To use C++ in Xcode, create a new project, select the C++ template, and write your code in the `main.cpp` file, as shown in the example below:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with Xcode for C++
Overview of Xcode
Xcode is Apple’s integrated development environment (IDE) designed for macOS. It provides a powerful suite of tools for developing software across Apple's platforms, including iOS, macOS, watchOS, and tvOS. For C++ programmers, Xcode’s features—such as its code editor, debugging environment, and project management abilities—make it a robust choice.
Xcode supports C++ development through its built-in support for the Clang compiler, easy project configuration, and a graphical interface that simplifies various programming tasks.
System Requirements
Before diving into using C++ in Xcode, ensure that your machine meets the minimum system requirements:
- macOS: A compatible version (make sure to install the latest version).
- Hardware: A Mac with at least 4GB of RAM and 10GB of available space.
- Xcode Installation: Download Xcode from the Mac App Store.
Make sure to keep Xcode updated to benefit from the latest features and improvements in C++ support.
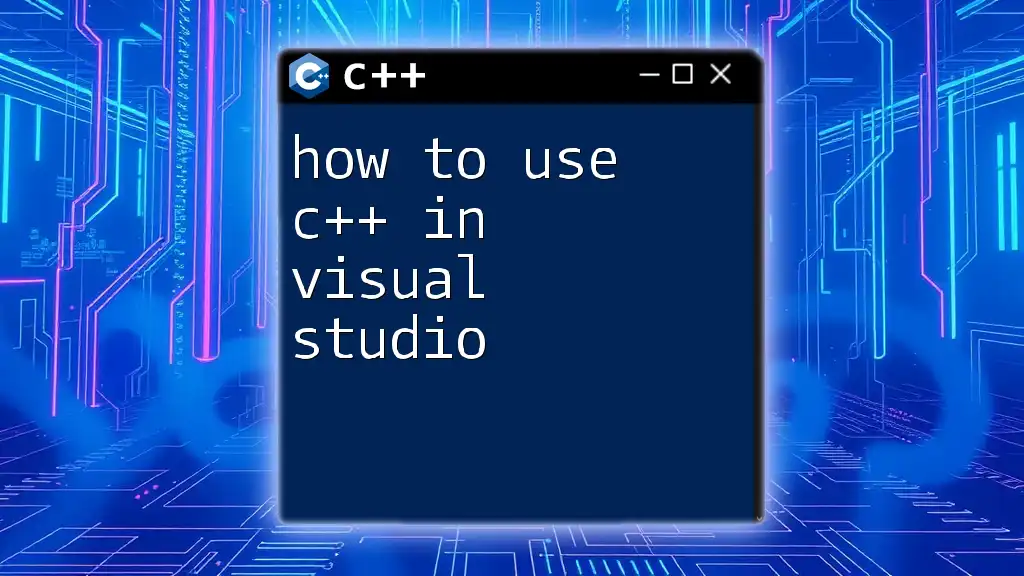
Setting Up a C++ Project in Xcode
Creating a New Project
To start your journey in learning how to use C++ in Xcode, the first step is creating a new project:
- Launch Xcode: Open Xcode from your applications menu.
- Create a New Project: Click on “Create a new Xcode project.”
- Select a Template: In the template chooser, select “macOS” and then pick “Command Line Tool.” This template is ideal for C++ development as it sets up a basic structure suitable for console applications.
Choosing the correct template is crucial as it will handle the necessary configurations for your C++ environment.
Configuring Project Settings
After creating your project, it’s important to configure your settings correctly to ensure smooth development:
- Project Settings: Go to the project settings by selecting the project name in the navigator.
- Choosing Compiler Options: Ensure that the “C++ Language Dialect” is set to the version of C++ you wish to use (C++11, C++14, C++17, etc.).
- Build Targets: Make sure your target platform is set correctly, especially if you plan to develop for multiple platforms in the future.
Proper configuration at this stage will save you from potential headaches later.
