To run a C++ code in Visual Studio, create a new project, write your code in the editor, and then build and run it using the "Local Windows Debugger" option.
Here's a simple C++ code snippet for demonstration:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio
Downloading and Installing Visual Studio
To get started with how to run a C++ code in Visual Studio, the first step is to download and install Visual Studio itself. You can find the IDE on the official Microsoft Visual Studio website. Simply navigate to the site and select the version suitable for your needs. Most users will opt for the free Community version, which provides all the essential features for C++ development.
Once you've downloaded the installer, run it. During installation, you will be greeted with a setup wizard that walks you through the necessary steps. Make sure to select "Desktop development with C++" from the list of workloads, which includes all the tools required for building C++ applications.
Selecting the Right Workload
When the installer prompts you for workloads, choosing "Desktop development with C++" is crucial if you're looking to write Windows applications or console apps using C++. This selection will install the C++ compiler, libraries, and toolset necessary to build and run C++ applications effectively.
After clicking "Install," the setup process will take some time to download and install all the selected components.
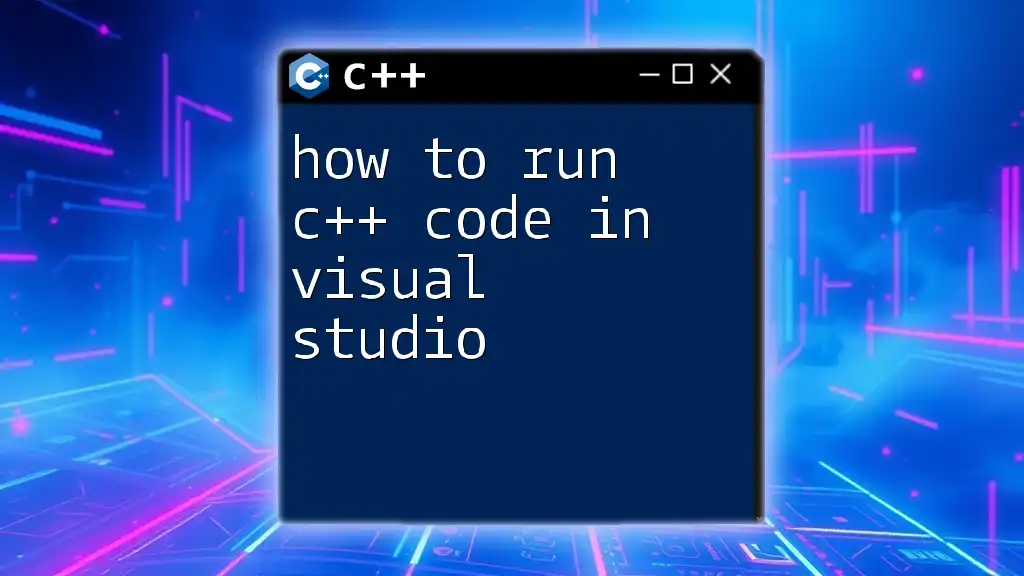
Creating a New C++ Project
Launching Visual Studio
Once your installation is complete, open Visual Studio. You'll be met with a start page displaying various options including new file creation, project templates, and more.
Starting a New Project
To create your first C++ project, click on "Create a new project." This will take you to a new window where you can filter project types to focus specifically on C++.
When the project template selection appears, you can use search filters to help narrow down your choices. The template we will focus on is the Console App, which is ideal for beginners wanting to learn how to run a C++ code in Visual Studio.
Choosing a C++ Project Template
After filtering the templates, look for "Console App." This template allows you to create a command-line application where you can easily input and execute C++ code. Click on it, then proceed to click "Next."
You will then be prompted to name your project, choose a location to save it, and select the solution name. After filling these out, click "Create."
