To execute a program in C++, you need to compile the source code using a C++ compiler, such as g++, and then run the resulting executable from the command line.
Here's a code snippet demonstrating the compilation and execution process:
g++ -o myProgram myProgram.cpp
./myProgram
Understanding C++ Execution Environment
Development Environments
To successfully execute a C++ program, it's essential to understand the available development environments. The two main categories are Integrated Development Environments (IDEs) and Command Line Interfaces (CLI).
Integrated Development Environments (IDEs)
IDEs like Visual Studio, Code::Blocks, and CLion provide a comprehensive environment for writing, compiling, and executing C++ programs.
- Benefits of using IDEs include:
- Code suggestions and syntax highlighting that improve coding efficiency.
- Debugging tools that help identify and resolve errors with ease.
- Build management features for easier project organization.
Command Line Interfaces (CLI)
Using a CLI is another effective way to work with C++. This method is often favored by more advanced users who prefer greater control over the build process. Popular compilers include GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++).
Setting Up Your C++ Environment
Before running a C++ program, you need the right tools in place.
Required Tools for C++ Development
-
Compiler Installation
- For Windows, consider installing MinGW to get GCC or use the Visual Studio suite.
- On Linux, you can usually install GCC from your package manager with a command like:
sudo apt-get install g++
- For Mac users, Clang comes pre-installed with Xcode.
-
Choosing Between Text Editors and IDEs
- Simple text editors like Notepad++ or Sublime Text are effective for writing small programs. However, IDEs are recommended for larger projects due to their comprehensive features.
Setting Environment Variables
Setting your environment variables ensures the system can locate your C++ compiler.
- For Windows:
- Open the System Properties window, navigate to Environment Variables, and add the path to your compiler (e.g., `C:\MinGW\bin`).
- For Unix-based systems:
- You can modify your `.bashrc` or `.bash_profile` file to include:
export PATH=$PATH:/usr/local/bin
- You can modify your `.bashrc` or `.bash_profile` file to include:
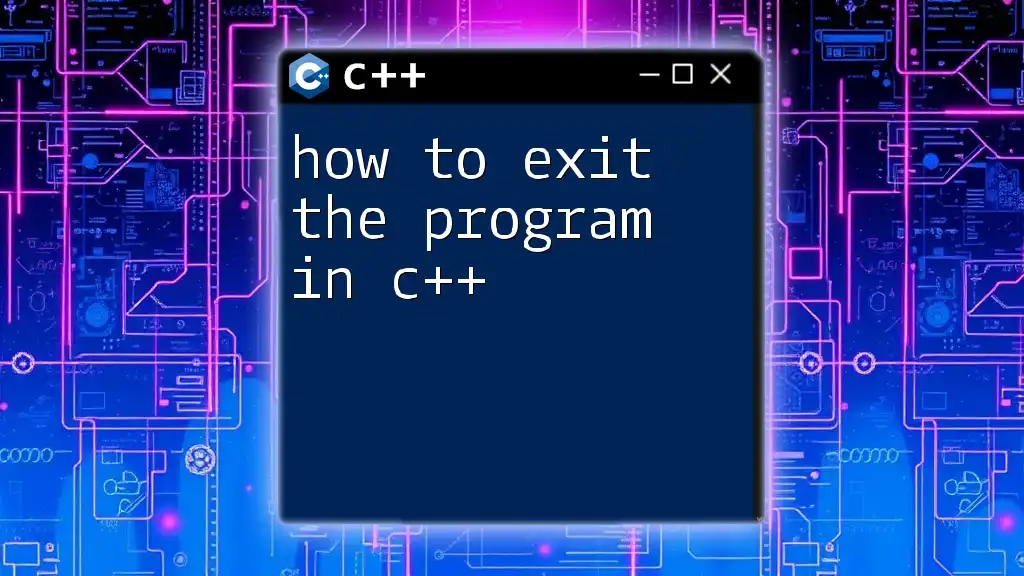
Writing Your First C++ Program
Choosing Your Editor
You can opt for lightweight text editors or robust IDEs based on your preference. Some popular choices include:
- Text Editors: Notepad++, Sublime Text, Visual Studio Code
- IDEs: Visual Studio, Code::Blocks, CLion
Writing a Sample Program
Here’s how to write a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet:
- `#include <iostream>` allows the program to use input and output streams.