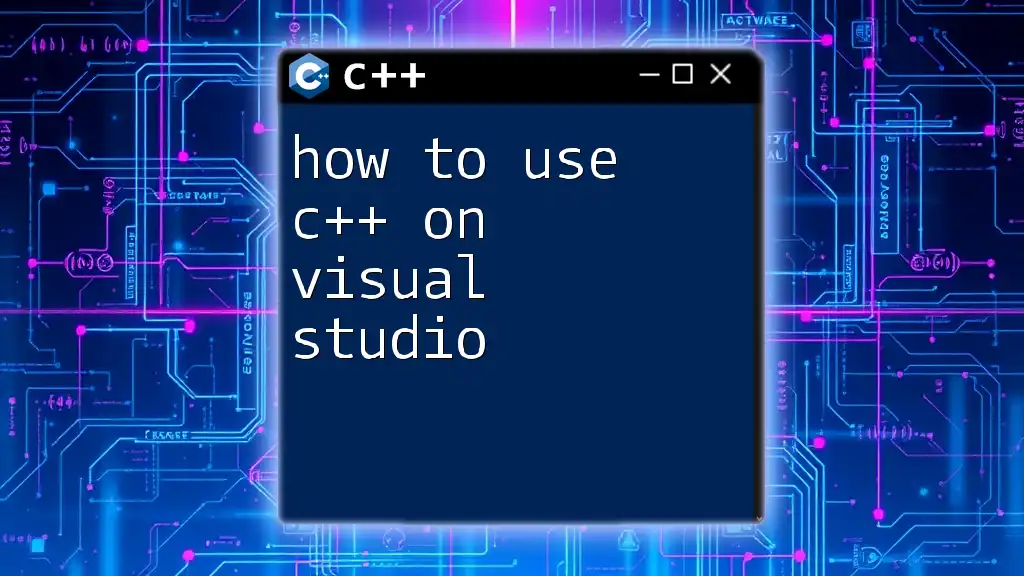
Writing Your First C++ Program in Xcode
Writing the Code
With your project set up, you can start writing your first C++ program. A simple “Hello, World!” program is the perfect way to begin:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- #include <iostream>: This line includes the Input/Output stream library, which is necessary for using `std::cout`.
- int main(): The starting point of any C++ program.
- std::cout: Outputs the string to the console.
Compiling and Running Your Code
Once you’ve written your code, it’s time to build and run your project.
- Build Your Project: Click on the “Product” menu and select “Build” or use the shortcut Command + B. Xcode compiles the code and checks for any errors.
- Run the Application: After a successful build, run your application by selecting “Run” from the Product menu or using Command + R. The output can be viewed in the console at the bottom of the Xcode window.
By following these steps, you will experience the instant gratification of seeing your code in action.
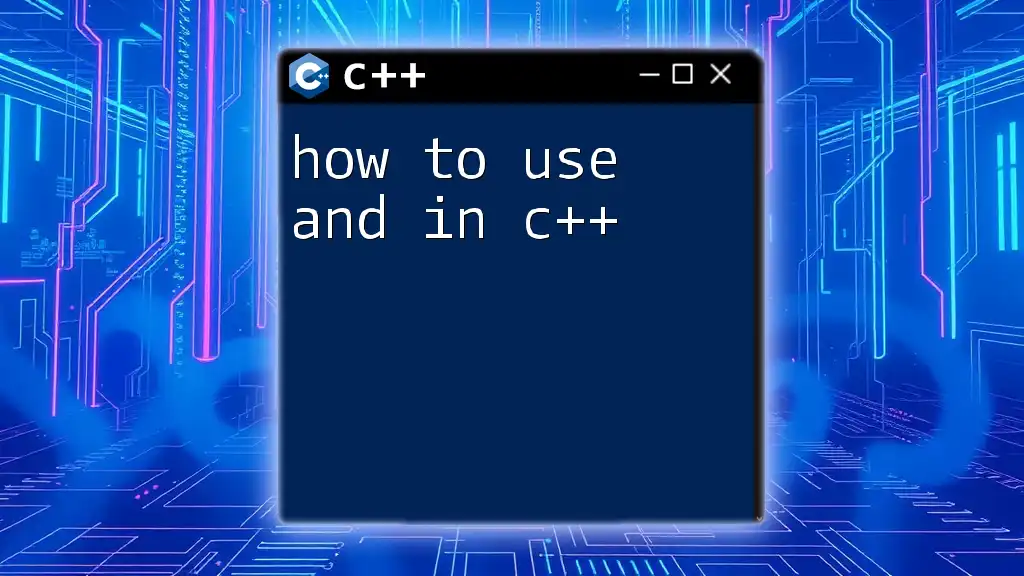
Debugging C++ Code in Xcode
Introduction to Xcode’s Debugger
Debugging is an essential part of programming, and Xcode offers a powerful built-in debugger that enables you to troubleshoot problems effectively. Understanding how to utilize these tools efficiently is key to mastering C++ in Xcode.