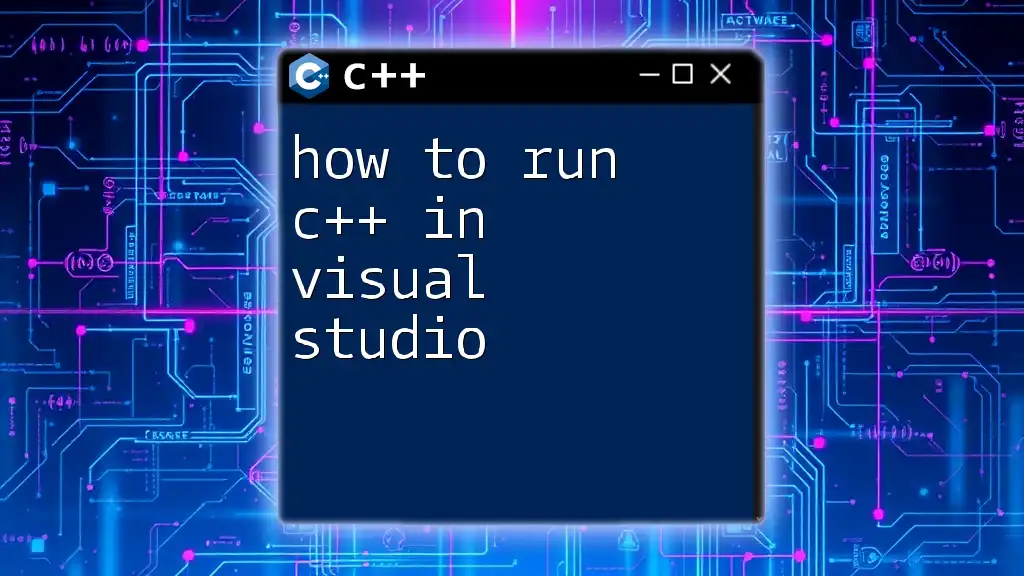
Writing Your First C++ Code
Understanding the IDE Text Editor
Once your project is created, Visual Studio will open the default source file (usually named `main.cpp`). The IDE features a text editor that provides syntax highlighting, making it easier to read and write code. You'll also benefit from auto-completion that helps prevent syntax errors as you code.
Sample C++ Code
Now let’s write a simple C++ program. Here's the classic "Hello, World!" code snippet:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation:
- `#include <iostream>` is a preprocessor directive that includes the standard input/output stream library.
- `using namespace std;` allows you to use elements from the standard namespace without prefixing them.
- `int main()` defines the main function where execution begins.
- The line `cout << "Hello, World!" << endl;` prints the text to the console and moves the cursor to the next line.
- Finally, `return 0;` signifies that the program has ended successfully.
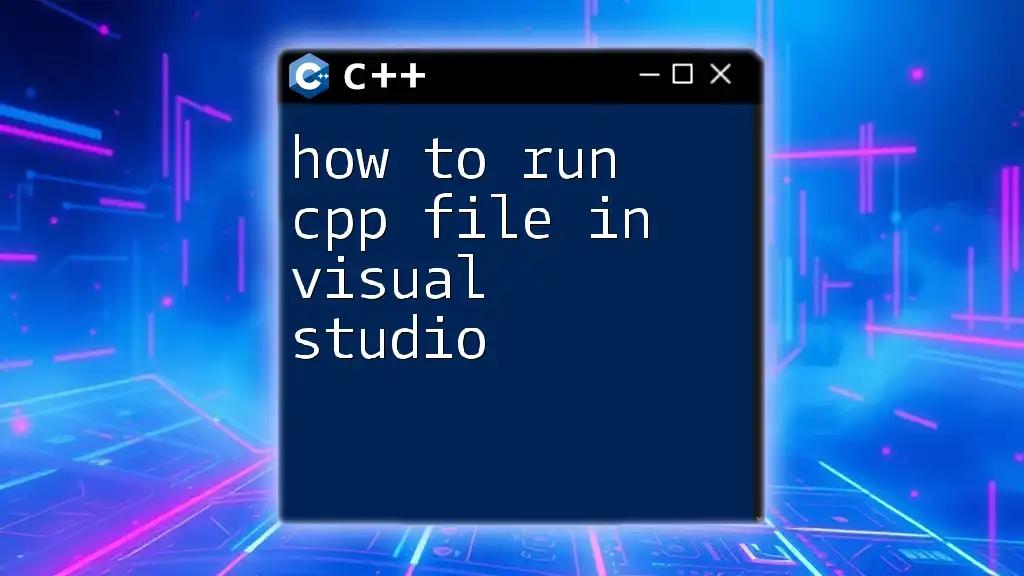
Building Your Project
Understanding the Build Process
Before you can run your C++ code, you must build the project. Building involves compiling your code into an executable format that the operating system can run.
