To code in C++ on a Mac, you can use the Xcode IDE or install a text editor along with the Terminal for compilation, and here's a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your Development Environment
Installing Xcode
To begin coding in C++ on your Mac, installing Xcode is essential. Xcode is Apple's Integrated Development Environment (IDE) that provides tools for software development, including a powerful source editor, debugger, and compiler for C++.
Step-by-step installation
- Accessing the App Store: Open the App Store on your Mac and search for "Xcode".
- Download and Installation Process: Click "Get" and then "Install App". The download may take some time, depending on your internet speed.
Once installed, you can launch Xcode to start creating your C++ projects.
Configuring Xcode for C++
After opening Xcode, follow these steps to create a new C++ project:
- Creating a New Project: Click on "Create a new Xcode project".
- Selecting C++ as the Preferred Language: Choose "macOS" and then select "Command Line Tool". In the next dialogue, set the language to "C++". Enter a project name and choose a location to save your project files.
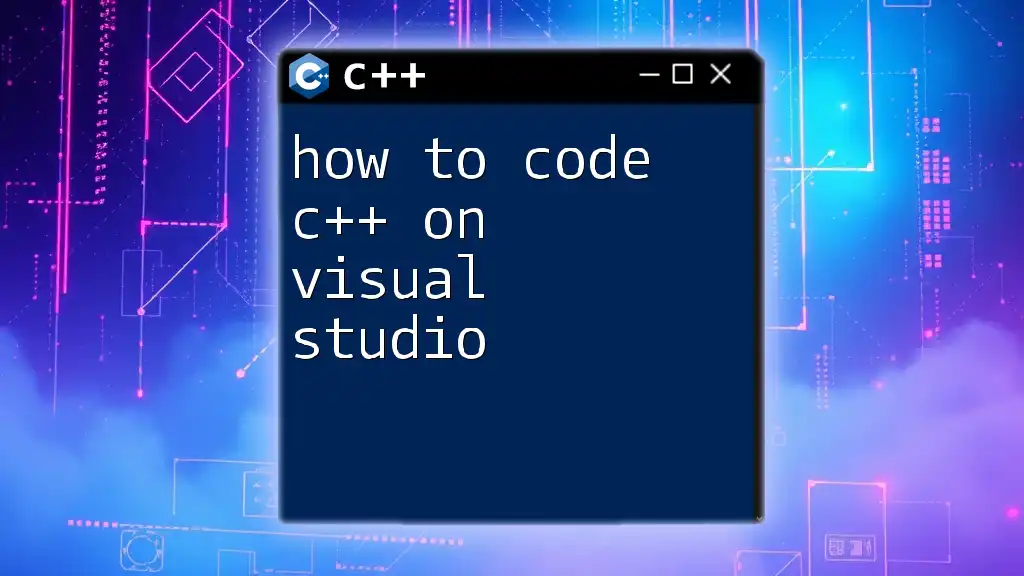
Writing Your First C++ Program
Creating a Simple C++ File
Once your project is set up, you’ll need to create a new C++ file to write your code. In Xcode:
- Navigating to the Project Directory: Right-click on the "Sources" folder, select "New File", and choose "C++ File".
- Sample Code: In your newly created file, write the following simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the Code
The above code demonstrates basic structure in C++:
- Header Files and `#include`: The line `#include <iostream>` includes the Input/Output Stream library, which allows you to use `std::cout` for console output.
- The `main()` function: This is the entry point of every C++ program.
- Outputting Text with `std::cout`: The `std::cout << "Hello, World!" << std::endl;` statement prints the text to the console followed by a new line.
Compiling and Running Your Program
Using Xcode
To run your program in Xcode:
- Click on the "Run" button (the play icon) in the upper left corner. This compiles and executes your program, and you should see "Hello, World!" in the console output area.
Using Terminal
You can also compile and run your C++ code using the Terminal:
- Open Terminal and navigate to your project directory.
