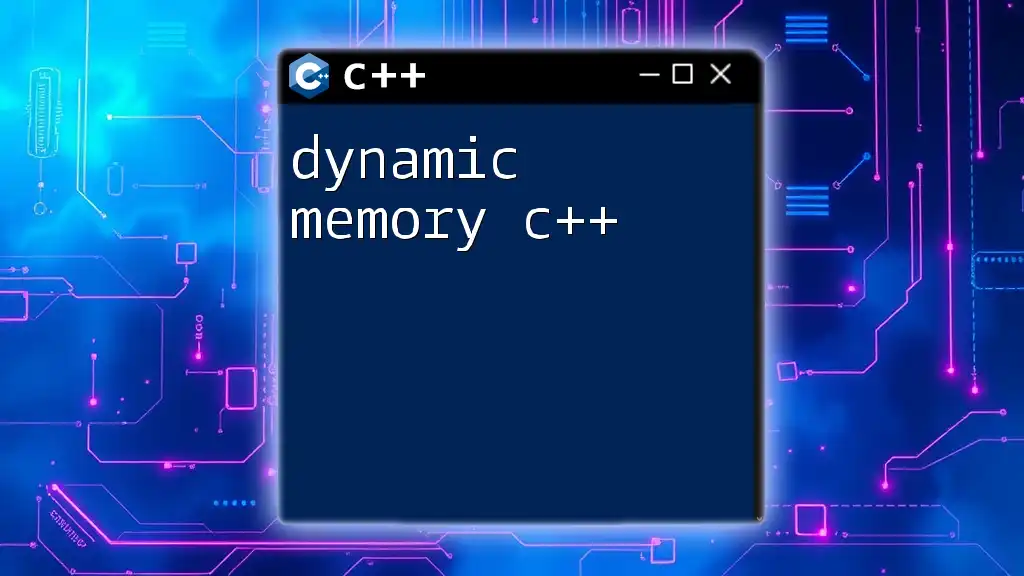
Why is Memory Deallocation Necessary?
Failing to deallocate memory leads to severe consequences, such as memory leaks, which occur when dynamically allocated memory is not freed. Over time, these leaks can accumulate, consuming more memory and potentially causing a program to crash or slowing it down significantly.
Proper memory deallocation also affects performance, allowing the system to reclaim unused memory promptly. When objects no longer need to exist, it is crucial to deallocate memory to avoid exhausting available resources. In this context, the C++ destructor plays a vital role by automatically releasing memory associated with an object when its scope ends or when it is explicitly deleted.
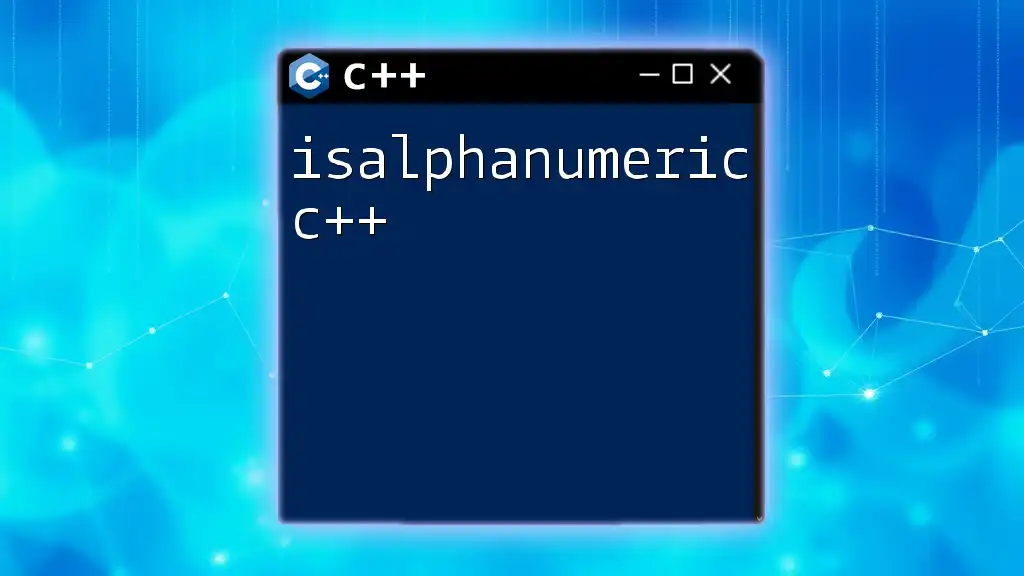
The Basics of Deallocating Memory in C++
In C++, there are specific methods for deallocating memory that you should become familiar with. The primary operator used for manual deallocation is delete.