- `int main()` defines the entry point of the program.
- `std::cout << "Hello, World!" << std::endl;` outputs the text "Hello, World!" to the console.
- `return 0;` signifies that the program ended successfully.
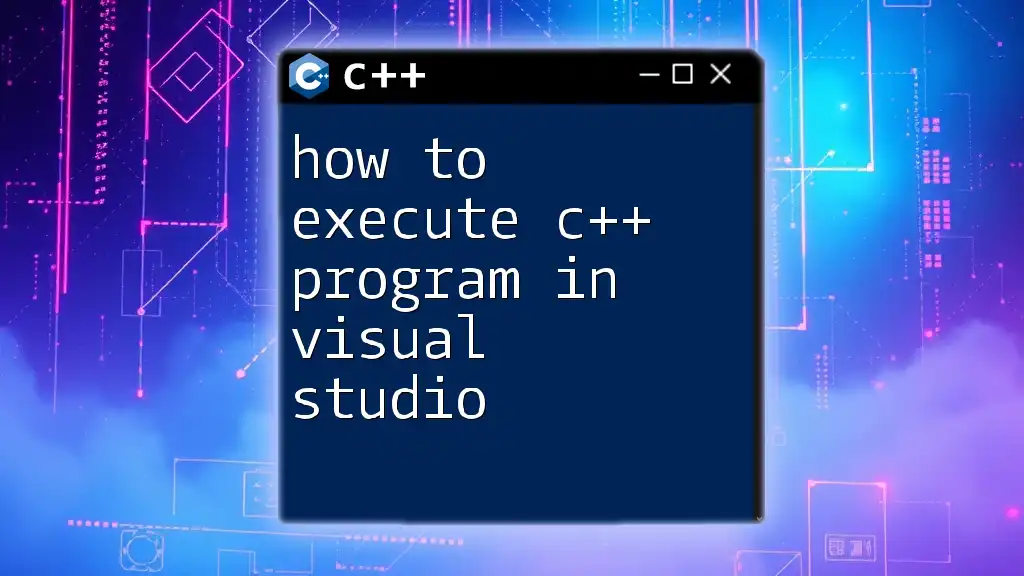
Compiling C++ Code
Using an IDE
If you’re using an IDE, the process for compiling your program is usually straightforward. For example, in Visual Studio:
- Open your project and write the code.
- Click on the Build option in the menu.
- Select Build Solution. The IDE will compile your code and check for errors.
Using Command Line
To compile your C++ code via the command line, follow these steps:
- Navigate to the directory where your `.cpp` file is saved.
- Use the following command if you are using GCC:
g++ -o HelloWorld HelloWorld.cpp
Here’s what the command does:
- `g++` indicates that you are using the GNU C++ compiler.
- `-o HelloWorld` specifies the name of the output file you want to create.
- `HelloWorld.cpp` is your source file.
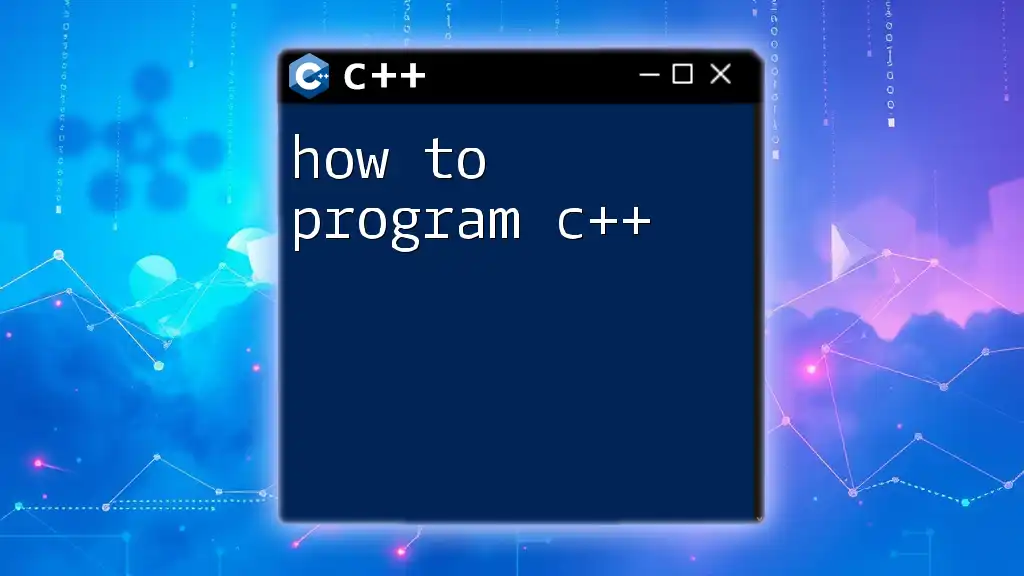
Executing Your C++ Program
Running from an IDE
Running the program from within an IDE is often done with a simple click. For instance, in Visual Studio, you can click on the Start button or press `F5`, and it will execute your program directly.
