In C++, the `rand()` function generates a pseudo-random number, and you can use it by including the `<cstdlib>` header and calling the function after optionally seeding it with `srand()`, as shown in the code snippet below:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed for randomness
int randomNum = rand(); // Generate a random number
std::cout << "Random Number: " << randomNum << std::endl;
return 0;
}
How rand Works in C++
The `rand()` function in C++ serves as a basic pseudo-random number generator. It produces integer values between `0` and `RAND_MAX`, which is a constant defined in `<cstdlib>`. The output of `rand()` is not truly random; rather, it is generated through arithmetic calculations based on an internal state. This means if you call `rand()` multiple times without varying your setup, you'll receive a repeating sequence of numbers.
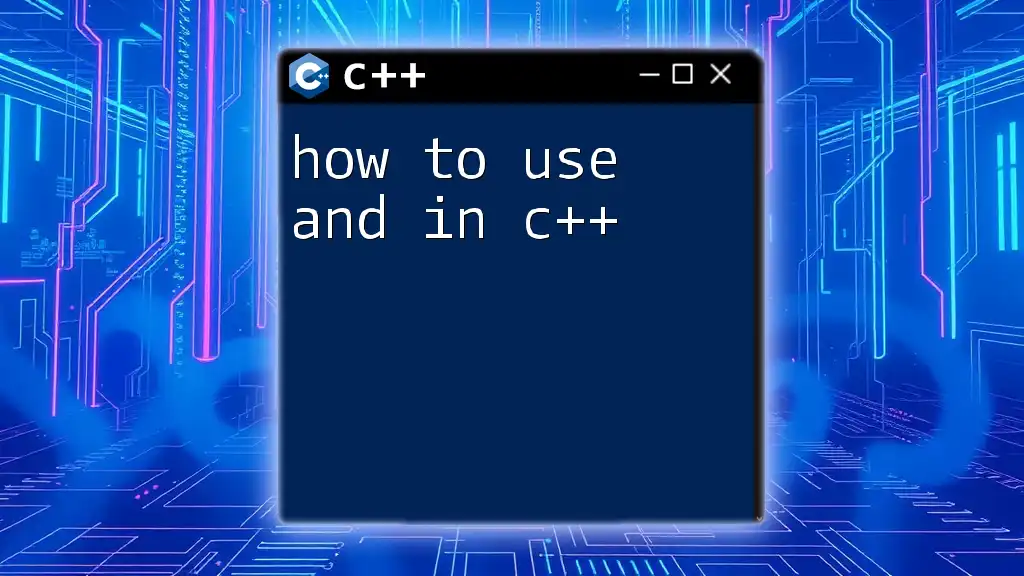
Setting Up rand in Your C++ Environment
To utilize rand(), you need to include the appropriate headers:
#include <cstdlib> // For rand() and srand()
#include <ctime> // For time() function
These headers provide essential functions for random number generation and time handling.
Once these headers are included, you can straightforwardly use `rand()` in your code. Don't forget to compile your program using a modern C++ compiler to ensure compatibility with these functions.
