To execute a C++ program in Visual Studio, simply create a new project, write your C++ code in the main file, and then press `Ctrl + F5` to compile and run it without debugging.
Here's a basic example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio
Choosing the Right Version
When starting with C++ programming, it’s crucial to choose an appropriate version of Visual Studio. There are three main options available: Community, Professional, and Enterprise. For beginners and those who are just learning how to execute a C++ program in Visual Studio, the Community version is the best option. It offers a fully-featured integrated development environment (IDE) at no cost and is ideal for students, open-source projects, and individual developers.
Installation Process
Once you've chosen your version, the next step is installation. Here are the steps to guide you through the process:
- Download Visual Studio: Go to the official Visual Studio website and select the Community version.
- Select C++ Development Tools: During the installation process, you'll be presented with different workload options. Ensure you check the box for Desktop development with C++ to install the necessary tools and libraries.
- Verify Installation: After installation, launch Visual Studio to confirm that all components are set up correctly.
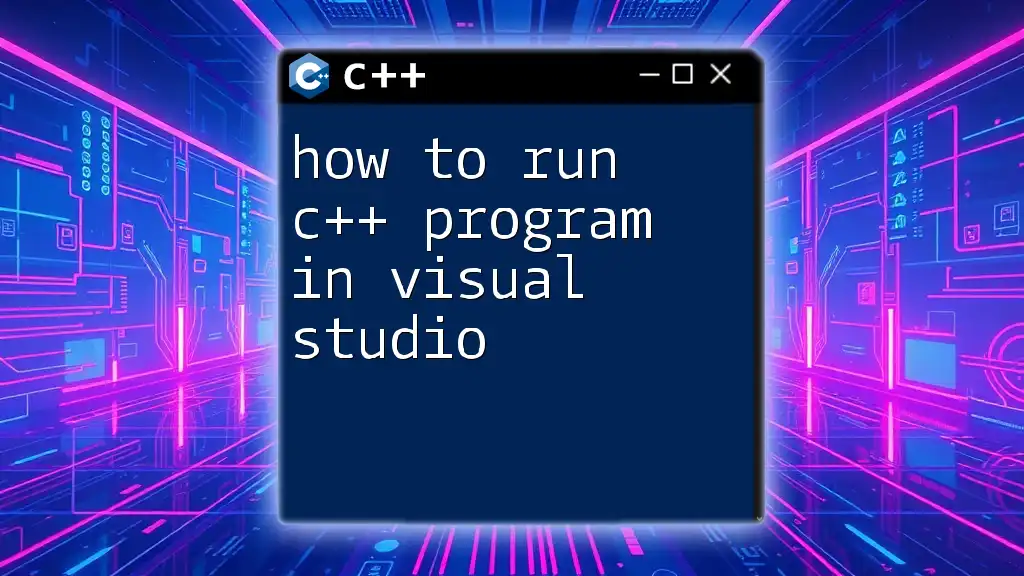
Creating a New C++ Project
Launching Visual Studio
Start by opening Visual Studio from your desktop or application menu. You may see a welcome screen that provides you with options for creating new projects or opening existing ones.
Starting a New Project
To create a new C++ project, follow these steps:
- Click on "Create a new project."
- In the project template window, use the search bar or filter options to locate C++ templates.
- Choose the Console App template for a simple command-line application. This is typically the best choice for beginners wanting to execute a C++ program.
Configuring the Project
After selecting the template, you'll need to configure your project settings:
- Project Name: Enter a meaningful name for your project. For instance, "HelloWorld."
- Location: Choose a directory on your computer where you want to save the project files.
- Solution Properties: You can leave the defaults for solutions and configurations unless you have specific needs (most new users will).
