In C++, a `map` is a container that stores key-value pairs, allowing for efficient data retrieval based on unique keys, and can be used as shown in the following code snippet:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap;
myMap["apple"] = 1;
myMap["banana"] = 2;
std::cout << "Apple count: " << myMap["apple"] << std::endl;
return 0;
}
What is a Map in C++?
A map in C++ is a container that stores elements in key-value pairs. This means each element consists of a unique key and an associated value, making it a powerful utility for scenarios where you need to lookup values based on specific identifiers.
Differences Between Maps and Other Containers
Maps differ from other C++ containers, like arrays and vectors, in several significant ways:
- Key-Value Structure: While arrays use indices for accessing elements, maps utilize keys. Each key must be unique, allowing for quick data retrieval.
- Automatic Sorting: By default, elements in a map are sorted based on the keys, which helps in optimizing search and retrieval operations.
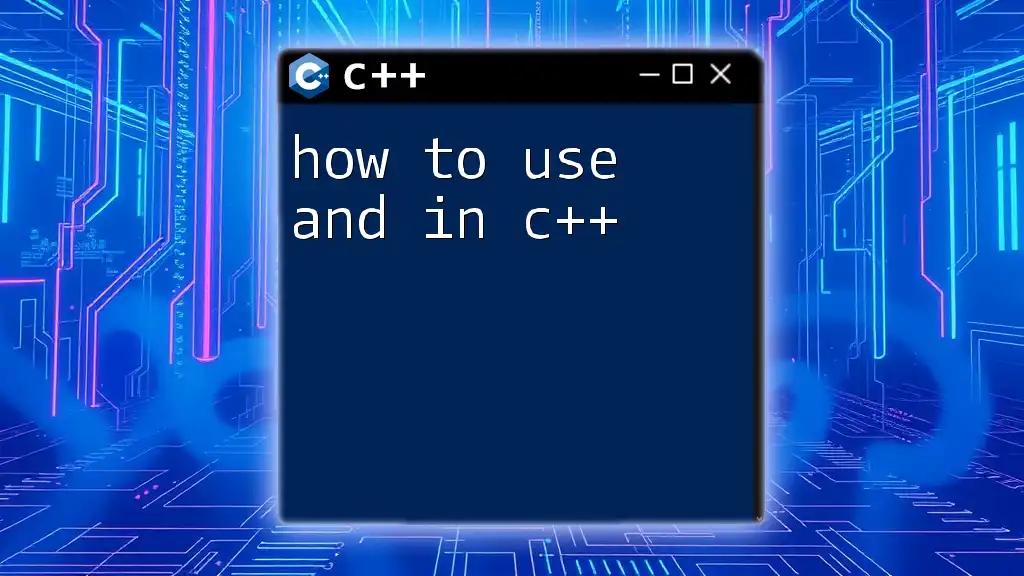
Why Use Maps?
Maps bring several advantages to programming:
- Efficiency: They allow for quick insertion, deletion, and access, typically in logarithmic time complexity.
- Flexibility: You can easily manage a dynamic collection of data.
- Associative Access: With maps, you can easily associate keys with their corresponding values, simplifying data management.
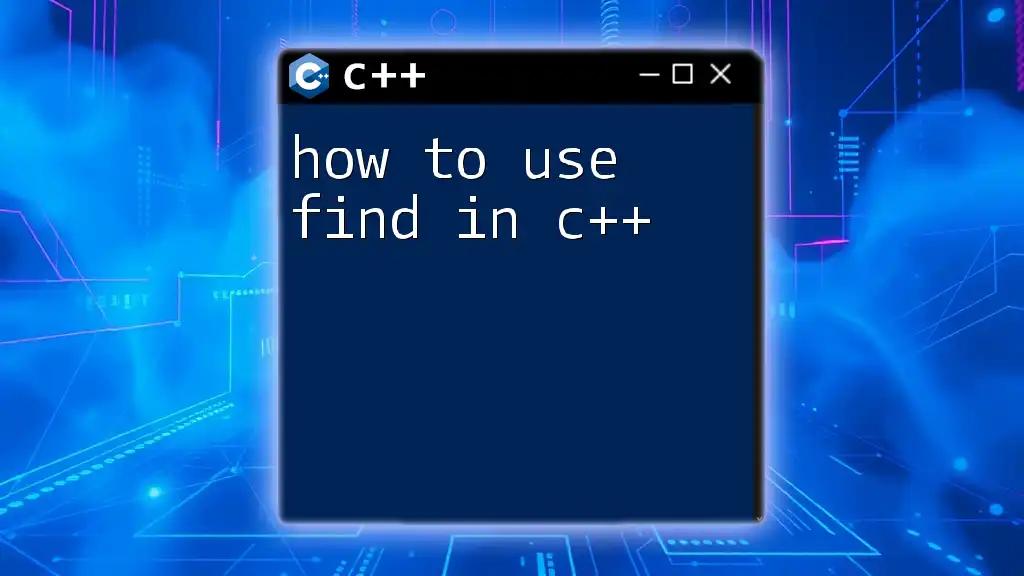
Understanding Key-Value Pairs
In a map, every element is a pair consisting of a key and a value. The key serves as a unique identifier for the associated value.