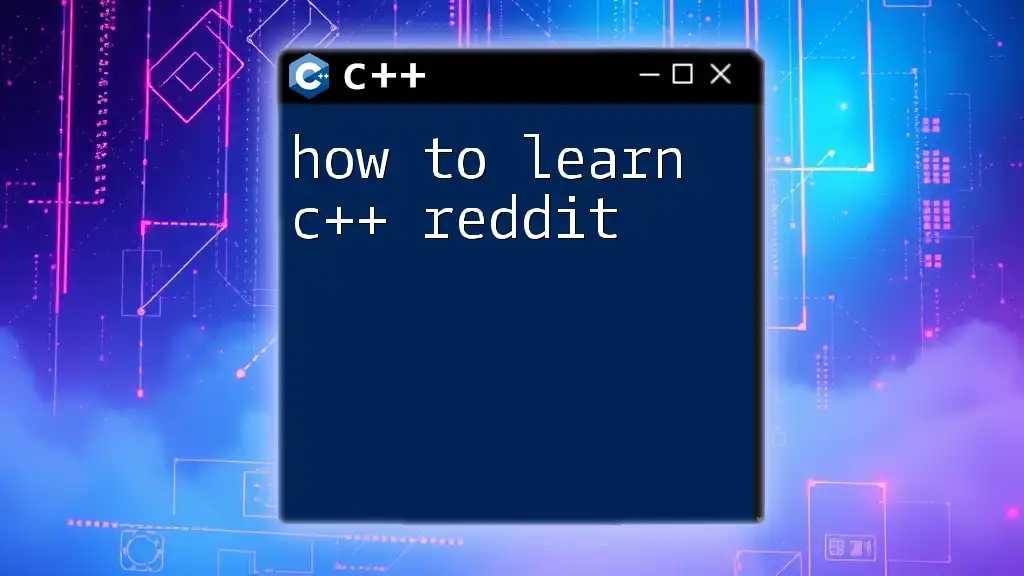
Seeding the Random Number Generator
What is Seeding?
Seeding is the process of initializing the pseudo-random number generator. By default, if you don’t set a seed, `rand()` will return the same sequence of numbers every time you run your program. This predictability can be detrimental in scenarios where randomness is critical, such as in games or simulations.
How to Seed rand in C++
Seeding is accomplished with the `srand()` function. You would typically seed the random number generator with the current time to ensure that you get different random numbers during different executions of your program.
Here’s how you can do it:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator with current time
std::cout << std::rand() << std::endl; // Output a random number
return 0;
}
In this example, using `std::time(0)` as the seed ensures that each program execution results in a different starting point in the sequence generated by `rand()`.
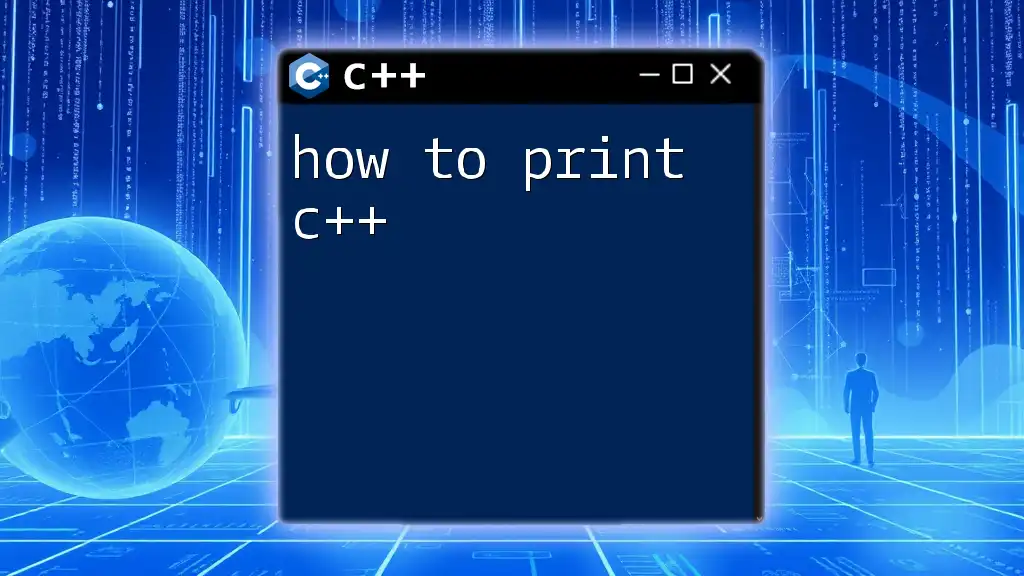
Generating Random Numbers with rand()
Generating Basic Random Numbers
To obtain a basic random value, you simply call `rand()`:
int randomNumber = std::rand();
Important Note: Each call to `rand()` after seeding will return a new number, but the sequence will repeat if the program is run multiple times without reseeding.