- Use the following command to compile your code:
g++ filename.cpp -o outputname
Replace `filename.cpp` with the name of your C++ file, and `outputname` with the desired name for the compiled output. 3. Run your program using:
./outputname
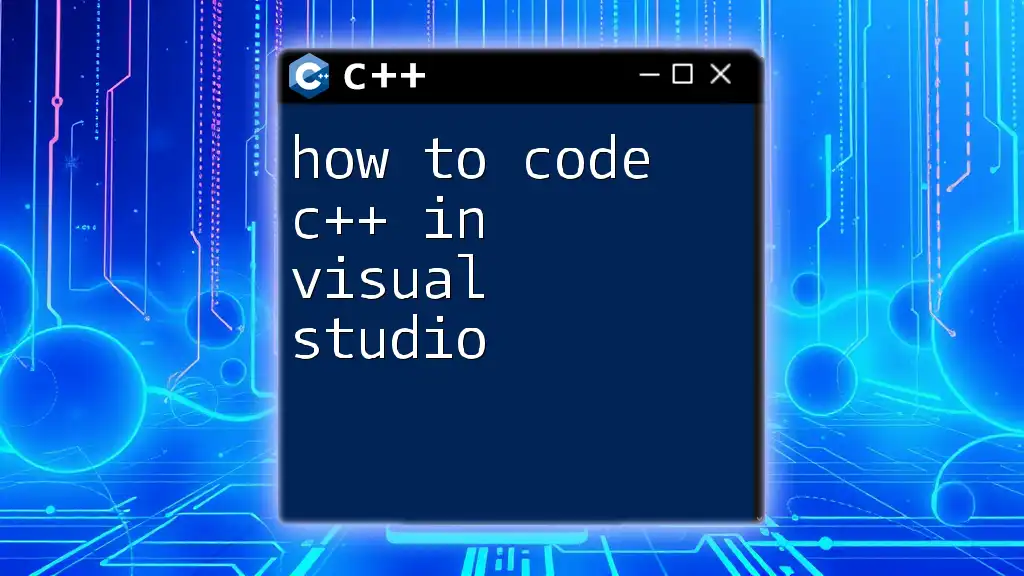
Understanding C++ Commands
Basic Commands and Syntax
Understanding C++ commands and syntax is crucial for effective programming.
Variable Declaration
In C++, variables need to be declared before use. Here’s an example:
int number = 5;
double pi = 3.14;
Control Structures
Control structures include conditionals and loops that guide the flow of your program.
- If Statements:
if (number > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Non-positive number" << std::endl;
}
- Loops:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Advanced C++ Features
To truly become proficient in C++, familiarize yourself with advanced concepts such as functions and classes.
Functions
Functions help to modularize code and promote reusability. Here’s how to declare and use a simple function:
void greet() {
std::cout << "Hello from function!" << std::endl;
}
To call the function, simply use `greet();` within your `main()` function.
Classes
C++ allows for Object-Oriented Programming (OOP), which includes the use of classes. Here’s a basic class structure:
class MyClass {
public:
void display() {
std::cout << "This is a class method." << std::endl;
}
};
To use the class:
MyClass obj;
obj.display();
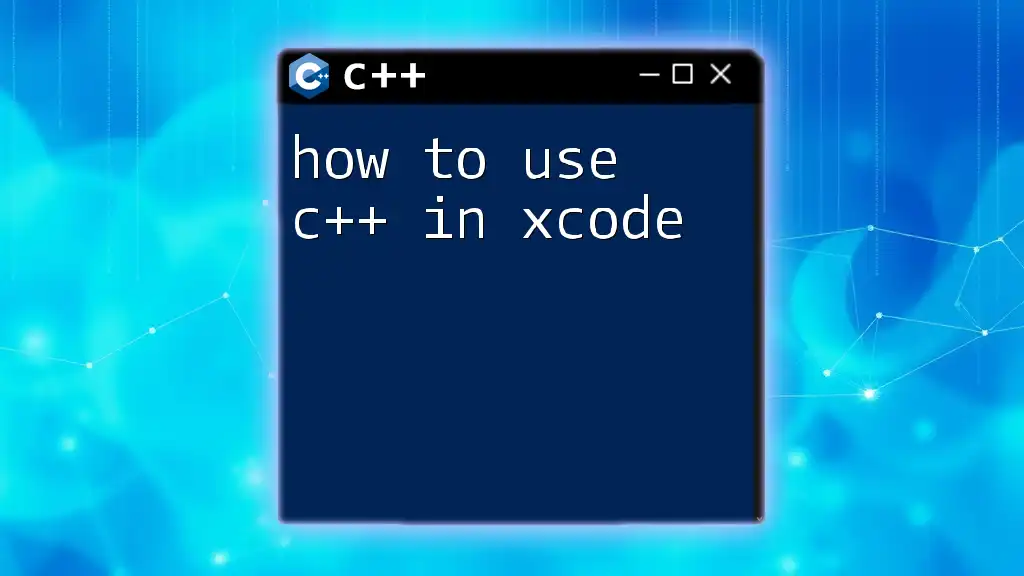
Debugging Your Code
Common C++ Errors
When coding, you may encounter various errors. Understanding the distinction between compilation errors and runtime errors is essential.
- Compilation Errors: These occur when the compiler cannot understand the code; they are usually syntax-related.
- Runtime Errors: These happen during program execution, such as division by zero or accessing array elements out of bounds.
Using Xcode Debugging Tools
Xcode comes with powerful debugging features:
- Setting Breakpoints: Click in the gutter next to the line number where you want execution to pause.
- Inspecting Variables During Runtime: Once execution is paused, hover over variables to view their current values.