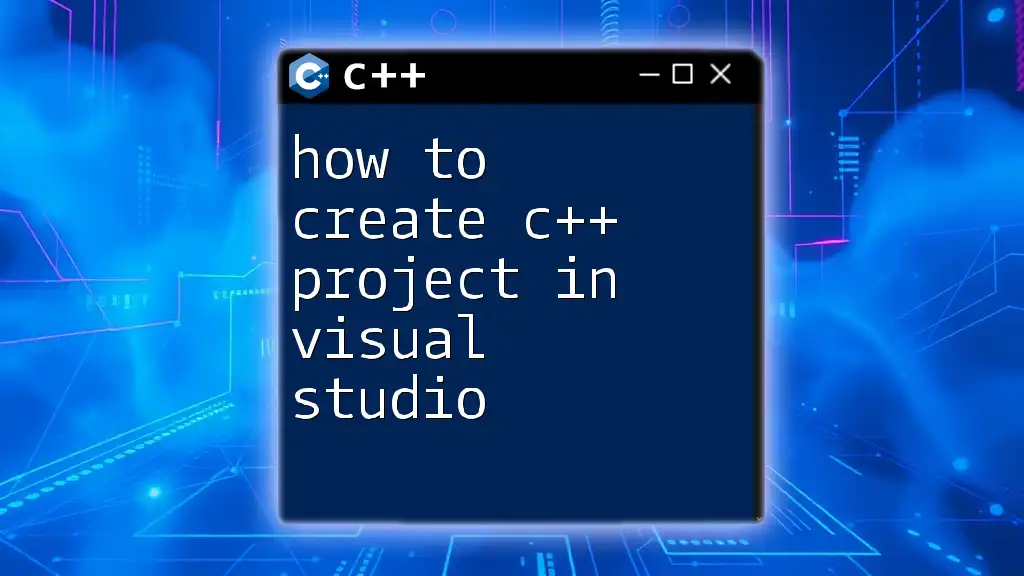
Writing Your First C++ Program
Code Structure Overview
Every C++ program must include a specific structure to be executable. Understanding this basic format is essential. Here’s a basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` allows you to use input and output features in C++.
- `int main()` defines the starting point of your program.
- `std::cout` is used to output text to the console, while `return 0;` signifies the successful termination of the program.
Adding Code in Visual Studio
Now that you've configured your project, it’s time to enter your code:
- Locate the Solution Explorer on the right side of the screen.
- Open the source file often named, `main.cpp`, depending on your project setup.
- Copy and paste the example code above into the code editor and feel free to modify it to suit your learning.
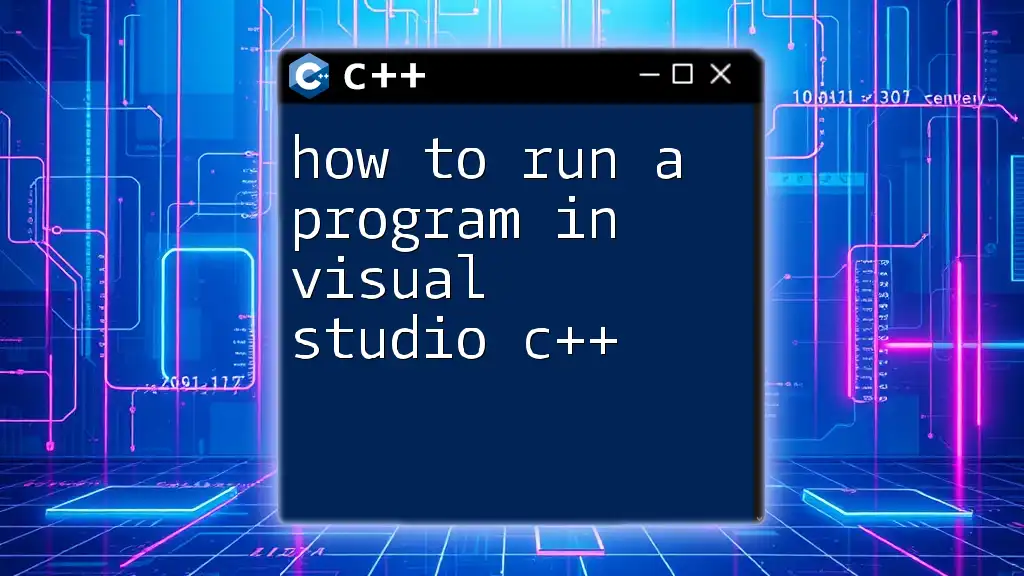
Executing the C++ Program
Building the Project
Before you can run your program, you must build it:
- Navigate to the Build menu at the top of Visual Studio.