Building the Project in Visual Studio
To build your project, navigate to the Build menu at the top and select Build Solution. Alternatively, you can use the shortcut `Ctrl + Shift + B`. The Output Window will display messages indicating whether the build was successful or if any errors occurred.
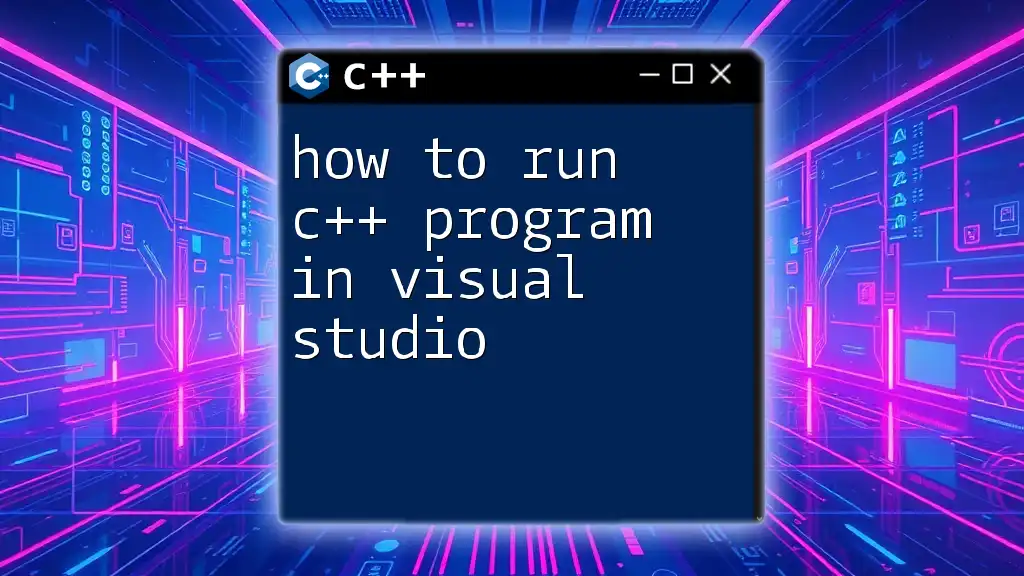
Running Your C++ Code
Executing Your Program
To run the program after successfully building it, you have two primary options:
- Select Debug > Start Without Debugging from the menu or
- Press the shortcut `Ctrl + F5`.
Both actions will execute the application, and you should see "Hello, World!" printed in the console window.
Understanding Output
As a new C++ programmer, understanding how to read the console output is essential. If there are any issues in your code, errors will also appear here, guiding you to improve your program.
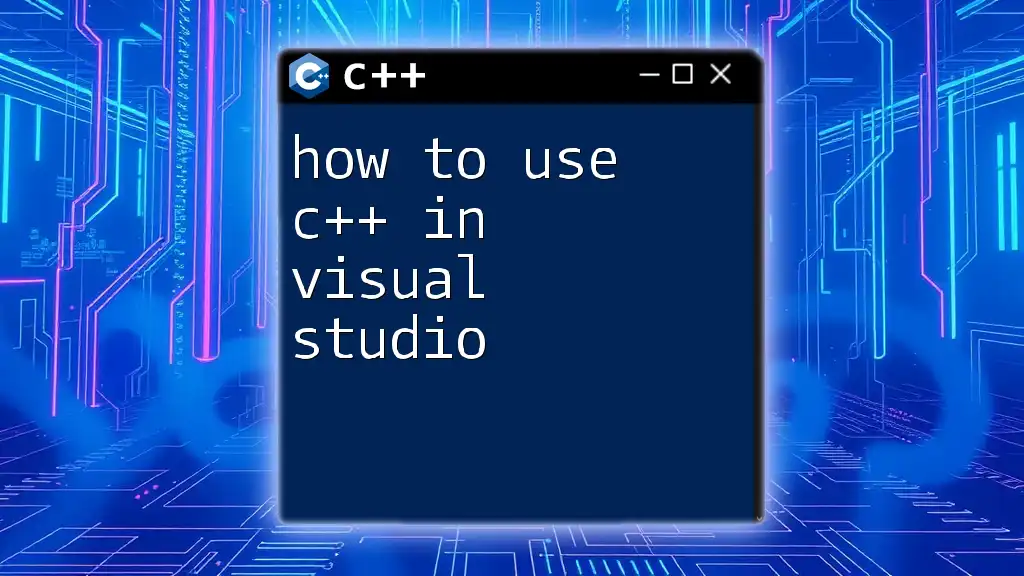
Debugging Your Code
What is Debugging?
