To code C++ in Visual Studio, simply create a new project, select the C++ template, and write your code in the main file; here's a basic example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio for C++
Downloading and Installing Visual Studio
To get started with coding C++ in Visual Studio, you'll first need to download and install the software. Visual Studio offers several editions: Community (free), Professional, and Enterprise. For most individual learners and small teams, the Community edition is sufficient.
Ensure your system meets the necessary requirements, which include a compatible Windows operating system and enough memory and storage space.
To download Visual Studio, follow these steps:
- Navigate to the [official Visual Studio website](https://visualstudio.microsoft.com).
- Select the Community edition, and click on the Download button.
- Once the installer is downloaded, run it. During installation, you'll be prompted to select Workloads. For C++ development, ensure to check the "Desktop development with C++" option.
It’s vital to enable all essential C++ features during this process. Look for options related to C++ libraries and tools for a complete setup.
Configuring Your Environment
Once Visual Studio is installed, it's crucial to configure the C++ development environment for optimal use.
Begin by ensuring that the C++ development workload is properly selected. You may also want to explore available extensions that can enhance your coding experience, such as productivity tools or additional libraries.
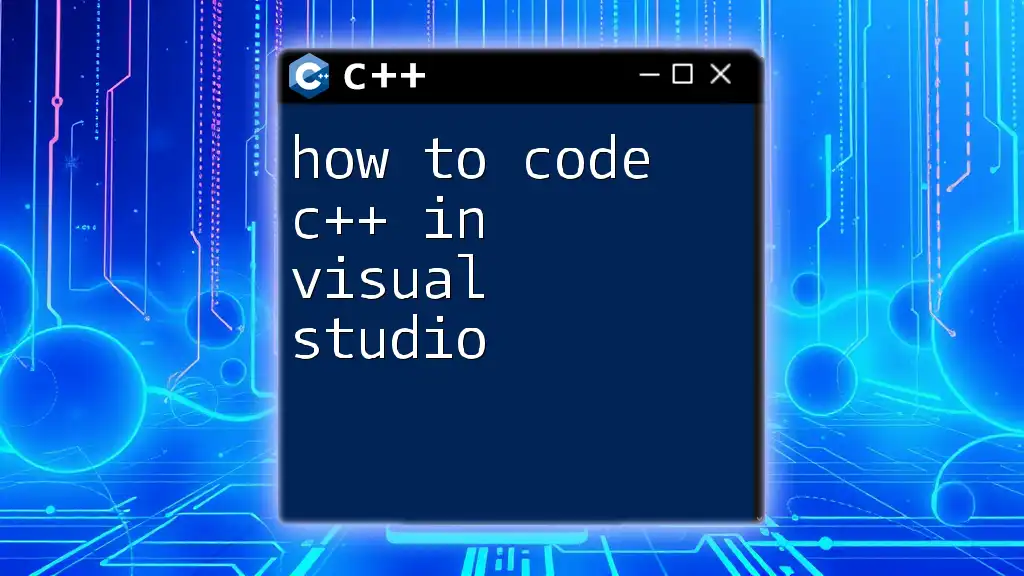
Creating a New C++ Project
Starting a New Project
Creating your first C++ project is a straightforward process in Visual Studio. To do so:
- Upon opening Visual Studio, you will be greeted with the Start Screen. Here, select "Create a new project."
- In the project template selection window, filter by C++ and choose the Console App template.
- You'll be prompted to name your project and decide its location on your drive. Choose a clear and descriptive name, as this will help you identify your project later.
Understanding Project Structure
Once your project is created, you will notice several components in the Solution Explorer. The most common file you'll be working with is `main.cpp`, which contains the entry point of your program.
It's essential to understand that Visual Studio organizes files into a hierarchy. You can create additional folders for headers, resources, or other related files, which helps keep your project organized.