Importance of Unique Keys
Unique keys are critical in ensuring that you do not overwrite values unintentionally. For instance, if you try to insert a value for an already existing key, the new value will replace the old one.
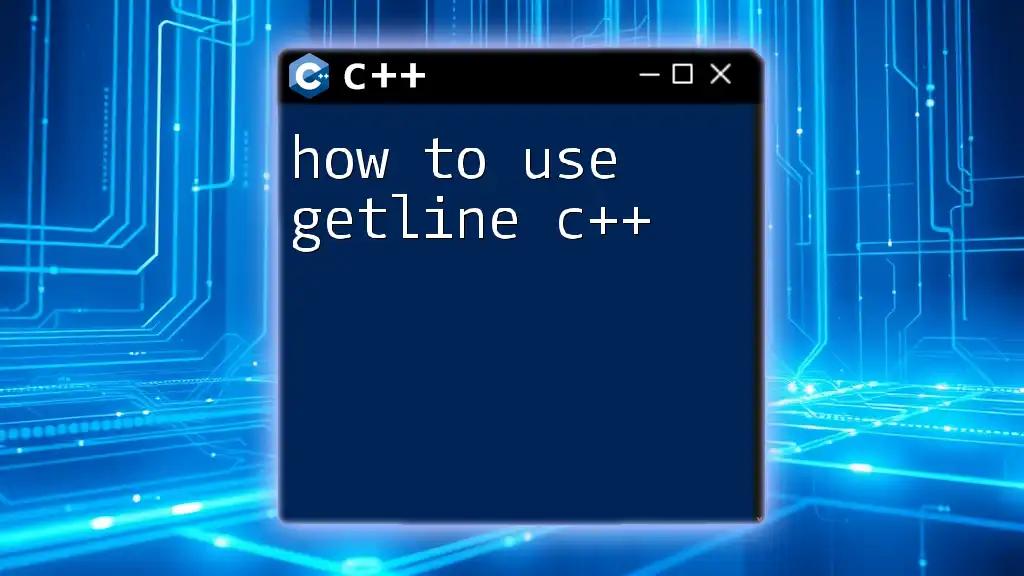
Types of Maps in C++
`std::map`
This is the standard associative container that stores elements in sorted order based on the key. It provides a balanced binary tree structure under the hood, ensuring efficient operations.
`std::unordered_map`
As the name suggests, this type of map does not maintain the order of elements. Instead, it uses hash tables to achieve average constant time complexity for insertions, deletions, and access.
Differences Between Ordered and Unordered Maps
- Order: `std::map` keeps elements sorted, while `std::unordered_map` does not guarantee any specific order.
- Performance: Accessing elements in an unordered map is generally faster than in ordered maps, thanks to hashing.