Debugging is the process of identifying and removing bugs or errors in your code. Understanding how to run a C++ code in Visual Studio effectively requires familiarity with debugging because it is crucial to developing functional software.
Using Breakpoints
Breakpoints allow you to pause execution at specific lines of code, making it easier to inspect the state of your application. To set a breakpoint, simply click in the margin to the left of the line number where you want execution to stop. When you run your code in debug mode (by pressing F5), the program will halt execution at that line, allowing you to examine variable values and step through your code line-by-line.
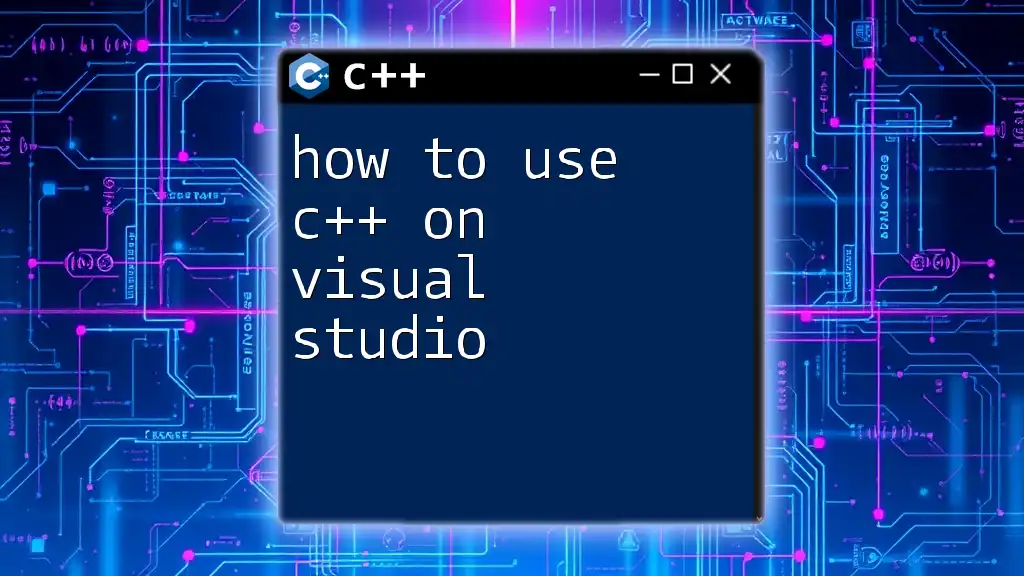
Common Issues and Solutions
Troubleshooting Build Errors
As a beginner, encountering build errors is common. Errors often arise from syntax mistakes, missing libraries, or improper configurations. Pay attention to the error messages in the Output Window, as they provide hints for resolving issues. Simple mistakes, like forgetting to put a semicolon at the end of a statement, can trigger build failures.
Console Window Issues
If you notice that the console window disappears immediately after running your program, it can be challenging to see the output. To prevent this, ensure you run the program using `Ctrl + F5` instead of F5. This will keep the console open until you press a key, giving you time to review the results.