Using the Debugger with C++
To effectively debug your C++ code in Xcode, follow these steps:
- Set Breakpoints: Click on the line number where you want to stop execution during debugging. This helps in examining the program state at specific points.
- Start Debugging: Run your project in debug mode. The debugger will pause execution at the breakpoints you’ve set.
- Inspect Variables: Use the variable navigator to check the value of variables in real-time. This can help pinpoint logic errors or incorrect values.
For example, if you modify your "Hello, World!" program to multiply two numbers and want to check the product, set breakpoints and step through the execution to observe the intermediate results.
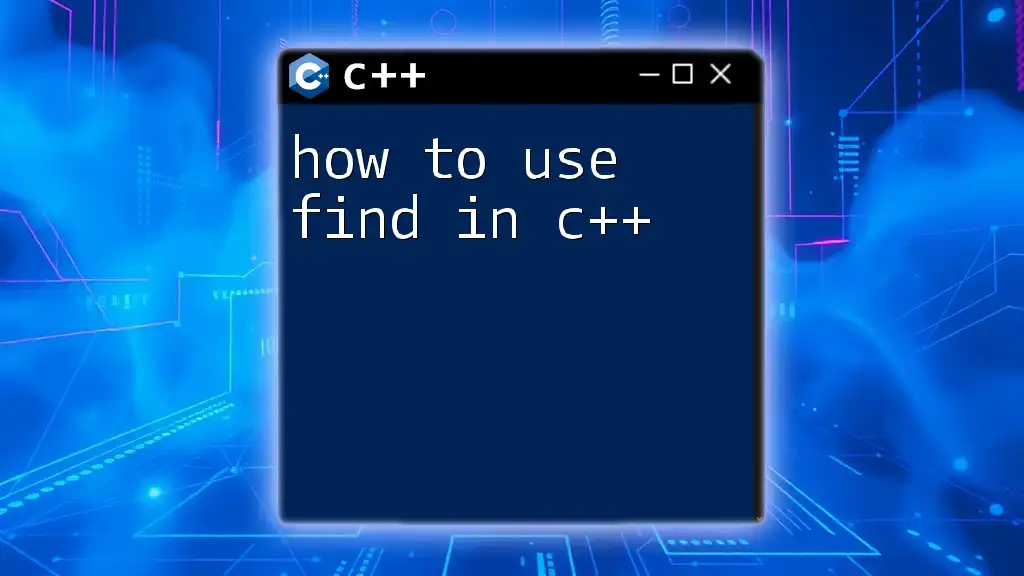
Utilizing C++ Libraries in Xcode
Adding External Libraries
Often, your C++ projects will require additional libraries to enhance functionality. Here’s how to link external libraries in Xcode:
- Download the Library: For this example, let’s say you want to use the Boost library. Ensure you download the appropriate binaries for macOS.
- Include the Library: In your Xcode project, navigate to Project Settings → Build Settings, and locate the “Header Search Paths” section. Add the path to your library headers.