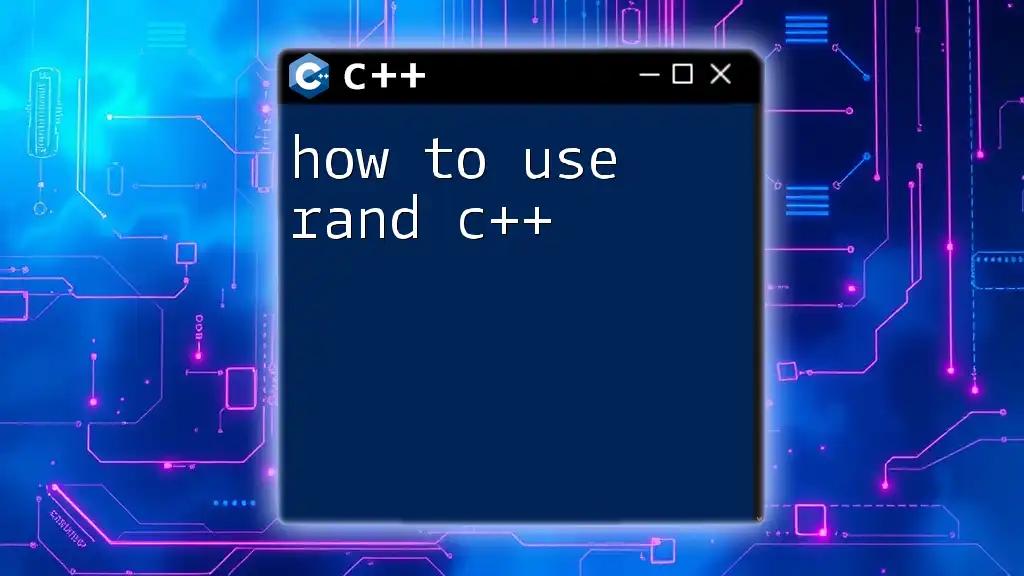
How to Use Maps in C++
Including the Necessary Header
To work with maps in C++, you need to include the `<map>` header. This is essential for utilizing map functionalities.
#include <map>
Declaring a Map
Declaring a map involves specifying the key and value types within angle brackets.
Example of declaring a map:
std::map<std::string, int> ageMap;
In this example, we create a map called `ageMap` where the keys are of type `std::string` and values are of type `int`.
Inserting Elements into a Map
You can insert elements into a map using various methods:
-
Using Operator[]: This allows direct assignment.
ageMap["Alice"] = 30; // Insert Alice with age 30
-
Using `insert()`: This method explicitly adds a key-value pair.
ageMap.insert(std::make_pair("Bob", 25)); // Insert Bob
-
Using `emplace()`: This constructs the element in-place, improving performance.
ageMap.emplace("Charlie", 35); // Insert Charlie
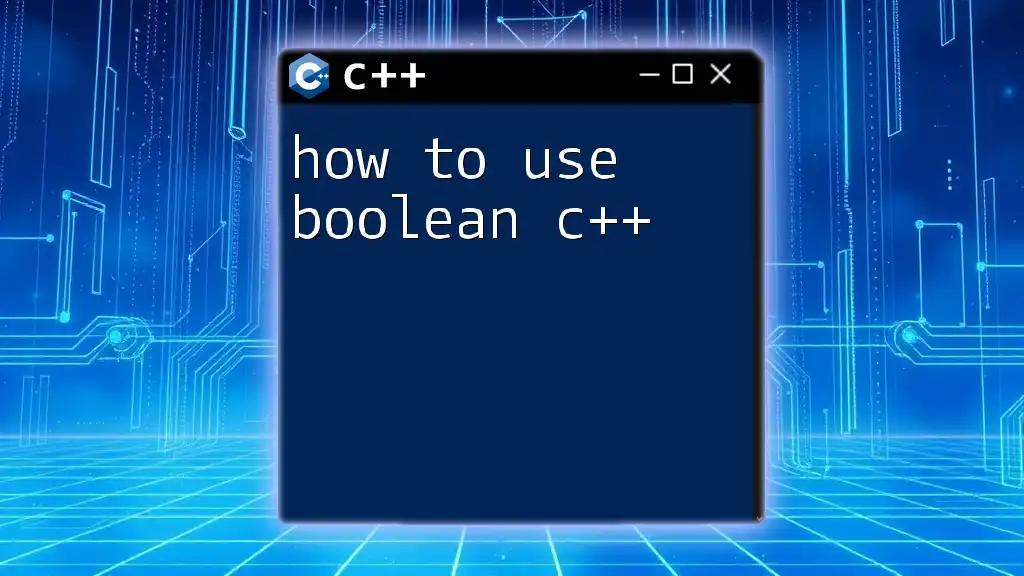
Accessing and Modifying Map Elements
Accessing Elements
You can retrieve values using their corresponding keys easily:
int aliceAge = ageMap["Alice"];
Modifying Elements in a Map
To change values associated with existing keys, simply assign a new value to the specific key:
ageMap["Alice"] = 31; // Update Alice's age to 31
Checking for Key Existence
It’s important to check whether a key exists before accessing or modifying it. You can do this using `find()` or `count()`:
if (ageMap.find("David") != ageMap.end()) {
// Key exists
}

Iterating over a Map
Using Iterators
You can iterate through a map using iterators. This provides flexibility in accessing keys and values:
for (auto it = ageMap.begin(); it != ageMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
Using Range-Based For Loops
Range-based for loops offer a more concise syntax for iterating through maps:
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}

Removing Elements from a Map
Removing Elements by Key
You can delete entries from a map using the `erase()` method:
ageMap.erase("Bob"); // Removes Bob from the map
Clearing the Entire Map
To remove all elements from a map, use the `clear()` method:
ageMap.clear(); // Clears all elements from ageMap

Common Use Cases for Maps in C++
Counting Frequency of Elements
Maps are particularly useful in counting the occurrences of elements in a dataset. Here is an example of counting words:
std::map<std::string, int> wordCount;
for (const auto& word : words) {
wordCount[word]++;
}
Storing Configuration Settings
Maps can also serve as efficient tools for managing application settings, where unique keys are associated with configuration values.