- Select Build Solution (or simply press Ctrl + Shift + B). This step compiles your code and checks for errors. If there are issues, they will be displayed in the Error List window.
Running the Program
After successfully building your project, it’s time to execute it:
- Click the green "Start" button located at the top of Visual Studio. Alternatively, you can press F5 to run your program. The console window will appear displaying the output of your program. In this case, it should show "Hello, World!".
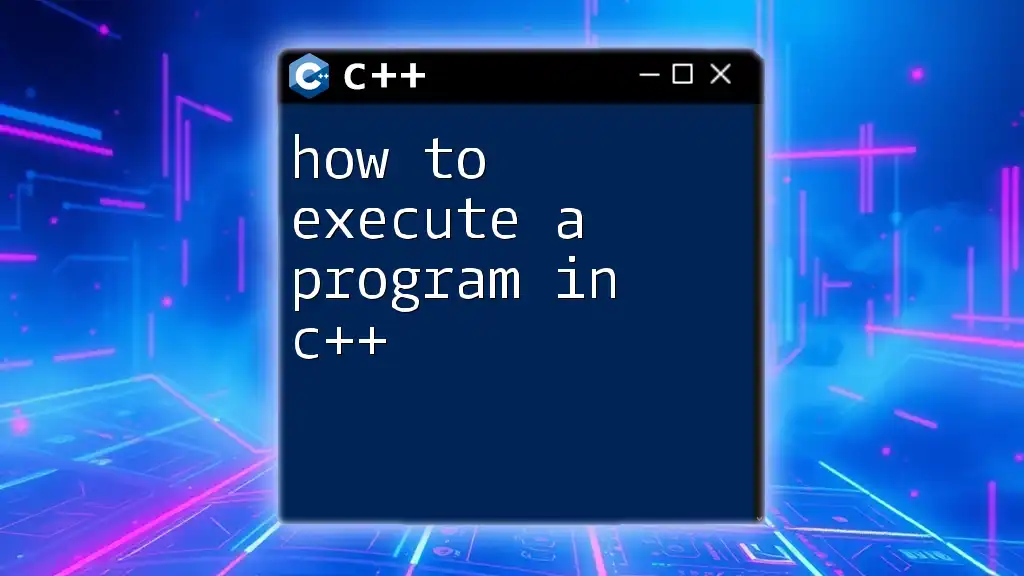
Debugging Your Code
What is Debugging?
Debugging is the process of identifying and correcting errors in your code. In Visual Studio, a robust debugging environment is available, allowing you to run your program step-by-step to pinpoint issues.
Setting Breakpoints
Breakpoints are markers that you set in your code to pause execution:
- Click to the left of the line numbers in your code editor to set a breakpoint (a red dot will appear).
- To start debugging, click the Start Debugging option from the Debug menu or press F5.
With the program paused at your breakpoint, you can inspect variable values and code flow.
Analyzing Output
As you debug, utilize the Output window to read debug information. This insight can help you adjust your program and fix bugs more effectively.
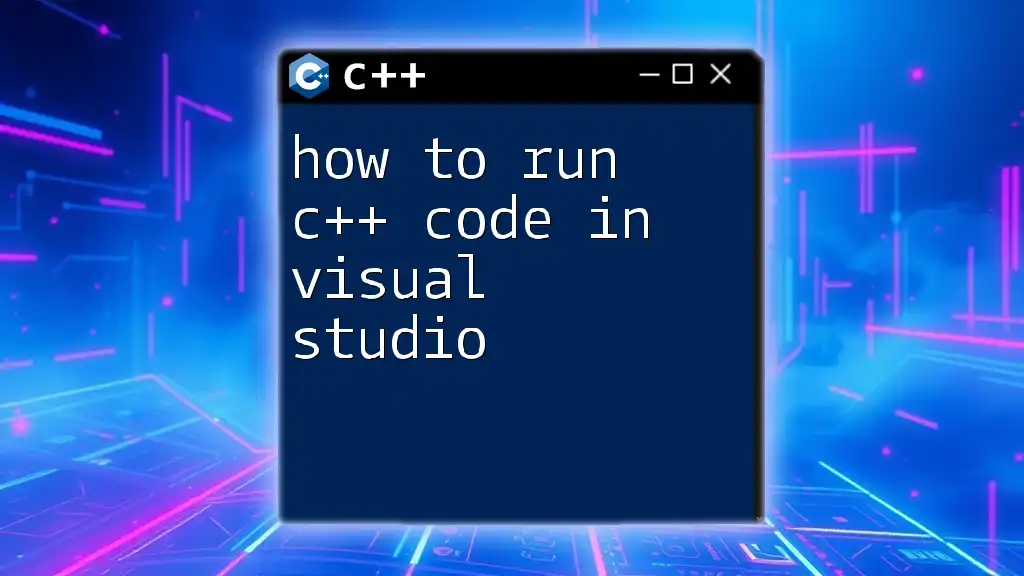
Common Issues and Troubleshooting
Compilation Errors
Compilation errors can stem from various issues, from syntax mistakes to linking problems. Common examples include:
- Missing semicolons at the end of lines
- Incorrectly spelled keywords or variable names
- Mismatched braces or parentheses
It’s essential to read the error messages carefully, as they often indicate the location and nature of the issue.