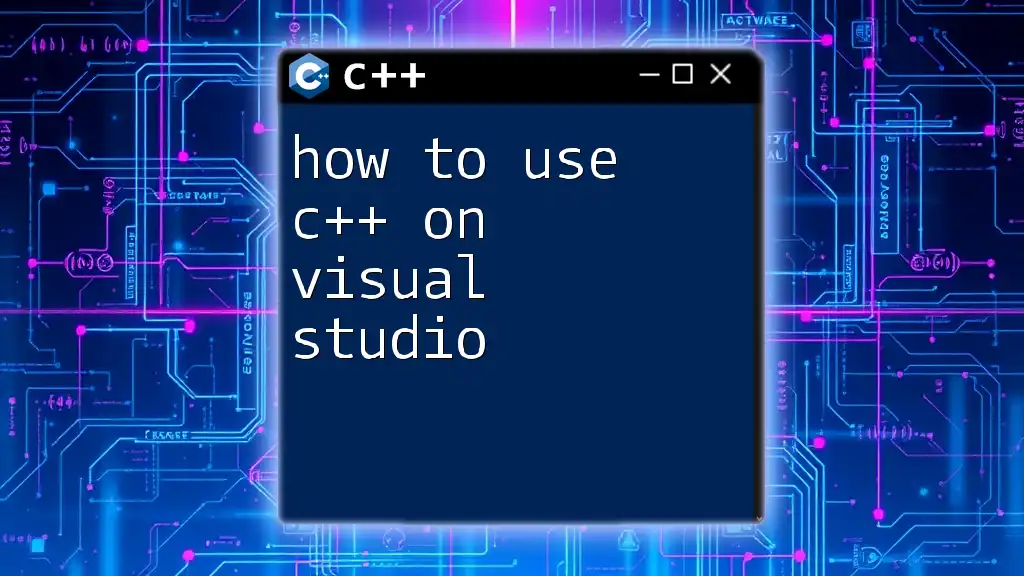
Writing Your First C++ Program
Writing the Code
Now that your project is created, it’s time to write your first line of C++ code! Start by opening `main.cpp`, and input the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program does a fundamental task: it prints "Hello, World!" to the console.
When coding in Visual Studio, take advantage of the auto-completion and IntelliSense features, which will provide suggestions and help you quickly navigate your code.
Compiling and Running the Program
With your code written, you can now compile and run the program. First, understand the difference between building and running your application. Build compiles your code into an executable, while Run executes the compiled application.
To run your program, navigate to the Build menu and select Build Solution. After a successful build, click on Start Debugging or press `F5` to run your application. You'll see the output in the console window!
Additionally, familiarize yourself with Debug and Release modes. Debug mode assists in finding bugs by including additional debug information, while Release mode produces an optimized binary suitable for deployment.
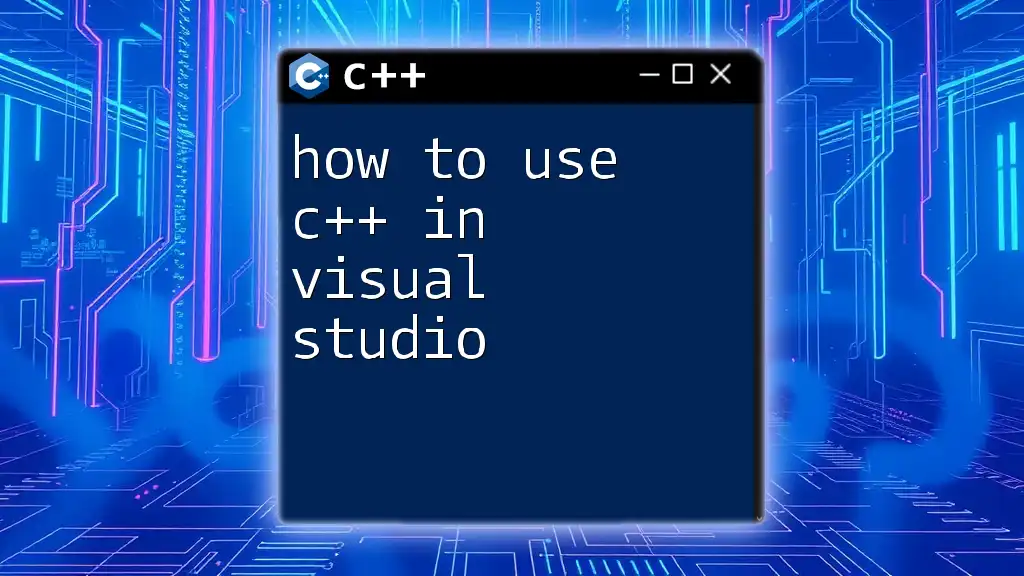
Debugging Your Code
Setting Breakpoints
Debugging is an essential skill for every developer. In Visual Studio, you can set breakpoints, which are markers that tell the debugger to pause execution so you can inspect your code's state.