Running from Command Line
To execute the compiled program using the command line, you simply enter the command corresponding to your operating system:
- For Unix-based systems:
./HelloWorld
- For Windows:
HelloWorld.exe
Executing these commands should display the output of your program in the console.
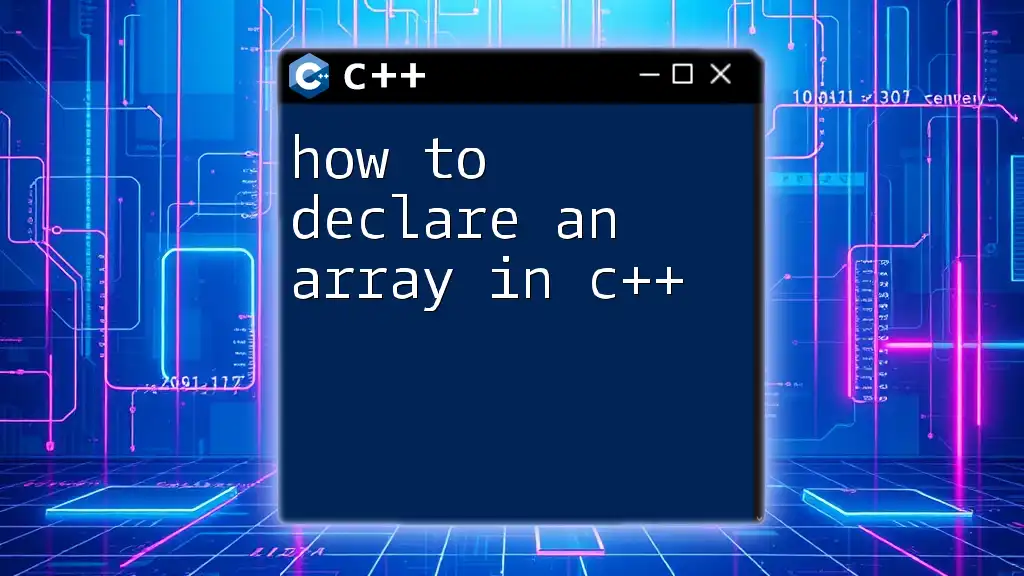
Debugging Common Issues
Troubleshooting Compilation Errors
Compilation errors are common, especially for beginners. Here are some typical issues and how to address them:
- Syntax Errors: Ensure that all your braces and parentheses are correctly paired.
- Missing Files: Confirm that your source file exists in the directory you're compiling from.
- Linking Problems: These errors usually indicate that you're calling a function or using a library that hasn't been included.
Runtime Errors and Debugging
Runtime errors occur when the program is executed. Common runtime problems can include dereferencing null pointers or array index out-of-bounds errors.
Basic debugging techniques include:
- Using breakpoints in an IDE to pause execution and inspect variable values.
- Adding `std::cout` statements to trace the program's execution flow.