Using the `delete` Operator
The `delete` operator is used to deallocate memory that was previously allocated with the `new` operator. Here are the basic usage patterns:
-
To deallocate a single object, use:
MyClass* obj = new MyClass(); delete obj; // Deallocates memory for a single object
-
To deallocate an array of objects, use `delete[]`:
MyClass* arr = new MyClass[10]; delete[] arr; // Deallocates memory for an array of objects
These operators ensure that the memory previously allocated for the objects is properly released for future use. Notably, using `delete[]` on a non-array object or just `delete` on an array can lead to undefined behavior.
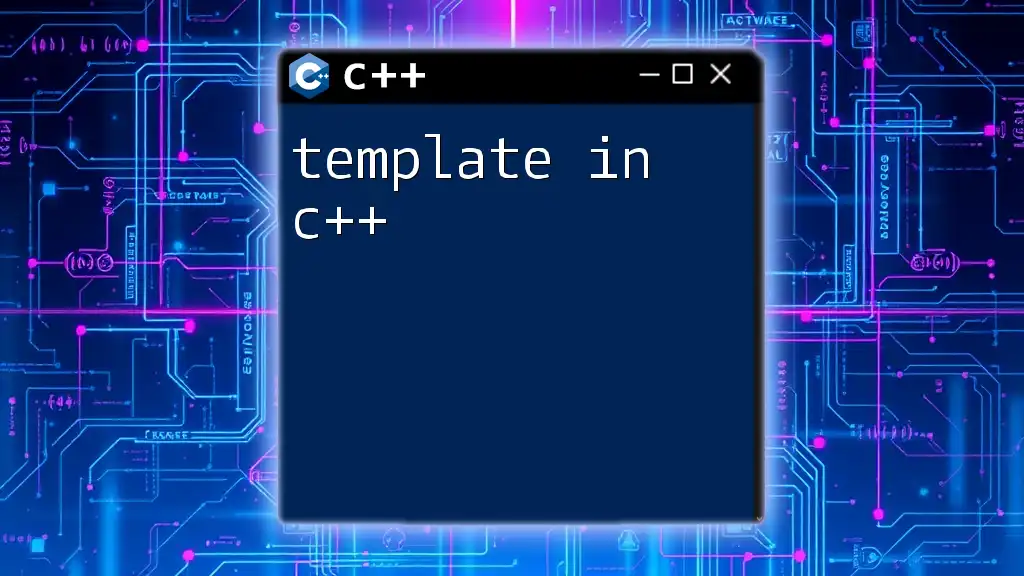
Using Smart Pointers for Automatic Memory Management
C++11 introduced smart pointers, which help automate memory management and reduce the risk of memory leaks.
What are Smart Pointers?
Smart pointers are wrappers around raw pointers that manage the memory they point to, ensuring that it is properly deallocated when no longer needed. This means lower risks of memory-related errors for developers.
Types of Smart Pointers
-
unique_ptr: This pointer represents sole ownership of an object. It cannot be copied; it can only be moved. When a unique_ptr goes out of scope, it automatically deallocates the memory it owns.
Code snippet:
std::unique_ptr<MyClass> ptr(new MyClass());
-
shared_ptr: This pointer allows multiple shared_ptr instances to own the same object, maintaining a reference count. When all shared_ptr instances pointing to an object are destroyed, the memory is automatically deallocated.
Code snippet:
std::shared_ptr<MyClass> ptr1(new MyClass()); std::shared_ptr<MyClass> ptr2 = ptr1; // Both ptr1 and ptr2 share ownership
-
weak_ptr: This pointer holds a non-owning reference to an object managed by shared_ptr, preventing circular references that can lead to memory leaks.
Using smart pointers greatly simplifies memory management, effectively preventing leaks and ensuring safety through automatic deallocation.