To set a breakpoint, click in the left margin next to the line number in your code editor. When you run the debugger, execution will halt at those points, allowing you to step through your code line by line.
Watching Variables
Using the Watch Window is incredibly beneficial during debugging. This window allows you to monitor the values of specific variables as your program executes. To add a variable to the watch list, right-click the variable in your code and select Add Watch. This way, you can track its value at various points during runtime.
Step Through Code
While debugging, you can control the flow of execution. Learn to use the debugger commands: Step Into (F11), Step Over (F10), and Step Out. These commands let you explore your code more deeply, providing insight into how each function is executed or how variables are manipulated.
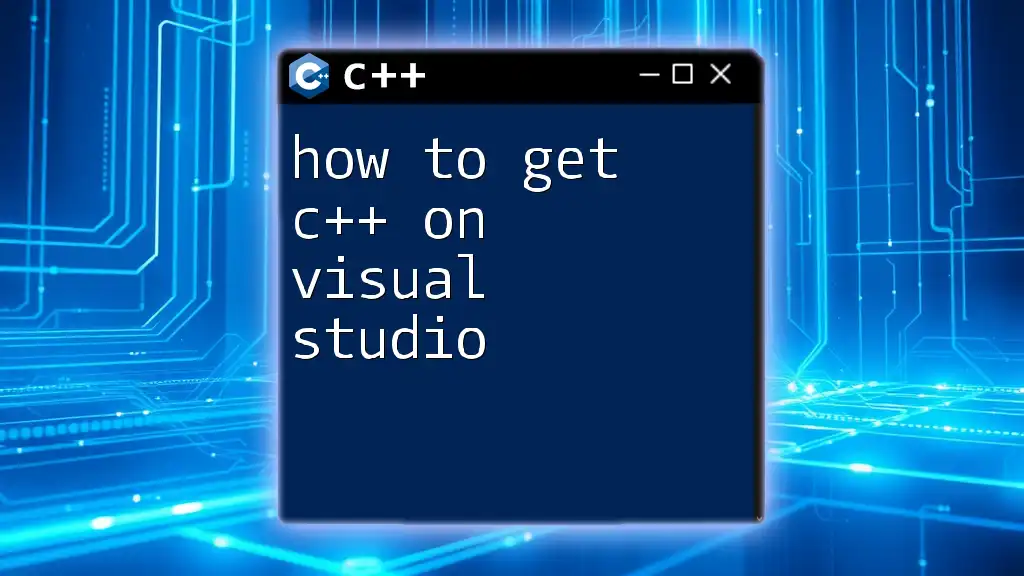
Advanced C++ Features
Using Libraries
C++ offers a rich set of libraries to extend functionality. You can easily include libraries within your project by using the `#include` directive at the top of your file. For example, let’s use the STL vector library:
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
// Additional code can sort or manipulate the 'numbers' vector here.
return 0;
}
This code snippet initializes a vector of integers, showcasing how to manage collections of data effectively.
Object-Oriented C++ Programming
C++ supports object-oriented programming (OOP), a paradigm that allows you to define objects and their behaviors. Here's a simple class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // This will print "Woof!"
return 0;
}
This class encapsulates the properties and behaviors of a dog, demonstrating the fundamentals of OOP. Learning to use classes effectively can significantly improve the structure and reusability of your code.