Generating Random Numbers Within a Specific Range
Often, you may want to restrict the generated numbers to a specific range. This can be done using the modulus operator to limit the range and then adjusting the result accordingly.
The formula is as follows:
int randomInRange = std::rand() % (max - min + 1) + min;
Here's an example that generates a random number between `1` and `100`:
int randomInRange = std::rand() % 100 + 1; // Random number between 1 and 100
In this code, `std::rand() % 100` generates a value from `0` to `99`, and adding `1` shifts the range to `1` to `100`. This technique enables you to control the output of `rand()` effectively.
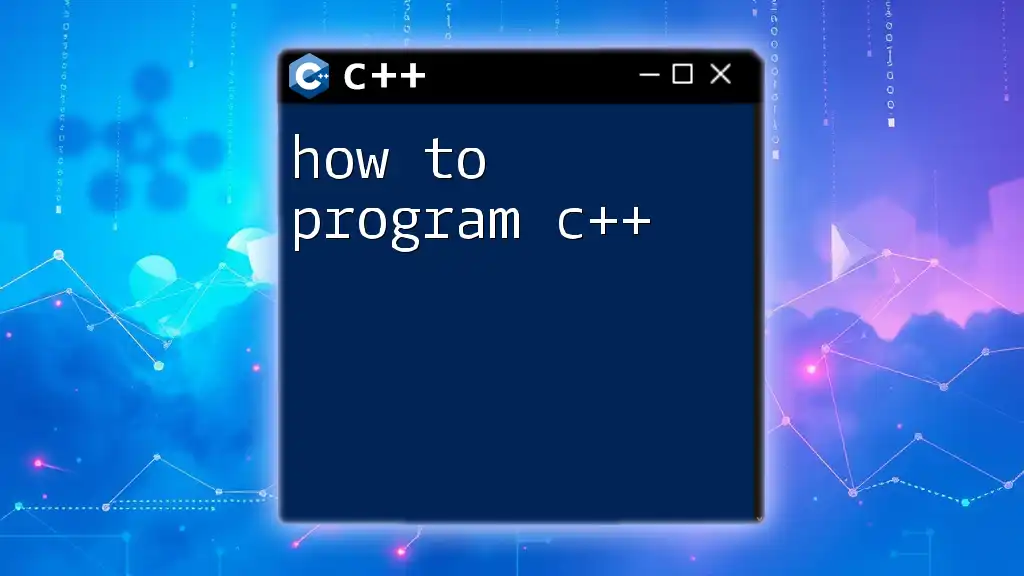
Best Practices for Using rand in C++
Avoiding Common Pitfalls
One critical error developers often make is to forget seeding the generator. If you skip this step, `rand()` will produce the same sequence of numbers each time you run the application. Always remember to seed your random number generator before you make any calls to `rand()`.
Improving Randomness
While `rand()` can handle basic random number generation, it is often better to utilize the modern C++ `<random>` library for improved randomness and flexibility. This library offers more advanced random number generation techniques and various distributions (uniform, normal, etc.).
Consider using `std::mt19937` (a Mersenne Twister algorithm) as a replacement for `rand()` for better randomness and reproducibility of results:
#include <random>
int main() {
std::random_device rd; // Obtain a random number from hardware
std::mt19937 eng(rd()); // Seed the generator
std::uniform_int_distribution<> distr(1, 100); // Define the range
std::cout << distr(eng); // Generate numbers in range
return 0;
}
The `std::random` library empowers you to customize distributions that better suit the needs of your program, enhancing both the randomness and structure of the output values.