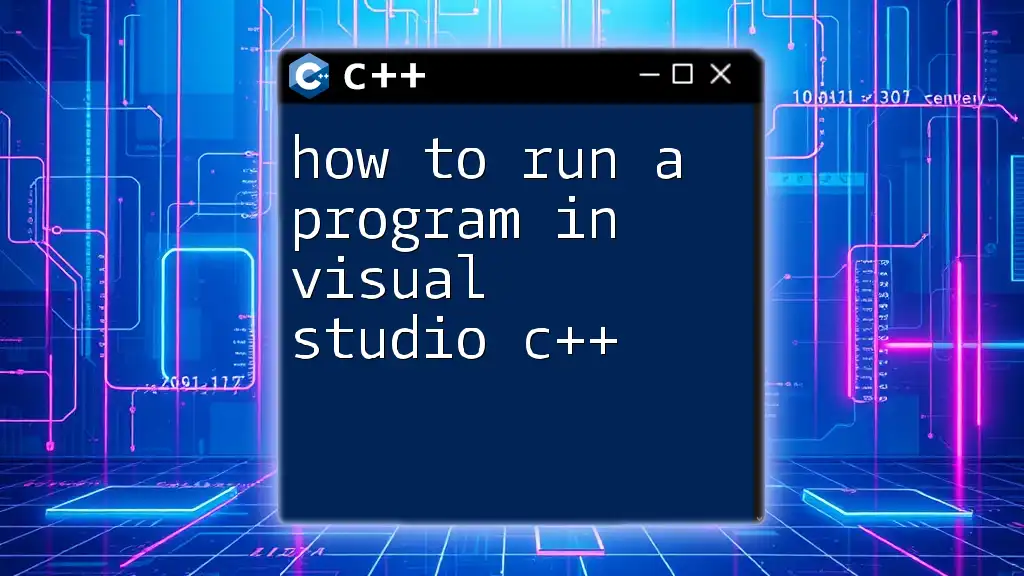
Conclusion
Learning how to run a C++ code in Visual Studio is a stepping stone into the world of programming. By following the outlined steps, you can efficiently set up projects, write code, build applications, and troubleshoot common issues. With continued practice, you'll enhance your skills and confidence in C++ development within Visual Studio, leading you toward more complex coding endeavors.
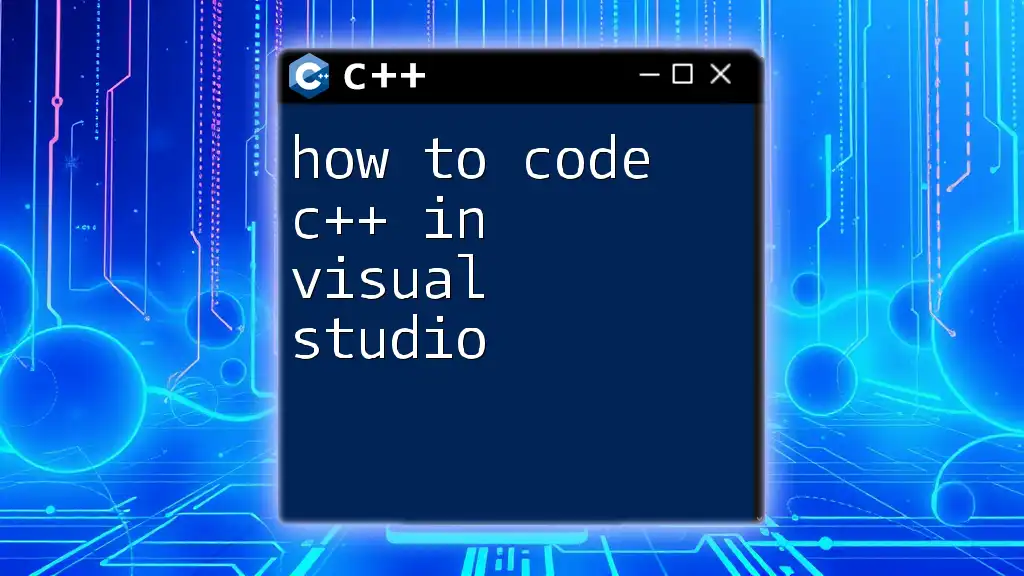
Additional Resources
To further strengthen your understanding, consider exploring additional resources such as C++ documentation, coding challenge platforms, or community forums dedicated to C++. These can provide exercises and support as you continue your programming journey.