- Link the Library: Under "Link Binary with Libraries," add the required library binaries.
Example: Using a C++ Library
Here’s a quick demonstration of how to include and use the Boost library in your project:
#include <boost/algorithm/string.hpp>
#include <iostream>
int main() {
std::string str = "Boost Libraries";
boost::to_upper(str);
std::cout << str << std::endl; // Outputs: BOOST LIBRARIES
return 0;
}
This snippet showcases utilizing an external library to manipulate strings, demonstrating how you can extend the capabilities of your C++ applications in Xcode.
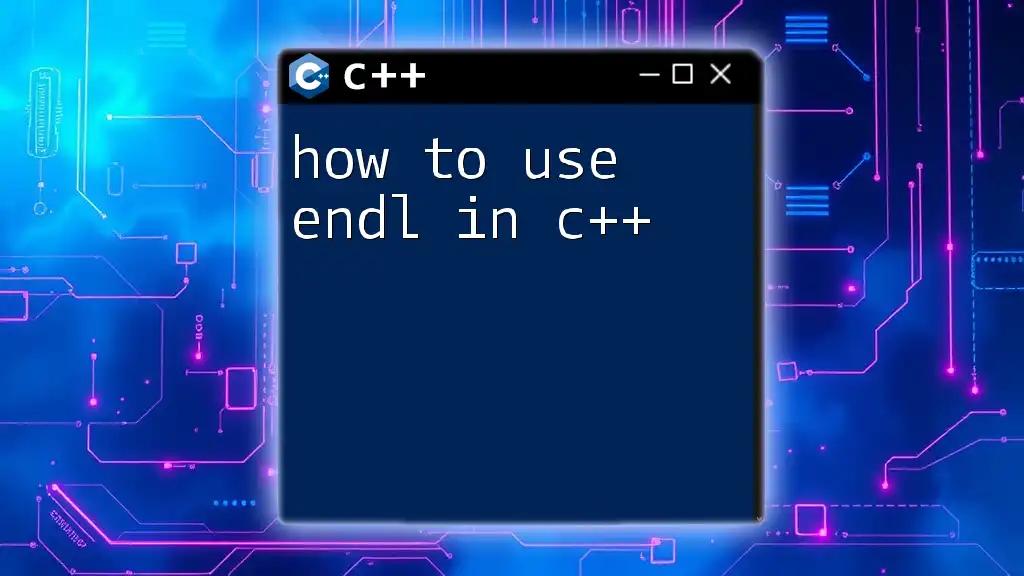
Best Practices for C++ Development in Xcode
Code Organization
Keeping your project organized is vital for maintainability. Always separate your header files and source files into dedicated folders. Naming conventions should be consistent, and each class should have its own header and corresponding source file.
Version Control with Xcode
Integrating version control into your workflow is essential. Xcode supports Git natively, allowing you to track changes, create branches, and manage merges right within the IDE. This is crucial for collaboration and maintaining an efficient development process.
Performance Optimization Tips
To ensure your applications run smoothly, consider profiling your C++ code. Xcode includes instruments for monitoring performance, which can help identify bottlenecks in your application. Optimize your code based on profiling results—avoid common pitfalls like excessive memory allocations or inefficient algorithms.