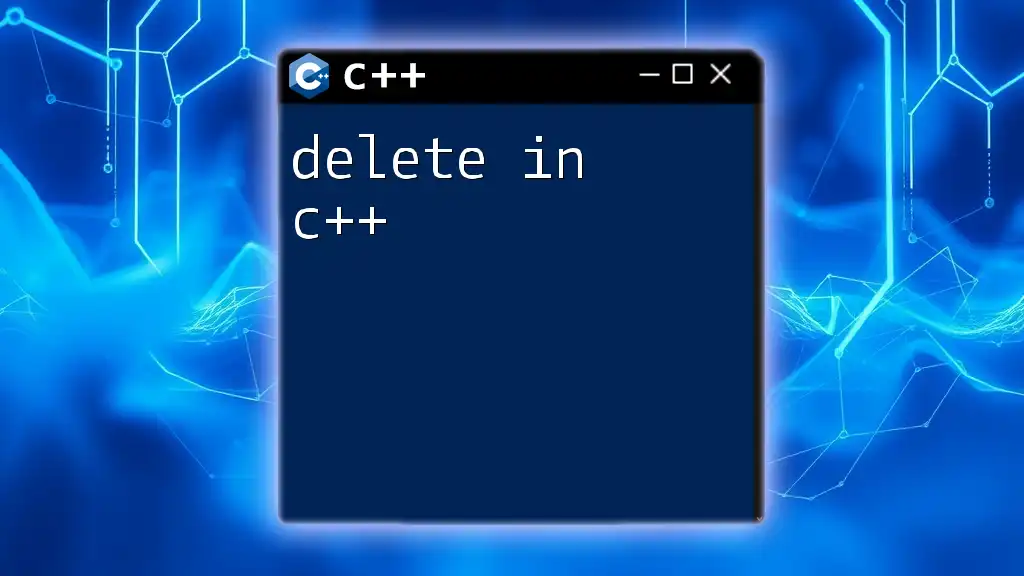
Common Memory Management Mistakes
Even experienced programmers can make mistakes in memory management that lead to significant issues.
Memory Leaks
Memory leaks occur when allocated memory is never deallocated. Identifying memory leaks is crucial as they can accumulate and degrade performance. Tools like Valgrind can help in detecting such leaks.
Dangling Pointers
A dangling pointer is a pointer that does not point to a valid object anymore, typically due to deallocation. Accessing a dangling pointer can lead to unpredictable behavior or crashes. To illustrate:
MyClass* obj = new MyClass();
delete obj; // obj is now a dangling pointer
Double Deletion
Double deletion refers to attempting to delete the same memory location more than once, causing undefined behavior. Always ensure that pointers are set to `nullptr` after deletion to avoid this issue:
MyClass* obj = new MyClass();
delete obj;
obj = nullptr; // Safe practice to avoid double deletion
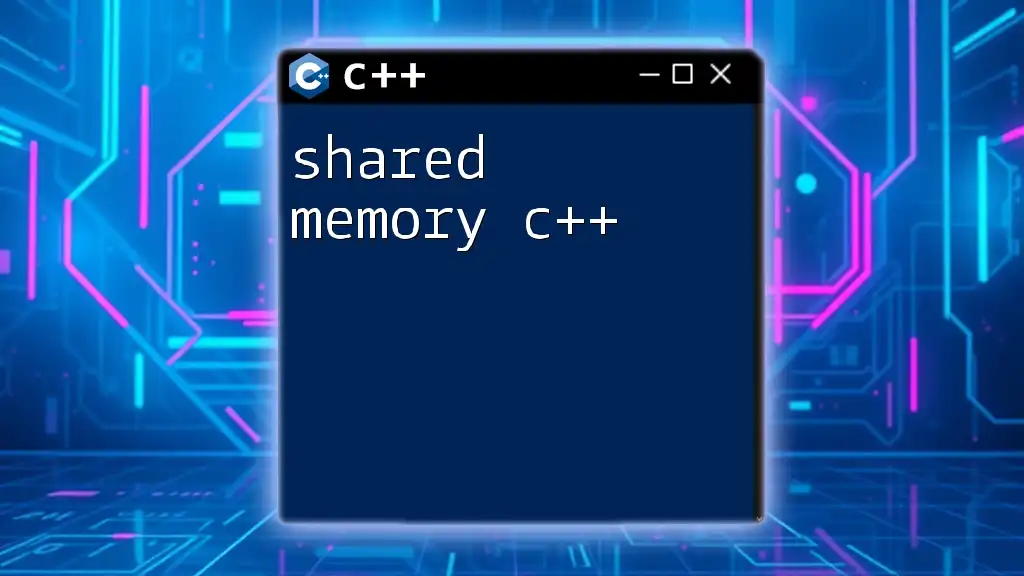
Best Practices for Memory Deallocation in C++
To effectively manage memory in C++, follow these best practices:
- Always pair `new` with `delete` and `new[]` with `delete[]` to ensure that memory is correctly deallocated.
- Utilize smart pointers wherever appropriate to automate memory management and reduce human error.
- Test and debug your programs rigorously to catch memory-related issues early on, utilizing tools designed to detect memory leaks and errors.