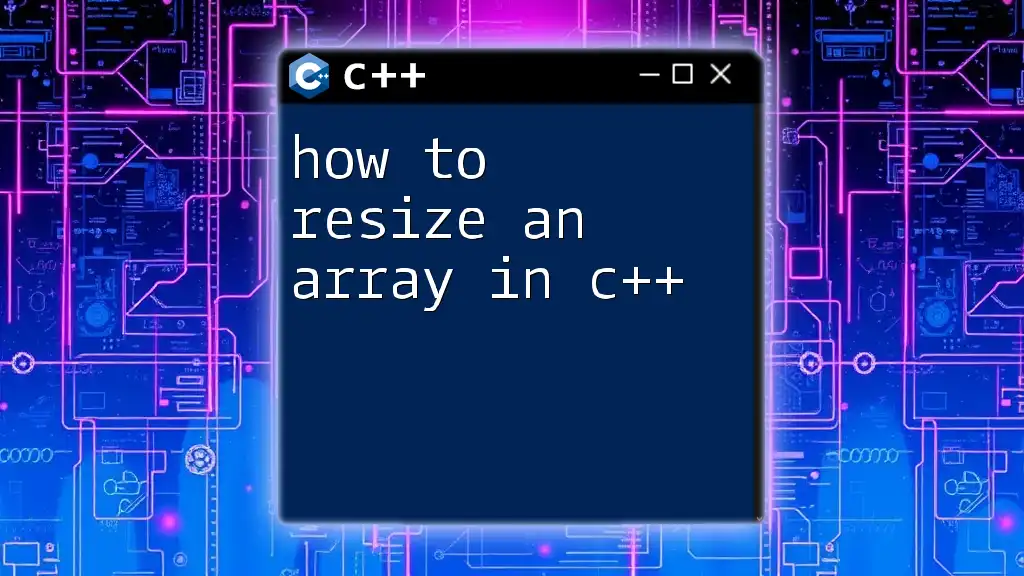
Conclusion
Learning how to execute a program in C++ is a fundamental step toward becoming proficient in C++ programming. Following the outlined steps, from setting up your environment to writing, compiling, and executing your first program, lays a solid foundation for more advanced topics. Practice regularly and explore further resources to enhance your skills.
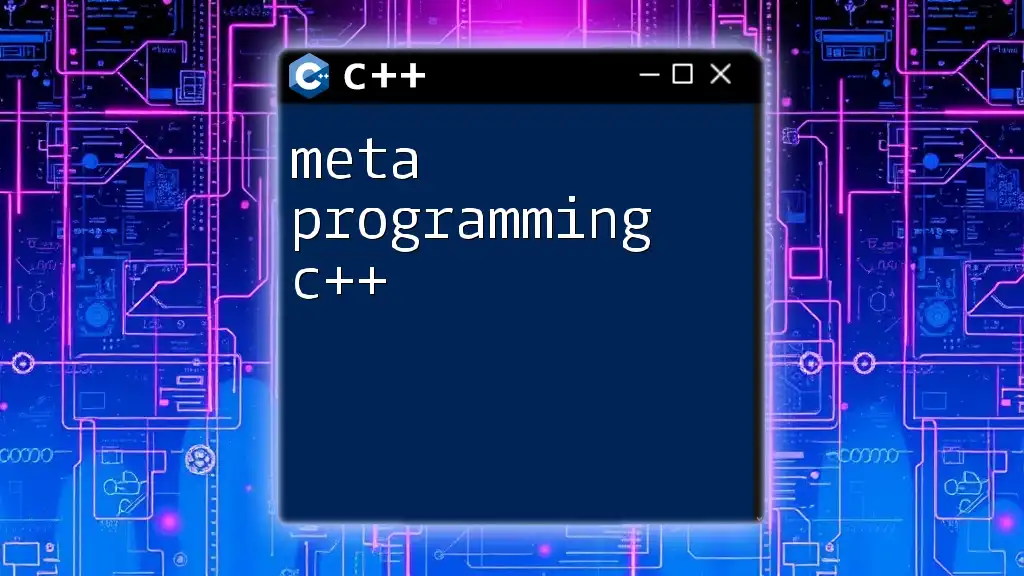
FAQs
Can I run C++ programs on any operating system?
Yes, C++ is a cross-platform language. With the appropriate compiler installed, you can run C++ programs on Windows, macOS, and Linux.
What should I do if my program doesn't compile?
If your program fails to compile, start by checking basic syntax errors or ensuring that all necessary files are present. Compiler error messages often provide clues on how to fix the issues.
Is it better to use an IDE or command line for C++?
The choice between using an IDE or the command line depends on your comfort level. IDEs offer more helpful features for debugging and project management, while the command line provides direct control and can encourage a deeper understanding of the compilation process.