Conclusion
In this article, we've explored how to use a map in C++ thoroughly. From declaring and inserting elements to accessing and modifying data, maps provide an efficient way to manage key-value pairs.
Encouragement to Practice
I encourage you to apply what you've learned about maps in your projects. Hands-on experience will solidify your understanding and open up new possibilities for your coding endeavors.

Additional Resources
For those eager to dive deeper into the topic, consider checking the official C++ documentation and exploring recommended tutorials and books on the C++ Standard Template Library (STL).
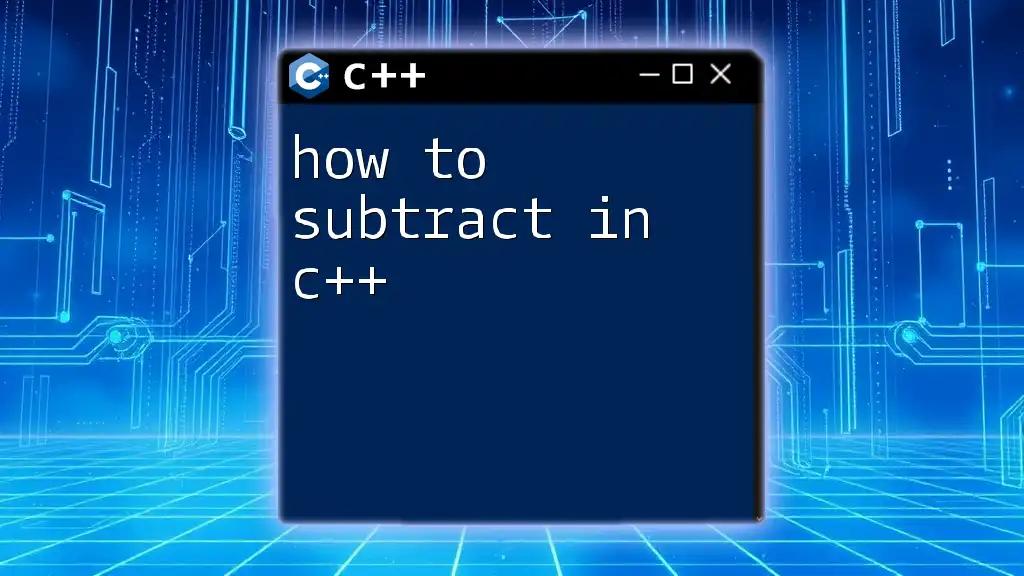
FAQs about Using Maps in C++
What is the difference between `std::map` and `std::unordered_map`?
`std::map` maintains sorted order of elements while `std::unordered_map` uses hashing, making it fundamentally faster for unordered access.
Can a map contain duplicate keys?
No, maps do not allow duplicate keys. If you attempt to insert a duplicate key, the new value will replace the existing one.
How do I convert a map to a vector?
To convert a map to a vector, you may need to traverse the map, collecting the key-value pairs into a vector. This process can be helpful for certain algorithms that operate on arrays.
By following the guidelines outlined in this article, you can confidently incorporate the power of maps into your C++ projects, enhancing both functionality and performance.