- Make a habit of initializing pointers to `nullptr` after deletion to prevent dangling pointers.
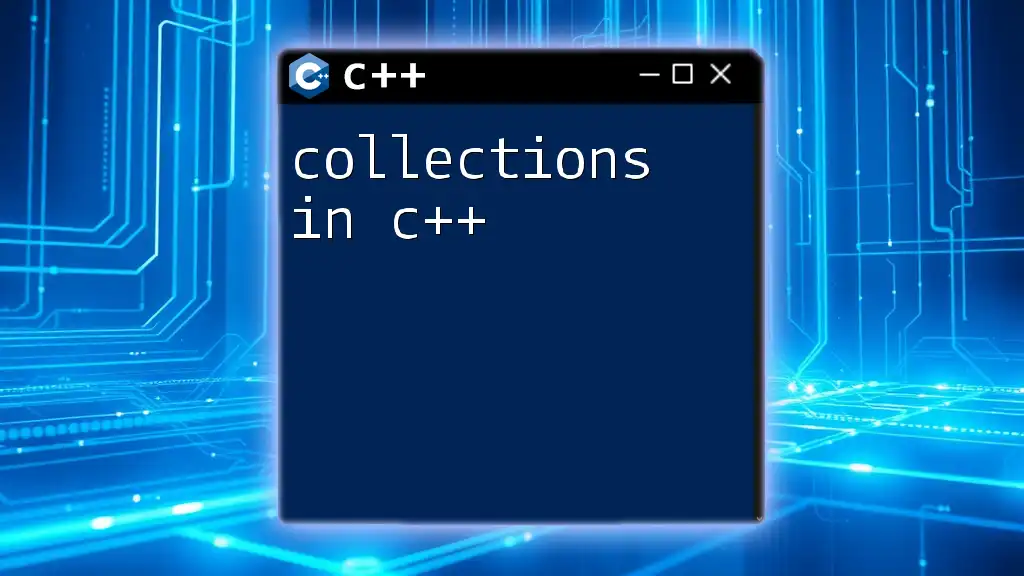
Conclusion
Understanding how to deallocate memory in C++ is vital for writing efficient, robust programs. By mastering the use of the `delete` operator, implementing smart pointers, and adhering to best practices, developers can manage memory effectively and prevent common pitfalls associated with memory management. Practicing these techniques will empower programmers to create high-quality, performance-optimized applications.
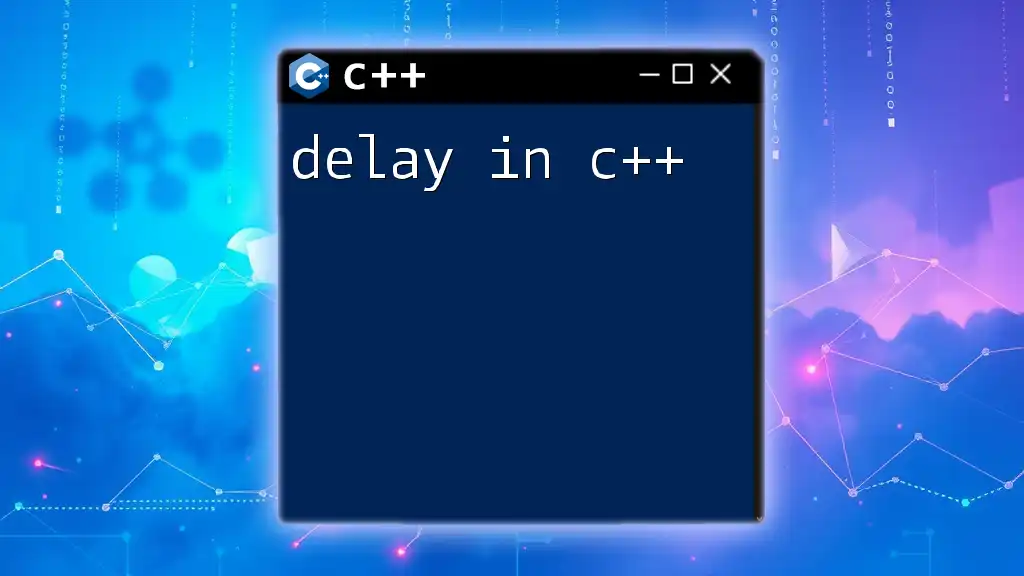
Additional Resources
For further exploration of memory management in C++, consider diving into the official C++ documentation, comprehensive tutorials available online, or advanced texts focusing on memory issues and optimization strategies.