Runtime Issues
Runtime issues occur when the program compiles successfully but fails during execution. This can include:
- Null pointer dereference: Trying to access a resource that hasn’t been allocated.
- Array out of bounds: Accessing elements outside the defined array limits.
To troubleshoot, utilize the debugger to examine the flow of execution and understand where the program fails.
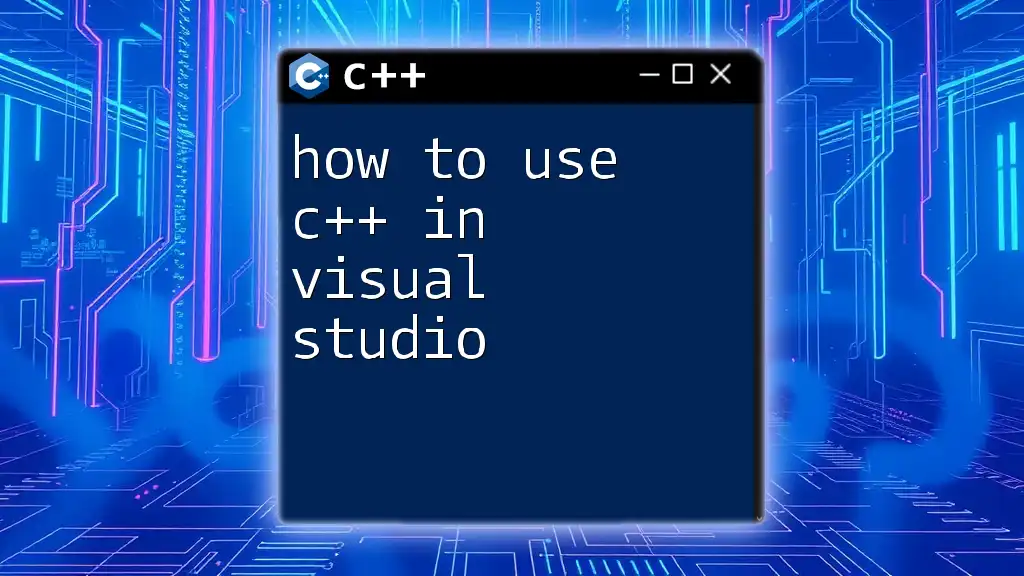
Tips for Efficient C++ Programming in Visual Studio
Writing Clean Code
Writing clear and maintainable code is crucial for any developer. Here are a few best practices:
- Comment your code: Explain complex logic or key sections to improve readability for yourself and others.
- Use meaningful names: Choose descriptive variable names that indicate their purpose.
Utilizing Extensions and Tools
Visual Studio supports numerous extensions to enhance your development experience. Some highly recommended ones include:
- Visual Assist: Provides powerful refactoring tools and improved navigation.
- CodeMaid: Helps clean up and maintain your code layout.