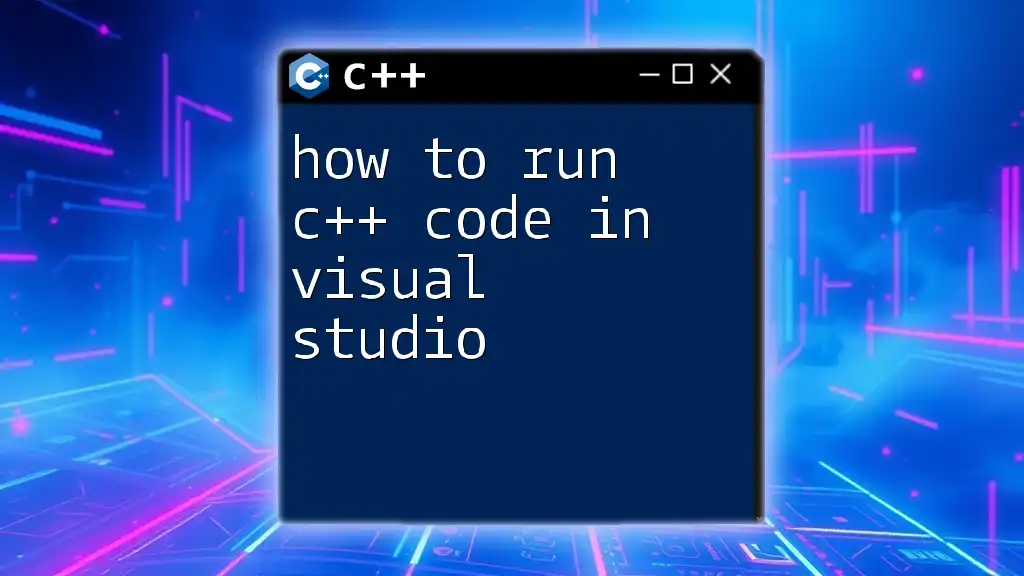
Troubleshooting Common Issues in Xcode with C++
Compilation Errors
It is common to encounter compilation errors when using C++. These errors can stem from syntax mistakes to misconfigured settings. Always pay close attention to the error messages Xcode provides; they are often indicative of where the problem lies.
Runtime Issues
After successfully compiling, you might still face runtime issues. Common problems include segmentation faults and logic errors. When troubleshooting, utilize the debugger to inspect variable states and control flow to help identify the problem.
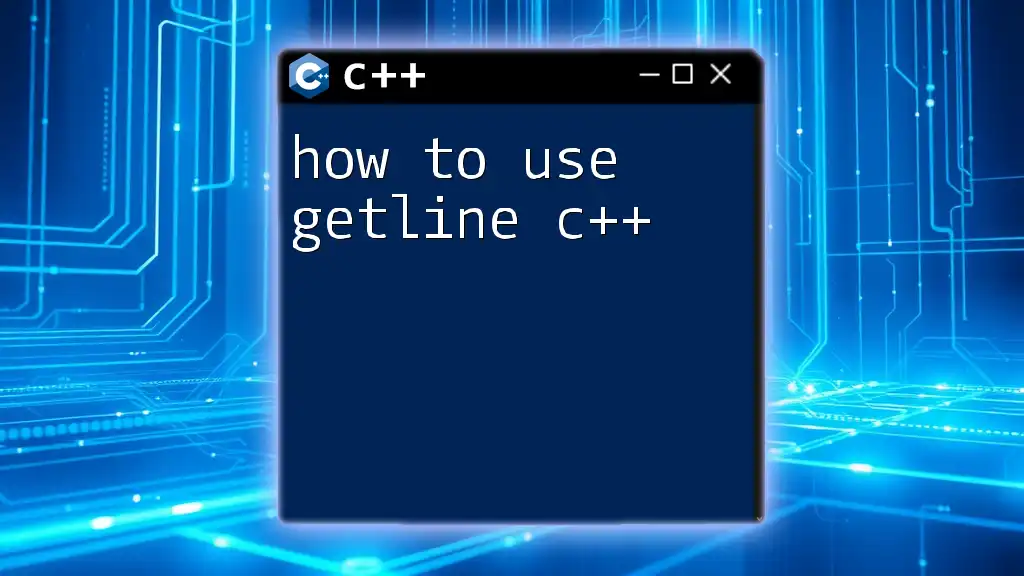
Conclusion
In this guide, we've covered the essential steps on how to use C++ in Xcode, starting from project setup to debugging and library utilization. By following these instructions and best practices, you’re well on your way to becoming proficient in C++ development within Xcode. As you continue learning, don’t explore resources beyond this guide to further enhance your understanding and skills in C++.