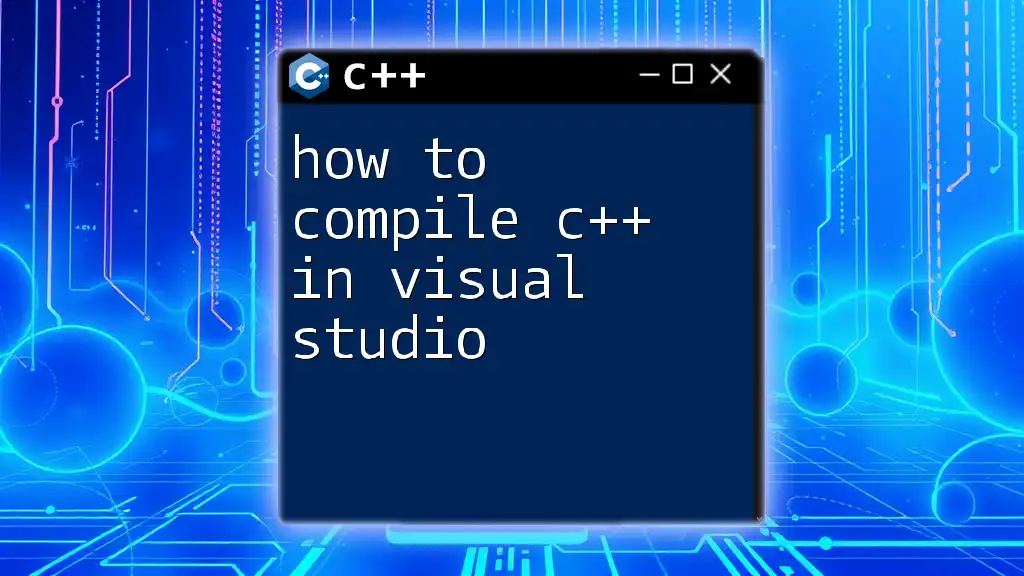
Tips for Effective C++ Development in Visual Studio
Code Formatting and Style
Clean code practices not only enhance readability but also maintainability. Visual Studio allows you to format your code automatically. Use the Format Document option (or shortcut `Ctrl + K, Ctrl + D`) to keep your code neat and organized. Remember to maintain consistent spacing, indentation, and commenting throughout your code.
Leveraging Extensions and Tools
Enhancing your development environment with extensions can boost productivity. Some popular extensions include Visual Assist, which provides improved code navigation and refactoring tools, and ReSharper, which offers advanced code analysis. Explore the Visual Studio marketplace to find tools that suit your workflow.
Resources for Further Learning
As you continue to explore how to code C++ on Visual Studio, consider various online resources for additional support. Websites like [Microsoft Docs](https://docs.microsoft.com/en-us/cpp/?view=msvc-160) provide comprehensive documentation on C++ features, while community forums such as Stack Overflow can offer quick answers to specific questions.
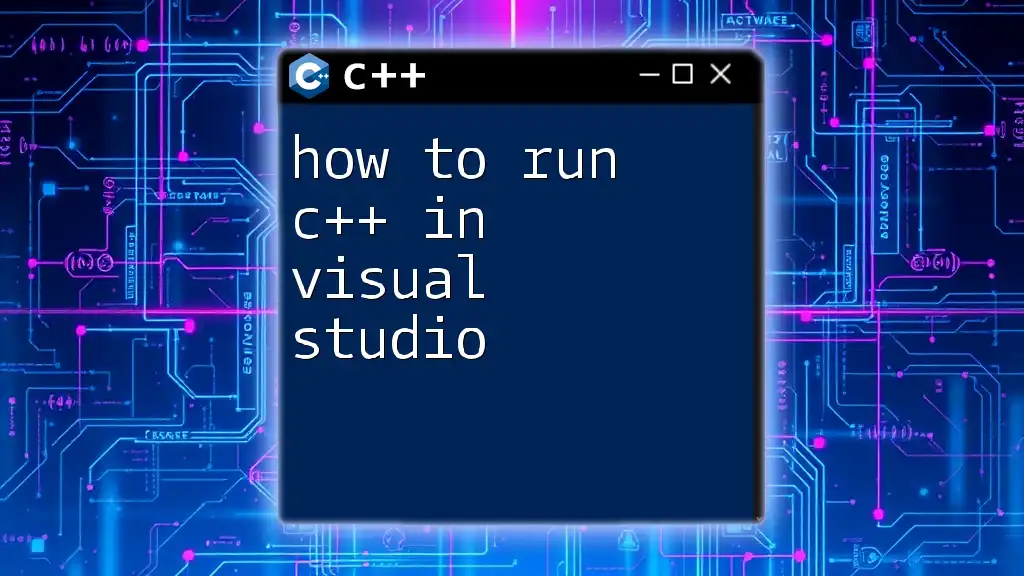
Conclusion
By following these steps, you have laid the groundwork for your journey in coding C++ on Visual Studio. Remember that practice is key, so continue to write and debug code regularly. As you advance, explore more complex topics within C++ and embrace the challenges that come with learning this powerful programming language. Happy coding!