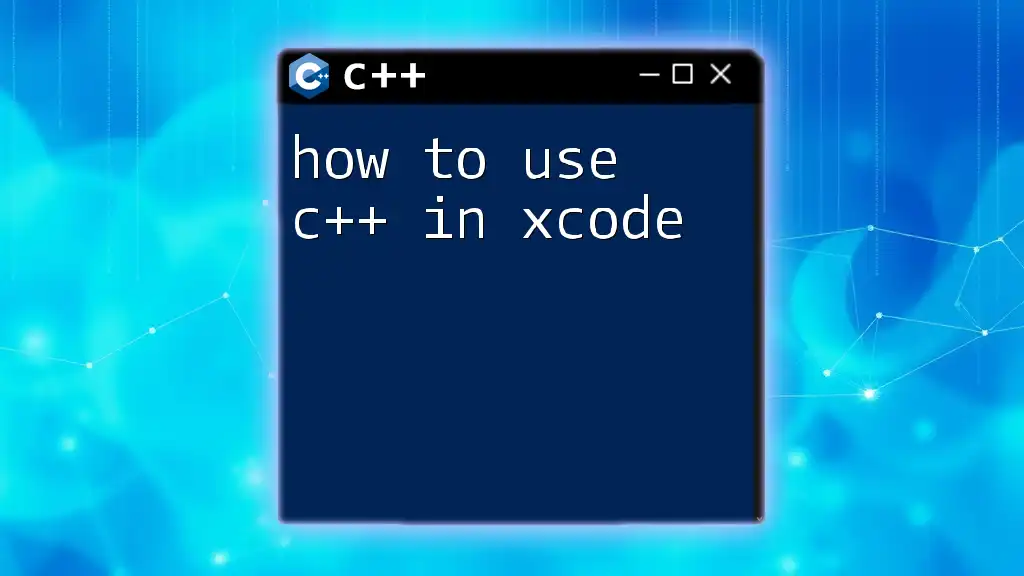
Conclusion
In summary, understanding how to use rand in C++ is crucial for beginners looking to implement random number generation. Remember to always seed your generator for varied results, and explore advanced methods provided by the `<random>` library for improved performance and flexibility. Aim to experiment with various uses of random numbers in your C++ projects to grasp the concepts further!
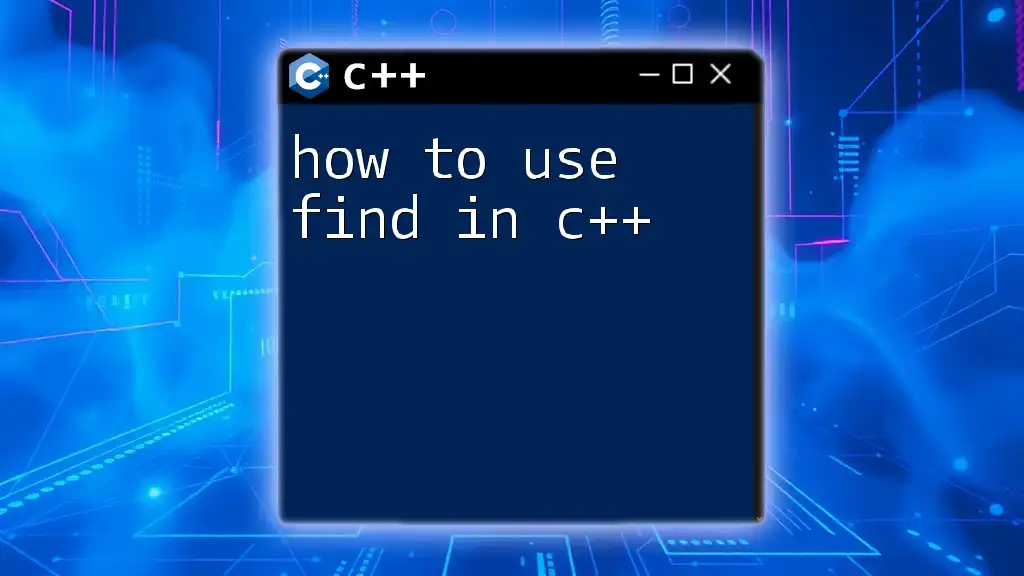
Additional Resources
For comprehensive details, consult the C++ documentation for `<cstdlib>` and `<ctime>`, and delve deeper into advanced random number techniques available in C++. Happy coding!