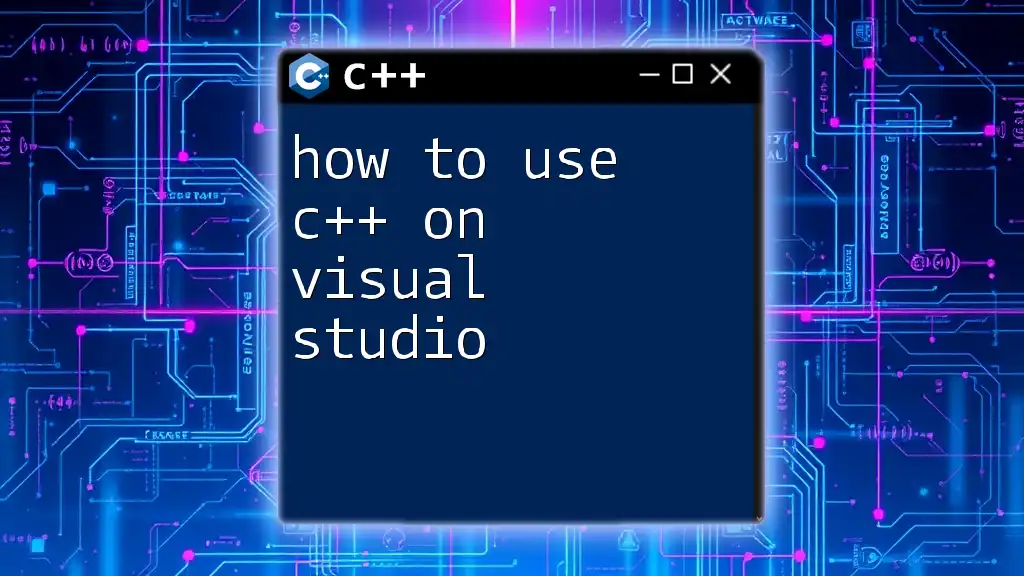
Conclusion
Mastering how to execute a C++ program in Visual Studio is a fundamental skill for aspiring developers. This guide has walked you through the installation, project setup, coding, debugging, and troubleshooting necessary to get you started. Don’t hesitate to continue exploring C++ programming, as there is a vast array of tools and techniques that can enhance your coding repertoire!
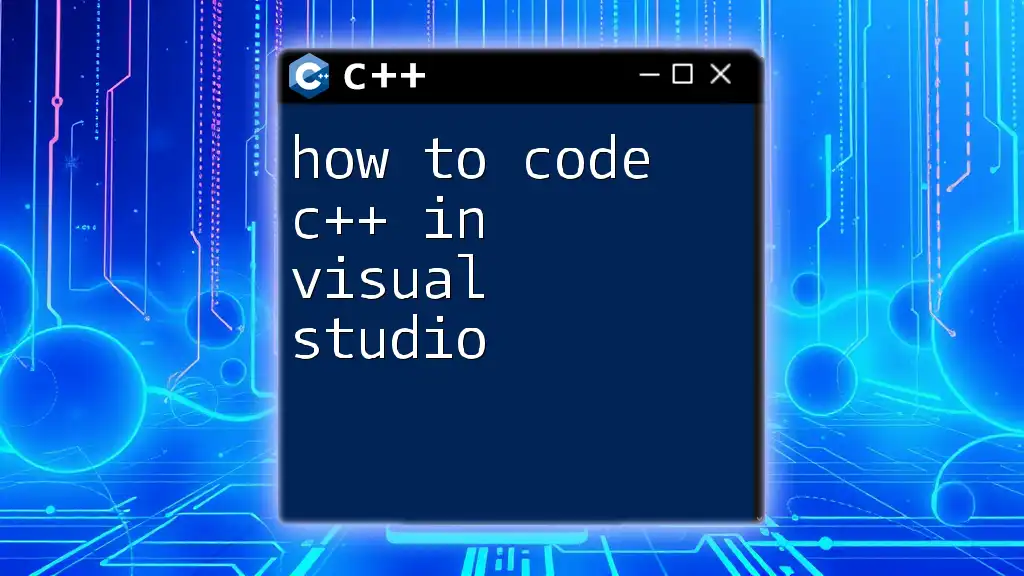
Additional Resources
To further your understanding of C++ programming and Visual Studio, consider exploring the official documentation, engaging in online courses, or reading recommended books. Join the community to stay updated and motivated in your programming journey!