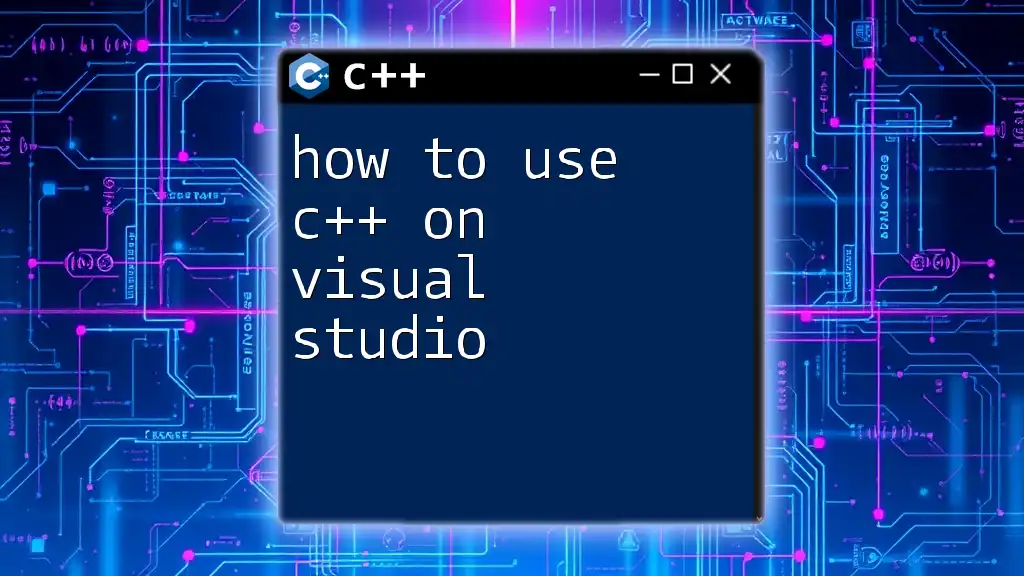
Best Practices for C++ on Mac
Code Organization
Properly organizing your code can enhance readability and maintainability. Make use of header files to separate declarations from definitions, and utilize comments to clarify complex sections of code.
Version Control Systems
Using a version control system like Git is crucial for managing your codebase.
- Introduction to Git: Git is a powerful tool for tracking changes and collaborating with others.
- Setting Up a Git Repository on Your Mac:
- Open Terminal and navigate to your project directory.
- Initialize a new repository using:
git init
This will create a folder named `.git` in your project directory that manages the version control.
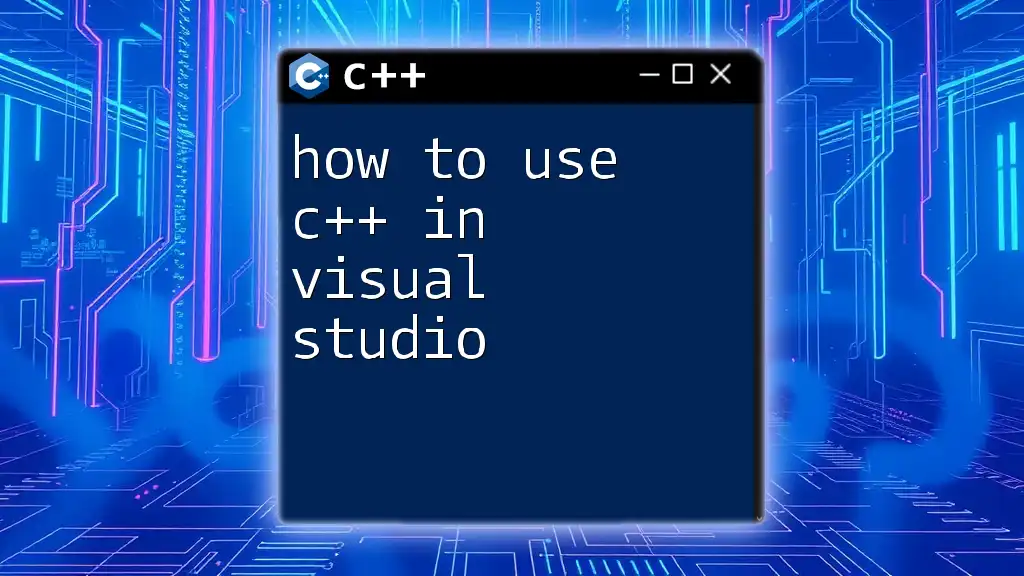
Conclusion
In conclusion, learning how to code C++ on a Mac involves setting up your environment, writing and debugging code, and following best practices. The combination of Xcode and the Terminal provides a strong foundation for developing C++ applications. With dedication and practice, you can enhance your skills and become proficient in C++ programming. Consider exploring additional resources such as books and online courses to further deepen your knowledge in this powerful language.